P1 - Exploring the Basics of GraphQL
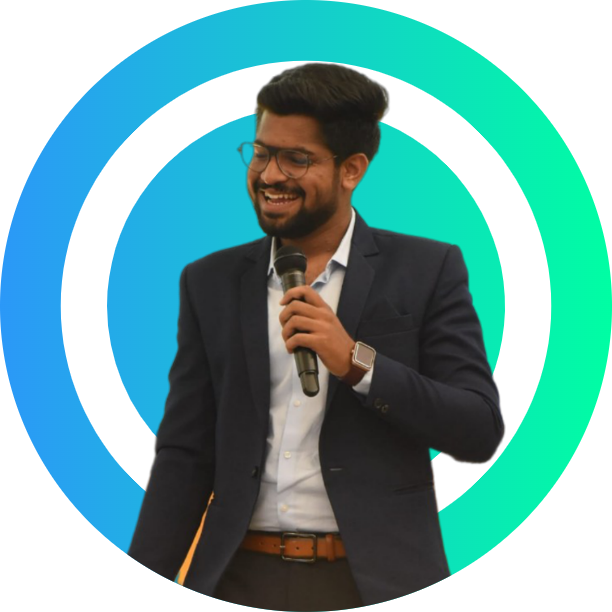
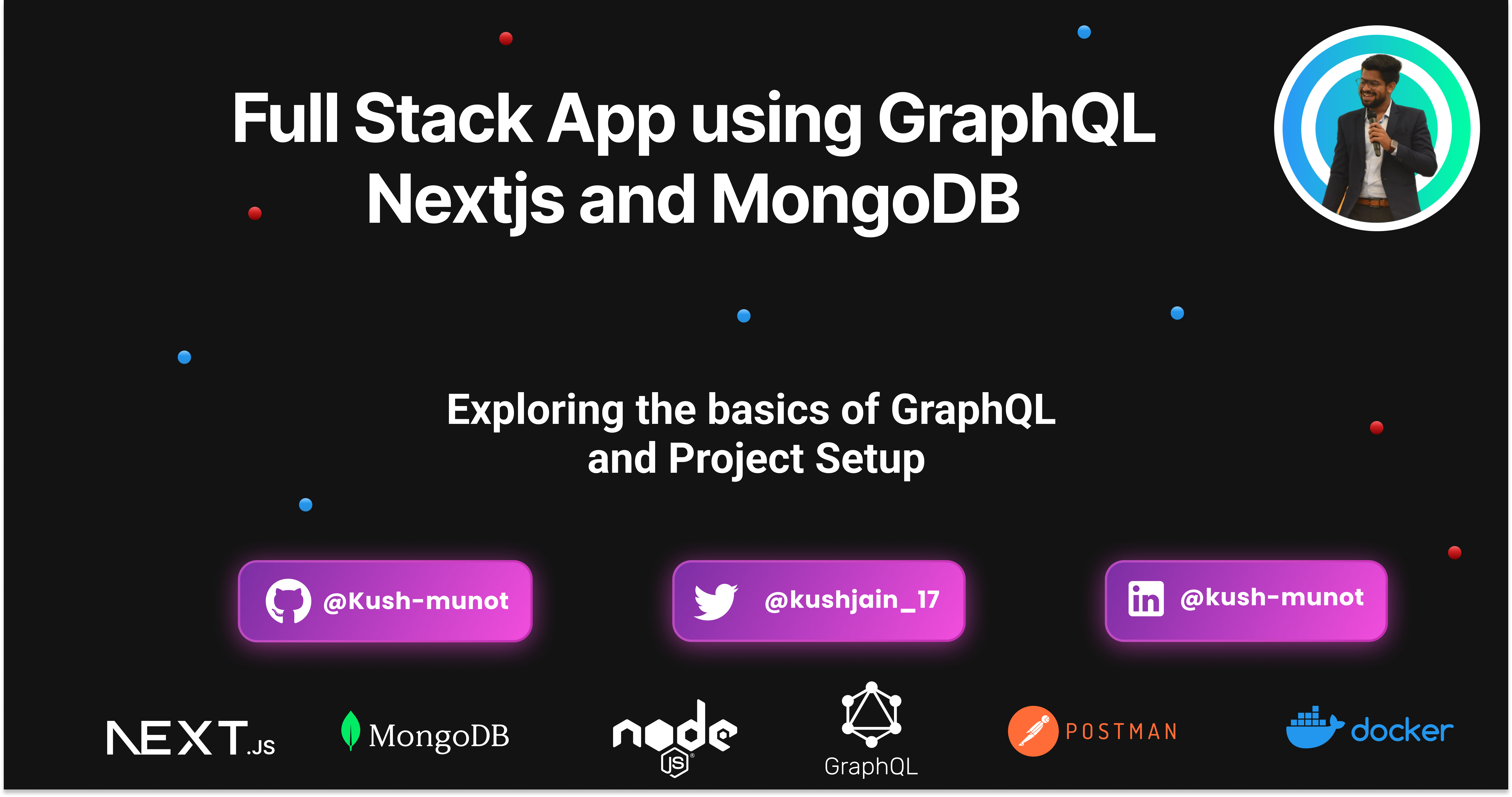
Understanding GraphQL
GraphQL is a query language that was developed specifically for APIs. It prioritizes giving clients exactly the data they request and no more. It is designed to make APIs fast, flexible, and developer-friendly. As an alternative to REST, GraphQL allows front-end developers to query multiple databases, microservices, and APIs with a single GraphQL endpoint.
