Day 6: Loops – Time Warp 🔄
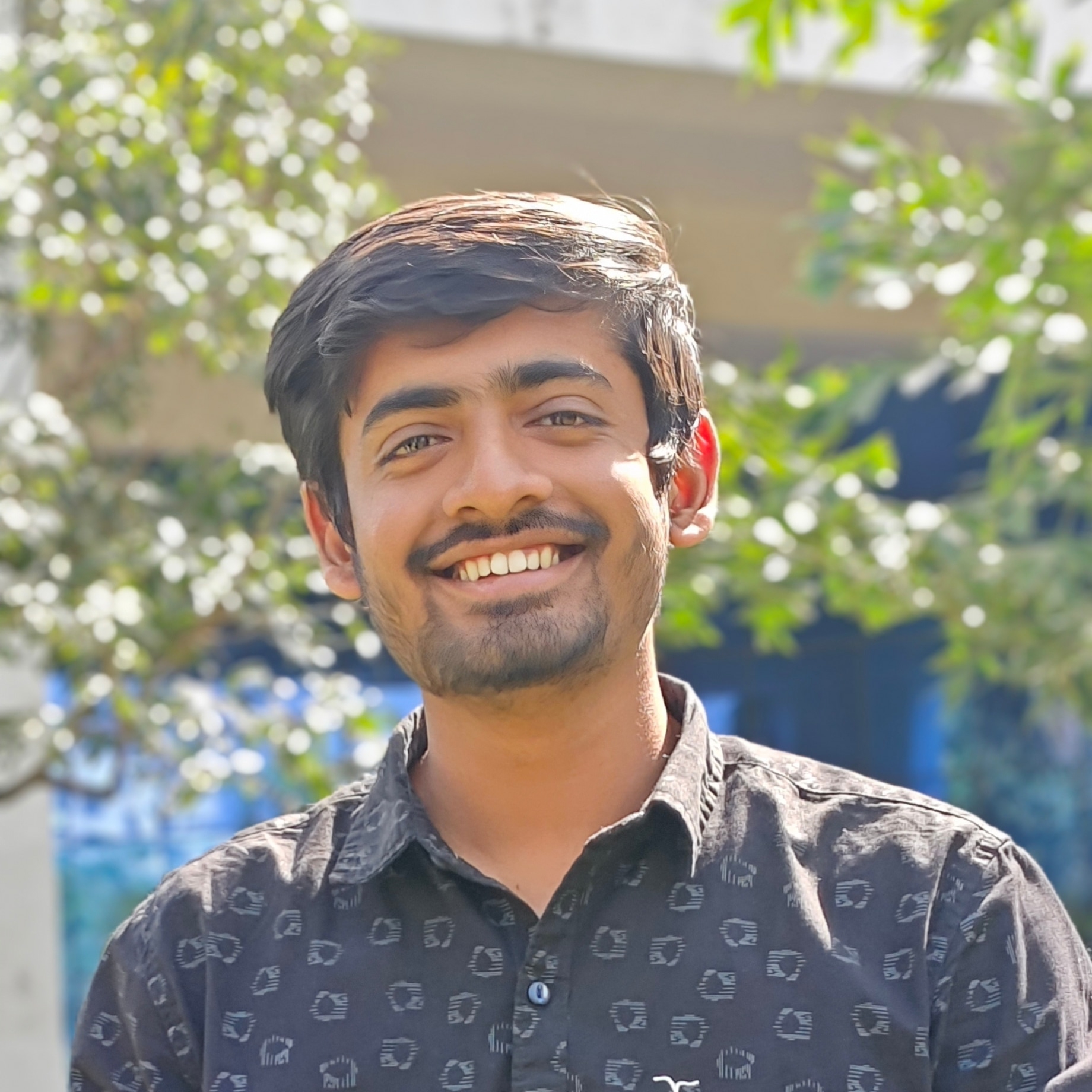
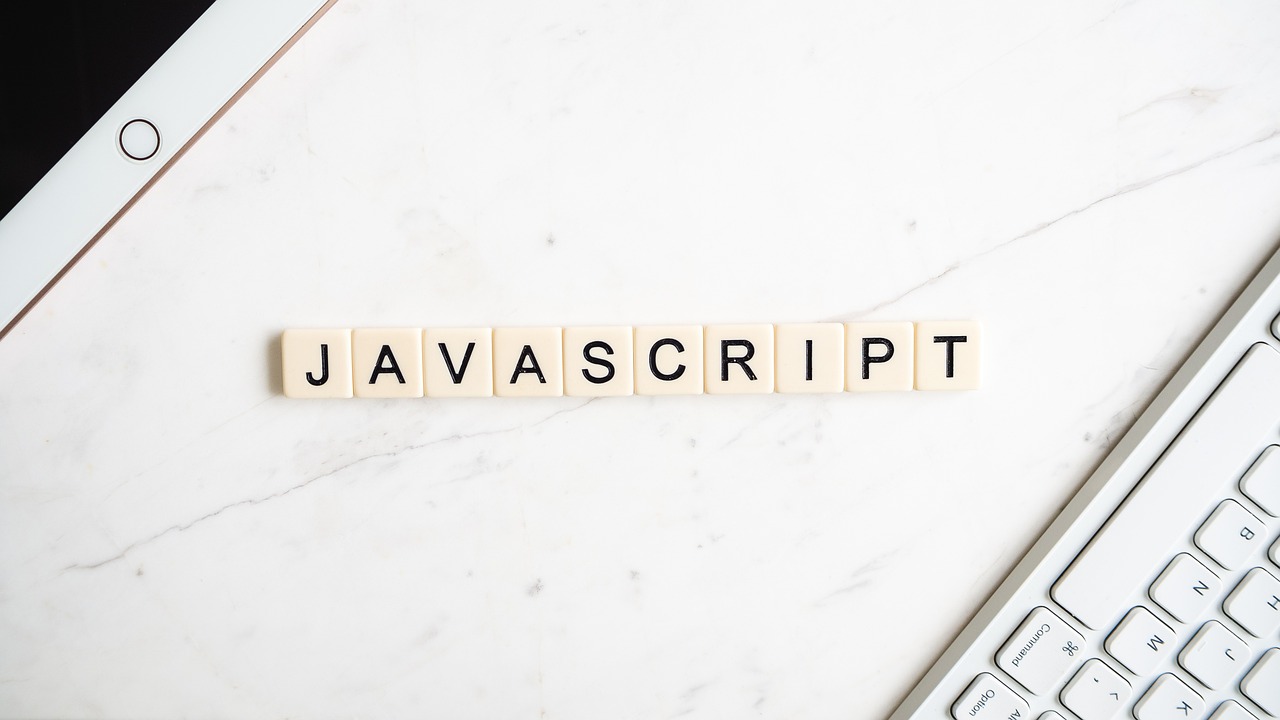
Welcome to Loop Central, where we learn to automate repetitive tasks like a pro. Imagine being able to "time travel" through an array of anime characters with just a few lines of code. Loops let you do just that! In this session, you'll understand the three core loops in JavaScript:
for loop
while loop
do-while loop
By the end, you’ll know how to loop through any data like a true anime sensei.
Why Loops?
Instead of writing repetitive code, loops help you automate actions by repeatedly executing a block of code until a condition is no longer true. This can be useful for:
Printing lists (like favorite anime characters).
Iterating over arrays.
Automating tasks like countdowns, animations, and more.
Loop Types: Explained
1. for Loop: The Classic Binge-Watcher
Use this loop when you know exactly how many times you want to iterate (like watching 12 episodes of a series).
javascriptCopy codeconst animeCharacters = ['Goku', 'Naruto', 'Luffy', 'Eren', 'Tanjiro'];
// Loop through the array using a for loop
for (let i = 0; i < animeCharacters.length; i++) {
console.log(`${i + 1}. ${animeCharacters[i]}`);
}
Output:
markdownCopy code1. Goku
2. Naruto
3. Luffy
4. Eren
5. Tanjiro
How it works:
let i = 0
: Start at index 0 (first character).i < animeCharacters.length
: Keep looping as long asi
is less than the length of the array.i++
: Incrementi
after each iteration.
