Star Rating feature in React
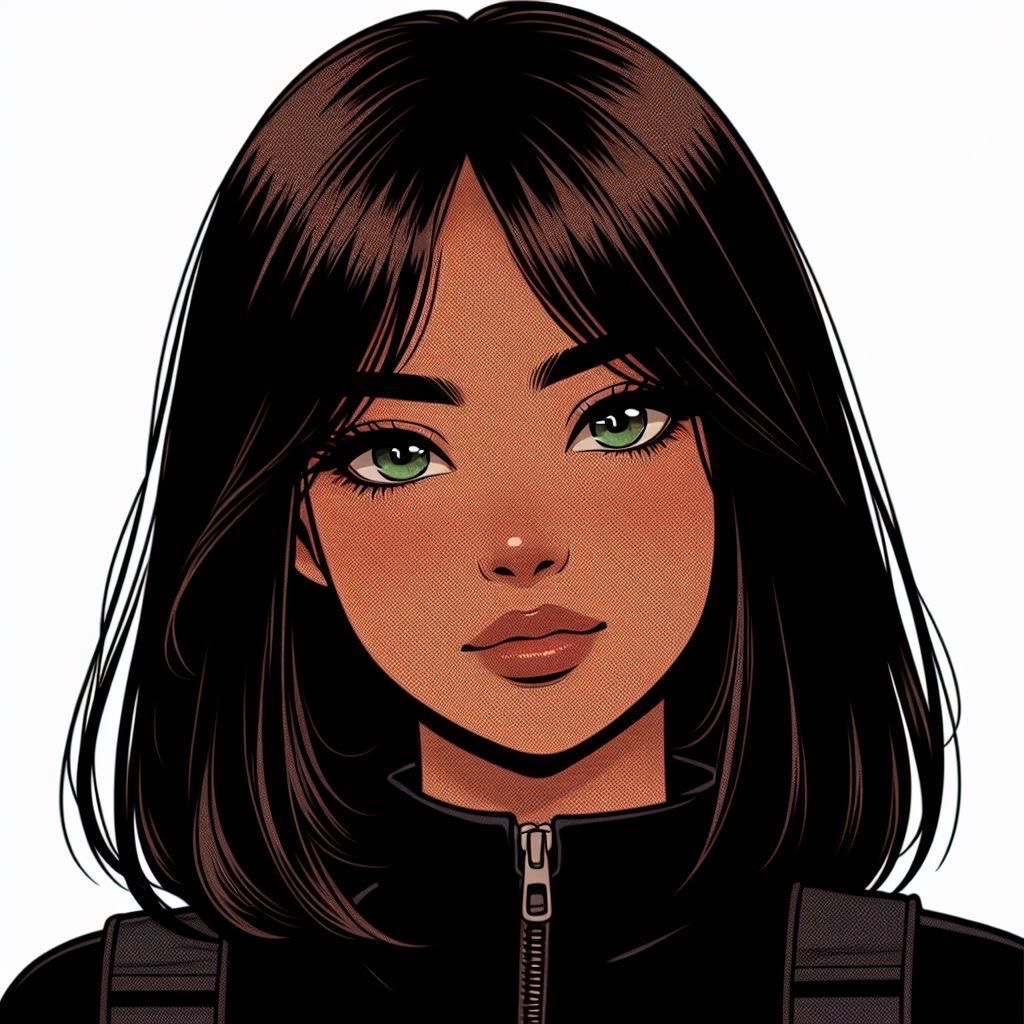
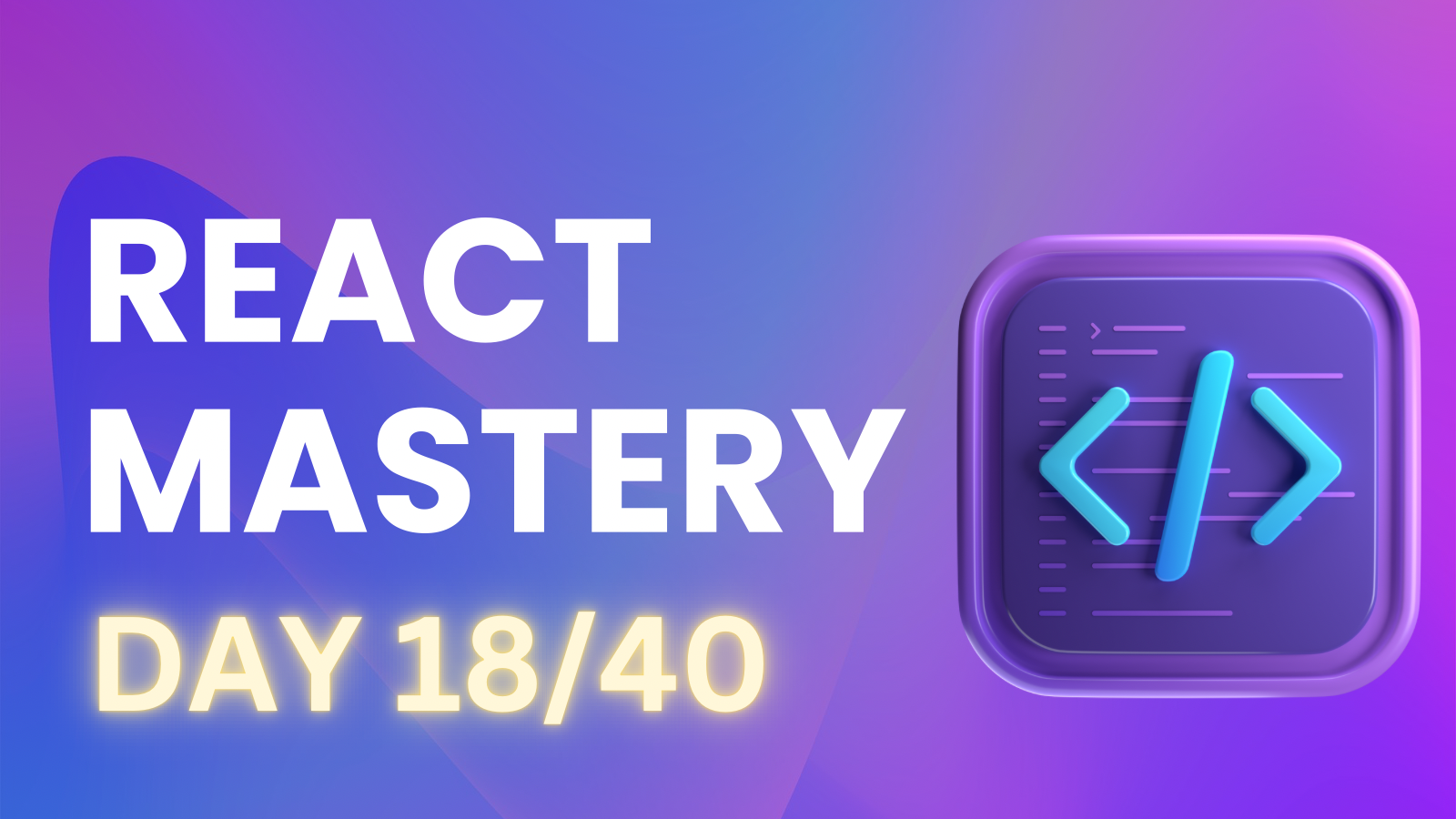
Today, I worked on a fun project where I created a star rating component using React. It’s a simple but interactive way to let users rate something with stars, and I learned a lot in the process. Here’s how I built it!
Setting Up the Project
I started by creating a new React app. After that, I made a new component called StarRating
in the components
folder. I also included the react-icons
library to use star icons, specifically the FaStar
icon from the Font Awesome collection.
The StarRating Component
Here’s the code for the StarRating
component:
import { FaStar } from 'react-icons/fa';
import '../App.css';
import React, { useState } from 'react';
export default function StarRating({ starNumbers = 5 }) {
const [rating, setRating] = useState(0);
const [hover, setHover] = useState(0);
function handleOnClick(getCurrentIndex) {
setRating(getCurrentIndex);
}
function handleOnHover(getCurrentIndex) {
setHover(getCurrentIndex);
}
function handleOnLeave() {
setHover(rating);
}
return (
<div className='stars'>
{
[...Array(starNumbers)].map((_, index) => {
index += 1;
return (
<FaStar
className={index <= (hover || rating) ? 'color' : 'grey'}
key={index}
size={50}
onClick={() => handleOnClick(index)}
onMouseMove={() => handleOnHover(index)}
onMouseLeave={handleOnLeave}
/>
);
})
}
</div>
);
}
How It Works
State Management: I used the
useState
hook to manage two pieces of state:rating
andhover
.rating
holds the currently selected star rating.hover
tracks which star is currently being hovered over.
