Advanced Concurrency Management Strategies
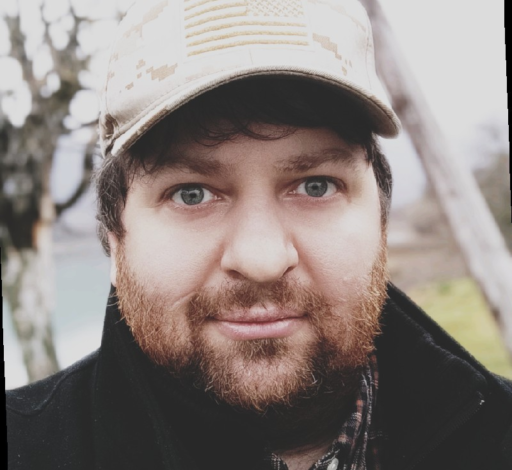
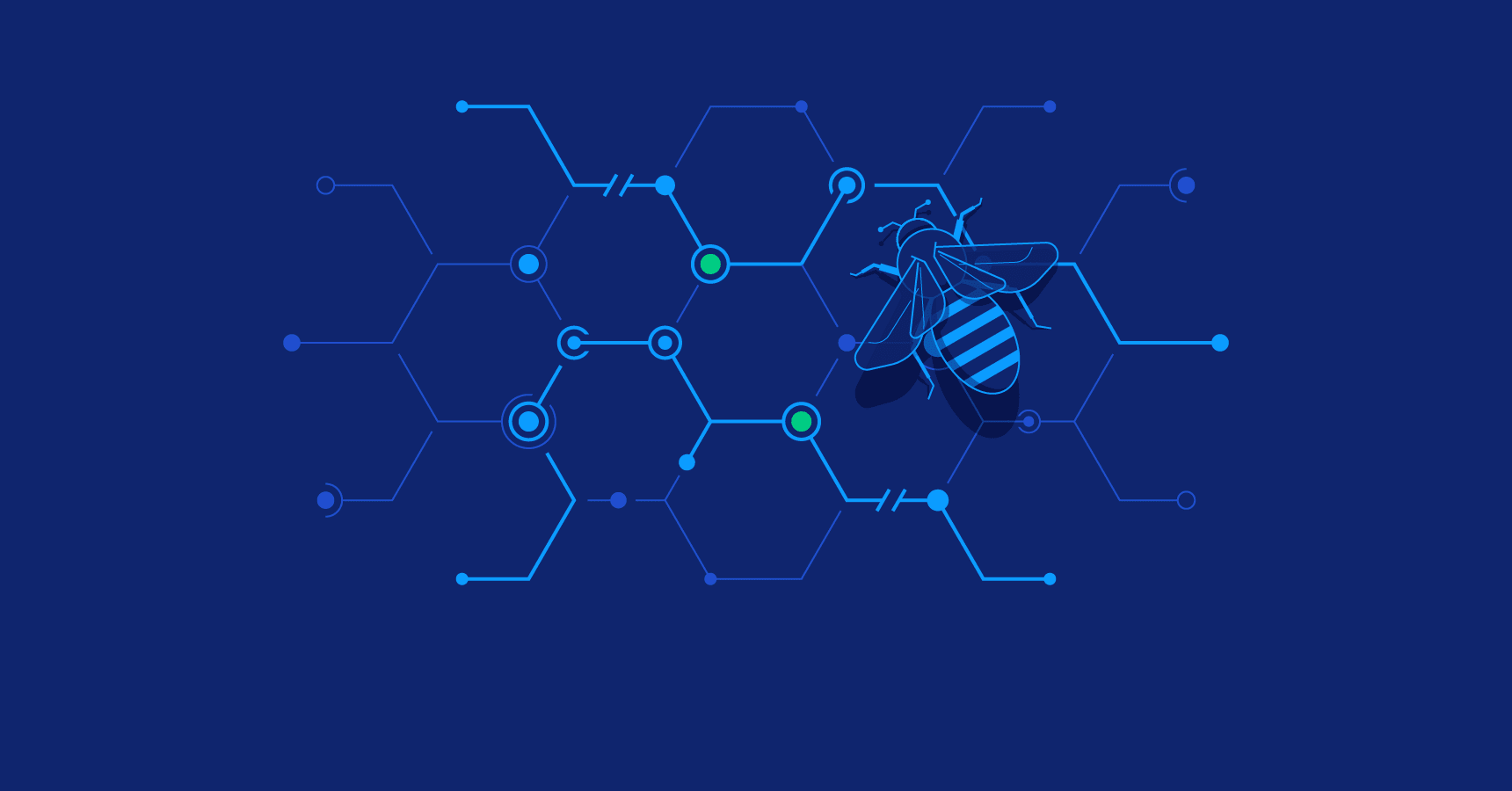
Introduction
In the realm of modern software engineering, mastering concurrency management is not just a technical necessity—it's a critical business imperative. This comprehensive guide delves into advanced concurrency strategies that can significantly impact system reliability, performance, and scalability. The knowledge shared here represents years of industry experience and cutting-edge research, potentially saving organizations millions in development costs and operational inefficiencies.
