My Interview Experience: Java Questions and Advice for Beginners

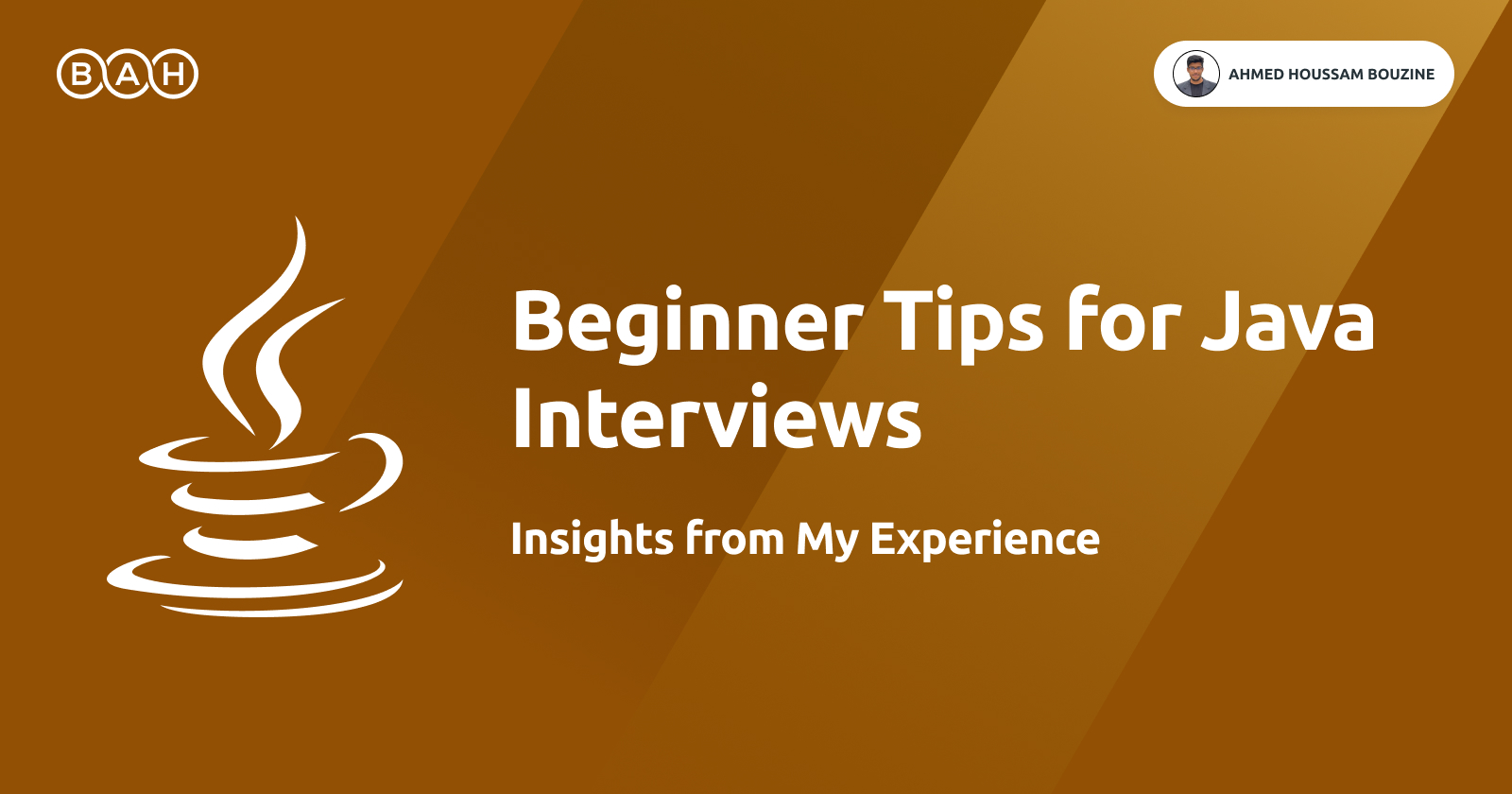
Introduction
During my search for an end-of-studies internship (PFE), I had a technical interview for a backend position, focusing on Java. This experience taught me that mastering Java fundamentals, articulating concepts clearly, and practicing coding challenges are essential for success and securing the job. Here's a comprehensive breakdown of the key areas I encountered and tips to help you prepare:
1. Master Core OOP Principles
What I was asked: The interviewer asked me to explain key Object-Oriented Programming (OOP) principles like Inheritance, Polymorphism, Encapsulation, and Abstraction.