Pro Tip:
Use reverse loops to print the characters in reverse order:
javascriptCopy codefor (let i = animeCharacters.length - 1; i >= 0; i--) {
console.log(`${animeCharacters[i]}`);
}
2. while Loop: The Determined Dreamer
The while loop is like Naruto—he keeps running until his goal is achieved! Use this loop when the number of iterations is unknown but you have a condition to control it.
javascriptCopy codelet j = 0;
while (j < animeCharacters.length) {
console.log(`While Loop: ${animeCharacters[j]}`);
j++;
}
Output:
vbnetCopy codeWhile Loop: Goku
While Loop: Naruto
While Loop: Luffy
While Loop: Eren
While Loop: Tanjiro
How it works:
Initialize
j = 0
.Run the loop as long as the condition
j < animeCharacters.length
is true.Increment
j
inside the loop to avoid infinite loops.
Watch out!
If you forget to increment j
, you’ll create an infinite loop—a developer’s worst nightmare!
3. do-while Loop: The Brave First Step
The do-while loop is like Eren from Attack on Titan—it acts at least once, even if failure is inevitable. This loop will run at least one time, even if the condition is false from the start.
javascriptCopy codelet k = 0;
do {
console.log(`Do-while: ${animeCharacters[k]}`);
k++;
} while (k < animeCharacters.length);
Output:
vbnetCopy codeDo-while: Goku
Do-while: Naruto
Do-while: Luffy
Do-while: Eren
Do-while: Tanjiro
How it works:
The code inside the
do
block runs once before checking the condition.If the condition in the
while
is true, it continues looping.
Challenge: Loop Through Your Favorite Anime Characters
Now that you know how loops work, try this fun challenge:
Create an array of your favorite anime characters (like above).
Use all three loops to print them out.
Add some fun formatting, like:
Print them in reverse order using a loop.
Use an if condition inside a loop to highlight your top 1 favorite character!
javascriptCopy codeconst animeCharacters = ['Goku', 'Naruto', 'Luffy', 'Eren', 'Tanjiro'];
for (let i = 0; i < animeCharacters.length; i++) {
if (animeCharacters[i] === 'Naruto') {
console.log(`🔥 ${animeCharacters[i]} - My Favorite!`);
} else {
console.log(`${animeCharacters[i]}`);
}
}
Output:
Copy codeGoku
🔥 Naruto - My Favorite!
Luffy
Eren
Tanjiro
Bonus: Loop Until You Drop!
Nested Loops: Loop within a loop—like listing all possible fights between characters.
Break and Continue:
Use
break
to stop the loop when a condition is met.Use
continue
to skip a specific iteration.
javascriptCopy codefor (let i = 0; i < animeCharacters.length; i++) {
if (animeCharacters[i] === 'Eren') break; // Stop at Eren
console.log(animeCharacters[i]);
}
Conclusion
With loops, you can automate actions and handle repetitive tasks efficiently. They’re the backbone of programs that need to process large datasets—whether it's fetching anime titles, processing user input, or animating elements on a webpage. Master loops today, and you’ll unlock limitless power in JavaScript!
