Understanding Object-Oriented Programming in Java

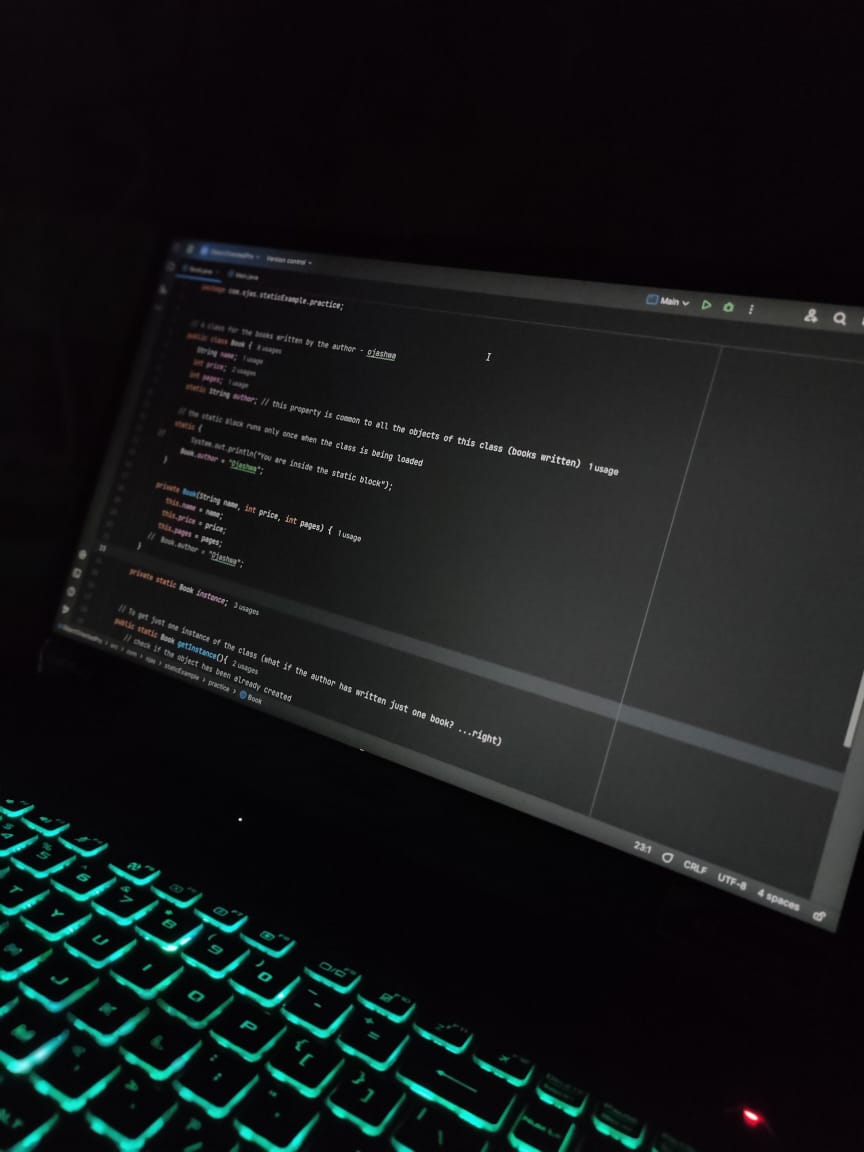
Hello, I'm Ojashwa, a member of Team Code Mosaic! Welcome to our very first blog post. As we embark on this journey to learn and grow as developers, we’ll be sharing what we discover along the way. In this post, I'll be diving into some key concepts of Object-Oriented Programming (OOP) in Java that I've recently explored, including Classes, Objects, Constructors, Wrapper Classes, and more. Whether you're a beginner or just brushing up on your Java skills, I hope this helps you on your coding journey as well!
OOPS Introduction -
Object-Oriented Programming (OOP) is a fundamental approach in software development, helping developers organize code through the use of objects and classes. In Java, mastering OOP concepts is key to writing efficient, scalable, and reusable code. In this blog, we’ll walk through some of the core OOP principles like Classes, Objects, Constructors, and more.
Classes and Objects in Java -
The first key concept that i came across is ‘class’, what is a class? - Classes in java are the blueprints to objects; they are the template (a schema) upon which an object is to be made (object here is an actual physical thing that we will soon explore) which actually consumes space in memory.
For example, a blueprint for a student is created that that has some properties like roll no., name, and marks and so on. So, this named group of properties is nothing but a class.
class Student{
int rno;
String name;
float marks;
}
- Now as for objects based on the class template, we can create them via using the ‘new’ keyword along with the constructor - constructor here is a method that determines what happens when an object is being created.
// creating a class
class Student{
//declaring instance variables
int rno;
String name;
float marks;
Student(int rno, String name, float marks){
this.rno = rno;
this.name = name;
this.marks = marks;
}
}
class Main{
public static void main(String[] args){
// this creates an object with reference variable named student1
Student student1 = new Student(318, "Random name", 78.89f);
System.out.println(student1.name);
}
}
Wrapper Classes and Singleton Class in Java
- A very useful concept i came across was this wrapper class, now we know that primitive data types as int, float, char etc. are not objects in java but what if we wanted them to be, for that purpose we can use the wrapper class which allows us to do it.
public class Main {
public static void main(String[] args) {
int num = 5;
// Wrapper class example
Integer numObj = 45;
System.out.println("Wrapper class object: " + numObj);
}
}
Classes allows us to create objects with properties defined in the class instance field (instance variables), but what if we wanted just one object to be created from the class, even if we were to create multiple objects only one instance of the class will be returned to us.
