Understanding JavaScript Functions
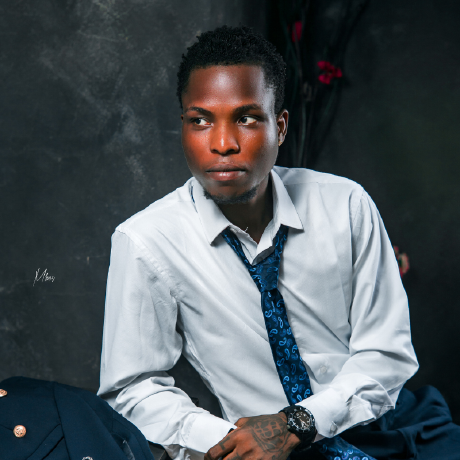
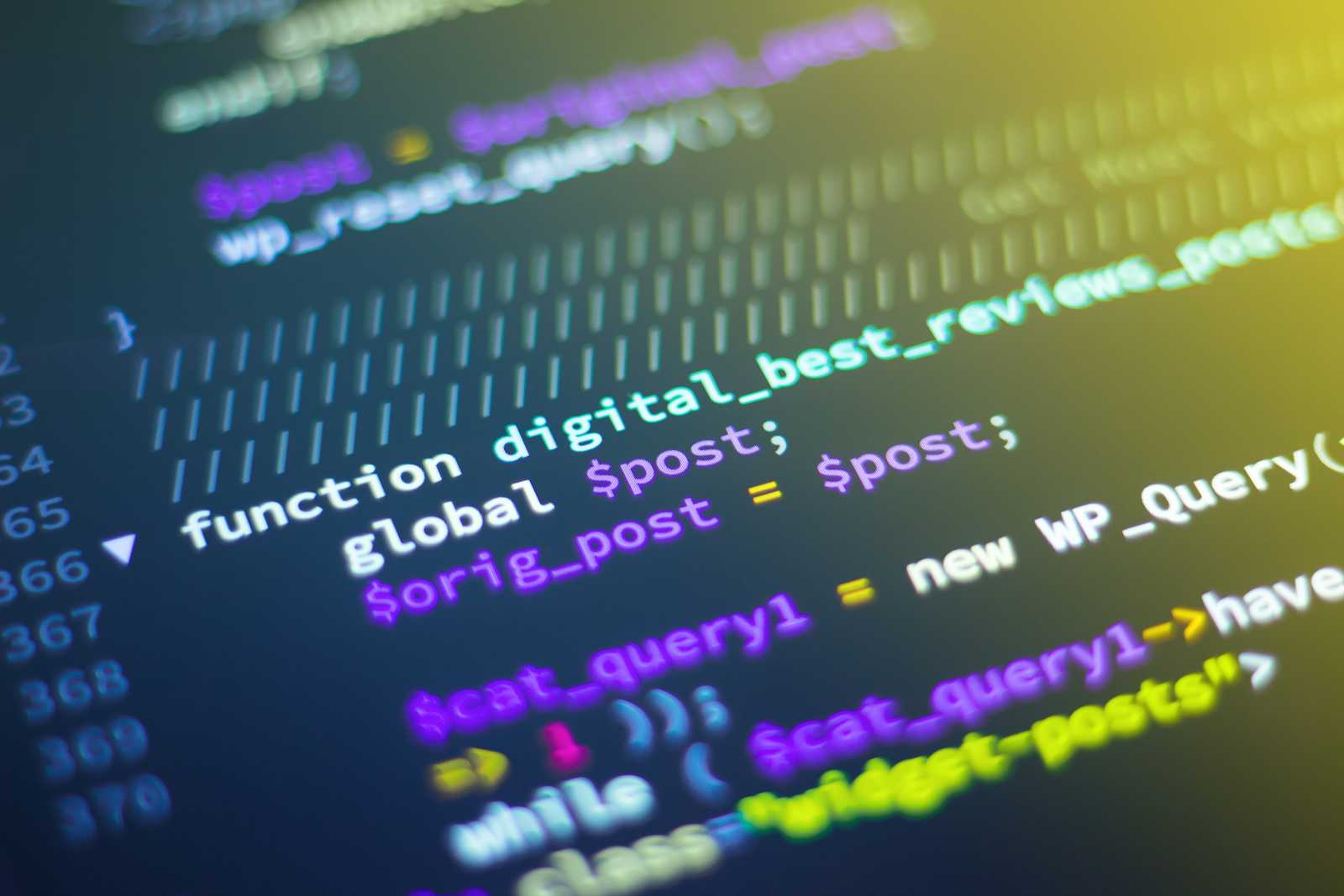
I’ve been learning JavaScript on my own—just me, my computer, and my little room with a bed, plastic chair, and desk. I open my computer every day, excited to dive into JavaScript using Google Chrome, my favorite browser.
