Day 7: Functions are Magic Spells ✨
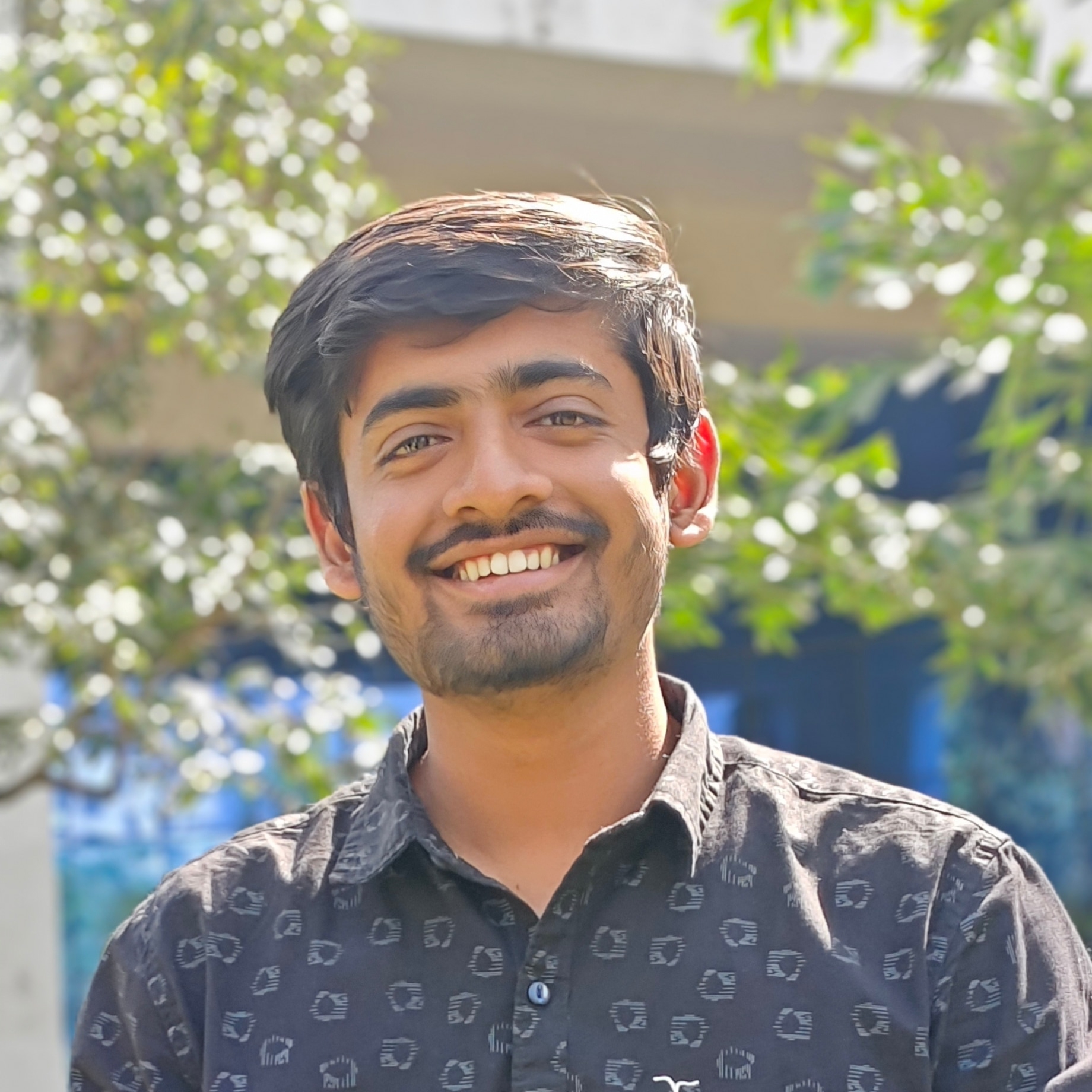
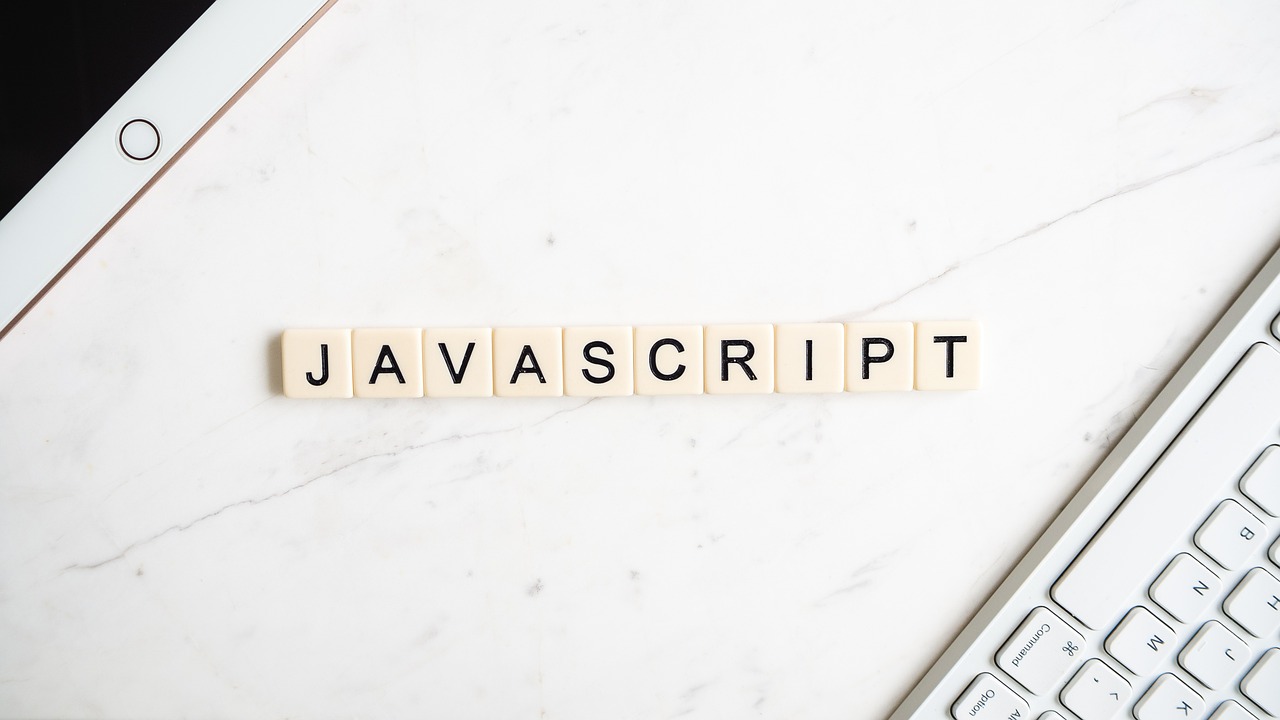
In JavaScript, functions are like magical spells – reusable snippets of code that let you summon the same logic without rewriting it every time! Whether it's casting an emoji charm 🧙♂️ or performing complex calculations, mastering functions is essential for every wizard... er, developer!
1️⃣ What is a Function?
A function is a block of code designed to perform a specific task. Think of it as a recipe that you can call whenever needed, instead of typing the same instructions again and again.
javascriptCopy codefunction greet() {
console.log("Hello, world!");
}
Here, greet()
is our magical spell – calling it prints "Hello, world!" every time.
2️⃣ Declaring Functions: Summon the Magic
You can create a function by declaring it.
Syntax:
javascriptCopy codefunction functionName(parameters) {
// code to execute
}
- Parameters are like ingredients. They can be passed into a function to customize what the function does.
Example:
javascriptCopy codefunction greetPerson(name) {
console.log(`Hello, ${name}!`);
}
greetPerson("Risharth"); // Hello, Risharth!
3️⃣ Calling Functions: Casting the Spell
To use (or invoke) a function, simply call its name followed by parentheses.
javascriptCopy codegreetPerson("John");
In this case, we pass "John"
as an argument to the function, making the output more personal.
4️⃣ Anonymous and Arrow Functions: New Spell Forms 🧙♀️
JavaScript also allows anonymous functions (no name) and arrow functions (a sleek ES6 way of writing functions).
javascriptCopy codeconst favorite = () => console.log("✨ JavaScript is magic!");
favorite(); // Output: ✨ JavaScript is magic!
Your Task 📝
Write a function that returns your favorite emoji!
javascriptCopy codefunction favoriteEmoji() {
return "😎";
}
console.log(favoriteEmoji()); // Output: 😎
You can call this function whenever you're in need of some emoji joy! 🎉
Why Functions are Awesome?
Reusability: Write once, use multiple times.
Maintainability: Organize your code into smaller, manageable pieces.
Readability: Makes your code neat and easier to understand.
🪄 Pro Tip: Functions help you work smarter, not harder. They're the ultimate JavaScript wizardry—whether you’re building websites, creating APIs, or just trying to return an emoji with flair!
