Exploring Software Design Principles
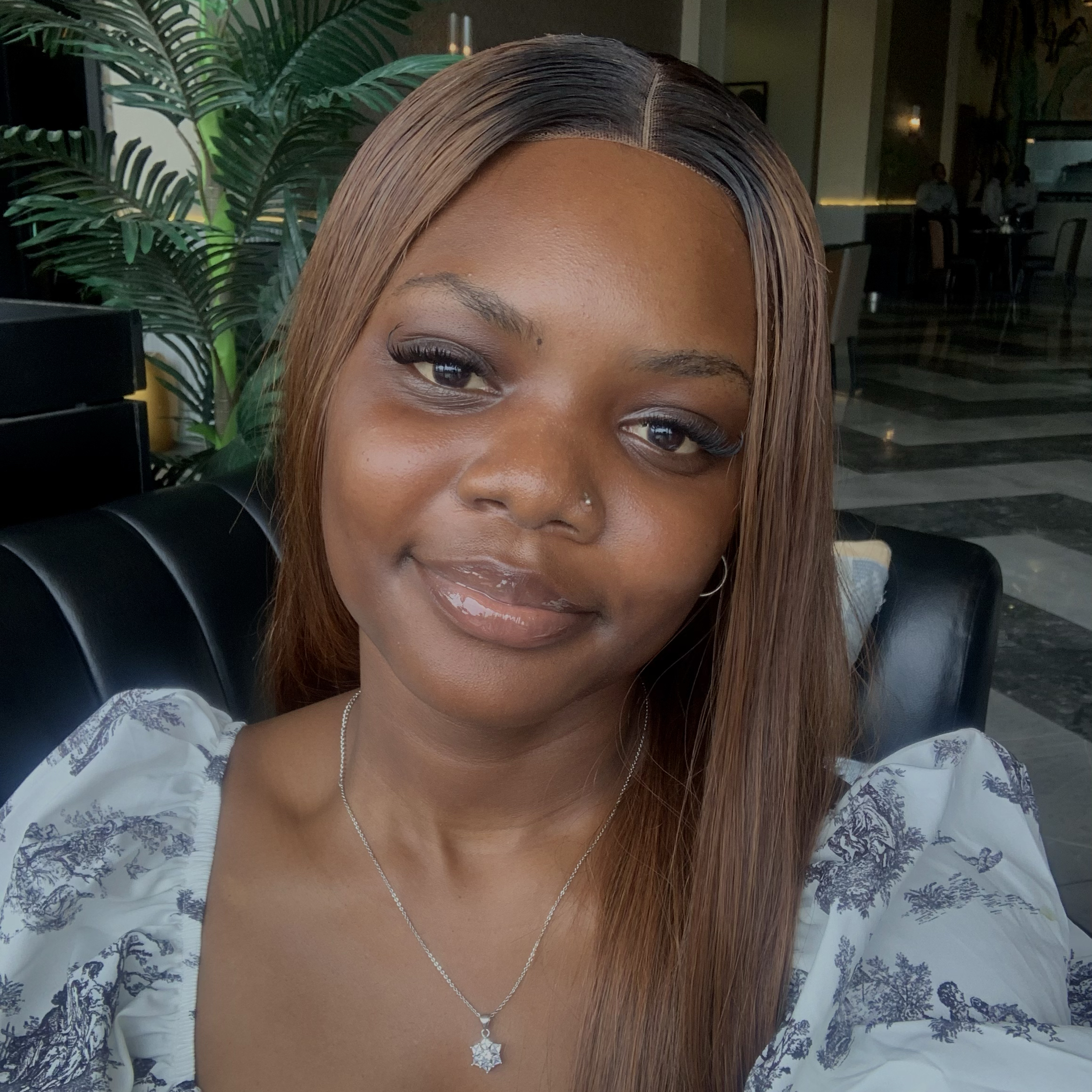
Table of contents
- What are Software Design Principles?
