Day 5: Conditionals – JS Drama 🥷
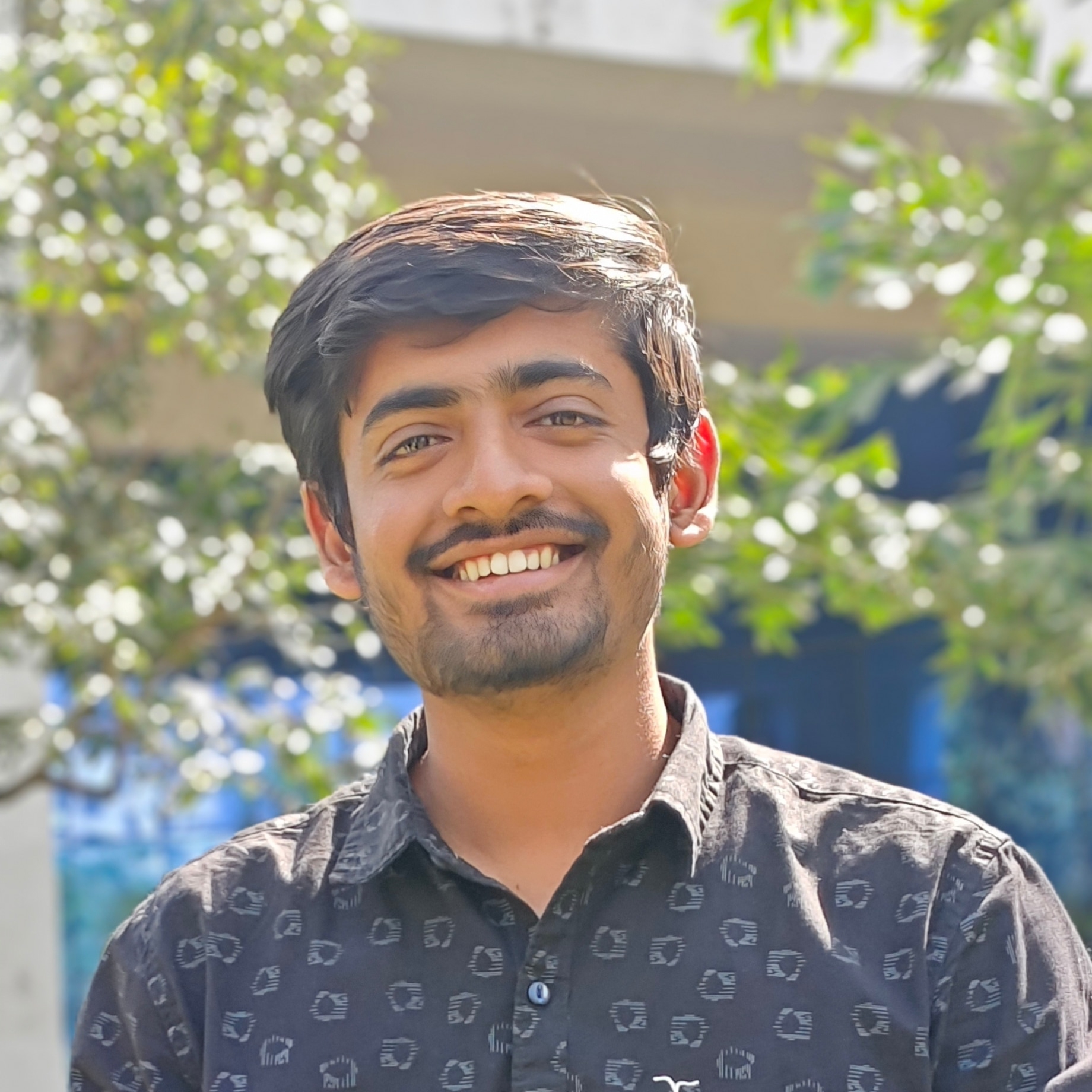
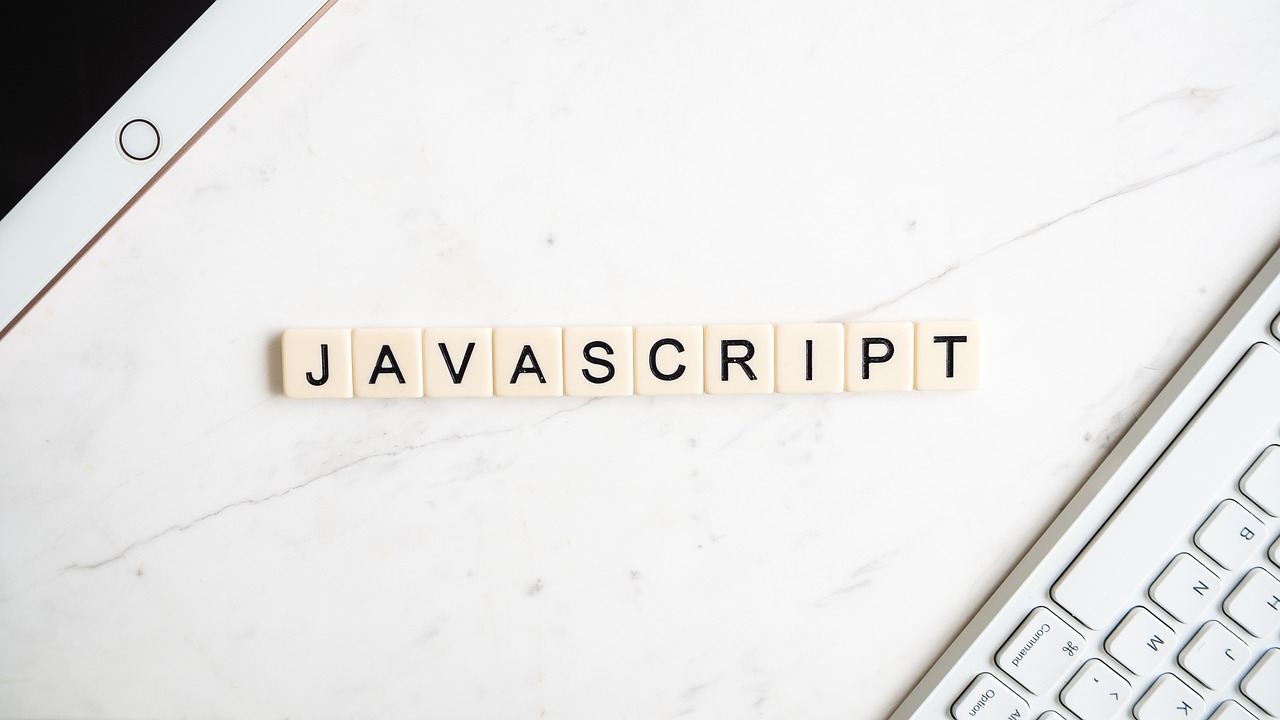
Theme: 🎭 Welcome to Code Theater! In today’s act, JavaScript will become the director of your life. Using if-else and switch, you’ll make dramatic decisions, just like choosing whether to binge Netflix or finally start working on that project (we all know what wins 🫣). After today, you’ll know how to make decisions in your code – the fun way!
1. The Art of Conditionals 🎬
Conditionals allow your code to make decisions based on certain conditions. Think of them as "Choose Your Own Adventure" paths—JavaScript checks conditions and decides what to do next.
Types of Conditionals:
if-else: Basic "If this, do that" logic.
else-if: Adds more specific conditions to the flow.
switch: When there are many options—like picking a movie genre.
2. if-else: The Code Soap Opera 🎭
Here’s how it works:
If a condition is true, one block of code runs.
Else another block will run if the condition is false.
Example: Should I Binge Netflix Today?
javascriptCopy codelet mood = "lazy";
if (mood === "lazy") {
console.log("Binge Netflix all day! 🍿");
} else {
console.log("Time to work on that project! 💼");
}
💡 Explanation:
If your mood is "lazy", Netflix wins.
If not, it’s time to be productive (or pretend to be).