Why it's important: OOP principles form the foundation of Java. A deep understanding of these concepts is essential to demonstrating your knowledge.
Key concepts:
Encapsulation: Grouping related data and methods in a class and restricting direct access using getters and setters.
Inheritance: Allowing one class to inherit properties and behaviors from another.
Polymorphism: The ability for objects to be treated as instances of their parent class or interface.
Abstraction: Hiding implementation details and showing only the essential features.
Tip: Be ready to explain each principle with real-life examples or a project you've worked on. This approach not only shows understanding but also brings theory to life.
2. Understand Java Memory Management and Garbage Collection
What I was asked: I was questioned about how Java handles memory management, specifically the heap and stack, and how garbage collection works.
Why it's important: While Java abstracts much of memory management, knowing how it works helps you avoid pitfalls like memory leaks, especially in large-scale systems.
Tip: You don't need to know every detail, but understanding key aspects like heap vs. stack memory and how garbage collection works is essential. In interviews, clarity on these fundamentals shows that you're not just relying on Java's automatic processes, but you understand them.
3. Exception Handling
What I was asked: The interviewer asked me to explain how exceptions work and how to handle them properly.
Why it's important: Exception handling ensures that your program can handle unexpected errors without crashing.
Key topics:
Checked vs. Unchecked Exceptions: Checked exceptions must be caught or declared, while unchecked exceptions typically occur during runtime.
Exception Hierarchy: Understand Throwable, the parent class of all exceptions, with subclasses Exception (checked) and RuntimeException (unchecked).
Try-Catch-Finally: Explain how try-catch handles exceptions and the role of finally for resource cleanup, even if exceptions occur.
Throw vs. Throws: throw is used to explicitly throw an exception, while throws declares exceptions in method signatures.
Custom Exceptions: When default exceptions are inadequate, create custom exceptions to represent specific error conditions.
Stack Trace: How it helps in debugging by showing the sequence of method calls leading to the exception.
Best Practices: Avoid catching generic exceptions, and ensure exceptions are logged for debugging.
Tip: Be ready to explain why and how you would throw and catch exceptions. Mention scenarios where it's better to create your own exceptions. This is a common interview topic that tests both your theoretical knowledge and practical application.
4. Java Features to Know
What I was asked: The interviewer inquired about features introduced in recent Java versions.
Why it's important: Staying up-to-date with recent Java features shows that you're current with the language's evolution and can use these features to write more efficient code.
Key features:
Java 8: Streams, Lambdas, Functional Interfaces, and the Optional class.
Java 11+: Local-variable syntax for lambda parameters (var), and enhancements to the Stream API.
Tip: Make sure you understand how to use these features in practical scenarios. For example, knowing how to simplify code with streams and lambdas can impress interviewers.
5. Differences Between Key Concepts
What I was asked: I had to explain the differences between commonly confused concepts in Java.
Examples:
HashMap vs. TreeMap: HashMap provides constant-time performance (O(1)) for basic operations, while TreeMap guarantees sorted keys with O(log n) complexity.
ArrayList vs. LinkedList: ArrayList offers fast random access but slow insertions/deletions, while LinkedList provides faster insertions/deletions but slower random access.