- Major Software Design Principles
- 1. SOLID Principles
- 2. Don’t Repeat Yourself (DRY)
- 3. Keep It Simple, Stupid (KISS)
- 4. You Aren’t Gonna Need It (YAGNI)
- 5. Separation of Concerns (SoC)
- 6. Modularity
- 7. Cohesion
- 8. Coupling
- 9. Encapsulation
- 10. Law of Demeter (LoD)
- 11. Principle of Least Privilege
- 12. Least Astonishment
- 13. Fail Fast
- 14. Design For Testing
- 15. Favor Immutability
- 16. Favor composition over inheritance
- 17. Don’t modify global state
- 18. Use Meaningful Names
- 19. Refactor Often
- 20. Document As you Code
- 21. Version Control
- 22. Automate repetitive tasks
- 23. Code to an Interface, Not an Implementation
- 24. Minimize Side Effects
- 25. Avoid Long Parameter Lists
- Conclusion
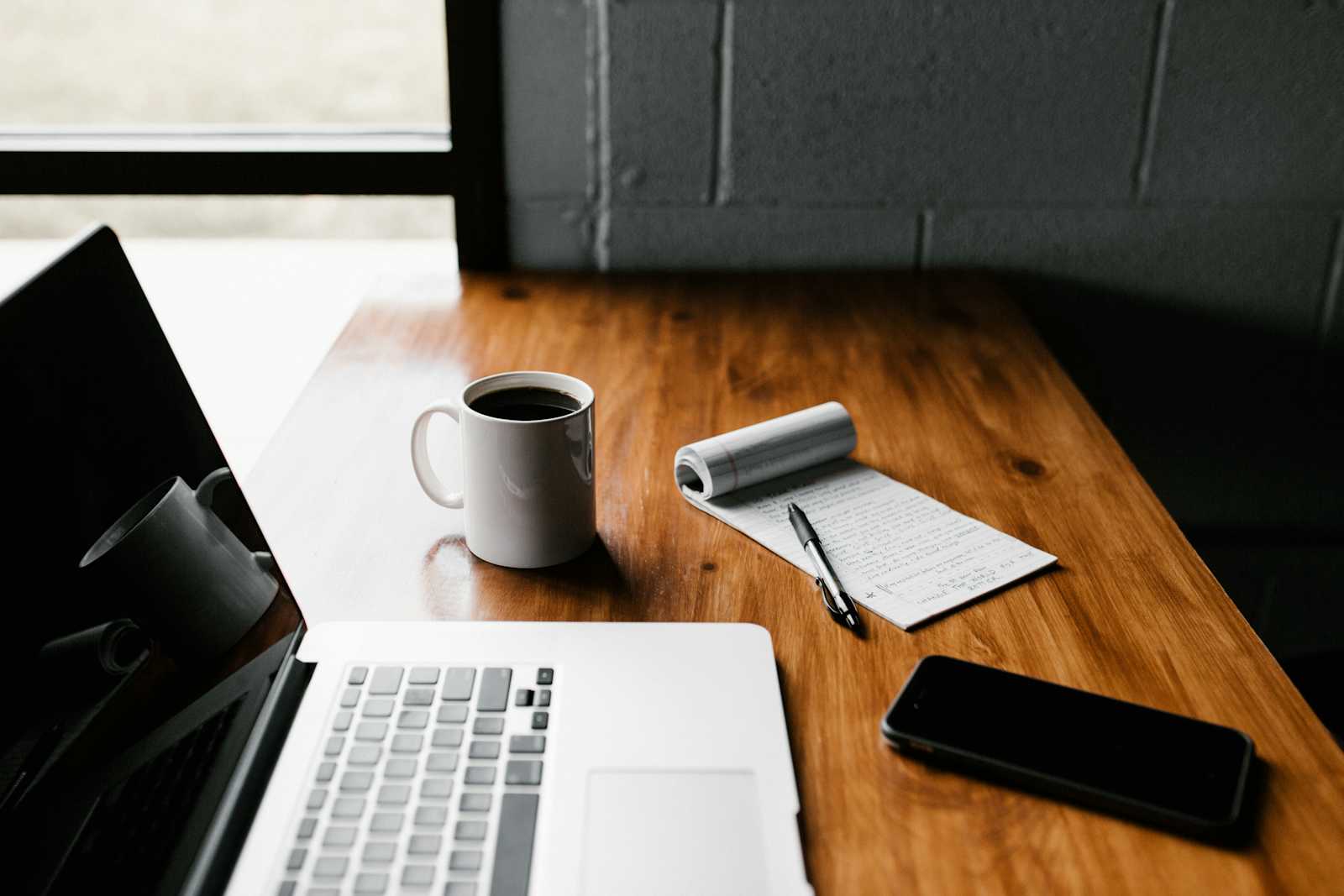
Developers often encounter a lot of bugs whenever trying to scale an application and add new features. This is why software design principles are important, because these principles guide programmers in building sustainable and maintainable software applications.
What are Software Design Principles?
