Concurrency, Mutex, and Arc in Rust: A Comprehensive Guide
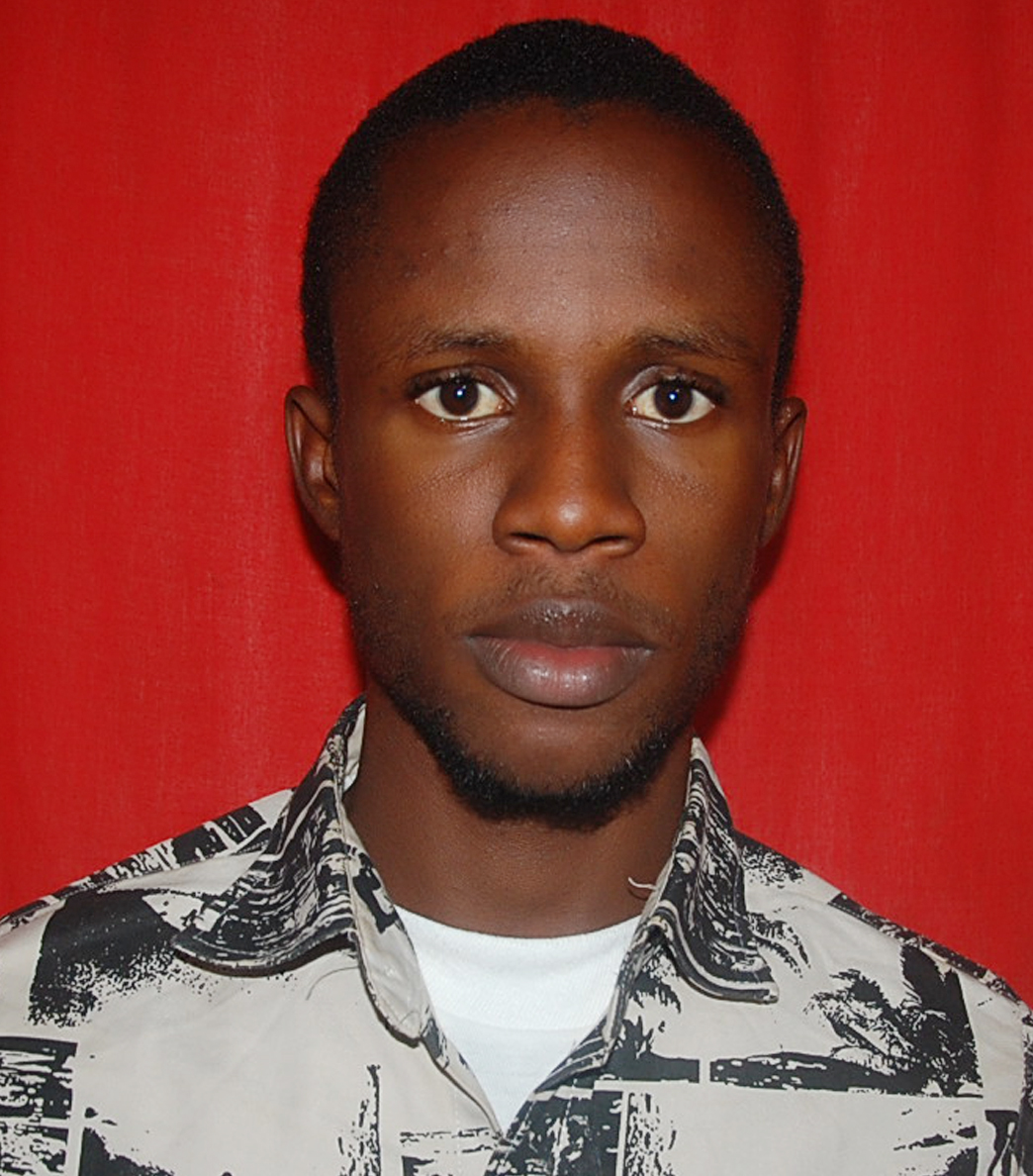
Introduction
Imagine you’re a conductor, leading an orchestra of a hundred musicians. Each musician is playing their part, but they need to harmonize, to come together at just the right moments. This is the essence of concurrency in programming, and Rust gives you the conductor’s baton.
