🌟 Understanding the Spread Operator and Rest Operator in JavaScript

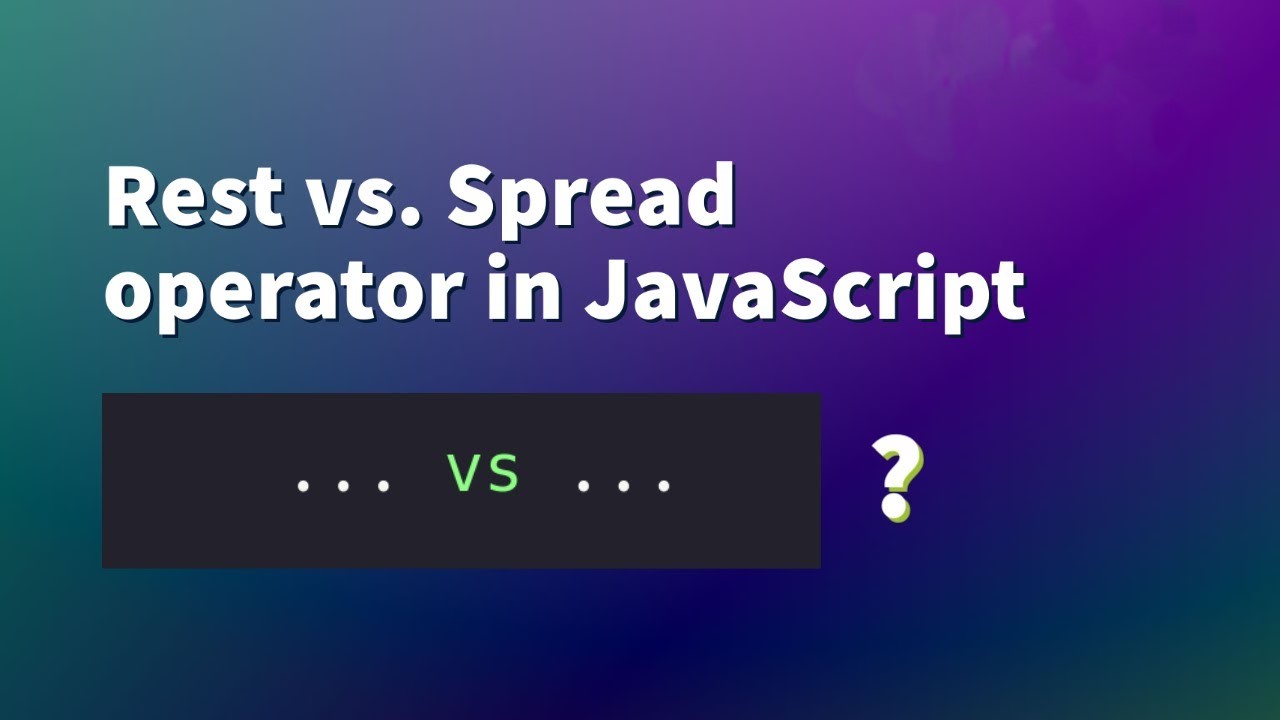
JavaScript has two versatile features, the Spread Operator and the Rest Operator, which are both represented by three dots (...
). While they look the same, they have different roles based on the context in which they're used. Let’s break down each of them and explore their unique purposes before diving into their differences.
🚀 What is the Spread Operator?
The Spread Operator (...
) allows an iterable (such as an array, string, or object) to be expanded into individual elements. It’s particularly useful for creating copies, merging arrays or objects, and passing array elements as function arguments.
1️⃣ Spread with Arrays
The spread operator can copy or merge arrays effortlessly:
// Copying an array
const arr1 = [1, 2, 3];
const copy = [...arr1];
console.log(copy); // [1, 2, 3]
// Merging arrays
const arr2 = [4, 5, 6];
const merged = [...arr1, ...arr2];
console.log(merged); // [1, 2, 3, 4, 5, 6]
In this example, copy
is a new array with the same elements as arr1
, while merged
combines elements from arr1
and arr2
.