Software design principles are guidelines that developers must follow to build efficient, maintainable and scalable applications. The particular aim of these principles is to ensure that applications are capable of evolving over time.
As software complexity increased over time, developers and computer scientists began establishing design principles to ensure maintainability, adaptability and scalability of software applications.
Major Software Design Principles
1. SOLID Principles
These are the five foundational principles aimed at improving object-oriented design:
- Single Responsibility Principle (SRP)
This principle states that a function should have only one responsibility. The aim of this is to reduce complexity and increase maintainability.
- Open Closed Principle (OCP)
This principle states that software entities like classes, functions, modules should be opened for extension but closed for modification. This prevents modifying existing code when adding new functionality. Instead, the behavior of a class is extended through inheritance or composition, ensuring that existing code remains unchanged.
- Liskov Substitution Principle (LSP)
This principle ensures that derived classes (subclasses) enhance or extend the functionality of base classes (superclasses) without changing the expected behavior, promoting flexibility in code reuse.
- Interface Segregation Principle (ISP)
This principle states that clients should not be forced to depend on interfaces they do not use. A class should implement only the methods it needs, which promotes clean code and avoids unnecessary dependencies.
- Dependency Inversion Principle (DIP)
This principle states that high-level modules should not depend on low-level modules; both should depend on abstractions (interfaces). Also, abstractions should not depend on details; details should depend on abstractions.
2. Don’t Repeat Yourself (DRY)
Avoid code duplication by abstracting code functionality. It reduces redundancy and improves maintainability.
3. Keep It Simple, Stupid (KISS)
Strive for simplicity in your code. Simple solutions are easier to maintain and less error-prone.
4. You Aren’t Gonna Need It (YAGNI)
Only build necessary functionality at the moment. Avoid over-engineering and anticipating requirements that might never arise
5. Separation of Concerns (SoC)
Modularize your program by dividing it into distinct sections, with each section addressing different parts of your code
6. Modularity
Break a system into smaller modules, with each module responsible for a specific piece of functionality.
7. Cohesion
Cohesion refers to how closely related the responsibilities of a module or class are. High cohesion within a class means that all the functionalities are closely related, which makes it more understandable and maintainable.
8. Coupling
Aim for low coupling, where classes or modules are minimally dependent on each other. This increases the flexibility of the system and makes changes easier.
9. Encapsulation
Encapsulation is the practice of bundling data (variables) and methods that operate on that data within a class, and restricting access to some of the object's components.
10. Law of Demeter (LoD)
This is also known as the principle of least knowledge. It states that objects should only talk to immediate objects and not expose their internals to other objects.
11. Principle of Least Privilege
This principle states that a user should only have access to the data or resources they need. This is done to minimize security risks.
12. Least Astonishment
The principle of least astonishment proposes that a component of a system should behave in a way that most users will expect it to behave, and therefore not astonish or surprise users. This helps developers create intuitive and easy-to-understand systems.
13. Fail Fast
A system should immediately report any failure as soon as it occurs, rather than attempting to continue execution with incorrect assumptions.
14. Design For Testing
Design your code in a way that makes it easy to write automated tests for it.
15. Favor Immutability
Favor using immutable objects - objects that cannot be modified after creation.
16. Favor composition over inheritance
It is better to compose objects to achieve polymorphic behavior and code reuse rather than inheriting from a base class.
17. Don’t modify global state
Avoid changing global variables as it can lead to unpredictable behaviour.
18. Use Meaningful Names
Class, methods and variables should have concise names that describe their use, making the code easy to understand.
19. Refactor Often
Clean and improve code regularly to make it simpler without changing its external behaviour.
20. Document As you Code
Clear documentation helps current and future programmers to understand the purpose and behaviour of your code.
21. Version Control
Always use version control to monitor changes in your code, enabling better collaboration and code management.
22. Automate repetitive tasks
Use automation tools for building, testing, and deployment to reduce errors and improve efficiency.
23. Code to an Interface, Not an Implementation
Rely on interfaces and abstractions rather than specific implementations. This increases flexibility and promotes loose coupling.
24. Minimize Side Effects
Aim for pure functions and methods with minimal side effects. This makes it easier to reason about code and avoid unintended consequences.
25. Avoid Long Parameter Lists
Too many parameters in a method call can lead to confusion. Where necessary, use objects to group related parameters.
Conclusion
Software design principles are crucial in the development of sustainable software applications. By adopting these practices, developers can consistently create more efficient, maintainable, and high-quality code.