My workspace inside my bedroom
I've been learning from amazing free resources like freeCodeCamp, YouTube Channels, Codecademy, and developer blogs. I’m here to share what I've learned about JavaScript Functions.
Understanding JavaScript Functions
JavaScript functions are essential building blocks that allow us to write reusable code. Think of them as mini-programs designed to perform specific tasks. Mastering functions can save you time by turning repeated code into callable functions that you can use anytime.
What is a Function?
At its core, a function is a set of instructions that you can execute whenever needed. Functions take inputs (parameters) and can return outputs, making them highly versatile. Here’s a simple example:
function add(a, b) {
return a + b;
}
In this case, the function
add
takes two parameters (a and b) and returns their sum. You can calladd(2, 3)
anywhere in your code to get the result—no need to rewrite the logic.
Types of Functions
JavaScript supports multiple function types, each with its advantages:
Function Declarations: Defined with the
function
keyword and can be called before they’re defined due to hoisting.function greet() { console.log("Hello, World!"); }
Function Expressions: Functions that can be anonymous must be defined before they’re called.
const greet = function() { console.log("Hello, World!"); };
Arrow Functions: Introduced in ES6, these offer a more concise syntax.
const greet = () => { console.log("Hello, World!"); };
Parameters and Return Values
Functions can accept parameters—placeholders for the values passed when the function is called. They can also return values, which makes functions extremely useful for calculations and transformations.
Example of a function calculating the area of a rectangle:
function calculateArea(length, width) {
return length * width;
}
You can now call calculateArea(5, 10) without rewriting the formula.
Scope and Closures
Understanding scope is vital in JavaScript. Variables inside a function are not accessible outside of it, which keeps things organized. Additionally, closures allow functions to retain access to variables even when executed outside their original scope. Here’s an example:
function makeCounter() {
let count = 0;
return function() {
count++;
return count;
};
}
const counter = makeCounter();
console.log(counter()); // 1
console.log(counter()); // 2
In this case, the count variable is kept alive within the closure, even after the makeCounter function has finished executing.
Best Practices for Writing Functions
Here are a few tips to make your functions clean and efficient:
Keep Functions Small: Each function should perform one task.
Use Descriptive Names: Make function names clear and informative.
Avoid Side Effects: Functions should not modify external variables if possible.
Document Your Functions: Leave helpful comments for future you (or others).
Test Regularly: Always test functions to ensure they work as expected.
Conclusion
Mastering JavaScript functions is about more than just getting the code to work—it's about creating code that's clean, understandable, and maintainable.
Whether you're a beginner or an experienced developer, learning to work with functions will significantly enhance the quality of your projects.
Thanks for reading!
What has been your biggest challenge while learning JavaScript? Let's discuss this in the comments below. 🚀
𝗖𝗼𝗻𝗻𝗲𝗰𝘁 𝘄𝗶𝘁𝗵 𝗠𝗲:
LinkedIn: https://buff.ly/48aBDaE
Twitter (X): https://buff.ly/4dVL3Ia
Instagram: https://buff.ly/48jTJak
GitHub: https://buff.ly/4dQJjQs
Subscribe to my newsletter
Read articles from Stanley Owarieta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
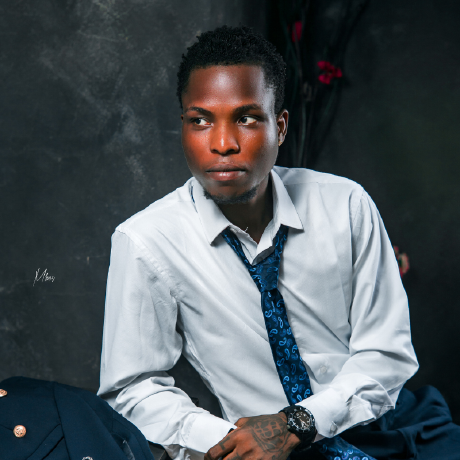
Stanley Owarieta
Stanley Owarieta
I help tech businesses and startups create engaging, SEO-driven content that attracts readers and converts them into customers. From in-depth blog posts to product reviews, I ensure every content is well-researched, easy to understand, and impactful. As a programming learner with HTML, CSS, and JavaScript knowledge, I bring a unique technical perspective to my writing. If you're looking for content that ranks and resonates, let's connect! 📩 Open to collaborations! Message me or email me at freelance@stanleyowarieta.com