Rust, a statically-typed systems programming language, is renowned for its focus on speed, memory safety, and parallelism. In software development, mastering concurrency and parallelism is crucial. This article delves into Rust’s powerful concurrency model — to ensure memory safety, with a particular focus on Arc
(Atomic Reference Counter) and Mutex
(Mutual Exclusion).
Understanding Concurrency in Rust
What is Concurrency?
Concurrency refers to the ability of different parts of a program to be executed independently and at once without affecting the final outcome. In Rust, this is primarily achieved through the use of threads and safe sharing mechanisms.
Threads in Rust
Rust provides a standard library module std::thread
for creating and managing threads. Let's look at a simple example:
use std::thread;
use std::time::Duration;
fn main() {
let handle = thread::spawn(|| {
for i in 1..5 {
println!("hi number {} from the spawned thread!", i);
thread::sleep(Duration::from_millis(1));
}
});
for i in 1..5 {
println!("hi number {} from the main thread!", i);
thread::sleep(Duration::from_millis(1));
}
handle.join().unwrap();
}
Let's break this down:
We use
thread::spawn
to create a new thread. It takes a closure containing the code to be executed in the new thread.Inside the spawned thread, we print numbers 1 to 4, with a small sleep between each print.
Concurrently, in the main thread, we do the same thing.
handle.join().unwrap()
ensures that the main thread waits for the spawned thread to finish before the program exits.
This example demonstrates basic thread creation and execution, showing how operations can run concurrently.
Arc (Atomic Reference Counter)
What is Arc?
Arc
stands for Atomic Reference Counter. It's a thread-safe reference-counting pointer that enables safe sharing of data across multiple threads. Arc
allows data to be shared safely between multiple threads, and the data is deallocated when the last reference to it is dropped.
Picture a grand concert hall, moments before a performance. In the center stands a lone figure — the sheet music distributor. This person holds the master copy of the symphony’s score. As musicians file in, each one needs their own copy of the music. The distributor doesn’t just hand out the master copy; instead, they make perfect duplicates, keeping track of every copy distributed.
Key Features of Arc
Shared Ownership: Multiple threads can own a reference to the same data.
Thread-Safe Reference Counting: The reference count is atomically updated, preventing data races.
Automatic Cleanup: The data is released when the last Arc pointing to it is dropped.
Example Usage of Arc
Let’s look at a more detailed example of using Arc
:
use std::sync::Arc;
use std::thread;
fn main() {
// Create an Arc containing a vector
let data = Arc::new(vec![1, 2, 3, 4, 5]);
let mut handles = vec![];
for i in 0..3 {
// Clone the Arc for each thread
let data_clone = Arc::clone(&data);
// Spawn a new thread
let handle = thread::spawn(move || {
println!("Thread {}: {:?}", i, *data_clone);
});
handles.push(handle);
}
// Wait for all threads to complete
for handle in handles {
handle.join().unwrap();
}
// Original data is still accessible here
println!("Original data: {:?}", *data);
}
Let’s break this down:
We create an
Arc
containing a vector usingArc::new()
.We spawn three threads, each getting its own clone of the
Arc
usingArc::clone()
.Each thread prints the data it sees.
We wait for all threads to complete using
handle.join().unwrap()
.After all threads complete, we can still access the original data.
This example showcases how Arc
allows multiple threads to safely share read-only access to data.
Mutex (Mutual Exclusion)
What is Mutex?
A Mutex (mutual exclusion) is a synchronization primitive that prevents multiple threads from concurrently accessing a shared resource.
Now, let’s introduce a new element to our orchestra: a solo microphone at the center of the stage. This microphone is special — it’s the only one that can capture the true essence of a soloist’s performance. But here’s the catch: only one musician can use it at a time.
