Top 5 JavaScript Design Patterns You Need to Know

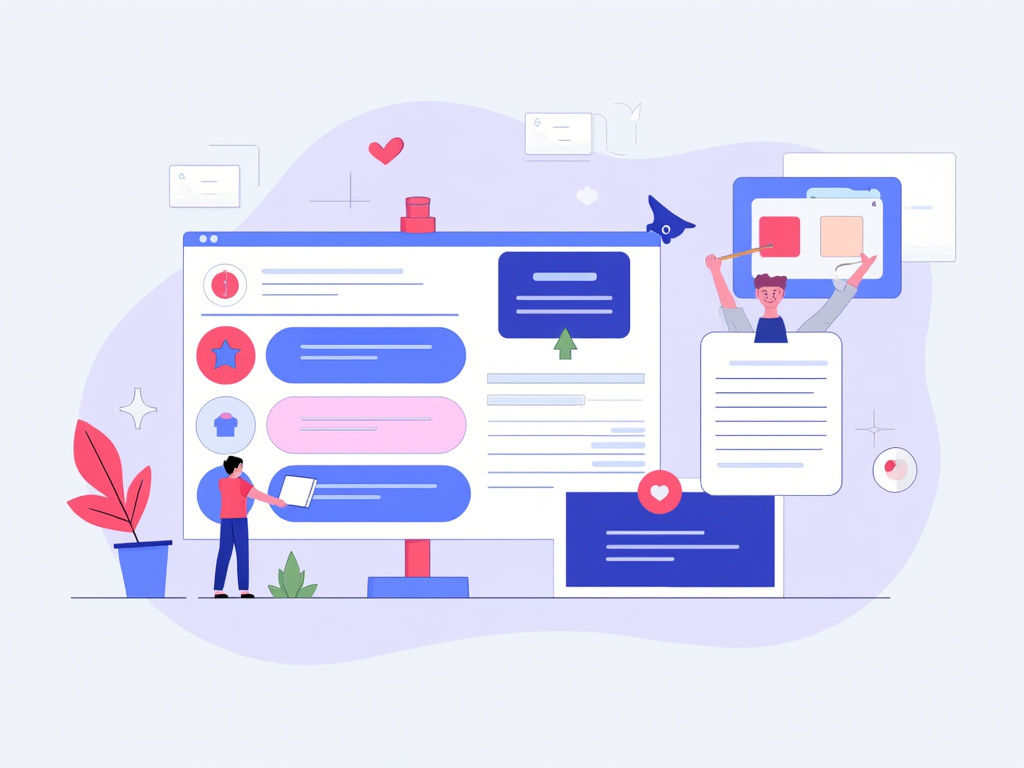
JavaScript is one of the most flexible and widely-used programming languages in the world, but with that flexibility comes complexity. As JavaScript projects grow, maintaining clean, readable, and scalable code becomes crucial. That’s where design patterns come in. Design patterns are tried-and-true solutions to common programming problems that make your code more efficient and easier to manage.
In this article, we’ll explore the top 5 JavaScript design patterns that every developer should master. Whether you're building a large-scale application or working on smaller projects, these patterns will help you write better code.
1. Module Pattern
The Module Pattern is one of the most commonly used design patterns in JavaScript. It allows you to organize your code in a way that encapsulates data and functionality, reducing the risk of polluting the global namespace and creating reusable components.
Why it’s useful:
By wrapping code inside a module, you can keep variables private, expose only what’s necessary, and create a cleaner, more organized project structure.
Example:
const CounterModule = (function() {
let counter = 0;
function increment() {
return ++counter;
}
function getCounter() {
return counter;
}
return {
increment,
getCounter,
};
})();
console.log(CounterModule.increment()); // 1
console.log(CounterModule.getCounter()); // 1
2. Factory Pattern
The Factory Pattern is a creational design pattern used when you want to create objects but let subclasses decide which class to instantiate. It’s particularly useful in scenarios where the creation process is complex and needs to be abstracted from the main codebase.
Why it’s useful:
The Factory Pattern is perfect for codebases that need flexibility when creating objects, especially when dealing with complex setups or configurations.
