CORS and How to Resolve It
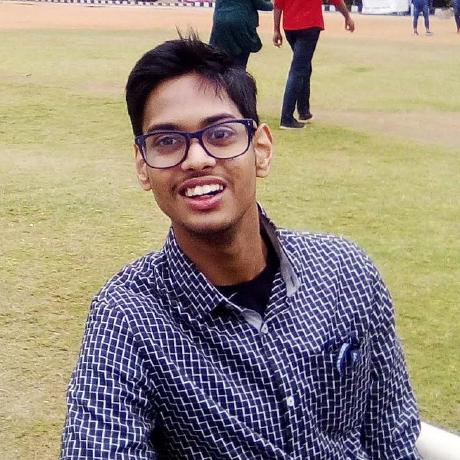
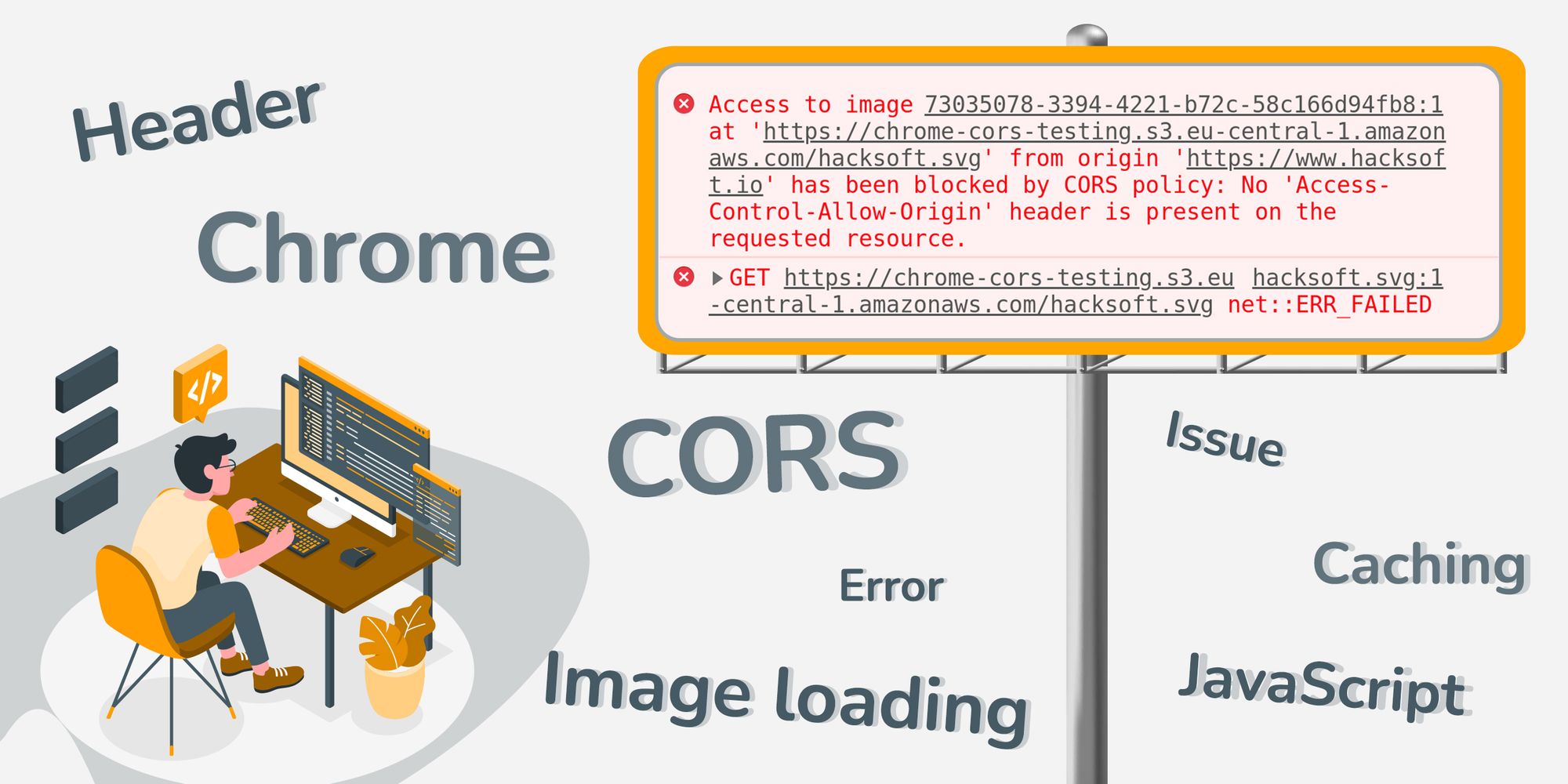
Cross-Origin Resource Sharing (CORS) is a critical concept in web development, especially when building applications that involve interaction between the front-end and back-end. If you’re working with the MERN stack (MongoDB, Express, React, Node.js), you might have encountered CORS-related issues. This guide will dive into what CORS is, why it’s important, common problems in a MERN stack application, and how to resolve them.
What is CORS?
CORS (Cross-Origin Resource Sharing) is a security feature implemented in web browsers to restrict web applications from making requests to a domain different from the one that served the web page. It’s a way for servers to specify who can access their resources and how the resources can be accessed.
