Simplify Dynamic View Creation in Laravel 11 with Blade Templating
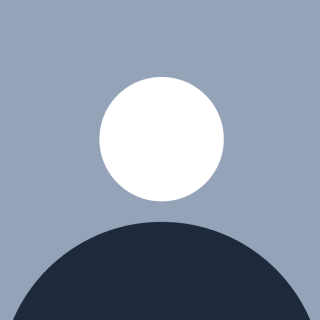
Table of contents
- Unveiling the Canvas: Mastering Laravel Views for Dynamic Interfaces.
- Harnessing Blade: Laravel's Powerful Templating Engine.
- Streamlined Syntax: Blade's Directives for Clean Code.
- Template Inheritance: Reuse and Extend with Blade.
- Reusable UI Elements: Blade Components and Slots Explained.
- Customize with Precision: Crafting Custom Blade Directives.

Picture this: You're developing a web application, and suddenly, you are drowning in a sea of PHP echo statements and HTML tags. Sound familiar? Dont Worry! Laravel's Views and Blade templating system are here to rescue you from this chaos and enhance your development experience" for simplicity.
Unveiling the Canvas: Mastering Laravel Views for Dynamic Interfaces.
Laravel Views are the foundation of your user interface. They're like the canvas on which we paint our web application's visual elements. But unlike a static painting, Laravel Views are dynamic and interactive.
Decoding Laravel Views: The Backbone of Your Web Interface.
At the heart of any Laravel application is the concept of MVC (Model-View-Controller) architecture, where views are responsible for presenting data to users. In Laravel, views are stored in the resources/views
directory and serve as the front-end layer that interacts with the data fetched from controllers.
To create a view, we use the .blade.php
extension, which signals to Laravel that Blade will be used. Each view in Laravel is simply an HTML file that can contain Blade directives to handle logic like loops, conditionals, and template inheritance.
Let's begin with a straightforward illustration:
<!-- resources/views/welcome.blade.php -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Welcome to My App</title>
</head>
<body>
<h1>Welcome, {{ $name }}!</h1>
<p>It's great to have you here.</p>
</body>
</html>
In this example, we've created a basic welcome page. The {{ $name }}
syntax is a Blade directive that we'll explore in more depth shortly.
Seamlessly Passing Data: Empower Your Views with Dynamic Content.
Data can easily be passed to views from controllers, enabling dynamic content generation. For instance:
public function showWelcome()
{
$name = 'John Doe';
return view('welcome', compact('name'));
}
In this example, the compact
function is used to pass the $name
variable to the view.
Harnessing Blade: Laravel's Powerful Templating Engine.
Blade is Laravel's templating engine, which adds a layer of functionality on top of plain PHP without sacrificing performance. Blade templates are compiled into raw PHP and cached for optimal performance.
One of the main advantages of Blade is its template inheritance, allowing us to define a master layout and extend it with child views. This ensures a DRY (Don’t Repeat Yourself) approach to creating view templates.
Mastering Blade Syntax: Clean, Efficient, and Intuitive.
Blade uses a clean, easy-to-read syntax, which includes variables, conditionals, and loops.
Effortless Data Display: Leveraging Blade's Variable Syntax.
In Blade, variables are displayed using double curly braces:
{{ $variable }}
Blade also provides a way to safely escape data:
{!! $variable !!}
Conditional Logic Made Easy: Blade's Intuitive Directives.
Blade’s conditional directives are intuitive. Here’s an example of an if
statement:
@if($user->isAdmin())
<p>Welcome, Admin!</p>
@else
<p>Welcome, User!</p>
@endif
Blade also supports elseif
and else
directives.
Iterate with Ease: Simplifying Loops in Blade.
Blade makes looping through data simple with directives like @foreach
, @for
, @while
, and @forelse
:
@foreach($users as $user)
<p>{{ $user->name }}</p>
@endforeach
This loop will iterate over a list of users and display their names.
Streamlined Syntax: Blade's Directives for Clean Code.
Blade syntax is clean, easy to read, and packed with functionality. Below are some common Blade directives:
@extends
: Used to extend a layout.@section
: Defines a section of content to be inserted into a layout.@yield
: Placeholder for content to be inserted from a section.@if
,@else
,@elseif
: Blade’s conditional logic.@foreach
,@for
: Loops for iterating over arrays or objects.
Template Inheritance: Reuse and Extend with Blade.
One of Blade’s most powerful features is template inheritance, allowing developers to define a base layout and extend it in child views. This promotes the reuse of common layouts, reducing the need for redundant code.
Crafting a Masterpiece: Building a Master Layout in Blade.
Let’s start by defining a master layout in layouts/app.blade.php
:
<!-- resources/views/layouts/app.blade.php -->
<!DOCTYPE html>
<html>
<head>
<title>App Name - @yield('title')</title>
</head>
<body>
<div class="container">
@yield('content')
</div>
<footer>
@include('partials.footer')
</footer>
</body>
</html>
Notice the @yield
and @include
directives? These are Blade’s way of saying, Put dynamic content here and Include this reusable chunk of code.
Extend and Conquer: Utilizing Blade's Layout Extensions.
Now, let’s create a child view that extends the layout:
@extends('layouts.app')
@section('title', 'Page Title')
@section('content')
<h1>Welcome to the Home Page</h1>
<p>This is a dynamically generated page using Blade templates.</p>
@endsection
The @extends
directive indicates that this view is using the app
layout, and the @section
directive allows us to inject content into the specified sections of the master layout.