Example:
class Car {
constructor(model, price) {
this.model = model;
this.price = price;
}
}
class CarFactory {
createCar(model, price) {
return new Car(model, price);
}
}
const factory = new CarFactory();
const tesla = factory.createCar("Tesla Model 3", 35000);
console.log(tesla); // Car { model: 'Tesla Model 3', price: 35000 }
3. Singleton Pattern
The Singleton Pattern ensures that a class has only one instance and provides a global point of access to it. This is particularly useful for scenarios where you want to ensure that a particular resource (e.g., a database connection or a logger) is used consistently throughout your application.
Why it’s useful:
Singletons are great for managing state across an entire application and ensuring that certain classes are not duplicated.
Example:
class Singleton {
constructor() {
if (Singleton.instance) {
return Singleton.instance;
}
this.state = {};
Singleton.instance = this;
}
getState() {
return this.state;
}
setState(key, value) {
this.state[key] = value;
}
}
const singleton1 = new Singleton();
const singleton2 = new Singleton();
singleton1.setState("name", "BaseStack");
console.log(singleton2.getState()); // { name: 'BaseStack' }
console.log(singleton1 === singleton2); // true
4. Observer Pattern
The Observer Pattern is a behavioral design pattern where an object (called the subject) maintains a list of observers who are notified whenever the subject's state changes. This pattern is commonly used in event-driven architectures.
Why it’s useful:
It’s perfect for implementing features like event listeners, reactive programming, or whenever you need one part of your application to automatically react to changes in another.
Example:
class Subject {
constructor() {
this.observers = [];
}
addObserver(observer) {
this.observers.push(observer);
}
notify(data) {
this.observers.forEach(observer => observer.update(data));
}
}
class Observer {
update(data) {
console.log(`Observer notified with data: ${data}`);
}
}
const subject = new Subject();
const observer1 = new Observer();
const observer2 = new Observer();
subject.addObserver(observer1);
subject.addObserver(observer2);
subject.notify("New event!"); // Observer notified with data: New event!
5. Decorator Pattern
The Decorator Pattern is a structural design pattern that allows you to add new functionality to an existing object without modifying its structure. This is done by wrapping the object with a decorator class that adds the desired features.
Why it’s useful:
It’s ideal for scenarios where you want to add behavior to objects dynamically, such as adding logging, data validation, or formatting.
Example:
class Coffee {
cost() {
return 5;
}
}
class MilkDecorator {
constructor(coffee) {
this.coffee = coffee;
}
cost() {
return this.coffee.cost() + 2;
}
}
const coffee = new Coffee();
const milkCoffee = new MilkDecorator(coffee);
console.log(milkCoffee.cost()); // 7
How to Choose the Right Design Pattern
Selecting the right design pattern depends on the problem you're trying to solve. Here's a quick guide:
Module Pattern: Use when you need to encapsulate code and avoid polluting the global namespace.
Factory Pattern: Ideal for flexible object creation.
Singleton Pattern: Perfect when you need a single instance of a class shared across your application.
Observer Pattern: Great for event-driven applications or state monitoring.
Decorator Pattern: Best when you want to add functionality to an object without changing its core structure.
Conclusion: Improve Your Code with Design Patterns
Design patterns are the backbone of clean, scalable, and maintainable JavaScript applications. By mastering these five essential patterns, you'll be better equipped to tackle any challenge in 2024, whether you’re developing a small side project or working on a large-scale application.
Explore Basestack Platform
Ready to elevate your development experience even further? Check out Basestack ↗, our open-source stack designed specifically for developers and startups. BaseStack offers a comprehensive suite of tools, including Feature Flags, with more exciting tools like Feedback and Forms on the horizon.
Discover BaseStack:
Join our community and take advantage of the tools that can empower you to build exceptional products
Subscribe to my newsletter
Read articles from Vitor Amaral directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vitor Amaral
Vitor Amaral
Hello! I am a skilled and motivated Full Stack Developer with a background in Management and Information Programming from Portugal 🇵🇹. Throughout my career, I have developed and managed a range of projects from start to finish, demonstrating my adaptability and strong communication skills. I have a diverse set of technical skills, including expertise in Serverless Computing, Cloud Management, and various forms of web, desktop, and mobile development such as React, Styled-Components, Svelte, Electron, WinForms, and React Native. In addition to these technical skills, I also have a strong understanding of UI/UX design principles and excellent problem-solving abilities.