Adding Spice with else-if 🌶️
What if there are more moods involved? Let’s extend the logic.
javascriptCopy codelet mood = "bored";
if (mood === "lazy") {
console.log("Binge Netflix all day! 🍿");
} else if (mood === "bored") {
console.log("Go for a walk or learn something new! 🚶♂️");
} else {
console.log("Focus on work! You got this 💪.");
}
3. switch: The Genre Picker 🍿
When you have multiple conditions to check, switch
works better. Think of it like a Netflix genre selector!
Example: What Should I Watch?
javascriptCopy codelet genre = "comedy";
switch (genre) {
case "comedy":
console.log("Watch Brooklyn Nine-Nine! 😂");
break;
case "horror":
console.log("Watch The Haunting of Hill House! 👻");
break;
case "action":
console.log("Watch John Wick! 💥");
break;
case "sci-fi":
console.log("Watch The Expanse! 🚀");
break;
default:
console.log("Just watch The Office. It’s always a good choice! 🏢");
}
💡 Explanation:
The
switch
statement checks the value of thegenre
variable.Each case represents a different genre, with a specific movie suggestion.
The default case runs if no matching genre is found.
4. Fun Task: Make a Mini Decision-Maker 🗳️
Let’s create a decision-maker tool to help you decide whether to binge Netflix today or do something productive.
javascriptCopy codefunction shouldIBingeNetflix(mood) {
if (mood === "lazy" || mood === "tired") {
return "Binge Netflix today! 🍿";
} else if (mood === "bored") {
return "Go for a walk or call a friend! 📞";
} else if (mood === "motivated") {
return "Focus on your goals! 🚀";
} else {
return "Follow your heart... and maybe open Netflix just a little? 😁";
}
}
// Test it out!
let todayMood = "tired";
console.log(shouldIBingeNetflix(todayMood));
5. Quick Quiz: Guess the Output! 🧐
- Question 1:
javascriptCopy codelet weather = "rainy";
if (weather === "sunny") {
console.log("Go to the beach! 🏖️");
} else {
console.log("Stay home and chill. 🌧️");
}
Answer:Stay home and chill. 🌧️
- Question 2:
javascriptCopy codelet genre = "romance";
switch (genre) {
case "action":
console.log("Fast & Furious time! 🚗");
break;
case "romance":
console.log("Watch Pride and Prejudice. ❤️");
break;
default:
console.log("Pick something fun!");
}
Answer:Watch Pride and Prejudice. ❤️
6. Summary: What Did We Learn Today?
if-else helps you make decisions based on conditions.
else-if adds more options to your logic flow.
switch is great for multiple conditions (like choosing a movie genre).
7. Extra Challenge: Mood-Based Day Planner 🗓️
Use if-else or switch to create a day planner. Based on your mood, decide what activity you should do next!
javascriptCopy codefunction dayPlanner(mood) {
switch (mood) {
case "lazy":
return "Relax and watch some Netflix! 🍿";
case "bored":
return "Try a new hobby or read a book. 📚";
case "motivated":
return "Tackle that project you’ve been putting off! 💼";
default:
return "Just go with the flow and enjoy your day! 🌊";
}
}
// Test it
console.log(dayPlanner("motivated"));
8. What's Next?
Tomorrow, we’ll dive into loops and explore how to repeat actions without writing the same code over and over again. Think of it as "Time Travel in JavaScript"! 🔄 Get ready for some awesome loop magic!
