The Complete Guide to Building and Scaling Rails SaaS MVP in 2024

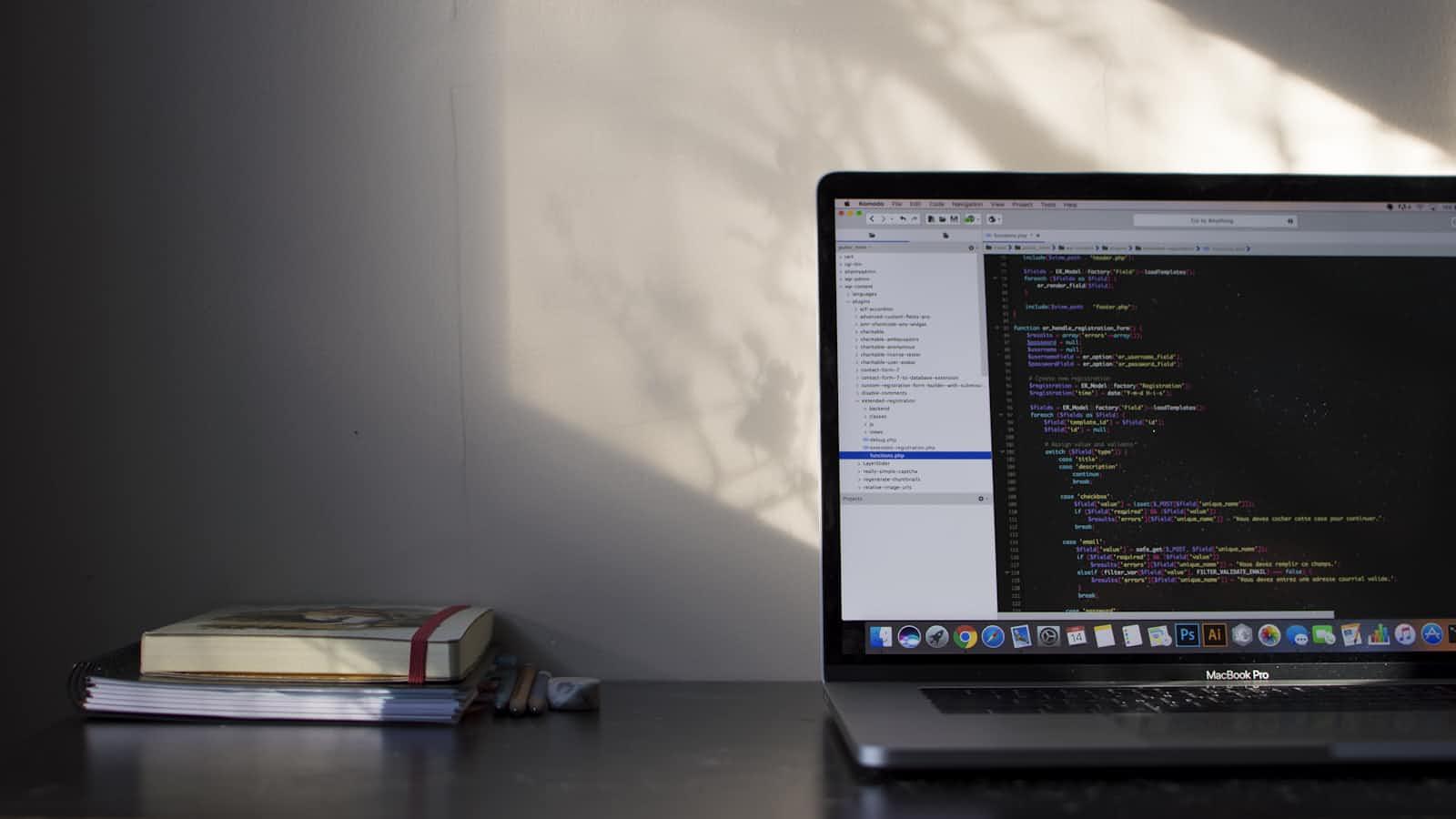
Part 1: 8 Rules to Ship Your Rails 8 SaaS MVP
Many books and articles have been written about MVPs since Eric Ries coined the term "Minimum Viable Product". In 2024, with Rails 8's powerful capabilities, these rules are more relevant than ever for getting your SaaS to market quickly and effectively.
1. Focus on Your Core Value Proposition
The foundation of any successful MVP is laser-focusing on your product's essential value. Strip away everything that isn't directly tied to solving your customers' primary pain point. Your product should have one or two key features that customers would happily pay for – nothing more.
Many founders in 2024's competitive SaaS landscape still struggle with this minimalist approach. Despite the temptation to compete feature-for-feature with established players, remember: your initial goal is proving your core value proposition, not matching your competitors' feature sets.
