Spring Boot Service Discovery with Eureka
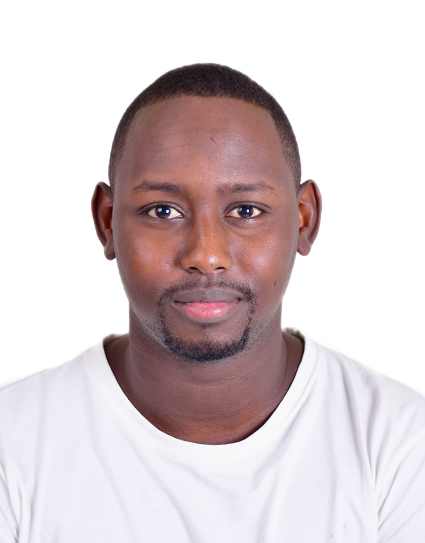
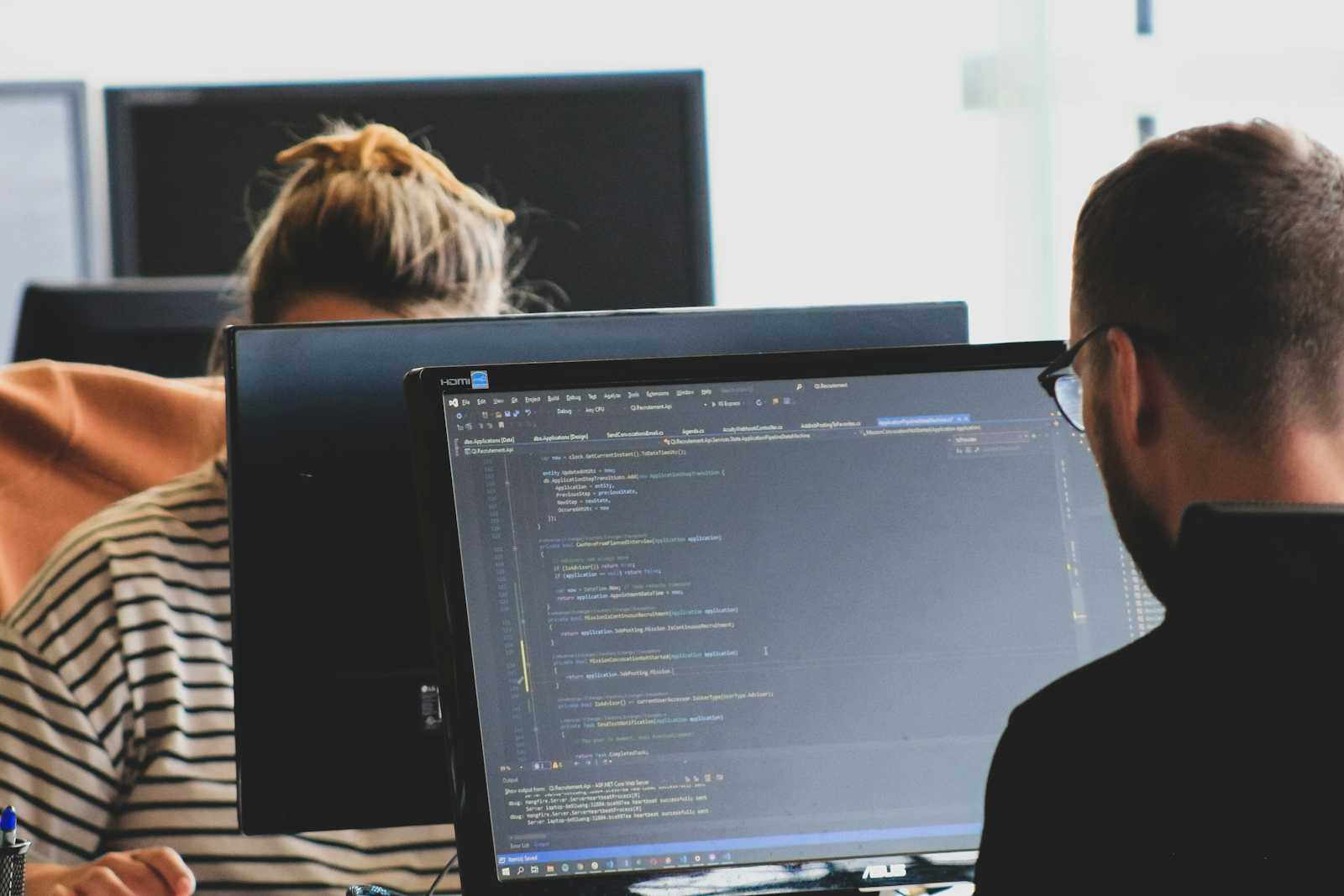
Introduction
In a microservices architecture, where applications are composed of multiple independent services, service discovery becomes a crucial part of managing communication between services. Eureka, developed by Netflix, is a service discovery tool that allows services to dynamically register themselves and discover other services. Spring Boot, with the Spring Cloud Netflix library, provides seamless integration with Eureka, making it easier to implement service discovery in your microservices-based applications. In this article, we'll explore the importance of service discovery, the role Eureka plays, and how to set up a Spring Boot application with Eureka.
