How to Implement Rate Limiting in Node.js APIs

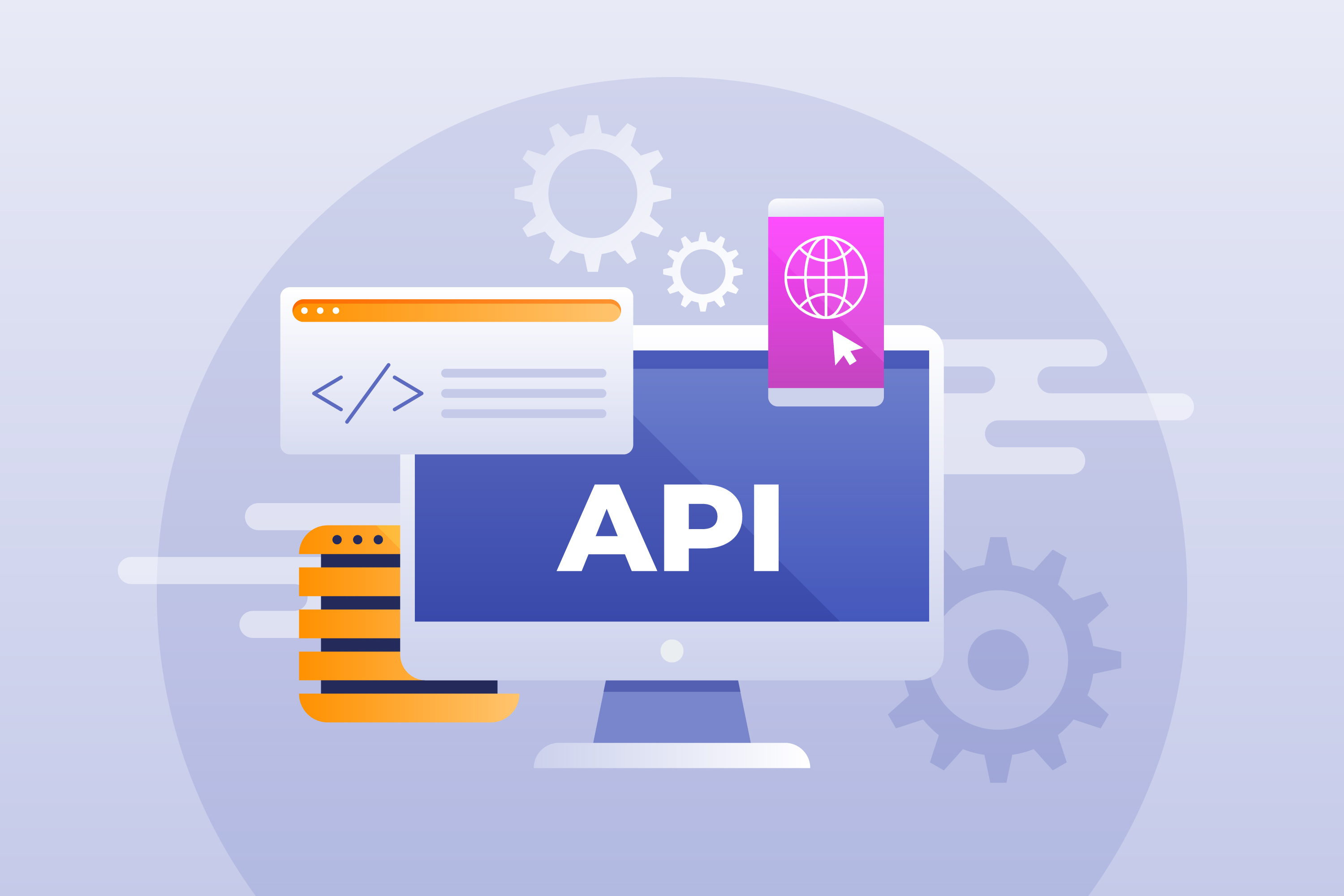
Rate limiting is a crucial technique for managing the number of requests that a server can handle within a specific timeframe. In Node.js backend APIs, it's essential to implement rate limiting to prevent abuse, protect resources, and ensure optimal performance.
Understanding Rate Limiting
Rate limiting involves setting limits on the number of requests a client can make within a given time window. This helps prevent excessive load on the server and ensures fair resource allocation among different clients.
Given that 32% of web traffic comes from malicious bots, rate limiting protects servers from abuse.
This article will provide a comprehensive guide on how to implement rate limiting in Node.js. Let’s get started!
rate-limiting-express-api/
|-- controllers/
| |-- apiController.js
|
|-- middleware/
| |-- rateLimiter.js
|
|-- routes/
| |-- apiRoutes.js
|
|-- index.js
|-- package.json
Step 1: Project setup
Create a new project folder and navigate into it by running the following commands:
mkdir rate-limiting-express-api
cd rate-limiting-express-api
Initialize a Node.js project and install dependencies:
npm init -y
npm install express cors express-rate-limit
In this example, we will use express-rate-limit package to limit requests to our API.
Step 2: Create the express server
Create an index.js file and add the following code:
const express = require('express');
const cors = require('cors');
const apiRoutes = require('./routes/apiRoutes');
const app = express();
const port = 3000;
app.use(cors());
app.use('/api', apiRoutes);
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
Step 3: Implement rate limiting middleware
Inside the middleware folder, create a new file called rateLimiter.js and add the following code:
const rateLimit = require('express-rate-limit');
const limiter = rateLimit({
windowMs: 60 * 1000, // 1 minute
max: 10, // maximum number of requests allowed in the windowMs
message: 'Too many requests, please try again later.',
});
module.exports = limiter;
Step 4: Create API routes and controller
Inside the routes folder, create apiRoutes.js and add the following code:
const express = require('express');
const apiController = require('../controllers/apiController');
const rateLimiter = require('../middleware/rateLimiter');
const router = express.Router();
router.get('/data', rateLimiter, apiController.getData);
module.exports = router;
Inside the controllers folder, create a new file apiController.js and add the following code:
const getData = (req, res) => {
res.json({ data: 'welcome to rate limiting in express apis' });
};
module.exports = { getData };
Step 5: Test the API
It’s finally time to test your API.
To start the server, run the following command.
node index.js
In your web browser, navigate to http://localhost:3000/api/data. You should see the API response message of 'welcome to rate limiting in express apis’.
The rate limiting middleware you implemented restricts the number of requests to 10 per minute. If the number of requests exceeds 10 per minute, the user will receive a warning - “Too many requests, please try again later”.
Congratulations! You have successfully secured your API with rate limiting.
Please note that this is a basic example of rate limiting using the express-rate-limit package. Its configurations can be further modified to meet your specific needs.
Thanks for reading. And happy coding!
Subscribe to my newsletter
Read articles from Chidi Okeke directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