For example - Basic Authentication with Rails 8
# app/models/user.rb
class User < ApplicationRecord
has_secure_password
validates :email, presence: true, uniqueness: true, format: { with: URI::MailTo::EMAIL_REGEXP }
validates :password, length: { minimum: 8 }, if: -> { new_record? || changes[:password_digest] }
end
# app/controllers/application_controller.rb
class ApplicationController < ActionController::Base
include Authentication
private
def current_user
@current_user ||= User.find_by(id: session[:user_id]) if session[:user_id]
end
def require_login
redirect_to login_path, alert: "Please log in" unless current_user
end
end
2. Ruthlessly Postpone Common Features
Even in 2024, many "standard" SaaS features can wait. Modern users understand that early-access products may have some rough edges. Features like password reset flows, user profile customization, and team management can often be handled manually at first.
Instead of building automated systems, consider managing these processes through support channels. Tools like Linear or Height can help track manual interventions until automation becomes necessary. Remember: automation without validation is premature optimization.
3. Leverage Familiar Technology
Rails 8 brings significant improvements in performance and developer experience, but the core philosophy remains: use what you know. The modern Rails stack with Ruby 3.3+, PostgreSQL, Hotwire (Turbo + Stimulus), and TailwindCSS continues to be a powerful combination for rapid MVP development.
Rails 8's built-in authentication system, improved caching, and better error handling make it even more suitable for quick MVP deployment. The ecosystem's maturity means you'll find gems and solutions for almost any common problem.
For example - Basic View Components with Hotwire
# app/components/dashboard_card_component.rb
class DashboardCardComponent < ViewComponent::Base
def initialize(title:, data:)
@title = title
@data = data
end
end
# app/components/dashboard_card_component.html.erb
<div class="p-4 bg-white rounded-lg shadow">
<h3 class="text-lg font-semibold"><%= @title %></h3>
<div class="mt-2">
<%= @data %>
</div>
</div>
4. Embrace Platform-as-a-Service
While container orchestration and serverless architectures are trending, not PaaS solutions like Heroku but VPS servers, along with Kamal, remain optimal for MVPs in 2024. They handle infrastructure complexities while keeping costs predictable. Rails 8's improved performance means you can run longer on basic tiers, often starting at $7-10/month.
Take advantage of modern PaaS features like automatic SSL, CDN integration, and managed databases to focus on your product instead of infrastructure.
5. Integrate No-Code Tools Strategically
The no-code ecosystem has matured significantly. Tools like Zapier, Make (formerly Integromat), or n8n can handle complex workflows without custom code. Consider using:
Resend or Postmark for transactional emails
PostHog for analytics
Cal.com for scheduling
Knock for notifications
6. Design for Future Expansion
While keeping things minimal, establish foundational patterns that support future growth. Rails 8's improved modularity and namespace features make this easier than ever. For example:
Use concerns and service objects judiciously
Implement multi-tenancy patterns early
Structure your database with future team features in mind
Leverage Rails 8's new query interface for efficient data access
For example - Improved Caching
# app/models/dashboard_stats.rb
class DashboardStats
include ActiveModel::Model
def total_revenue
Rails.cache.fetch("dashboard_stats/revenue", expires_in: 1.hour) do
Subscription.successful.sum(:amount)
end
end
def active_users
Rails.cache.fetch("dashboard_stats/active_users", expires_in: 15.minutes) do
User.active.count
end
end
end
7. Embrace Minimalist UI
With Rails 8's ViewComponent support and modern TailwindCSS, creating a clean, professional UI is easier than ever. Focus on:
Consistent spacing and typography
Clear visual hierarchy
Mobile-first responsiveness
Essential interactive elements
8. Keep Billing Simple
Modern billing tools like Stripe have evolved significantly, but the principle remains: start with a single plan. Rails 8's improved background job processing makes handling webhooks and subscription events more reliable.
For example - Simple Subscription Setup with Stripe
# config/initializers/stripe.rb
Stripe.api_key = Rails.application.credentials.stripe[:secret_key]
# app/models/subscription.rb
class Subscription < ApplicationRecord
belongs_to :user
def process_payment(payment_method_id)
customer = Stripe::Customer.create(
email: user.email,
payment_method: payment_method_id,
invoice_settings: { default_payment_method: payment_method_id }
)
Stripe::Subscription.create(
customer: customer.id,
items: [{ price: 'price_H5ggYwtDq4fbrJ' }],
expand: ['latest_invoice.payment_intent']
)
rescue Stripe::StripeError => e
errors.add(:base, e.message)
false
end
end
Part 2: Scaling from MVP to MSP (Minimum Sellable Product)
Understanding the Product Evolution
The journey from MVP to a fully mature product follows a clear progression:
MVP (Minimum Viable Product)
Tests core value proposition
Validates basic assumptions
Appeals to early adopters
MSP (Minimum Sellable Product)
Adds essential reliability
Includes must-have features
Focuses on core user experience
MMP (Minimum Marketable Product)
Polished for broader market
Competitive feature set
Ready for scaling
Key Focus Areas for MSP Development
1. Essential Feature Completion
Complete any half-implemented features from MVP
Add crucial missing functionality
Ensure core workflows are smooth
2. Reliability Improvements
Implement proper error tracking
Add system health monitoring
Ensure data consistency
Implement backup systems
3. User Experience Refinement
Smooth out rough edges in UI
Add basic onboarding
Implement essential feedback mechanisms
Ensure mobile responsiveness
4. Basic Support Infrastructure
Set up help documentation
Implement basic support channels
Create essential admin tools
5. Payment and Billing
Implement proper billing cycles
Add invoice generation
Set up payment failure handling
Evaluating MSP Readiness
Ask yourself these questions before considering your product an MSP:
Can customers successfully use the product without direct support?