2️⃣ Spread with Objects
With ES2018, the spread operator became available for objects, making it a powerful way to clone or merge objects:
const user = { name: "Yasin", age: 23 };
const updatedUser = { ...user, city: "Dhaka" };
console.log(updatedUser);
// { name: "Yasin", age: 23, city: "Dhaka" }
Here, updatedUser
is a new object containing the properties of user
along with a new property city
.
3️⃣ Spread in Function Calls
It can be used to expand an array into individual arguments for a function:
function sum(a, b, c) {
return a + b + c;
}
const numbers = [1, 2, 3];
console.log(sum(...numbers)); // 6
Instead of passing numbers
as an array, the spread operator breaks it into individual values for the function.
🌿 What is the Rest Operator?
The Rest Operator (...
) is used to collect multiple elements into a single array or object. It is often used in function parameters to handle an indefinite number of arguments or during destructuring to gather remaining properties or array elements.
1️⃣ Rest with Function Parameters
The rest operator allows a function to accept any number of arguments, gathering them into an array:
function sum(...args) {
return args.reduce((acc, curr) => acc + curr, 0);
}
console.log(sum(1, 2, 3, 4, 5)); // 15
Here, ...args
gathers all arguments into a single array called args
, which is then processed using reduce()
.
2️⃣ Rest in Array Destructuring
Rest can also be used when destructuring arrays, to gather remaining elements:
const numbers = [1, 2, 3, 4, 5];
const [first, second, ...rest] = numbers;
console.log(first); // 1
console.log(second); // 2
console.log(rest); // [3, 4, 5]
In this example, first
gets the value 1
, second
gets 2
, and rest
captures [3, 4, 5]
.
3️⃣ Rest in Object Destructuring
Similarly, it can be used to collect remaining properties when destructuring objects:
const user = { name: "Yasin", age: 23, city: "Dhaka", country: "Bangladesh" };
const { name, ...details } = user;
console.log(name); // "Yasin"
console.log(details); // { age: 23, city: "Dhaka", country: "Bangladesh" }
In this example, name
is extracted, while details
gathers the remaining properties of user
.
🔍 Spread vs. Rest: Understanding the Differences
Although both operators use the same syntax (...
), their functions are distinct based on context. Here’s a comparison to make it clearer:
Feature | Spread Operator (...) | Rest Operator (…) |
Purpose | Expands iterable elements into individual items | Collects multiple elements into a single array or object |
Use Case | Copying or merging arrays/objects, passing elements as function arguments | Function parameters to accept varying numbers of arguments, array/object destructuring |
Position | Used in function calls, arrays, and objects | Used in function definitions and during destructuring |
Example | [...arr] expands an array into elements | (...args) gathers function arguments into an array |
📌 Key Takeaways
Spread Operator is like unpacking the elements of an array or object into individual components. It’s helpful when you need to pass data around as separate pieces.
Rest Operator is like packing multiple elements into a single collection. It’s useful when you need to gather multiple values into one entity for further processing.
🎯 Conclusion
Understanding the difference between the Spread Operator and the Rest Operator is crucial for handling JavaScript data structures and function arguments effectively. Whether you are expanding elements with the spread operator or gathering them with the rest operator, knowing when to use each one will help you write more readable and efficient code. 💡
Happy coding! 🚀😊
📝 Feel free to comment if I make any mistakes! 😊
💬 Your feedback helps me improve, and I truly appreciate it! 👍 Whether it's a typo, a confusing explanation, or something that could be made clearer—let me know!
📚 I'm always eager to learn and grow, so don’t hesitate to share your thoughts! Let's make this a space where we all improve together. 🌱
Subscribe to my newsletter
Read articles from Yasin Sarkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Yasin Sarkar
Yasin Sarkar
Front-End Developer. I create dynamic web applications using HTML, Tailwind CSS, JavaScript, React, and Next.js. I share my knowledge on social media to help others enhance their tech skills. An Open Source Enthusiast and Writer.