For example, if your React front-end is hosted at http://localhost:3000
and your Express server is running at http://localhost:4000
, the browser will block requests from the React app to the Express server because they are on different origins. This behavior is known as the same-origin policy.
Why Does CORS Matter?
Security: CORS helps prevent malicious sites from accessing restricted resources hosted on a different domain.
Resource Sharing: In many applications, particularly those with separate front-end and back-end architectures like in the MERN stack, CORS configuration is essential for allowing the front-end to communicate with the back-end.
Without proper CORS configuration, your front-end might encounter errors like:
Access to fetch at 'http://localhost:4000/mind' from origin 'http://localhost:3000' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource.
How CORS Works
When a client (like your React app) sends a request to a server on a different origin, the browser first sends a preflight request (an OPTIONS
request) to the server. The server responds with headers that tell the browser whether the real request is allowed or not.
Key headers used in CORS include:
Access-Control-Allow-Origin: Specifies which origins are allowed to access the resource.
Access-Control-Allow-Methods: Specifies the HTTP methods (
GET
,POST
, etc.) that are allowed.Access-Control-Allow-Headers: Lists the headers that clients can use when making a request.
Resolving CORS Issues in the MERN Stack
Step 1: Understanding the Problem
Let’s assume the following setup:
React Front-end:
http://localhost:3000
Express Back-end:
http://localhost:4000
When your front-end tries to make a request to the back-end, you might see a CORS error because the origins differ.
Step 2: Using the cors
Middleware in Express
The most straightforward way to resolve CORS issues in an Express application is to use the cors
middleware.
Installation:
npm install cors
Configuration: In your Express server file (e.g., index.js
or server.js
):
const express = require('express');
const cors = require('cors');
const app = express();
// Basic CORS setup
app.use(cors());
// More complex CORS setup (if needed)
app.use(cors({
origin: 'http://localhost:3000', // Replace with your front-end URL
methods: ['GET', 'POST', 'PUT', 'DELETE'],
allowedHeaders: ['Content-Type', 'Authorization']
}));
app.get('/mind', (req, res) => {
res.json({ message: 'Hello from the server!' });
});
const PORT = 4000;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
With this setup:
The
origin
field allows only the specified front-end domain to access the server.The
methods
field specifies which HTTP methods are allowed.The
allowedHeaders
field lists the headers that are acceptable.
Step 3: Handle CORS Errors on the Client-Side
While the server-side setup often resolves the issue, you may also need to configure your client-side requests properly. For instance, if you are using the fetch
API or axios
in React:
Using fetch
:
fetch('http://localhost:4000/mind', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Using axios
:
import axios from 'axios';
axios.get('http://localhost:4000/mind')
.then(response => console.log(response.data))
.catch(error => console.error('Error:', error));
In most cases, setting up CORS on the server with the cors
middleware should be sufficient, and you don’t need to adjust much on the client side.
Step 4: Debugging Common CORS Issues
Origin Not Allowed:
Ensure that the
origin
specified in the server'scors
configuration matches the front-end's URL.You can allow multiple origins using:
app.use(cors({ origin: ['http://localhost:3000', 'http://example.com'] }));
No
Access-Control-Allow-Origin
Header:- This often means the
cors
middleware is not applied correctly. Ensure it’s added before defining your routes.
- This often means the
Preflight Request Failing:
- Make sure that the
OPTIONS
method is allowed on the server. Express handles this automatically if you use thecors
middleware, but custom middleware might interfere.
- Make sure that the
Step 5: Enabling CORS in Production
If you are deploying your MERN stack application, you might want to allow multiple domains or use environment variables for CORS configuration:
const allowedOrigins = ['http://localhost:3000', 'https://your-production-domain.com'];
app.use(cors({
origin: (origin, callback) => {
if (allowedOrigins.includes(origin) || !origin) {
callback(null, true);
} else {
callback(new Error('Not allowed by CORS'));
}
}
}));
This configuration will allow requests from specific origins while blocking others.
Conclusion
CORS is a fundamental security mechanism that protects users and servers from malicious interactions. When developing a MERN stack application, understanding and configuring CORS is crucial for seamless communication between the client and server. Using the cors
middleware in Express, setting proper headers, and debugging common issues can help ensure that your application runs smoothly without CORS-related interruptions.
By following this guide, you should be able to resolve CORS issues and have a better understanding of how to manage cross-origin requests in your MERN stack projects. Happy coding!
Subscribe to my newsletter
Read articles from Anish Agrawal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
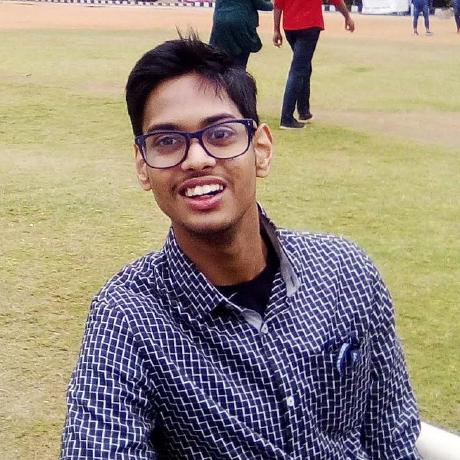