Key Concepts of Mutex
Exclusive Access: Only one thread can access the protected data at a time.
Locking Mechanism: Threads must acquire a lock before accessing the data and release it afterward.
Blocking: If a thread tries to acquire a lock that’s already held, it will block until the lock becomes available.
Using Mutex
Here’s a detailed example of using Mutex
:
use std::sync::{Arc, Mutex};
use std::thread;
fn main() {
// Create a Mutex containing a vector, wrapped in an Arc
let data = Arc::new(Mutex::new(vec![]));
let mut handles = vec![];
for i in 0..5 {
// Clone the Arc for each thread
let data_clone = Arc::clone(&data);
// Spawn a new thread
let handle = thread::spawn(move || {
// Acquire the lock and get mutable access to the vector
let mut vec = data_clone.lock().unwrap();
vec.push(i);
// Lock is automatically released here when `vec` goes out of scope
});
handles.push(handle);
}
// Wait for all threads to complete
for handle in handles {
handle.join().unwrap();
}
// Print the final state of the vector
println!("Final data: {:?}", *data.lock().unwrap());
}
Let’s break this down:
We create a
Mutex
containing an empty vector, and wrap it in anArc
for shared ownership.We spawn five threads, each getting its own clone of the
Arc
.In each thread, we: (a). Acquire the lock using
lock()
. (b). Get mutable access to the vector. (c). Push a value onto the vector. (d). Automatically release the lock whenvec
goes out of scope.We wait for all threads to complete.
Finally, we print the contents of the vector, which now contains the numbers 0 to 4 in some order.
This example demonstrates how Mutex
allows multiple threads to safely modify shared data by ensuring exclusive access.
Combining Arc and Mutex
When you need shared ownership Arc
and mutability Mutex
) across threads, you often combine them as Arc<Mutex<T>>
. This pattern is so common that it's worth exploring in detail:
use std::sync::{Arc, Mutex};
use std::thread;
struct Counter {
count: i32,
}
fn main() {
// Create a Counter, wrap it in a Mutex, then in an Arc
let counter = Arc::new(Mutex::new(Counter { count: 0 }));
let mut handles = vec![];
for _ in 0..10 {
let counter_clone = Arc::clone(&counter);
let handle = thread::spawn(move || {
// Acquire the lock and modify the counter
let mut counter = counter_clone.lock().unwrap();
counter.count += 1;
// Lock is released here
});
handles.push(handle);
}
// Wait for all threads to complete
for handle in handles {
handle.join().unwrap();
}
// Print the final count
println!("Final count: {}", counter.lock().unwrap().count);
}
In this example:
We define a
Counter
struct with a singlecount
field.We create an instance of
Counter
, wrap it in aMutex
, and then wrap that in anArc
.We spawn 10 threads, each incrementing the counter.
Each thread: (a). Clones the
Arc
(b). Acquires the lock (c). Increments the counter (d). Releases the lock (automatically whencounter
goes out of scope)After all threads complete, we print the final count, which should be 10.
This pattern allows multiple threads to safely share and modify the same data, combining the shared ownership of Arc
with the exclusive access of Mutex
.
Conclusion
Rust’s concurrency model, built on concepts like Arc
and Mutex
, provides a powerful and safe way to write concurrent programs. By leveraging these tools, you can write efficient, parallel code while avoiding common pitfalls like data races and deadlocks. Remember:
Use
Arc
when you need shared ownership across threads.Use
Mutex
when you need mutable access to shared data.Combine
Arc
andMutex
when you need both shared ownership and mutable access.
While these tools are powerful, they should be used carefully. Always consider the simplest solution first, and reach for concurrency primitives when they truly solve your problem more effectively.
If you found this article helpful I would appreciate some claps 👏👏👏👏. I would like to connect with you, here are my social media links; LinkedIn, Twitter, and Github.