The Anatomy of Concurrency Challenges
Concurrency issues arise when multiple processes or users simultaneously attempt to access and modify shared resources. These challenges are often subtle, difficult to reproduce, and can lead to catastrophic system failures if not properly managed.
Real-world Scenario: High-Frequency Trading Platform
Consider a high-frequency trading platform processing millions of transactions per second:
Transaction A: Buy 1000 shares of Stock X
Transaction B: Sell 500 shares of Stock X
Initial State: 10,000 shares available
Without proper concurrency control:
Transaction A reads available shares: 10,000
Transaction B reads available shares: 10,000
Transaction A updates: 9,000 shares remaining
Transaction B updates: 9,500 shares remaining
Final State: 9,500 shares (Incorrect!)
The correct final state should be 8,500 shares. This discrepancy could result in millions of dollars in losses and regulatory issues.
Advanced Concurrency Management Strategies
1. Optimistic Concurrency Control (OCC)
OCC operates on the assumption that conflicts are rare but verifies this assumption before committing changes.
Implementation Strategy in C#:
public class OptimisticConcurrencyService
{
private readonly DbContext _context;
public OptimisticConcurrencyService(DbContext context)
{
_context = context;
}
public async Task<bool> UpdateStockQuantityAsync(int stockId, int quantityChange)
{
using var transaction = await _context.Database.BeginTransactionAsync();
try
{
var stock = await _context.Stocks
.FirstOrDefaultAsync(s => s.Id == stockId);
if (stock == null)
throw new NotFoundException("Stock not found");
var originalVersion = stock.Version;
stock.Quantity += quantityChange;
stock.Version++;
_context.Entry(stock).Property(x => x.Version).OriginalValue = originalVersion;
var affected = await _context.SaveChangesAsync();
if (affected == 0)
{
await transaction.RollbackAsync();
return false; // Conflict detected
}
await transaction.CommitAsync();
return true;
}
catch
{
await transaction.RollbackAsync();
throw;
}
}
}
This implementation uses Entity Framework Core's built-in concurrency token (Version
property) to detect conflicts. If a conflict is detected, SaveChangesAsync()
returns 0, and we roll back the transaction.
Advantages:
High performance in low-contention scenarios
No long-term locking of resources
Disadvantages:
- This can lead to frequent retries in high-contention scenarios.
2. Pessimistic Concurrency Control (PCC)
PCC assumes conflicts are frequent and locks resources preemptively.
Implementation Strategy in C#:
public class PessimisticConcurrencyService
{
private readonly DbContext _context;
public PessimisticConcurrencyService(DbContext context)
{
_context = context;
}
public async Task<bool> UpdateStockQuantityAsync(int stockId, int quantityChange)
{
using var transaction = await _context.Database.BeginTransactionAsync(IsolationLevel.Serializable);
try
{
var stock = await _context.Stocks
.FromSqlRaw("SELECT * FROM Stocks WITH (UPDLOCK, ROWLOCK) WHERE Id = {0}", stockId)
.FirstOrDefaultAsync();
if (stock == null)
throw new NotFoundException("Stock not found");
stock.Quantity += quantityChange;
await _context.SaveChangesAsync();
await transaction.CommitAsync();
return true;
}
catch
{
await transaction.RollbackAsync();
throw;
}
}
}
This implementation uses SQL Server's UPDLOCK
and ROWLOCK
hints to lock the record. IsolationLevel.Serializable
Ensures maximum isolation.
Advantages:
Ensures data integrity in high-contention scenarios
Reduces the likelihood of conflicts
Disadvantages:
This can lead to deadlocks if not carefully managed
This may reduce system throughput due to increased waiting times
3. Multi-Version Concurrency Control (MVCC)
MVCC maintains multiple versions of data, allowing readers to work concurrently with writers.
