Understanding the Builder Design Pattern in Java
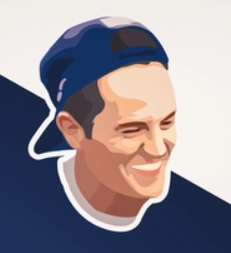

In application development, creating complex objects can become cumbersome, especially when they have multiple attributes or require specific configurations. The Builder Design Pattern is a solution that simplifies this process. In this post, we will explore why we need this pattern, what it is, and provide a detailed explanation of a Car
class implementation using the Builder Design Pattern in Java.
1. Why We Need the Builder Design Pattern
Creating objects with many parameters can lead to several issues:
Complex Constructors: When a class has many fields, the constructor can become lengthy and hard to read. This can lead to confusion and errors when instantiating the object.
Immutability: Often, you want the created objects to be immutable after construction. This means all fields should be set upon creation and not change thereafter.
Clarity and Maintainability: Using numerous parameters can make the code harder to maintain and understand, especially when many of them are optional.
Example: Consider a Car
class that requires attributes like carId
, carName
, brand
, model
, price
, and color
. A constructor that accepts all these parameters can be unwieldy and error-prone.
public class Car {
public Car(Integer carId, String carName, String brand, String model, Double price, String color) {
// Initialization
}
}
With this approach, it’s easy to mix up parameters, especially if they are of the same type (e.g., String
).
2. What is the Builder Design Pattern?
The Builder Design Pattern is a creational design pattern that allows for the step-by-step construction of complex objects. Instead of requiring all parameters to be provided at once, the builder provides methods to set individual parameters, returning the builder itself for method chaining. This approach enhances readability and flexibility.
Key Characteristics:
The builder class encapsulates the construction logic.
The main class (the product) has a private constructor, ensuring that objects can only be created through the builder.
This pattern is particularly useful for objects that require numerous parameters or have optional parameters.
3. Explanation with Code
Let’s implement the Car
class using the Builder Design Pattern to illustrate how it works.
public class Car {
private Integer carId;
private String carName;
private String brand;
private String model;
private Double price;
private String color;
// Private constructor to enforce the use of the builder
private Car(CarBuilder builder) {
this.carId = builder.carId;
this.carName = builder.carName;
this.brand = builder.brand;
this.model = builder.model;
this.price = builder.price;
this.color = builder.color;
}
// Getters
public Integer getCarId() {
return carId;
}
public String getCarName() {
return carName;
}
public String getBrand() {
return brand;
}
public String getModel() {
return model;
}
public Double getPrice() {
return price;
}
public String getColor() {
return color;
}
@Override
public String toString() {
return "Car{" +
"carId=" + carId +
", carName='" + carName + '\'' +
", brand='" + brand + '\'' +
", model='" + model + '\'' +
", price=" + price +
", color='" + color + '\'' +
'}';
}
// Inner Builder class
public static class CarBuilder {
private Integer carId;
private String carName;
private String brand;
private String model;
private Double price;
private String color;
public CarBuilder setCarId(Integer carId) {
this.carId = carId;
return this;
}
public CarBuilder setCarName(String carName) {
this.carName = carName;
return this;
}
public CarBuilder setBrand(String brand) {
this.brand = brand;
return this;
}
public CarBuilder setModel(String model) {
this.model = model;
return this;
}
public CarBuilder setPrice(Double price) {
this.price = price;
return this;
}
public CarBuilder setColor(String color) {
this.color = color;
return this;
}
public Car build() {
return new Car(this);
}
}
}
Explanation of the Code
Private Constructor: The
Car
class has a private constructor that accepts aCarBuilder
object. This ensures that cars can only be created through the builder.Static Inner Builder Class: The
CarBuilder
class contains methods for setting each attribute. Each setter method returns the builder instance (this
), allowing for method chaining.Build Method: The
build()
method creates a newCar
instance using the values set in the builder.
Create the Car object using CarBuilder Class
public class CarDriver {
public static void main(String[] args) {
Car car = new Car.CarBuilder()
.setCarId(1)
.setCarName("Model S")
.setBrand("Tesla")
.setModel("S")
.setPrice(79999.99)
.setColor("Red")
.build();
// Print car details
System.out.println(car);
}
}
Conclusion
The Builder Design Pattern is an excellent solution for constructing complex objects. It enhances code clarity and maintainability by allowing for step-by-step configuration of an object's properties. By implementing the Car
class with its CarBuilder
, we demonstrate how this pattern can streamline object creation in Java, making your code cleaner and less error-prone.
Subscribe to my newsletter
Read articles from Hemant Besra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
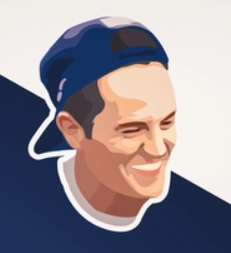
Hemant Besra
Hemant Besra
Experienced Full Stack Java developer. Have Strong Experience in JSP/Servlet, JSF, Jasper Report, Spring Framework, hibernate, Angular 5+, Microservices. Experienced in Front-end technologies such as HTML, CSS, JavaScript, angular 6+, AJAX, JSON, and XML. Strong Hands-on experience on working with Reactive Forms to build form-based application in Angular 6+.