Subscribe to my newsletter
Read articles from Risharth pardeshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
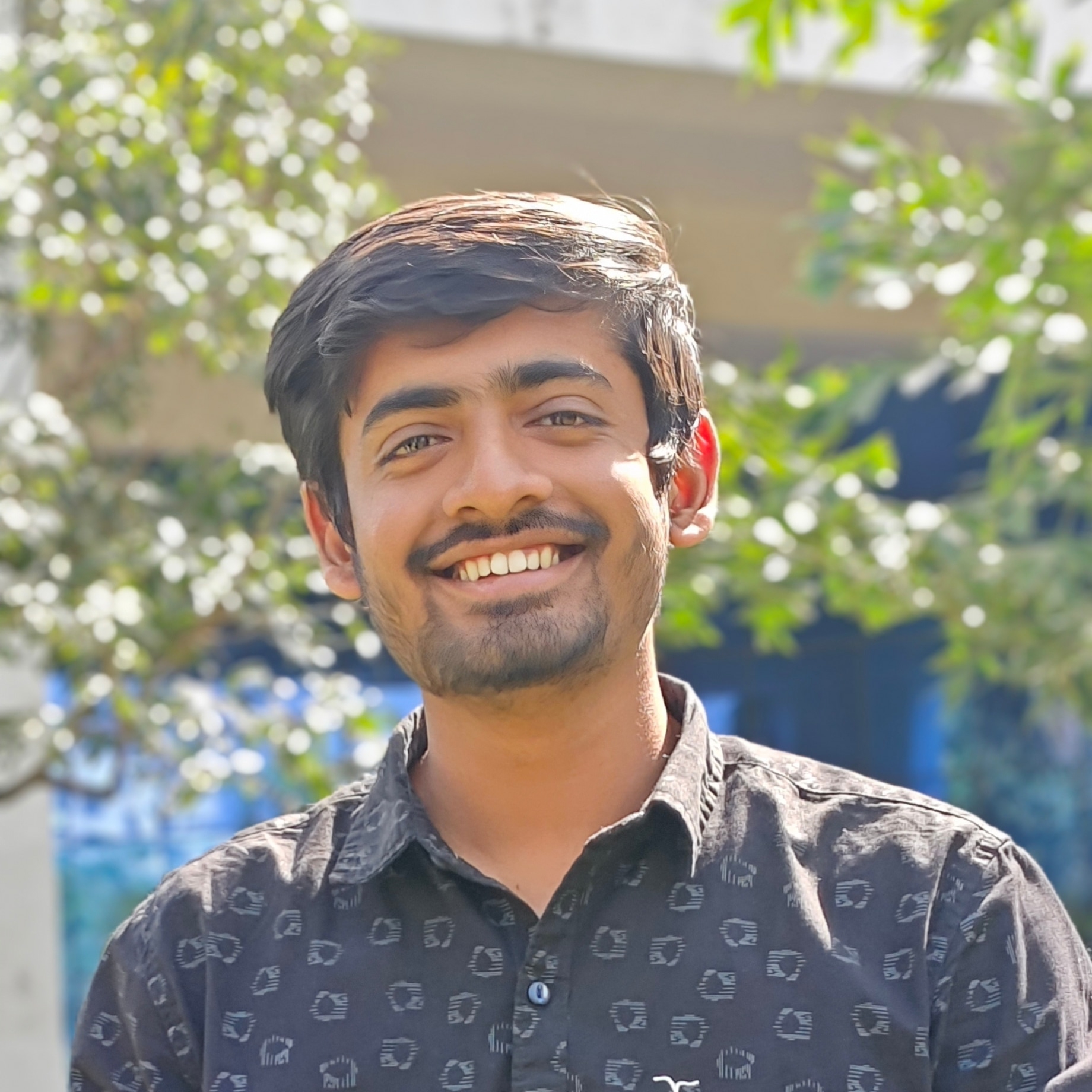
Risharth pardeshi
Risharth pardeshi
I hope this message finds you well. I am Risharth Pardeshi I am passionate about Computer Science and Engineering, and I have a strong foundation in this field. I graduated with a Bachelor of Engineering from the Laxmi Narayan College of Technology in Indore, India, in July 2024, with a CGPA of 7.98. Here is a brief overview of my technical strengths: Languages: C++, HTML, CSS, JavaScript, SQL Frameworks: React.js, Next.js, Tailwind CSS, Node.js Databases/OS: MySQL, MongoDB, Firebase, Linux Technical Skills: Data Structures and Algorithms, Git, DBMS, OS, Object-Oriented Programming, Computer Networking, Vercel, Postman