REST vs GraphQL
Feature | GraphQL | REST |
Data Fetching | Clients request only the specific data they need in one call | Fixed endpoints; may require multiple calls for related data |
Endpoints | Single endpoint for all queries (/graphql ) | Multiple endpoints for each resource (e.g., /users , /posts ) |
Data Relationships | Can query related data in one request (nested queries) | Requires multiple requests for related data |
Versioning | No versioning needed; schema evolves by adding fields | Typically requires versioning (/v1 , /v2 ) when API changes |
Performance | Reduces over-fetching and under-fetching | May lead to over-fetching or under-fetching |
Flexibility | Highly flexible; clients define the structure of the response | Less flexible; server defines the response structure |
Error Handling | Returns structured data and errors fields | Relies on HTTP status codes for errors |
Tooling | Strong tools for introspection and real-time query building | Traditional tools like Postman, but no introspection |
Real-time Data | Supports real-time data through subscriptions | Typically requires polling or separate WebSockets |
Problems GraphQL Solves
GraphQL addresses common API challenges by offering:
Over-fetching: Clients can request only the specific data they need, reducing wasted bandwidth.
Under-fetching: A single GraphQL request can gather all required data, avoiding multiple API calls.
Versioning: New fields and types can be added without creating new endpoints, simplifying version management.
Flexibility: Clients can define the exact data structure they need, improving adaptability.
Basic Project Setup
It’s enough theory let’s get coding !! 🧑🏻💻
So, we will be using trending Technologies like Next.js, MongoDB with Docker and GraphQL to make an Expense Tracker Application that will help you learn Database Queries, GraphQL APIs, Using Postman to test backend Microservices, and Next.js.
Setting Up the Next.js Project
Run the below command to initialize a Next.js Project.
npx create-next-app@latest graphql-expense-tracker
Then the project setup guide will ask you the following questions please select the following configuration
√ Would you like to use TypeScript? ... Yes
√ Would you like to use ESLint? ... Yes
√ Would you like to use Tailwind CSS? ... No
√ Would you like to use `src/` directory? ... Yes
√ Would you like to use App Router? (recommended) ... Yes
√ Would you like to customize the default import alias (@/*)?... No
This will install the required dependencies and at last you will see a Success Statement on fulfillment of the process.
Installing required libraries
cd graphql-expense-tracker
npm install apollo-server-micro mongoose @apollo/client graphql
npm install @mui/material @emotion/react @emotion/styled @mui/icons-material
apollo-server-micro: A lightweight GraphQL server library for building GraphQL APIs in Next.js projects using the Micro framework.
mongoose: A MongoDB object modeling library used to define schemas, models, and interact with MongoDB databases in a structured way.
@apollo/client: A comprehensive state management library used in React apps to connect the frontend to the GraphQL API, handle queries, mutations, and caching.
graphql: A query language used to define, query, and interact with the GraphQL API for handling CRUD operations.
@mui/material: A popular React component library used to build responsive, modern, and visually appealing UI components with Material Design.
@emotion/react & @emotion/styled: Libraries used for writing CSS-in-JS styles, allowing component-level styling in a performant and flexible way.