Event Handlers:
handleOnClick
: This function updates therating
state when a star is clicked.handleOnHover
: This function updates thehover
state when a star is hovered over.handleOnLeave
: This resets thehover
state to the current rating when the mouse leaves the stars.
Rendering Stars: I created an array of stars based on the
starNumbers
prop (defaulting to 5). For each star, I checked if its index was less than or equal to thehover
orrating
to determine its color (colored or grey).
Integrating the Component
Next, I integrated the StarRating
component into the main App
component:
import StarRating from './components/Star';
import './App.css';
function App() {
return (
<>
<StarRating />
</>
);
}
export default App;
Conclusion
And that’s it! I now have a fully functional star rating component that users can interact with. I really enjoyed this small project it was really fun. I'm excited to see how I can implement in the future!
Subscribe to my newsletter
Read articles from Aaks directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
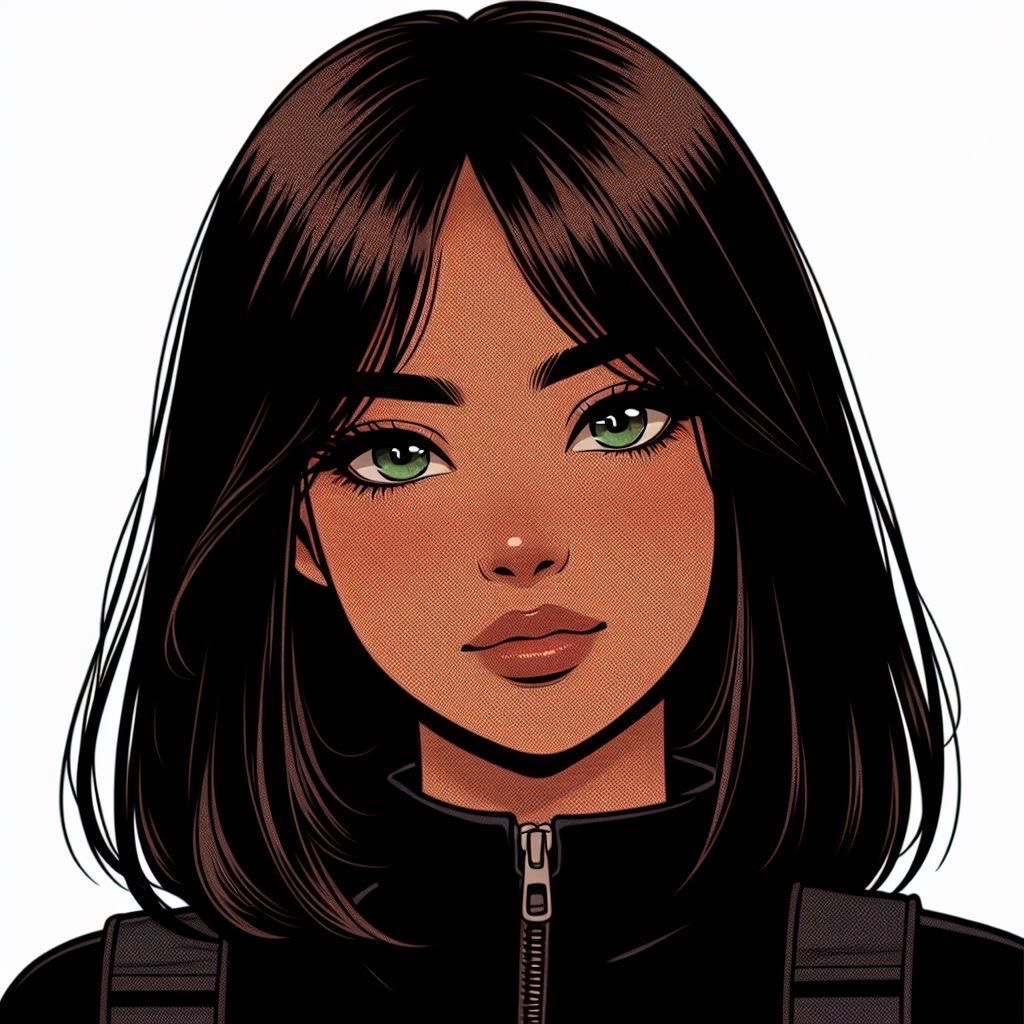