Interface vs. Abstract Class: Interfaces declare methods without implementation and support multiple inheritance, while abstract classes can have both declared and implemented methods but don't support multiple inheritance.
String vs. StringBuilder: String is immutable, while StringBuilder is mutable and more efficient for string manipulations.
Overloading vs. Overriding: Overloading involves multiple methods with the same name but different parameters in one class, while overriding occurs when a subclass provides a specific implementation for a superclass method.
Synchronous vs. Asynchronous: Synchronous operations wait for tasks to complete, while asynchronous operations can proceed without waiting.
Stack vs. Queue: Stacks follow Last-In-First-Out (LIFO), while queues follow First-In-First-Out (FIFO).
Tip: Always be prepared to clarify differences with examples. Practical distinctions are often more important than textbook definitions during an interview.
6. Threads and Multithreading
What I was asked: The interviewer asked me to explain multithreading in Java and how to manage concurrency.
Why it's important: Java applications often need to perform tasks concurrently. Mastering multithreading and concurrency is essential for building scalable and efficient systems.
Key topics:
Thread: A basic unit of CPU execution in Java, created by extending Thread class or implementing Runnable interface.
Thread Lifecycle: Know the states of a thread (New, Runnable, Blocked, Waiting, Timed Waiting, Terminated) and how threads transition between these states.
Volatile Keyword: Used to ensure visibility of changes to variables across threads, preventing the use of cached values by multiple threads.
Locks and Deadlock Avoidance: Understand how to use locks (ReentrantLock, ReadWriteLock) and avoid deadlocks by properly ordering locks and using tryLock or timeout strategies.
Concurrency Utilities: Learn the key utilities in java.util.concurrent, like CountDownLatch, CyclicBarrier, Semaphore, and ConcurrentHashMap.
Fork/Join Framework: A framework designed for parallelism in large, recursive tasks, where tasks can be split into smaller subtasks.
Thread Communication: Understand wait(), notify(), and notifyAll() for inter-thread communication in synchronized blocks.
Multithreading: Allows multiple tasks to execute simultaneously, but requires careful management to avoid issues like race conditions and deadlocks.
Synchronization: Ensures only one thread can access a resource at a time, preventing race conditions. Use synchronized blocks/methods or locks like ReentrantLock.
Thread Pools: Use ExecutorService to create thread pools for efficient thread management.
Tip: Be ready to explain thread lifecycles, how to handle shared resources with proper synchronization, and how to avoid common pitfalls like deadlocks. Practice writing concurrent programs to solidify your understanding.
7. Understanding the Java Virtual Machine (JVM)
Question: The interviewer delved into the Java Virtual Machine (JVM) and its role in Java's "Write Once, Run Anywhere" philosophy.
Why it's important: The JVM is fundamental to Java's operation and portability. Understanding it demonstrates a deep knowledge of how Java works under the hood.
Tip: Be prepared to discuss how the JVM contributes to Java's performance, security, and portability. Understanding JVM internals can set you apart in an interview and demonstrate a deeper comprehension of Java's ecosystem.
Conclusion
Preparing for a Java interview can feel overwhelming, but mastering the core concepts, practicing problem-solving, and understanding the fundamentals will go a long way. From Object-Oriented Programming principles to memory management, exception handling, and multithreading, each topic plays a critical role in your ability to showcase your skills effectively.
Remember, interviews aren’t just about having the right answers—they're also about demonstrating clear thinking and problem-solving approaches. Take time to explain your thought process, ask clarifying questions, and don’t hesitate to break down complex problems step by step.
With the right preparation, persistence, and a solid grasp of the topics discussed here, you’ll be well-equipped to tackle your next Java interview with confidence. Good luck!
Subscribe to my newsletter
Read articles from Ahmed Houssam BOUZINE directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ahmed Houssam BOUZINE
Ahmed Houssam BOUZINE
Tech enthusiast with a strong focus on Linux, Java, Rust, Cloud, and DevOps.