Using Angular Helper Functions: Direct Access vs. Pipes
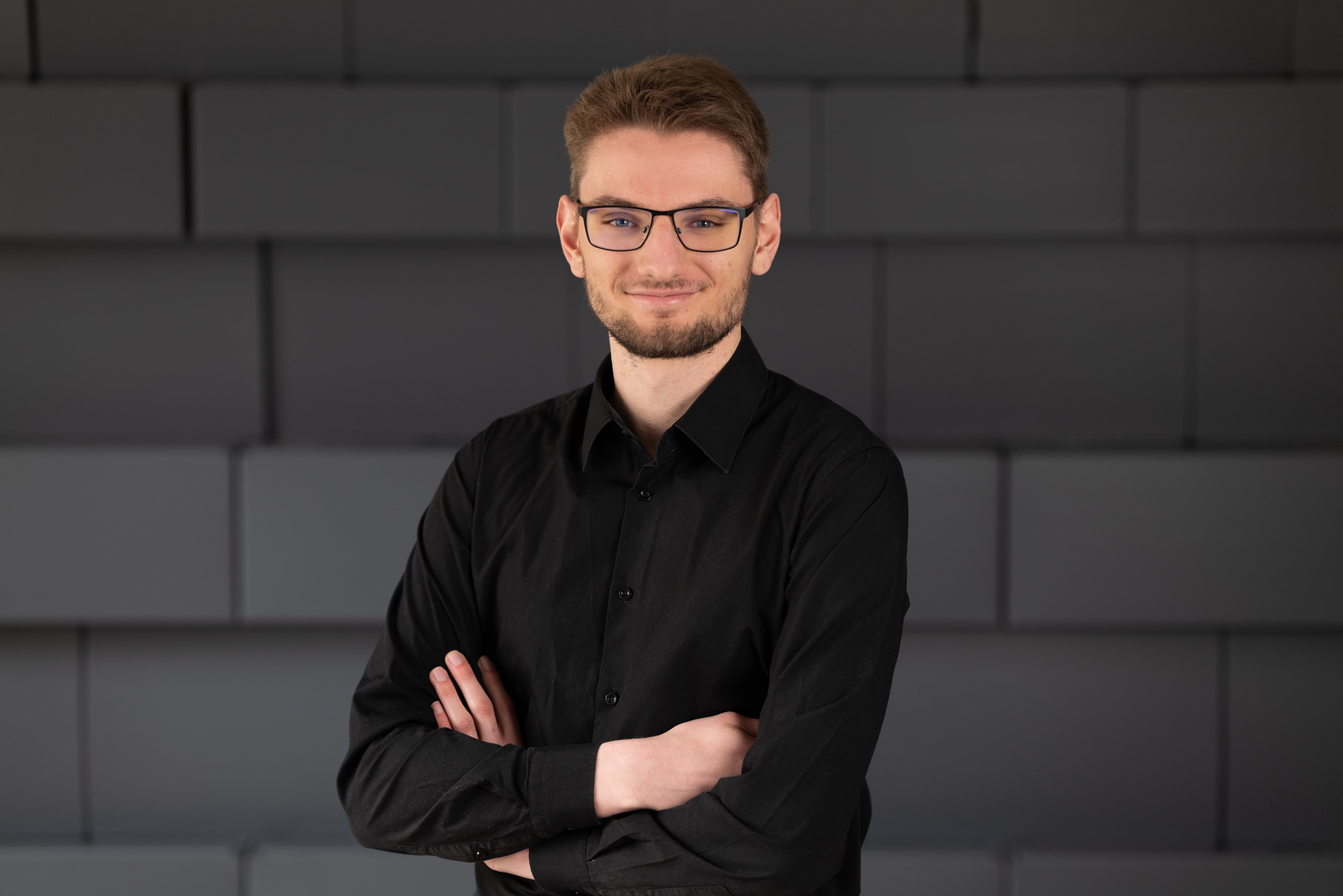
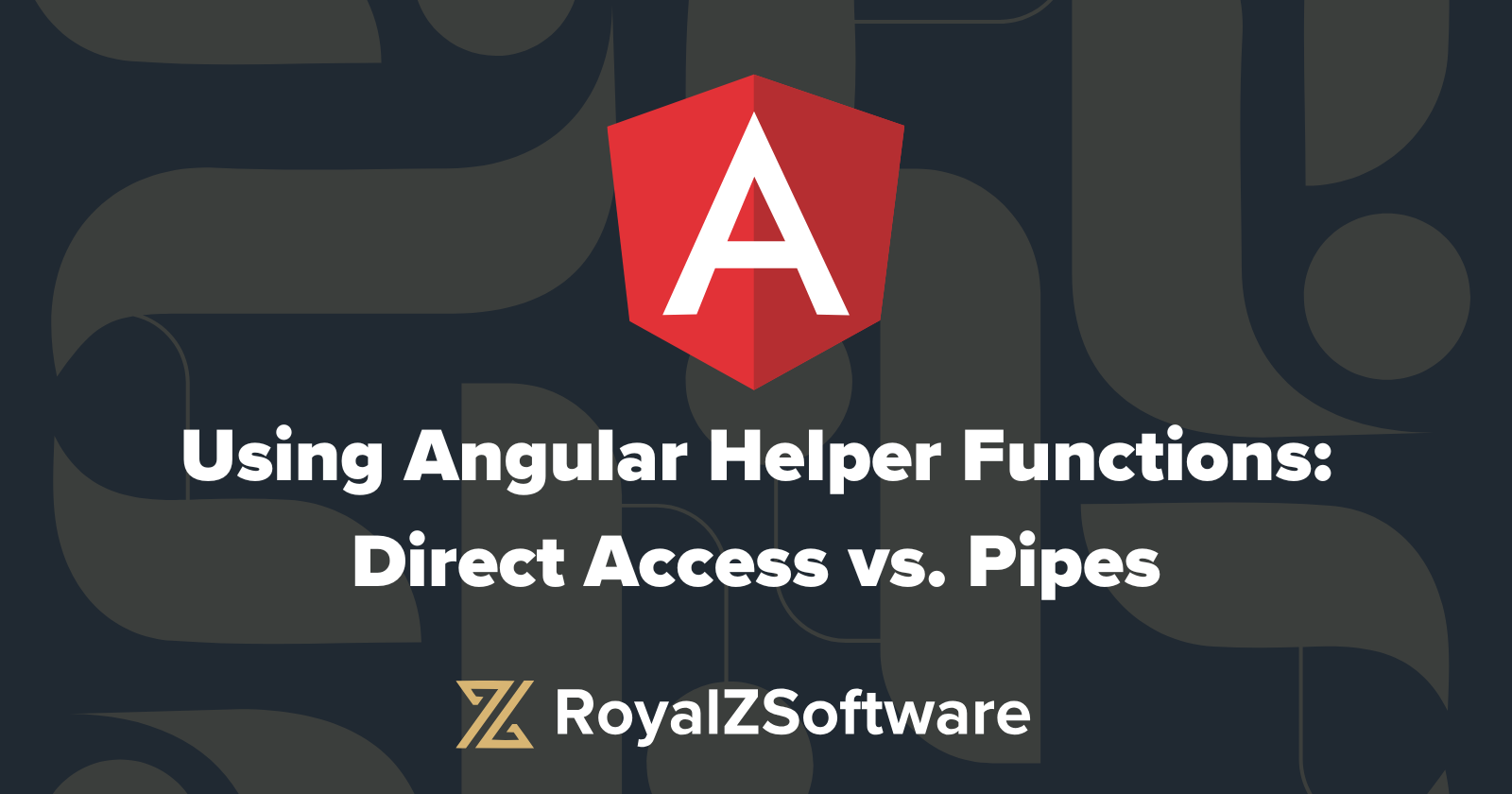
In Angular, it’s common to need helper functions or utility methods to transform or calculate data directly within your templates.
While these functions often reside in services, there are times when you don’t want or need to inject them into components, especially if they’re simple, static methods.
So how do you use these helper functions effectively in Angular templates? Two main approaches are available: using pipes or assigning them to instance variables for direct access.
Pipes: The Preferred Approach for Helper Functions
When you have a helper function that transforms data, a custom Angular pipe is often the best solution. Pipes are reusable, clean, and declarative, making them ideal for repetitive tasks like formatting dates, filtering data, or manipulating text.
For example, if you have a helper function that formats a string to title case, you can easily create a custom pipe:
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({name: 'titleCase'})
export class TitleCasePipe implements PipeTransform {
transform(value: string): string {
return value.replace(/\b\w/g, char => char.toUpperCase());
}
}
In your template, you simply use the pipe like this:
<p>{{ someText | titleCase }}</p>
Be aware that you need to import the pipe in the module where the template controller is registered.
UserPageComponent → AppModule or Standalone.
AppModule: declarations: [TitleCasePipe]
if standalone: make the pipe standalone aswell and import the pipe in the standalone component
Direct Access to Helper Functions
There are cases where creating a pipe might feel unnecessary, especially if the function is used only once or twice. In such cases, you can assign the helper method or class to a property in the component controller (TypeScript class) and access it directly in your template.
Here's an example:
import { Component } from '@angular/core';
import { MyHelperClass } from './helpers/my-helper-class';
@Component({
selector: 'app-example',
templateUrl: './example.component.html'
})
export class ExampleComponent {
helperInstance = MyHelperClass;
}
And then, in your template:
<p>{{ helperInstance.someStaticMethod(someInput) }}</p>
This approach provides quick access to static methods without the overhead of creating a pipe, especially for simple transformations or calculations that don’t require reusability.