Let’s see if you can create a loop-based program with your favorite anime characters! Ready to take on the challenge? 🎌
Subscribe to my newsletter
Read articles from Risharth pardeshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
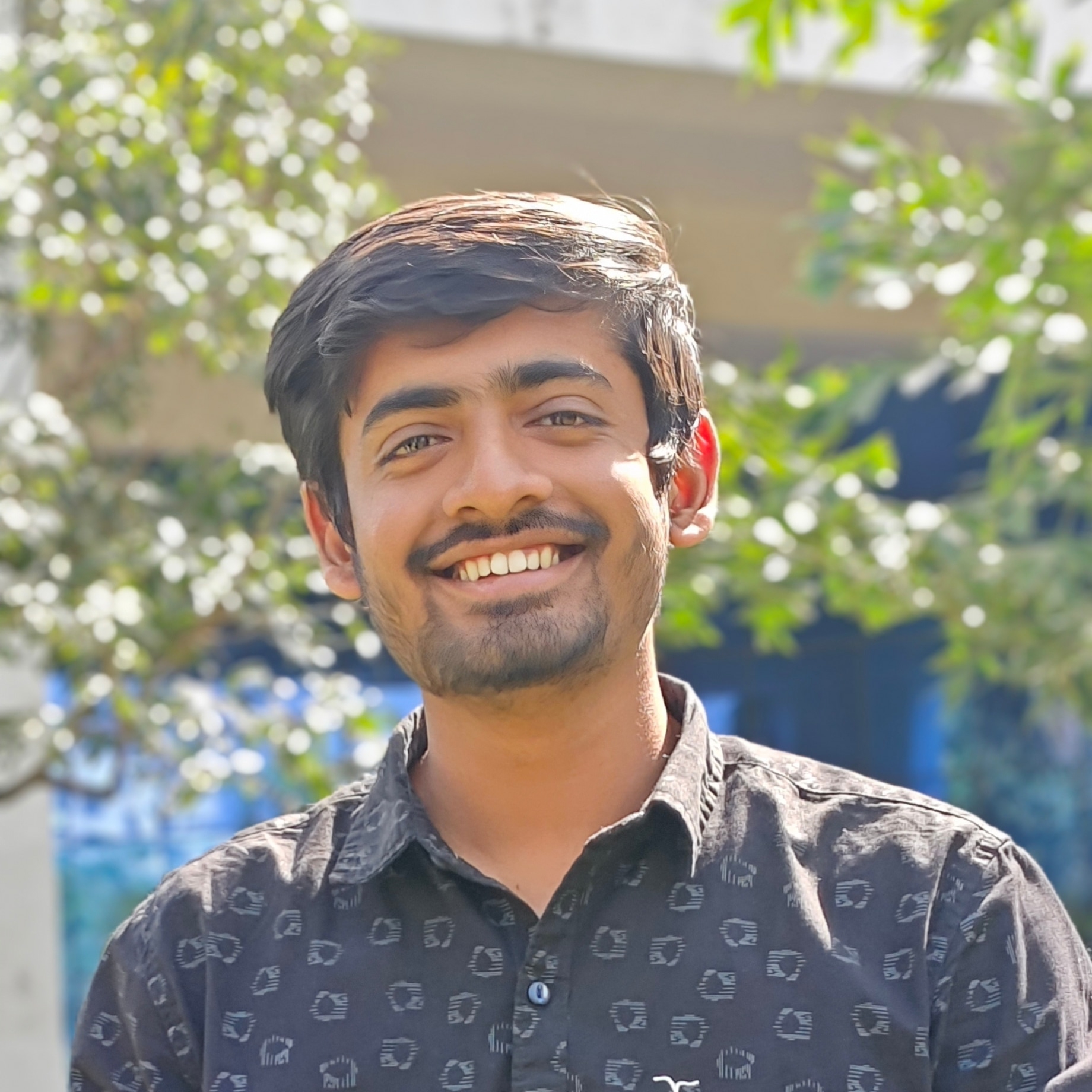
Risharth pardeshi
Risharth pardeshi
I hope this message finds you well. I am Risharth Pardeshi I am passionate about Computer Science and Engineering, and I have a strong foundation in this field. I graduated with a Bachelor of Engineering from the Laxmi Narayan College of Technology in Indore, India, in July 2024, with a CGPA of 7.98. Here is a brief overview of my technical strengths: Languages: C++, HTML, CSS, JavaScript, SQL Frameworks: React.js, Next.js, Tailwind CSS, Node.js Databases/OS: MySQL, MongoDB, Firebase, Linux Technical Skills: Data Structures and Algorithms, Git, DBMS, OS, Object-Oriented Programming, Computer Networking, Vercel, Postman