So instead of using constructors, we can create a method named as getInstance() which will do the task of creating that one instance of the class -
class Singleton {
private static Singleton instance;
// Private constructor prevents instantiation
private Singleton() {}
// this method will check if the instance of the object already exists or not
// if it does, it will return the same instance instead of creatong a new object.
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
public void showMessage() {
System.out.println("Singleton instance");
}
}
public class Main {
public static void main(String[] args) {
Singleton obj = Singleton.getInstance();
// static method is being accessed through the class name to get the instance of
// the class
obj.showMessage();
}
}
Throughout my journey in learning Object-Oriented Programming in Java, I've been following the tutorials of Kunal Kushwaha (youtube) for clear and beginner-friendly explanations. His content has been a valuable guide, and I highly recommend checking out his videos if you're looking to strengthen your understanding of Java and other tech concepts. #DSAwithKunal #OOPs #Interview
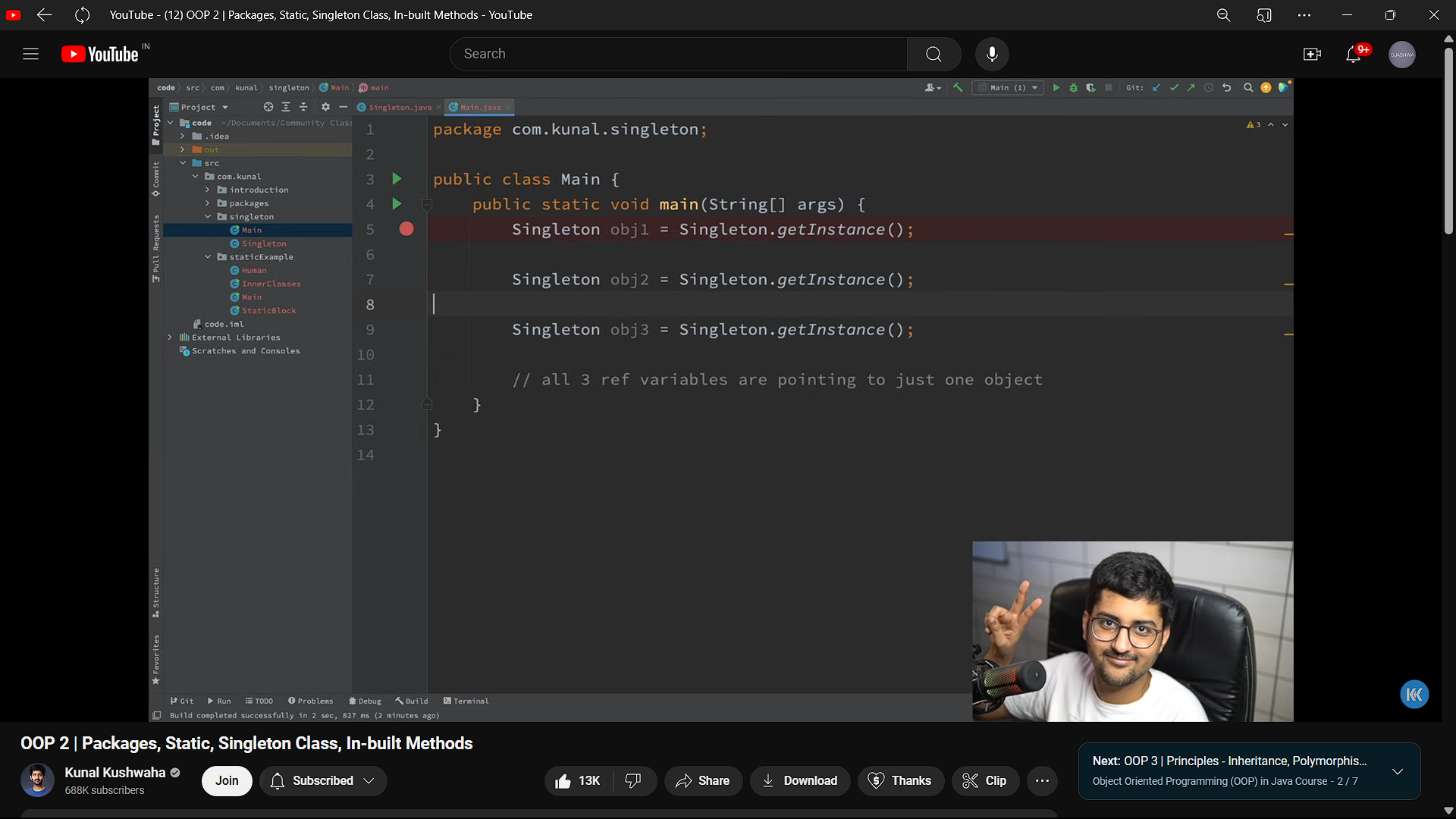
Subscribe to my newsletter
Read articles from Ojashwa Tripathi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
