React and Responsiveness
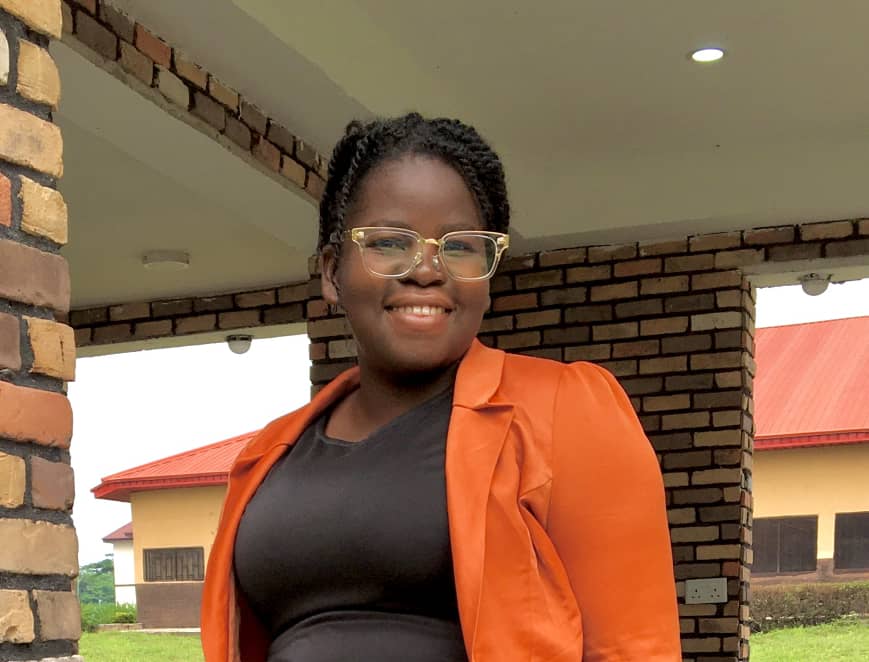
Introduction
React.js is a popular JavaScript library for building user interfaces, particularly single-page applications (SPAs). Developed and maintained by Facebook, React emphasizes component-based architecture, enabling developers to create reusable UI components that efficiently update and render as data changes. This reactivity, combined with the virtual DOM, ensures better performance and maintainability. Responsiveness is a key aspect of React, ensuring that those interfaces work well across various screen sizes and devices. It involves techniques and strategies that allow components to adapt seamlessly to different viewports, enhancing user experience regardless of the platform.
Importance of Responsiveness in Web Design
Responsiveness in web design is the capability of a website or application to adjust to various screen sizes and devices, providing a smooth user experience across desktops, tablets, and smartphones. As the range of devices used to access websites continues to expand, developing responsive user interfaces has become essential for enhancing user engagement and ensuring accessibility.
Essential Concept for Building Responsive React.js Applications
CSS Media Queries: The most common method for implementing responsive designs is using CSS media queries. Media queries apply different styles based on the screen's width, height, resolution, and orientation. Example:
@media (max-width: 768px) { .navbar { flex-direction: column; } }
Responsive Design Libraries: Several CSS frameworks and libraries integrate seamlessly with React to simplify responsive design. Some popular choices are:
Bootstrap: Provides pre-built responsive grid systems and components.
Tailwind CSS: is a utility-first framework that provides precise control over responsiveness. Tailwind will be discussed in more detail in the next article.
Ant Design: Offers built-in responsive components with customization options for grids, modals, tables, etc.
React Responsive Grid Systems: Many UI libraries offer grid systems that work well with React components to build responsive layouts:
Bootstrap Grid: Uses 12-column responsive grid system.
Ant Design Grid: Supports responsive breakpoints for layouts.
Material-UI Grid: Provides a highly customizable flexbox-based grid.
