JavaScript Function Syntax
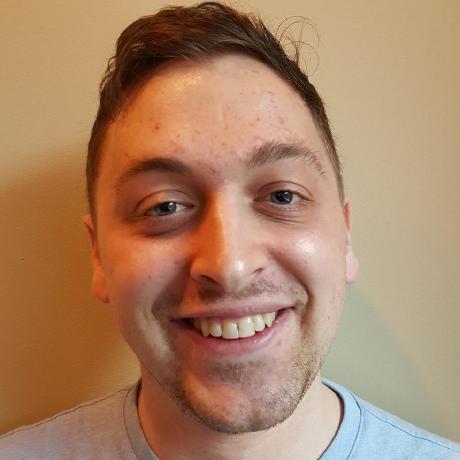
Function declaration
function multiply(a, b) {
return a * b;
}
Function declarations are a common in JavaScript, they are hoisted in that they can be declared after they are called like this:
const result = multiply(5, 5);
function multiply(a, b) {
return a * b;
}
Function expression
const multiply = function(a, b) {
return a * b;
}
Function expressions are used to assign a function to a variable. These are usually anonymous functions but can be named functions as the function name is optional. These are not hoisted so they can only be called after they are defined.
Arrow functions
const multiply = (a, b) => a * b;
Arrow functions were introduced in ES6 and they are basically a compact alternative to a function expression. One powerful thing with arrow functions besides the more concsise syntax is that they bind the lexical this to the surrounding context which eliminates common issues with callbacks.
Here is a few common examples of how an arrow function is used as a callback function argument:
[1, 2, 3, 4].map(n => n * 2); //[2, 4, 6, 8]
[1, 2, 3, 4].reduce((a, b) => a + b); //10
Methods
When you use functions inside of a class they are called methods. Classes were introduced in ES6 (2015). Methods are a shorter syntax for defining a function property on an object or class.
class MyClass {
add(a: number, b: number){
return a + b;
}
}
Here are three ways you can define a function/method in a class:
class MyClass {
// Method Definition - standard way to define a method in a class
// and is part of the class prototype
add(x: number, y: number) {
return x + y;
}
// Function Expression - assigns anonymous function to a class property
// The function is created and assigned at run time and is not part of
// the class prototype but is an instance property.
subtract = function(x:number, y:number) {
return x - y;
}
// Arrow Function - Just like a Function Expression but does not have its
// own `this` context but inherits it from the surrounding lexical scope.
multiply = (x: number, y: number) => x * y;
}
When the above code is transpiled to JS it looks like this:
"use strict";
class MyClass {
constructor() {
this.subtract = function (x, y) {
return x - y;
};
this.multiply = (x, y) => x * y;
}
add(x, y) {
return x + y;
}
}
Subscribe to my newsletter
Read articles from Brandon Feinstein directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
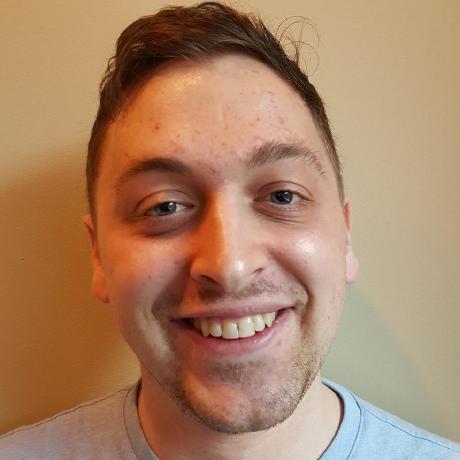