Advantages of Service Discovery
Service discovery offers the following benefits in a microservices architecture:
Dynamic Scaling: New services can be added or removed from the system, and other services will dynamically discover these changes without any manual intervention.
Resilience: Service discovery helps to manage failover by directing requests to healthy instances of a service, ensuring availability.
Decoupling: Services can interact with one another without needing to know about their concrete network locations, allowing more flexibility and easier maintenance.
Load Balancing: Eureka, combined with Ribbon (a client-side load balancer), allows distributing requests across multiple instances of a service, improving performance and reducing bottlenecks.
Eureka Server vs. Eureka Client
Eureka Server: The Eureka Server acts as a registry where all microservices (Eureka clients) register themselves. It serves as the "phone book" for the system, maintaining information about service instances and their health.
Eureka Client: A Eureka Client is any microservice that registers with the Eureka Server. The client also has the ability to discover other services registered on the server. Spring Boot applications that are Eureka clients can easily communicate with one another via the service registry.
Code Example
This section demonstrates setting up a Eureka Server and a Eureka Client (a Spring Boot service) for service discovery.
Eureka Server
pom.xml (dependencies)
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-server</artifactId>
</dependency>
</dependencies>
Main Application Class
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
@SpringBootApplication
@EnableEurekaServer // Enable Eureka Server
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
application.yml
server:
port: 8761 # Eureka Server typically runs on port 8761
eureka:
client:
register-with-eureka: false # Eureka Server does not need to register itself
fetch-registry: false
server:
wait-time-in-ms-when-sync-empty: 0
To run the Eureka Server:
- Build and start the application, and the Eureka dashboard will be available at
http://localhost:8761
.
Eureka Client (Spring Boot Service)
pom.xml (dependencies)
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
Main Application Class
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
@SpringBootApplication
@EnableEurekaClient // Enable Eureka Client
public class MyServiceApplication {
public static void main(String[] args) {
SpringApplication.run(MyServiceApplication.class, args);
}
}
application.yml
server:
port: 8081 # Port for this service
spring:
application:
name: my-service # Name of the service registered with Eureka
eureka:
client:
service-url:
defaultZone: http://localhost:8761/eureka/ # Pointing to the Eureka server
instance:
prefer-ip-address: true # Optional: to register with IP instead of hostname
Service Discovery in Action Once the Eureka Server is running and the Eureka Client (my-service) is started, it will automatically register with the Eureka Server, making it discoverable by other services.
You can access the Eureka Server dashboard at http://localhost:8761
to see the registered services.
Conclusion
Service discovery is a foundational piece in a microservices architecture, helping services find and communicate with each other dynamically. Eureka simplifies this process by acting as a registry for all microservices in the system. With Spring Boot’s integration, you can easily set up both Eureka Servers and Clients, enabling dynamic discovery, load balancing, and fault tolerance in your applications. Implementing service discovery with Eureka not only improves flexibility and scalability but also ensures resilience in microservice interactions.
Subscribe to my newsletter
Read articles from Muhire Josué directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
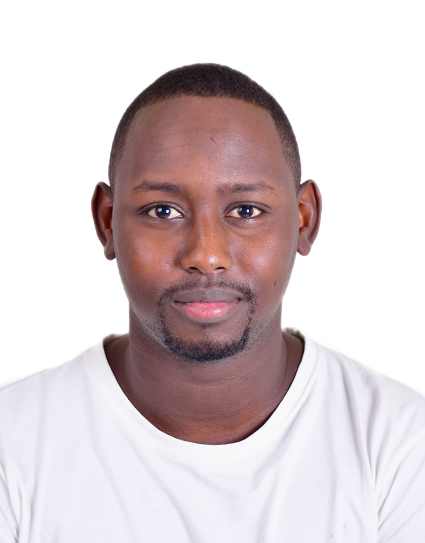
Muhire Josué
Muhire Josué
I am a backend developer, interested in writing about backend engineering, DevOps and tooling.