Subscribe to my newsletter
Read articles from Abiodun Awoyemi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
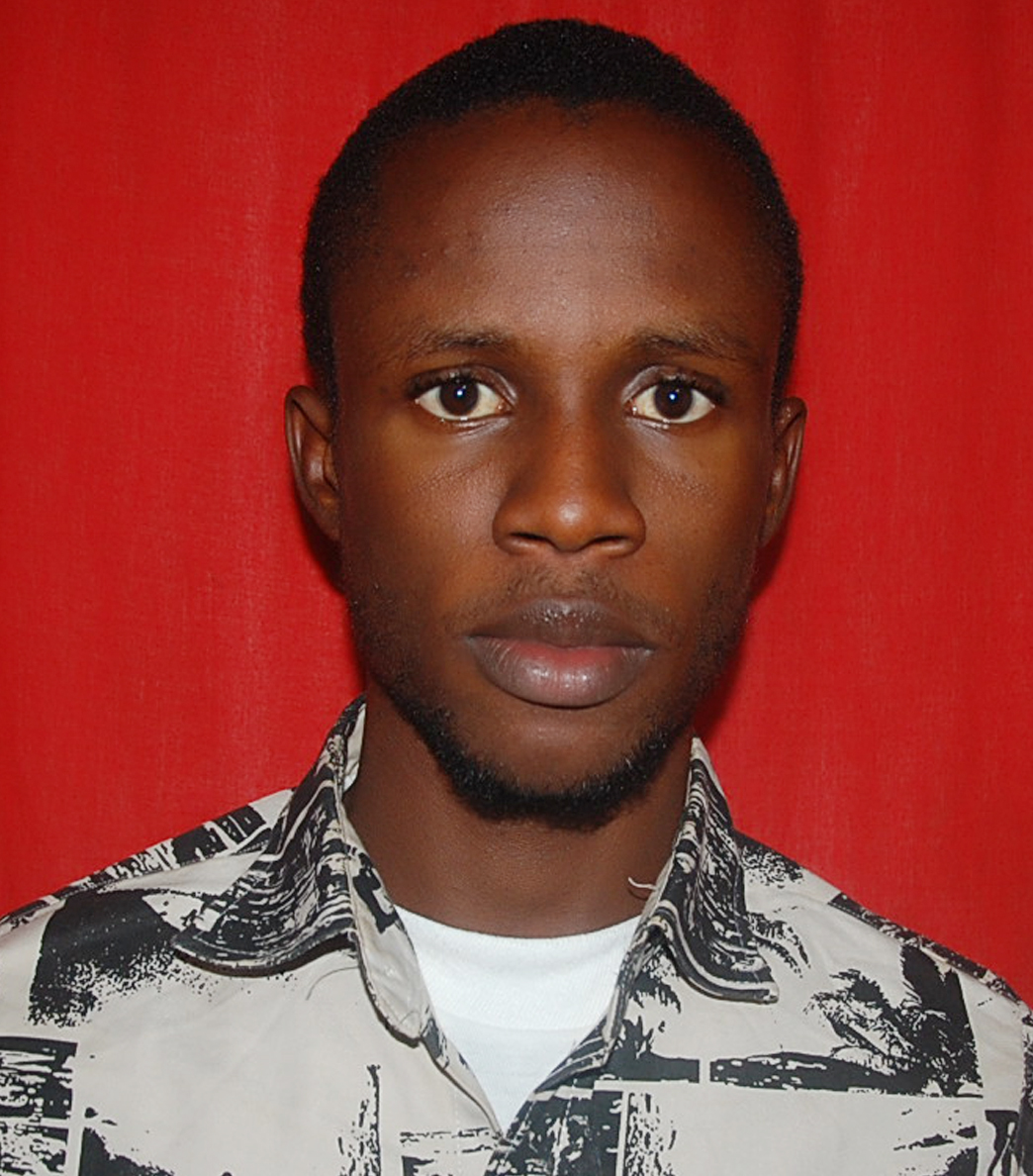