Day 4: React Learning Journey: Understanding Props in React
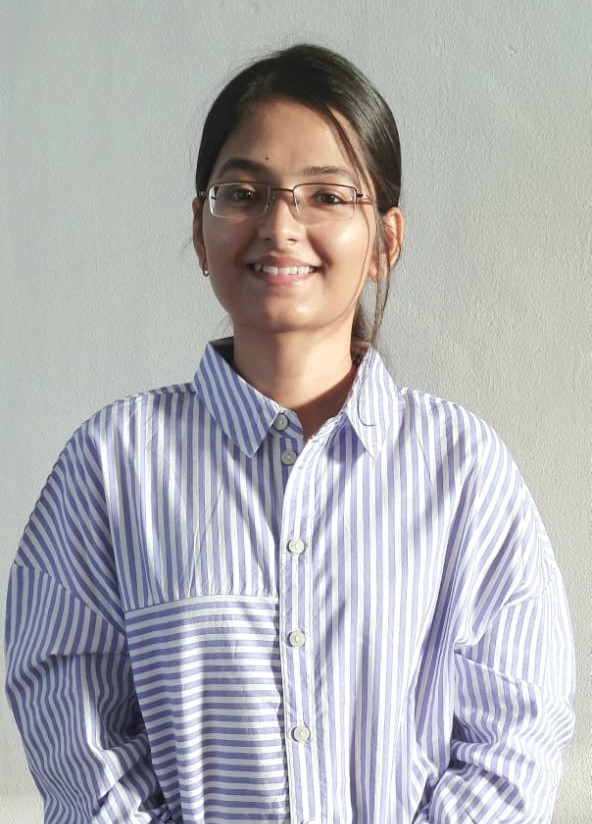
Table of contents
- Introduction
- What are Props in React?
- How Props Work in React
- Props vs State: Understanding the Difference
- Why Use Props in React?
- How to Pass Props to Components
- Accessing Props Inside a Component
- Destructuring Props in Functional Components
- Default Properties: Assigning Default Values
- Prop Types: Validating Props
- Passing Functions as Props
- Passing Complex Data Types as Props
- Props as Read-Only Data
- Common Mistakes When Working with Props
- Conclusion
- FAQs
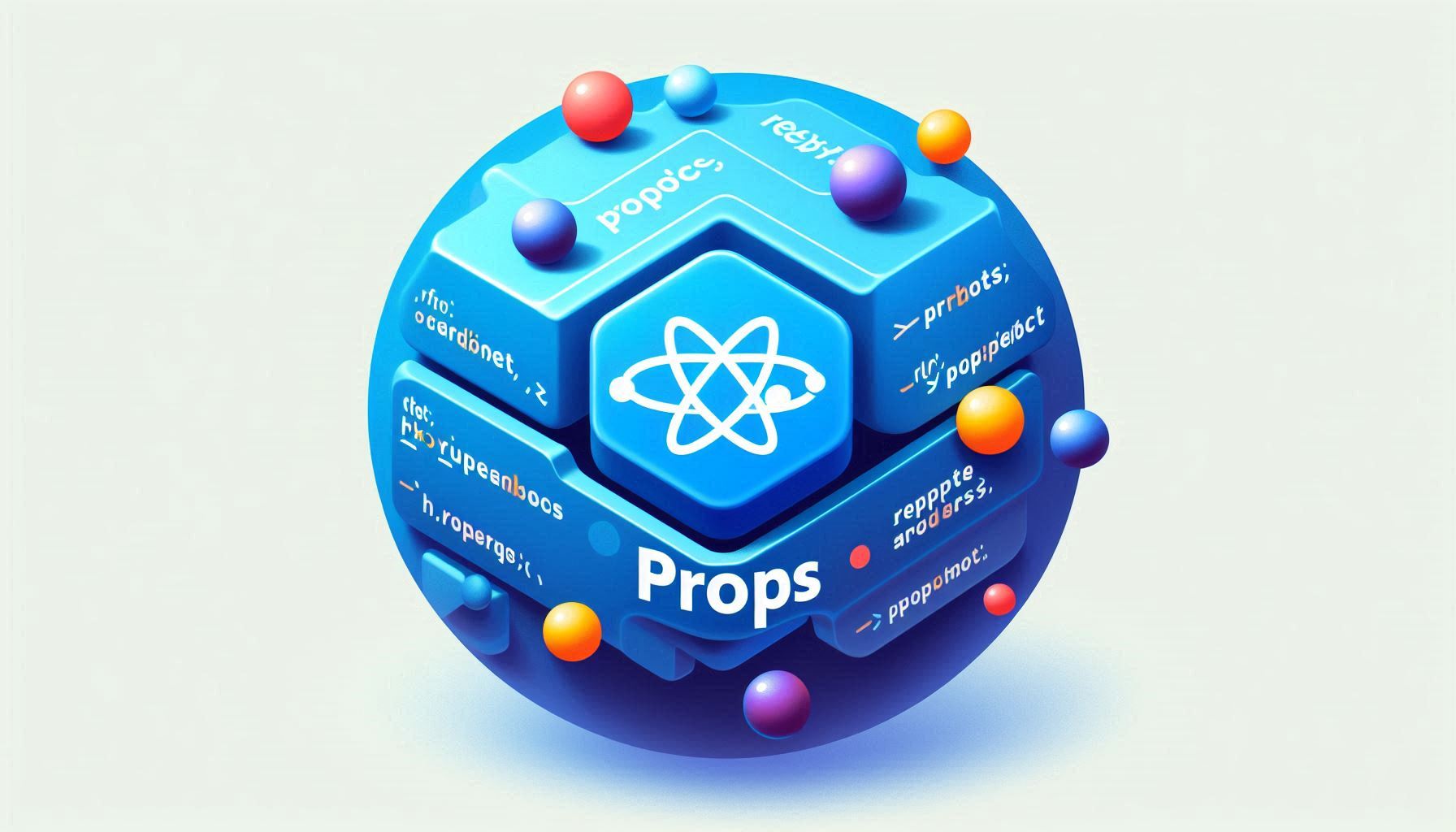
Introduction
Welcome back to Day 4 of your React Learning Journey! At this stage, you're probably starting to feel more comfortable with components and JSX. Today, we'll dive into a crucial concept in React that takes your app's interactivity to the next level—Props. We'll learn what they are, how they work, and why they are an essential part of React development.
Ready to enhance your React skills? Let's dive in!
What are Props in React?
Props, short for "properties," are how we pass data from one component to another in React. Think of props like the arguments you pass to a function. They allow components to be dynamic and reusable by letting you customize their behavior or appearance based on the data you pass.
Props are essentially the way React components communicate with each other. Just as people share information through conversation, React components share data using props.
How Props Work in React
The most common way to pass props from a parent component to a child component. The parent sends data down to the child, and the child can access and use this data to display content, adjust behavior, or trigger functions.
One key point to understand about props is that they flow in one direction—downward. React follows a unidirectional data flow model, meaning that data only moves from parent components to child components. This simplifies the structure of your app and makes it easier to understand.
Props vs State: Understanding the Difference
A common point of confusion when starting with React is understanding the difference between props and state. Here's a simple way to remember it:
Props are read-only and controlled by the parent component. They can't be changed by the component receiving them.
State, on the other hand, is mutable and controlled by the component itself. State can change over time, allowing the component to react to user inputs or other events.
Why Use Props in React?
One of the major benefits of using props is that they allow you to reuse components. Instead of hard-coding static values into components, you can pass different data via props, making components more flexible and maintainable. This is especially useful when building dynamic UIs that need to change based on user input or external data.
Props make your components more modular. For example, you can create a button component and reuse it in different parts of your app by simply passing different labels, styles, or actions as props.
How to Pass Props to Components
Passing props to a component is as easy as adding attributes to an HTML tag. Here’s a simple example:
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
<Greeting name="Alice" />
In the example above, the name
prop is passed to the Greeting
component, and it's accessed using props.name
inside the function.
Accessing Props Inside a Component
Depending on the type of component, the way you access props differs slightly:
In class components, props are accessed using
this.props
.In functional components, you access props through the parameter passed into the function:
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
Destructuring Props in Functional Components
Destructuring is a JavaScript feature that allows you to extract values from objects and arrays. It’s common to destructure props directly in the function's parameter list to keep the code clean and readable.
For example:
function UserProfile({ username, age }) {
return (
<div>
<h2>{username}</h2>
<p>Age: {age}</p>
</div>
);
}
This approach avoids having to write props.username
or props.age
repeatedly inside your component.
Default Properties: Assigning Default Values
Sometimes you may want to set default values for props in case they are not provided. React allows you to assign default props easily:
function Greeting({ name = "Guest" }) {
return <h1>Hello, {name}!</h1>;
}
This will render "Hello, Guest!" if no name prop is passed.