Implementation Strategy:
While MVCC is often implemented at the database level (e.g., PostgreSQL), we can leverage this functionality in C# by selecting appropriate isolation levels:
public class MVCCService
{
private readonly DbContext _context;
public MVCCService(DbContext context)
{
_context = context;
}
public async Task<Stock> GetStockSnapshotAsync(int stockId, DateTime asOfDate)
{
using var transaction = await _context.Database.BeginTransactionAsync(IsolationLevel.Snapshot);
try
{
var stock = await _context.Stocks
.TemporalAsOf(asOfDate)
.FirstOrDefaultAsync(s => s.Id == stockId);
await transaction.CommitAsync();
return stock;
}
catch
{
await transaction.RollbackAsync();
throw;
}
}
}
This example uses SQL Server's temporal tables feature to simulate MVCC behavior.
Advantages:
High performance in read-heavy systems
Readers do not block writers
Disadvantages:
Increased storage requirements
Complex implementation and maintenance
Cutting-Edge Techniques
1. Software Transactional Memory (STM)
STM brings the concept of database transactions to in-memory operations, providing a high-level abstraction for managing concurrent access to shared state.
Conceptual Implementation in C#:
public class STM<T>
{
private T _value;
private readonly object _lock = new object();
public T Read()
{
lock (_lock)
{
return _value;
}
}
public bool TryCommit(Func<T, T> updateFunction)
{
lock (_lock)
{
var newValue = updateFunction(_value);
_value = newValue;
return true;
}
}
}
// Usage
var account = new STM<decimal>(1000m);
var success = account.TryCommit(balance => balance - 500m);
if (success)
{
Console.WriteLine("Transaction successful");
}
This simplified implementation demonstrates the core concept of STM. In practice, more sophisticated STM libraries would be used.
2. Actor Model
The Actor Model provides a higher level of abstraction for concurrent computation, where "actors" are the universal primitives of concurrent computation.
Implementation using Akka.NET:
public class BankAccount : ReceiveActor
{
public decimal Balance { get; private set; }
public BankAccount()
{
Receive<Deposit>(deposit => {
Balance += deposit.Amount;
Sender.Tell(new OperationResult(true, Balance));
});
Receive<Withdraw>(withdraw => {
if (Balance >= withdraw.Amount)
{
Balance -= withdraw.Amount;
Sender.Tell(new OperationResult(true, Balance));
}
else
{
Sender.Tell(new OperationResult(false, Balance));
}
});
}
}
// Usage
var system = ActorSystem.Create("BankSystem");
var account = system.ActorOf<BankAccount>("account");
var result = await account.Ask<OperationResult>(new Deposit(100m), TimeSpan.FromSeconds(5));
The Actor Model inherently handles concurrency by processing messages sequentially within each actor, eliminating many traditional concurrency issues.
Advanced Considerations
1. Distributed Consensus Algorithms
For distributed systems, consensus algorithms like Raft or Paxos are crucial for maintaining consistency across nodes.
2. Conflict-Free Replicated Data Types (CRDTs)
CRDTs are data structures that can be replicated across multiple computers in a network, updated independently, and eventually achieve a consistent state automatically.
3. Time-Based Concurrency Control
Techniques like Google's Spanner use TrueTime API to provide globally consistent timestamps, enabling precise ordering of distributed transactions.
Conclusion
Mastering concurrency management is a continuous journey that requires a deep understanding of both theoretical concepts and practical implications. The strategies and techniques outlined in this guide represent the cutting edge of concurrency control in modern software systems. By applying these advanced concepts, organizations can build highly reliable, scalable, and efficient systems capable of handling the most demanding computational tasks.
Subscribe to my newsletter
Read articles from David Shergilashvili directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
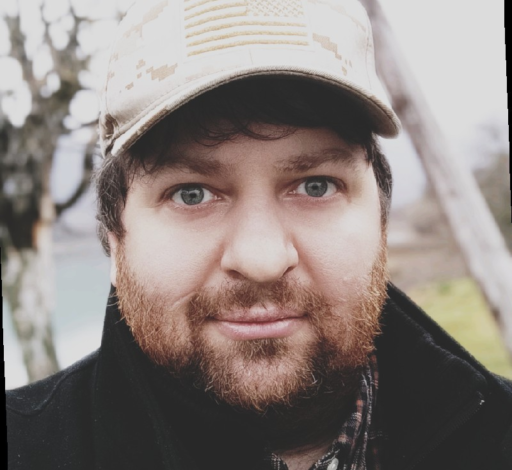