TypeScript + React Redux: Pro Tips for Type-Safe and Scalable Apps
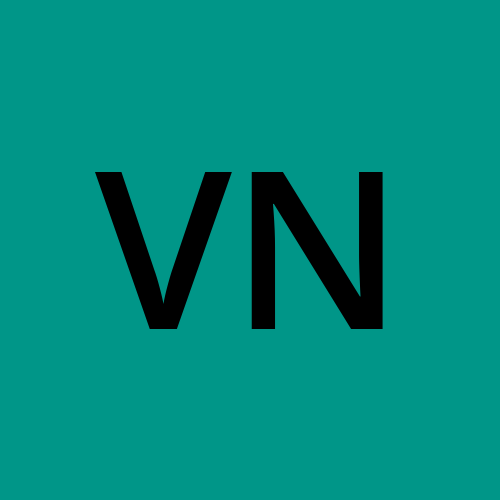
Table of contents
- 1. Enable Strict Mode in TypeScript
- 2. Define Typed Hooks
- 3. Use Type Annotations for Props and State
- 4. Define Slice State and Action Types
- 5. Avoid Using any Type
- 6. Implement Error Handling with Custom Types
- 7. Optimize Performance with Memoization
- 8. Keep UI State Separate from Network State
- 9. Use Third-Party Libraries with TypeScript Support
- 10. Leverage ESLint for Consistency
- Conclusion
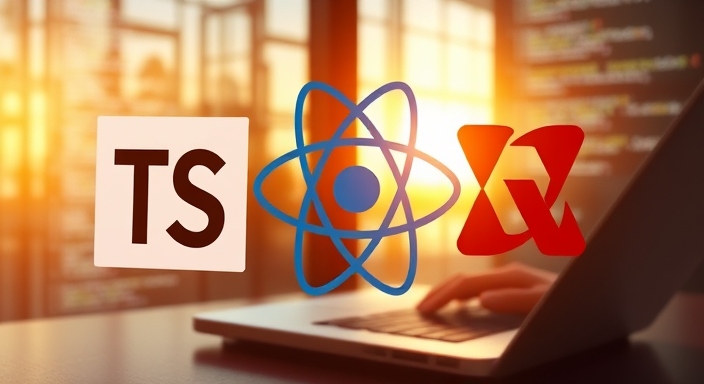
In the rapidly evolving landscape of web development, combining TypeScript with React and Redux has become a popular choice among developers. This powerful trio enhances code quality, maintainability, and developer experience. In this blog post, we’ll explore best practices for effectively using TypeScript with React Redux to build robust applications.
1. Enable Strict Mode in TypeScript
The first step towards a type-safe application is to enable strict mode in your TypeScript configuration. By setting "strict": true
in your tsconfig.json
, you enforce strict type checking, which helps catch potential errors at compile-time. This practice significantly reduces runtime errors and improves code reliability.
json{
"compilerOptions": {
"strict": true
}
}
2. Define Typed Hooks
One of the most effective ways to enhance type safety is by creating typed versions of the useDispatch
and useSelector
hooks. This allows you to avoid repetitive type definitions throughout your application.
typescriptimport { TypedUseSelectorHook, useDispatch, useSelector } from 'react-redux';
import type { RootState, AppDispatch } from './store';
export const useAppDispatch = () => useDispatch<AppDispatch>();
export const useAppSelector: TypedUseSelectorHook<RootState> = useSelector;
3. Use Type Annotations for Props and State
Always provide explicit type annotations for component props and state. This practice not only ensures type safety but also improves code readability and maintainability.
typescriptinterface MyComponentProps {
name: string;
age: number;
}
const MyComponent: React.FC<MyComponentProps> = ({ name, age }) => {
// Component implementation
};
4. Define Slice State and Action Types
When using Redux Toolkit, define types for your slice state and actions explicitly. This approach allows TypeScript to infer types correctly in reducers and action creators.
typescriptimport { createSlice, PayloadAction } from '@reduxjs/toolkit';
interface CounterState {
value: number;
}
const initialState: CounterState = { value: 0 };
const counterSlice = createSlice({
name: 'counter',
initialState,
reducers: {
increment: (state) => { state.value += 1; },
decrement: (state) => { state.value -= 1; },
},
});
export const { increment, decrement } = counterSlice.actions;
5. Avoid Using any
Type
Refrain from using the any
type as much as possible. Instead, leverage TypeScript’s type inference capabilities to maintain type safety throughout your application. This practice ensures that your code remains predictable and easier to debug.
6. Implement Error Handling with Custom Types
Use custom types for error handling to ensure that error states are well-defined and easy to manage. This approach enhances clarity in your codebase when dealing with different error scenarios.
7. Optimize Performance with Memoization
Utilize React.memo
, useCallback
, and useMemo
to prevent unnecessary re-renders and optimize performance in functional components. These techniques help maintain efficient rendering cycles in your application.
typescriptconst MyComponent: React.FC<Props> = React.memo(({ propA, propB }) => {
// Component implementation
});
8. Keep UI State Separate from Network State
Maintain a clear separation between UI state (local component state) and network state (global application state managed by Redux). This separation simplifies component management and testing.
9. Use Third-Party Libraries with TypeScript Support
When integrating third-party libraries, prefer those that provide TypeScript support and type definitions. This ensures better integration with your codebase and helps catch potential issues early on.
10. Leverage ESLint for Consistency
Configure ESLint with TypeScript rules to enforce coding standards across your project. This setup helps maintain a consistent code style and catches potential errors early in the development process.
Conclusion
By following these best practices for using TypeScript with React Redux, you can create applications that are not only robust but also maintainable and scalable. The combination of static typing with powerful state management tools like Redux leads to improved developer experience and fewer runtime errors.
Embrace these practices to elevate your development workflow and build high-quality applications that stand the test of time!
Happy Coding!! 🚀🌟
Subscribe to my newsletter
Read articles from Vishal Nayak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
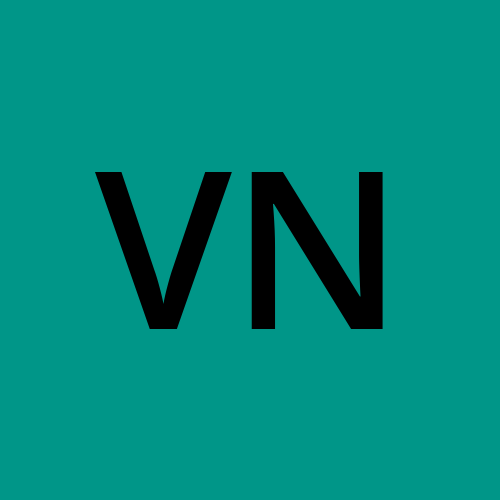