Prop Types: Validating Props
Proper validation ensures that components receive the correct type of data. React provides a way to validate the props a component receives using the PropTypes
package. Here’s how you can define PropTypes for a component:
import PropTypes from 'prop-types';
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
Greeting.propTypes = {
name: PropTypes.string.isRequired,
};
In this example, React will throw a warning if the name
prop is not a string.
Passing Functions as Props
Props aren’t limited to just data; you can also pass functions as props to components. This is extremely useful when you want a child component to interact with or trigger some logic in the parent component.
function Button({ handleClick }) {
return <button onClick={handleClick}>Click Me</button>;
}
<Button handleClick={() => console.log("Button clicked!")} />
Here, handleClick
is passed as a prop, and the button component can call it when clicked.
Passing Complex Data Types as Props
You can pass complex data types like arrays and objects through props as well.
function ItemList({ items }) {
return (
<ul>
{items.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
}
const itemList = [
{ id: 1, name: "Item 1" },
{ id: 2, name: "Item 2" }
];
<ItemList items={itemList} />
In this case, an array of items is passed as a prop, and the component renders them dynamically.
Props as Read-Only Data
Props are immutable—meaning that once a component receives props, it cannot change them. This is crucial for maintaining predictable behavior in your app. Trying to modify props inside a child component will lead to bugs or unintended side effects.
If a child component needs to modify data, you should handle it through state or by triggering a function passed down via props.
Common Mistakes When Working with Props
Forgetting to pass required props: If your component expects a prop and you forget to pass it, React will render incorrectly.
Modifying props: Attempting to modify props inside a child component will break React’s predictable data flow and could result in errors.
Conclusion
In summary, props are an essential part of building dynamic and reusable components in React. By understanding how to pass, access, and validate props, you're now one step closer to mastering React. In the next part of this journey, we'll dive deeper into more complex React concepts like state management and lifecycle methods.
Keep experimenting with props and try passing different data types and functions to see how they work in various scenarios!
FAQs
Can you pass state as a prop in React?
Yes, you can pass state from a parent component as a prop to a child component.How can I prevent a child component from re-rendering when a prop doesn’t change?
You can use itReact.memo()
to memoize the component and prevent re-rendering if the props haven't changed.What happens if a required prop is not passed?
If you have prop validation set up using ReactPropTypes
, React will log a warning in the console. The component may not render as expected.Can I pass multiple props to a component in React?
Absolutely! You can pass as many props as you want to a component by including them as attributes in the JSX tag.How do I pass props to deeply nested components?
You can pass props down the component tree manually, or use React Context to avoid "prop drilling" and pass data to deeply nested components efficiently.
Subscribe to my newsletter
Read articles from Sonal Diwan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
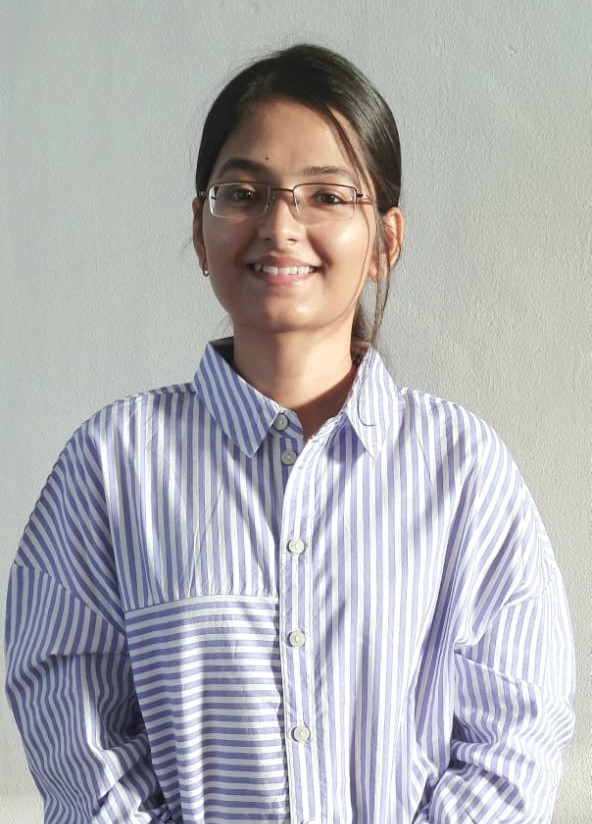
Sonal Diwan
Sonal Diwan
Data-Driven Fresher | Leveraging full stack technologies | Exploring tech-trends