Is the UI self-explanatory?
Are there clear error messages?
Is basic documentation available?
Is the product reliable enough for daily use?
Are there any critical bugs?
Is performance acceptable?
Is data being saved and processed correctly?
Can you effectively charge for the product?
Is billing working properly?
Are subscriptions handled correctly?
Can customers manage their billing information?
Does it solve the core problem better than alternatives?
What's your competitive advantage?
Are core features complete?
Is the user experience satisfactory?
Common MSP Pitfalls to Avoid
Feature Creep
Stick to essential features
Resist adding "nice-to-have" features
Focus on core value proposition
Over-engineering
Keep technical solutions simple
Avoid premature optimization
Build for current needs, not future scale
Perfectionism
Accept that some rough edges are okay
Focus on functionality over polish
Remember it's still a stepping stone
Ignoring Early Feedback
Continue gathering user feedback
Stay responsive to critical issues
Keep channels open with early adopters
Technical Considerations for Rails 8 MSPs
Performance Optimization
# Use Rails 8's improved caching class ProductsController < ApplicationController def index @products = Product.all.with_rich_text_description fresh_when last_modified: @products.maximum(:updated_at) end end
Error Handling
# Implement proper error boundaries rescue_from ActiveRecord::RecordNotFound do |e| respond_to do |format| format.html { redirect_to root_path, alert: "Resource not found" } format.json { render json: { error: "Not found" }, status: :not_found } end end
Background Jobs
# Use Rails 8's improved ActiveJob class ProcessSubscriptionJob < ApplicationJob retry_on Stripe::Error, wait: :exponentially_longer, attempts: 3 def perform(subscription_id) # Process subscription end end
Transition Plan Template
Week 1-2: Assessment
Audit MVP feedback
List critical missing features
Identify performance bottlenecks
Week 3-4: Core Improvements
Implement critical features
Fix major bugs
Add essential monitoring
Week 5-6: User Experience
Improve UI/UX
Add basic onboarding
Implement feedback mechanisms
Week 7-8: Testing and Polish
Comprehensive testing
Performance optimization
Documentation creation
Remember: The goal of an MSP is to create a product that customers can use independently and are willing to pay for. It doesn't need to be perfect, but it needs to be reliable and complete enough to provide real value.
Part 3: Building Your MMP (Minimum Marketable Product)
Requirements for MMP Success
Customer Focus
Solve real customer problems
Add features that provide clear value
Ensure usability meets market expectations
Build competitive advantages
Technical Requirements
Performance optimization
Scalability planning
Reliability improvements
Thorough testing
Business Requirements
Clear monetization strategy
Market differentiation
Support infrastructure
Marketing readiness
Prioritizing Development Work
Address Critical Pain Points
Focus on customer feedback
Solve major usability issues
Improve core workflows
Add Market-Required Features
Implement industry-standard features
Add competitive differentiators
Enhance user experience
Prepare for Scale
Optimize performance
Improve infrastructure
Enhance monitoring
Part 4: What Comes Next
Establish Metrics and Monitoring
Set up error tracking
Implement proper logging and APM
Use analytics tools effectively
Monitor key business metrics
Technical Evolution
Audit and optimize performance
Enhance security measures
Improve scalability
Implement advanced features
Business Growth
Expand marketing efforts
Build sales processes
Develop customer success programs
Consider strategic partnerships
Continuous Improvement
Regular feature releases
Ongoing optimization
Customer feedback loops
Market adaptation
Final Thoughts
Building a successful SaaS product is a journey that extends far beyond the initial MVP. By following these guidelines and maintaining a focus on customer value throughout each stage, you'll be well-positioned to create a product that not only serves your customers' needs but also builds a sustainable business.
Remember that each stage - MVP, MSP, and MMP - serves a specific purpose in your product's evolution. Don't rush through them, but also don't get stuck perfecting any single stage. The key is to maintain momentum while ensuring each release provides real value to your customers.
Subscribe to my newsletter
Read articles from BestWeb Ventures directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

BestWeb Ventures
BestWeb Ventures
From cutting-edge web and app development, using Ruby on Rails, since 2005 to data-driven digital marketing strategies, we build, operate, and scale your MVP (Minimum Viable Product) for sustainable growth in today's competitive landscape.