Spring Cloud Gateway: A Comprehensive Guide
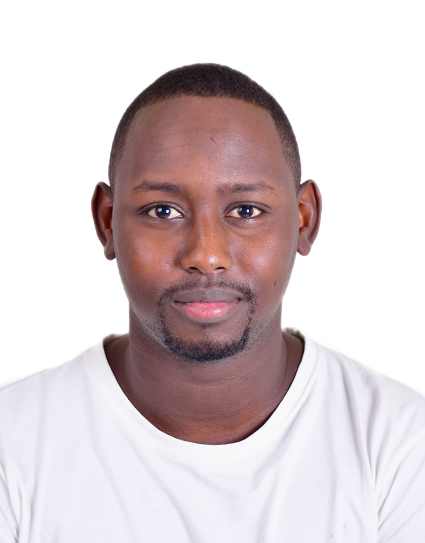
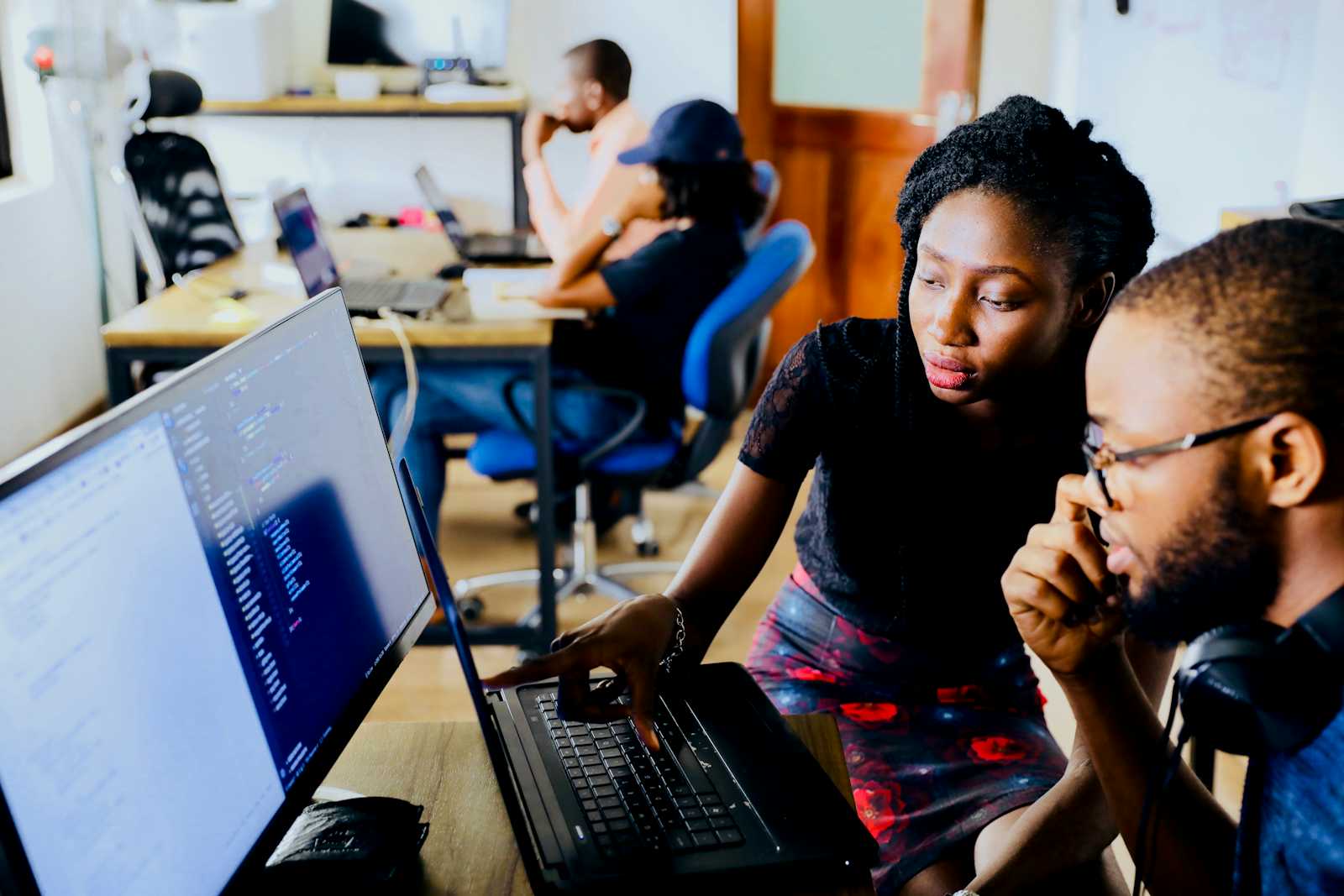
Introduction
In modern microservices architectures, managing traffic between services is a critical task. API Gateways act as intermediaries that handle requests, route them to appropriate services, apply security policies, and provide other cross-cutting concerns like logging and monitoring. Spring Cloud Gateway, part of the Spring Cloud ecosystem, is a powerful and customizable API Gateway built on top of the Spring Framework. It offers a flexible way to handle routing, filtering, and load balancing for microservice requests. In this article, we'll discuss the core features of Spring Cloud Gateway and how to set it up in a Spring Boot application.