React Responsive Design Tools and Libraries:
- react-responsive: A popular utility for media query detection in React components, allowing you to render components based on screen size. Example:
import { useMediaQuery } from 'react-responsive';
const ExampleComponent = () => {
const isMobile = useMediaQuery({ query: '(max-width: 767px)' });
return <div>{isMobile ? 'Mobile View' : 'Desktop View'}</div>;
};
react-device-detect: A library that provides device-specific rendering in React. It helps detect the device type and customize the UI accordingly.
- Flexbox and CSS Grid Layouts: Flexbox and CSS Grid are powerful CSS features for creating flexible, responsive layouts. Flexbox works best for one-dimensional layouts (either rows or columns), while CSS Grid is ideal for two-dimensional layouts (rows and columns).
Flexbox Example:
CSS Grid and Flexbox
Using Flexbox and CSS Grid in your React components enables dynamic layout adjustments. Flexbox is excellent for aligning items in one dimension (row or column), while Grid handles two-dimensional layouts.
.container {
display: flex;
flex-direction: row;
justify-content: space-between;
}
CSS Grid Example:
.grid-container {
display: grid;
grid-template-columns: repeat(auto-fill, minmax(200px, 1fr));
}
React Hooks for Responsiveness
You can leverage React's state and hooks, such as useState and useEffect, to dynamically adjust component behavior based on screen size. However, it’s important to note that useEffect may not always be recommended because of possible rendering issues.
import { useState, useEffect } from 'react';
const useWindowWidth = () => {
const [width, setWidth] = useState(window.innerWidth);
useEffect(() => {
const handleResize = () => setWidth(window.innerWidth);
window.addEventListener('resize', handleResize);
return () => window.removeEventListener('resize', handleResize);
}, []);
return width;
};
const ResponsiveComponent = () => {
const width = useWindowWidth();
return (
<div>
{width < 600 ? <MobileView /> : <DesktopView />}
</div>
);
};
Conclusion
Responsive design is a vital aspect of modern web development, providing a smooth experience on various devices. With its component-based architecture and support for a range of responsive design tools and libraries, React.js simplifies the process of creating applications that adjust seamlessly to diverse screen sizes and resolutions.
Subscribe to my newsletter
Read articles from FASUNHANMI PELUMI directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
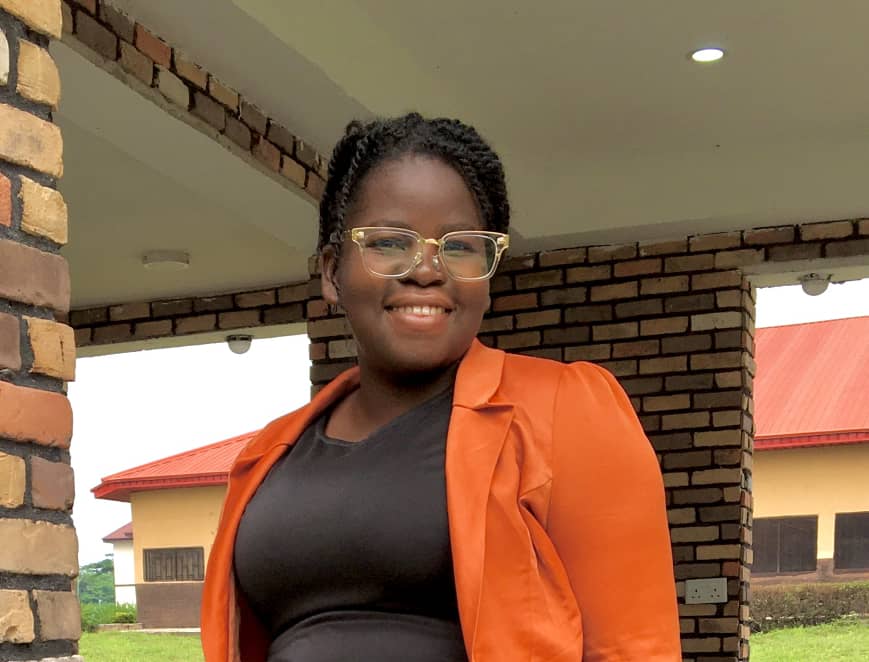