Choosing Between Pipes and Direct Access
When deciding between pipes and direct access, consider these factors:
Reusability: Pipes shine when you need to apply the transformation across multiple places.
Performance: Pipes are optimized for change detection, while direct access might require recalculating the value each time the template re-renders.
Simplicity: If the helper function is used only once, direct access may be more straightforward than creating a new pipe.
Conclusion
Both pipes and direct access provide powerful ways to use helper functions in Angular templates. For reusable and complex transformations, pipes are the way to go. For simpler, one-off functions, direct access can be a quick and efficient solution.
If you want to dive deeper into Angular best practices, make sure to sign up for our newsletter! We share tips, tricks, and advanced techniques to help you level up your Angular development skills.
Subscribe to my newsletter
Read articles from Alexander Panov directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
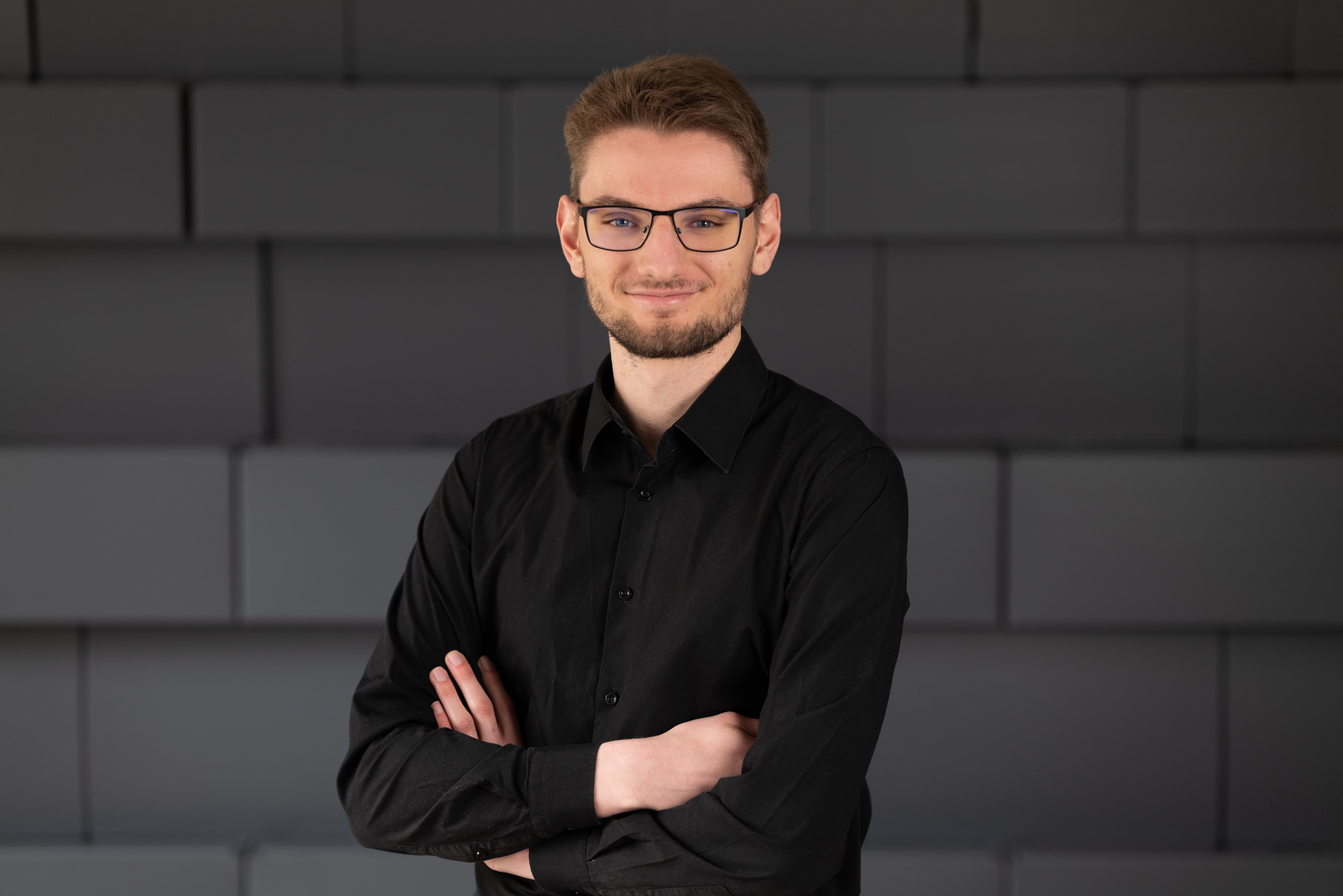
Alexander Panov
Alexander Panov
Software developer and CEO at RoyalZSoftware. I build web applications for startups with Ruby on Rails, Angular and React.