Thank you for reading! If you found this article helpful and informative, please subscribe and give it a like; it helps support the content and keep you updated with future posts.
Subscribe to my newsletter
Read articles from Jadesola Adeagbo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
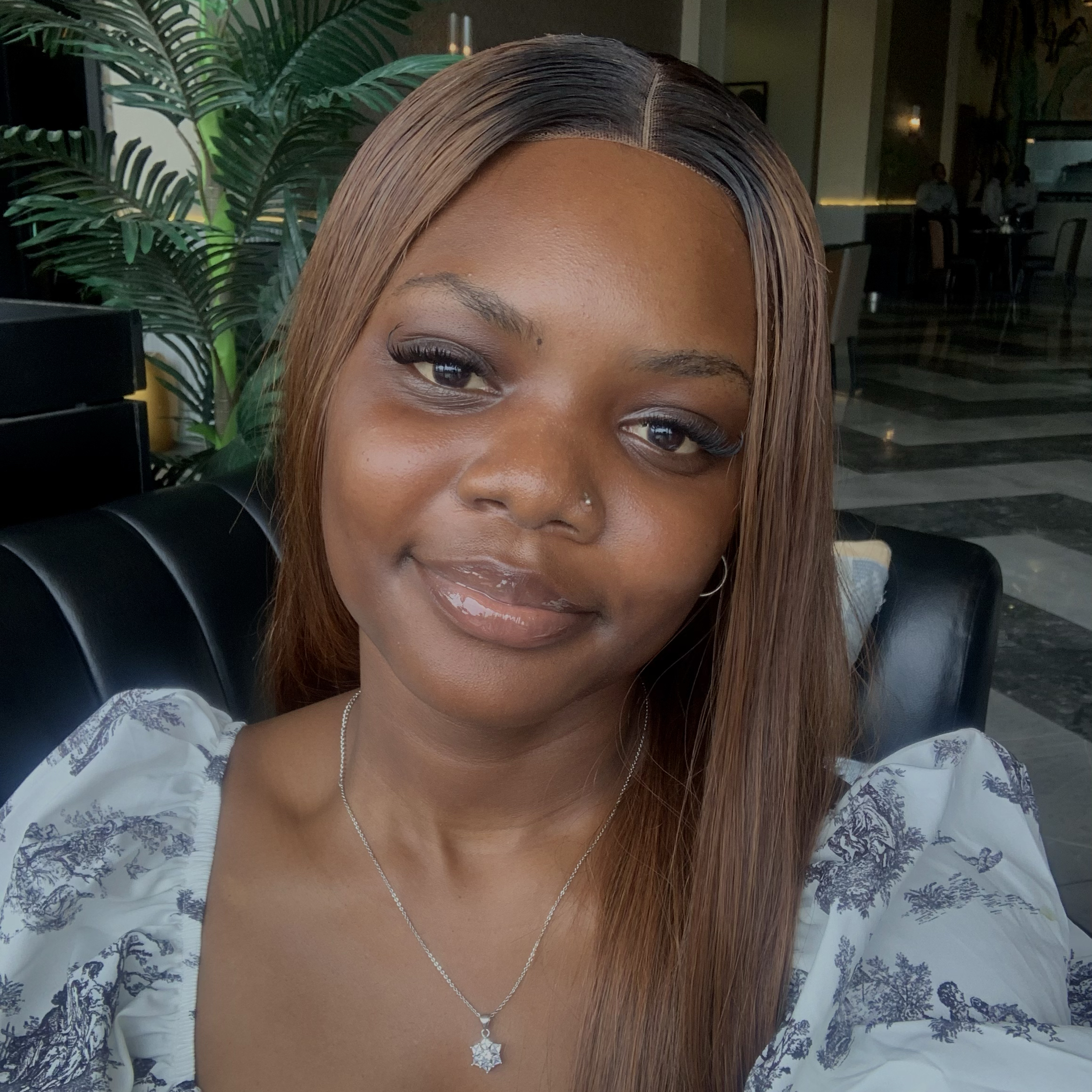
Jadesola Adeagbo
Jadesola Adeagbo
Hi🙋🏽♀️, I'm Jadesola, a software developer based in Nigeria 🛠️. Driven by a passion for solving problems with code, I'm currently refining my skills as a front-end developer while delving into the world of back-end development. I am dedicated to sharing my knowledge and experience as I grow in the tech world. Join me on my journey and let's grow together!