🎉 You’re now officially the Master of Conditionals! Go flex your newfound knowledge in your projects, and remember: When in doubt... always binge Netflix! 😉
Subscribe to my newsletter
Read articles from Risharth pardeshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
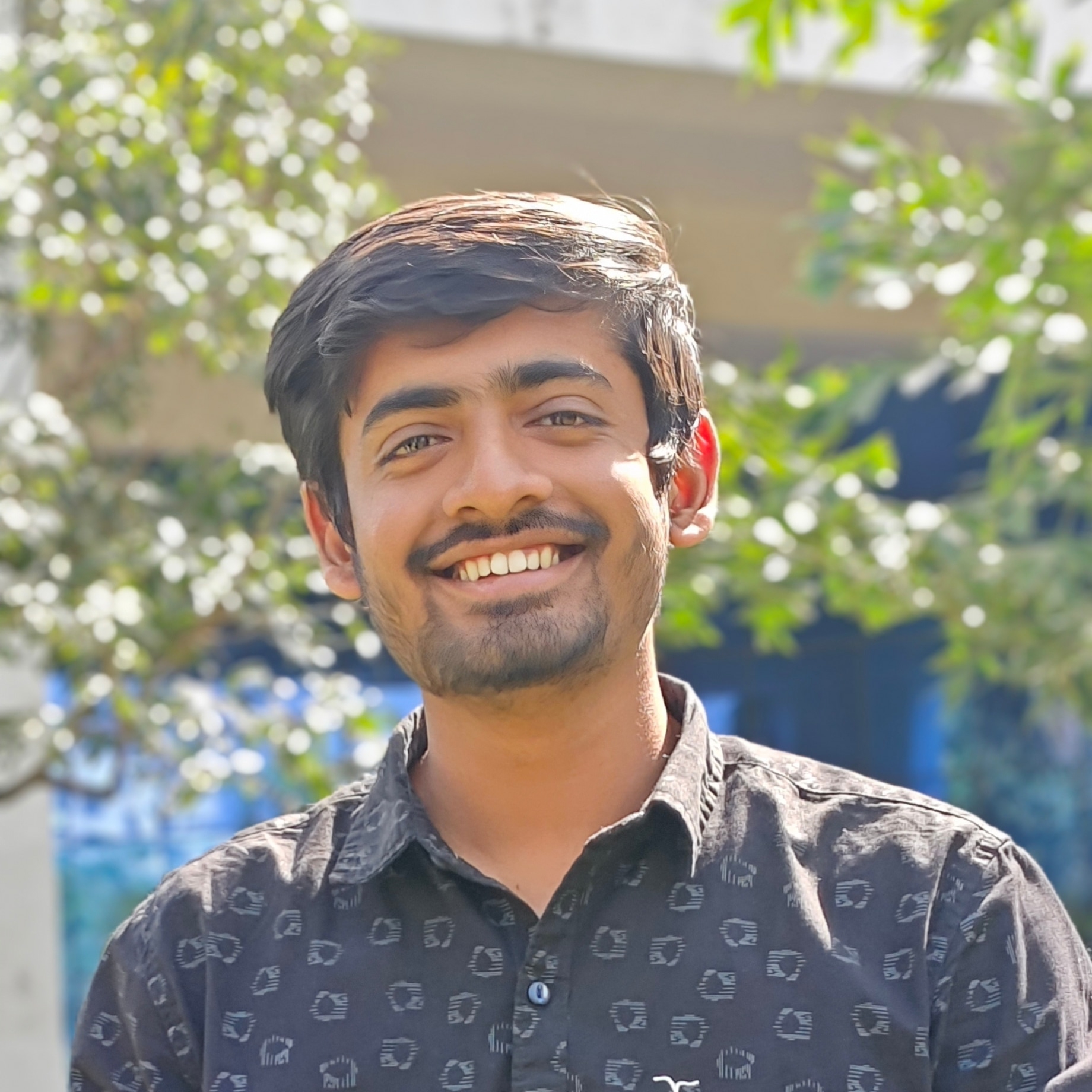
Risharth pardeshi
Risharth pardeshi
I hope this message finds you well. I am Risharth Pardeshi I am passionate about Computer Science and Engineering, and I have a strong foundation in this field. I graduated with a Bachelor of Engineering from the Laxmi Narayan College of Technology in Indore, India, in July 2024, with a CGPA of 7.98. Here is a brief overview of my technical strengths: Languages: C++, HTML, CSS, JavaScript, SQL Frameworks: React.js, Next.js, Tailwind CSS, Node.js Databases/OS: MySQL, MongoDB, Firebase, Linux Technical Skills: Data Structures and Algorithms, Git, DBMS, OS, Object-Oriented Programming, Computer Networking, Vercel, Postman