JWT (JSON Web Token )

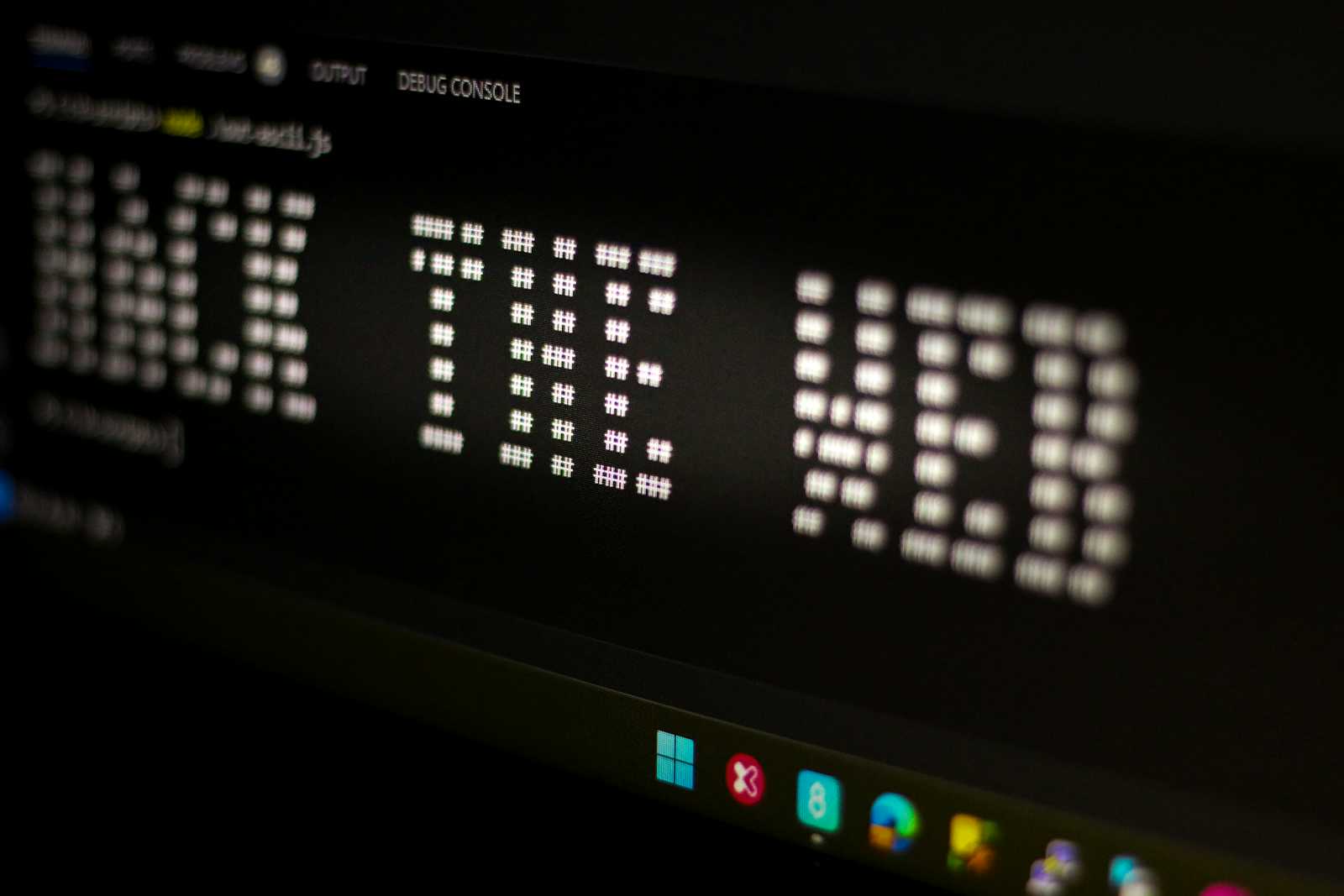
What is JWT and Why it is using?
JWT stands for the JSON Web Token. It’s a compact way to send data between two parties,usually between our browser and a server, in a safe and verifiable way.
JWT is mostly used to make sure you stay logged in as you browse different pages of a website. instead of storing your session data on the server sends you a JWT, and your browser sends it back with every request. This saves memory on the server and makes the process faster.
Works flow of JWT
You logged in a website
Then server checks your details, then creates a JWT and send it to you.
Your browser stores this JWT(usually in localStorage or cookies)
Every time you visit a new page or make a request, the browser sends the JWT along.
The server looks at the JWT to confirm who you are, without needing to remember your session
Structure of JWT
Header.Payload.Signature
Header:Information about how the token is made (e.g., what algorithm is used).
Payload: The actual data, like your user ID.
Signature: The signature in a JWT ensures that the token hasn’t been changed by verifying it with a secret key.
Example:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VySWQiOiIxMjM0NTYifQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
JWT in Authentication
When you log in, the server gives you a JWT. Your browser keeps it, and when you go to another page, your browser sends the JWT to prove you are logged in. This is how websites allow you to browse different sections without asking you to log in again and again.
How is JWT Different from Sessions?
With traditional sessions:
The server stores all user data.
Your browser sends a session ID, and the server uses it to find your session.
With JWT:
The data is inside the token itself, and the server doesn't need to remember anything.
This makes things faster and easier to scale.
Is JWT Safe?
JWT is safe as long as it's used correctly. Some key security tips are:
Never store sensitive data in the JWT payload (since anyone can decode it).
Always use HTTPS to keep the token safe from hackers.
Set an expiration time so tokens don’t last forever.
How to Use JWT (Simple Code Example)
JWT with Node.js and Express
const express = require('express');
const jwt = require('jsonwebtoken');
const app = express();
const SECRET = 'mysecretkey';
app.post('/login', (req, res) => {
const user = { id: 1, name: 'John' }; // pretend user
const token = jwt.sign(user, SECRET, { expiresIn: '1h' });
res.json({ token });
});
app.get('/protected', (req, res) => {
const token = req.headers['authorization'];
if (!token) return res.sendStatus(403);
jwt.verify(token.split(' ')[1], SECRET, (err, user) => {
if (err) return res.sendStatus(403);
res.json({ message: 'Welcome!', user });
});
});
app.listen(3000, () => console.log('Server running on port 3000'));
Conclusion
JWT is an easy and effective way to handle authentication, especially for modern web apps. It keeps the process quick, scalable, and secure when used correctly.
Subscribe to my newsletter
Read articles from Sonu Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