Reusable UI Elements: Blade Components and Slots Explained.
In addition to template inheritance, Blade offers components and slots, which allow us to define reusable pieces of the UI. This is particularly useful for building dynamic interfaces like forms, buttons, and alerts.
Component Creation: Building Reusable UI with Blade.
Let’s create a simple Blade component for an alert:
<!-- resources/views/components/alert.blade.php -->
<div class="alert alert-{{ $type }}">
{{ $slot }}
</div>
Integrate with Ease: Implementing Blade Components in Views.
We can use this alert component in our views as follows:
<x-alert type="success">
This is a success message.
</x-alert>
The type
the attribute is passed to the component, and the content between the component tags is passed as a slot.
Dynamic Rendering: Unleashing Blade's Component Flexibility.
Blade also supports dynamic components, allowing us to render components dynamically at runtime. For example:
@php
$componentName = 'alert';
@endphp
<x-dynamic-component :component="$componentName" type="warning">
This is a dynamic warning message.
</x-dynamic-component>
Customize with Precision: Crafting Custom Blade Directives.
Blade comes with many built-in directives for handling tasks like loops, conditionals, and including other views. However, we can also define custom directives for more complex logic.
Tailor-Made Directives: How to Create Custom Blade Logic.
Here’s an example of how to create a custom Blade directive to format dates:
use Illuminate\Support\Facades\Blade;
Blade::directive('datetime', function ($expression) {
return "<?php echo ($expression)->format('m/d/Y H:i'); ?>";
});
Now, we can use this directive in our views:
@datetime($user->created_at)
Comprehensive Guide: Exploring Blade's Built-In Directives.
Here’s a comprehensive list of Blade's built-in functions that are covered in the blog, along with some additional ones commonly used in Laravel Blade templating:
@extends
Defines the base layout that a view will inherit from.@extends('layouts.master')
@section
Defines a section of content that can be yielded or overwritten in a parent layout.@section('content') <p>This is my content section.</p> @endsection
@yield
Used in layouts to render content from a section.@yield('content')
@include
Includes a sub-view or partial inside another Blade template.@include('partials.header')
@if
/@elseif
/@else
Handles conditional logic inside views.@if ($user->isAdmin()) <p>Welcome, Admin!</p> @elseif ($user->isModerator()) <p>Welcome, Moderator!</p> @else <p>Welcome, User!</p> @endif
@foreach
Iterates over a collection or array.@foreach ($users as $user) <p>{{ $user->name }}</p> @endforeach
@for
/@while
Loops for fixed or conditional iteration.@for ($i = 0; $i < 10; $i++) <p>Iteration: {{ $i }}</p> @endfor
@csrf
Inserts a CSRF (Cross-Site Request Forgery) token into forms for security.<form method="POST"> @csrf </form>
@push
/@stack
Allows adding content to specific sections (useful for scripts or meta tags).@push('scripts') <script src="/js/custom.js"></script> @endpush
@stack('scripts')
@verbatim
Disables Blade’s templating engine to allow raw output, useful when working with JavaScript frameworks.@verbatim <div id="app"> {{ message }} </div> @endverbatim
@unless
The inverse of@if
; only runs the block of code if the condition is false.@unless($user->isGuest()) <p>Welcome back, {{ $user->name }}!</p> @endunless
@isset
/@empty
Checks if a variable is set or empty, respectively.@isset($name) <p>The name is {{ $name }}</p> @endisset
@empty($name) <p>No name provided</p> @endempty
@auth
/@guest
Check if a user is authenticated or not.@auth <p>Welcome, authenticated user!</p> @endauth
@guest <p>Please log in.</p> @endguest
@php
Executes raw PHP code inside Blade templates.@php $name = 'John Doe'; @endphp <p>Hello, {{ $name }}!</p>
{!! !!}
Outputs raw, unescaped HTML. Use cautiously to avoid security risks.{!! $content !!}
{{ }}
Outputs escaped data, useful for preventing XSS attacks.<p>{{ $user->name }}</p>
@component
/@slot
Allows creating reusable components with slots for content.@component('components.alert', ['type' => 'danger']) @slot('title') Error @endslot Something went wrong! @endcomponent
@error
Checks for validation errors for a specific form field.@error('email') <p class="text-danger">{{ $message }}</p> @enderror
@can
/@cannot
Checks user permissions.@can('update', $post) <p>You can edit this post.</p> @endcan
@method
Used to spoof HTTP methods (like PUT or DELETE) in forms.<form method="POST"> @method('PUT') @csrf </form>
These functions make Blade an efficient, clean, and powerful templating engine that allows developers to build dynamic, maintainable interfaces easily.
Conclusion: Elevate Your Development with Blade's Power
Blade is not just a templating engine—it’s a tool that simplifies the process of creating dynamic, modular, and reusable interfaces in Laravel. With Blade’s components, layouts, and directives, you can build complex interfaces while keeping your code maintainable and elegant.
Next time you are thinking about building dynamic views, remember: Blade is your best friend. It will save you time, reduce code repetition, and make your front-end development smoother than ever. Happy coding!
Subscribe to my newsletter
Read articles from Aman jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Aman jain
Aman jain
I'm a developer who shares advanced insights and expertise through technology-related articles. Passionate about creating innovative solutions and staying up-to-date with the latest tech trends. Let's connect and explore the world of technology together!