@mui/icons-material: Provides Material UI icons to enhance the UI with easily customizable vector icons.
This ensures all the required libraries are there in the project setup and we can start building !!
Setting up the directory structure
Initially the directory structure should look like this
└── 📁graphql-expense-tracker
└──📁src
└── 📁app
└── 📁fonts
└── GeistMonoVF.woff
└── GeistVF.woff
└── favicon.ico
└── globals.css
└── layout.tsx
└── page.module.css
└── page.tsx
└── .eslintrc.json
└── .gitignore
└── next-env.d.ts
└── next.config.mjs
└── package-lock.json
└── package.json
└── README.md
└── tsconfig.json
We're going to organize our project in a way that keeps things clear and easy to find. Here's how:
Create the
pages
folder:- This folder will hold all the pages of our application.
Inside
pages
, create theapi
folder:- This folder will contain all the backend logic, including API calls and data fetching.
Inside
api
, create thegraphql
folder:- This folder will be specifically for GraphQL, a powerful way to fetch and manipulate data.
Inside
graphql
, create two files:index.ts
: This file will define the queries and mutations that our application can perform. Think of these as the instructions for fetching and modifying data.models
folder: This folder will hold the definitions of the data we're working with.schema
folder: This will hold all the typeDefs, resolvers and types needed for querying the database.
Inside
models
, create theExpense.ts
file:- This file will define the structure of an expense, specifying its properties like amount, category, and date.
Inside
schema
, create three files:resolvers.ts
: The resolvers file will define the resolver functions for queries and mutations, using the types defined intypes.ts
.typeDefs.ts
: This file will define the GraphQL schema. You can usegql
from Apollo Server to create the schema.types.ts
: This file will store all the TypeScript types and interfaces related to expenses.
Now, let's turn to the frontend:
- Inside
pages
, create two files:
_app.ts
: This file is a global component that wraps all other pages in our application. It's a great place to set up global styles, themes, or data fetching.index.ts
: This file will be the main page of our application. It's where we'll display the list of expenses, a form to add new expenses, and other relevant components.
- Make sure you empty all the existing files in your app directory.
Finally the Directory structure will look like this
└── 📁graphql-expense-tracker
└── 📁public
└── file.svg
└── globe.svg
└── next.svg
└── vercel.svg
└── window.svg
└── 📁src
└── 📁app
└── 📁pages
└── 📁api
└── 📁graphql
└── 📁models
└── Expense.ts
└── 📁schema
└── typeDefs.ts
└── resolvers.ts
└── types.ts
└── index.ts
└── _app.tsx
└── index.tsx
└── .env.local
└── .eslintrc.json
└── .gitignore
└── next-env.d.ts
└── next.config.ts
└── package-lock.json
└── package.json
└── README.md
└── tsconfig.json
Up Next ⏭️
We will be understanding the Queries and Mutations that will be used in our codebase and also get our hands dirty with some code to connect MongoDB and do some CRUD operation on it !!
Subscribe to my newsletter
Read articles from Kush Munot directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
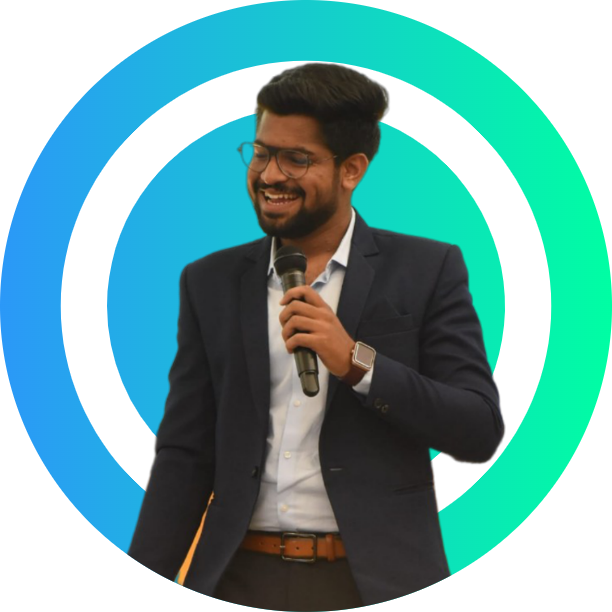