Advantages of Spring Cloud Gateway
Dynamic Routing: Spring Cloud Gateway allows dynamic routing based on request parameters, headers, or path variables, directing the request to the appropriate microservice.
Built-in Filters: The Gateway provides pre-built filters for various purposes like authentication, logging, rate-limiting, and request transformation, allowing you to add security and monitoring layers with minimal code.
Load Balancing: Integrated with Spring Cloud LoadBalancer, Spring Cloud Gateway allows for easy load balancing across multiple service instances, improving performance and reliability.
Circuit Breaking: Spring Cloud Gateway can integrate with resilience tools like Resilience4J to provide circuit-breaking patterns, ensuring that failed services do not bring down the entire system.
Scalability: By acting as the central access point, Spring Cloud Gateway scales easily with the application, offering high availability and efficient routing for thousands of requests.
Code Example
Below is a simple example of how to configure Spring Cloud Gateway to route requests to multiple microservices.
pom.xml (dependencies)
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-gateway</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>Hoxton.SR12</version> <!-- Adjust version accordingly -->
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
Main Application Class
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class GatewayApplication {
public static void main(String[] args) {
SpringApplication.run(GatewayApplication.class, args);
}
}
application.yml
server:
port: 8080 # Gateway running on port 8080
spring:
application:
name: gateway-service
cloud:
gateway:
routes:
- id: my-service-route
uri: lb://my-service # Load balanced microservice URI via Eureka
predicates:
- Path=/service1/** # Routes requests to /service1/* to my-service
filters:
- StripPrefix=1 # Removes /service1 from the forwarded request
- id: another-service-route
uri: lb://another-service
predicates:
- Path=/service2/**
filters:
- StripPrefix=1
eureka:
client:
service-url:
defaultZone: http://localhost:8761/eureka/ # Eureka server URL
Explanation:
Dependencies:
spring-cloud-starter-gateway
: Includes all the necessary components for setting up the gateway.spring-cloud-starter-netflix-eureka-client
: Used to register the gateway with Eureka and perform service discovery for other services.
application.yml:
We define two routes:
/service1/**
maps tomy-service
, which is a service registered in Eureka./service2/**
maps toanother-service
.
StripPrefix=1
removes the prefix (/service1
or/service2
) before forwarding the request to the service.
Running the Gateway:
Ensure your Eureka server and other microservices (
my-service
,another-service
) are running.Start the Gateway by running the Spring Boot application.
Access the services through the gateway:
http://localhost:8080/service1/
...
will route tomy-service
.http://localhost:8080/service2/
...
will route toanother-service
.
Conclusion
Spring Cloud Gateway offers a highly configurable and efficient way to route and manage traffic in microservices architectures. By centralizing the routing logic and applying filters such as authentication and rate-limiting, it simplifies the architecture while enhancing security and scalability. Additionally, its seamless integration with Spring Cloud's service discovery (Eureka) and resilience tools make it a powerful solution for modern cloud-based applications.
Subscribe to my newsletter
Read articles from Muhire Josué directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
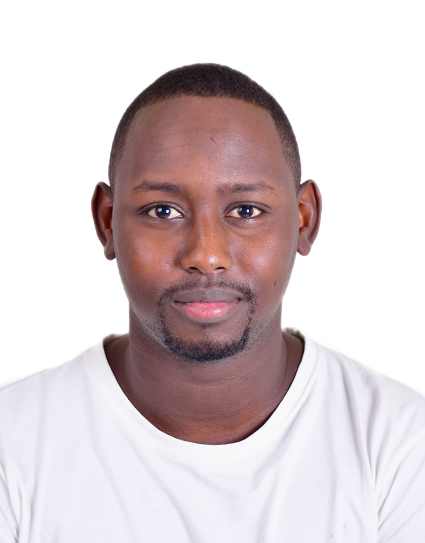
Muhire Josué
Muhire Josué
I am a backend developer, interested in writing about backend engineering, DevOps and tooling.