Mastering JavaScript Arrays: From Basics to Powerful Methods
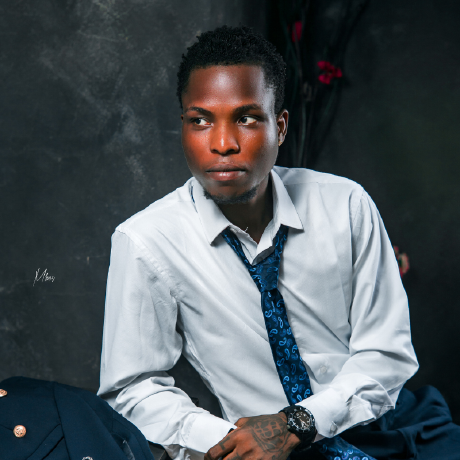
Table of contents
- What is a JavaScript Array?
- Creating and Accessing Arrays
- Useful JavaScript Array Methods
- 2. pop(): Removing the Last Element
- 3. shift(): Removing the First Element
- 4. unshift(): Adding Elements to the Beginning
- 5. splice(): Adding/Removing Elements at Specific Indexes
- 6. slice(): Copying Parts of an Array
- 7. map(): Transforming Array Elements
- 8. filter(): Filtering Elements Based on Conditions
- 9. reduce(): Reducing Array to a Single Value
- 10. forEach(): Iterating Over an Array
- Conclusion
- Thank you for reading! Happy coding!
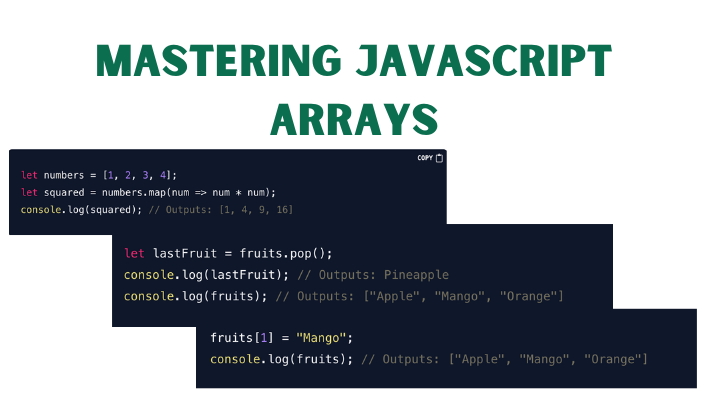
Arrays are one of the most commonly used data structures in JavaScript. If you’re new to JavaScript or looking to refine your skills, mastering arrays, and their methods can significantly enhance how you write code.
Today, I’ll share key insights into JavaScript Arrays and the Array Methods that make them versatile.
What is a JavaScript Array?
A JavaScript array is a data structure that stores multiple values in a single variable. Arrays can hold data types such as numbers, strings, objects, or even other arrays (making them multidimensional).
Here’s an example of a basic array:
let fruits = ["Apple", "Banana", "Orange"];
In this example, the array
fruits
holds three string values: "Apple", "Banana", and "Orange".
Creating and Accessing Arrays
You can create arrays using square brackets [], and access their elements using an index, where the first item starts at index 0.
console.log(fruits[0]); // Outputs: Apple
console.log(fruits[1]); // Outputs: Banana
You can also change the values by directly referencing their index:
fruits[1] = "Mango";
console.log(fruits); // Outputs: ["Apple", "Mango", "Orange"]
Useful JavaScript Array Methods
JavaScript provides several built-in array methods that simplify tasks like adding, removing, or manipulating elements. Let’s explore some of the most powerful ones:
1. push(): Adding Elements to an Array
The push() method adds one or more elements to the end of an array.
fruits.push("Pineapple");
console.log(fruits); // Outputs: ["Apple", "Mango", "Orange", "Pineapple"]
2. pop(): Removing the Last Element
The pop() method removes the last element from an array and returns that element.
let lastFruit = fruits.pop();
console.log(lastFruit); // Outputs: Pineapple
console.log(fruits); // Outputs: ["Apple", "Mango", "Orange"]
3. shift(): Removing the First Element
The shift() method removes the first element from an array and shifts all other elements down one position.
let firstFruit = fruits.shift();
console.log(firstFruit); // Outputs: Apple
console.log(fruits); // Outputs: ["Mango", "Orange"]
4. unshift(): Adding Elements to the Beginning
The unshift() method adds one or more elements to the beginning of an array.
fruits.unshift("Strawberry");
console.log(fruits); // Outputs: ["Strawberry", "Mango", "Orange"]
5. splice(): Adding/Removing Elements at Specific Indexes
The splice() method allows you to add or remove elements from any position in an array.
// Adding elements at index 1
fruits.splice(1, 0, "Blueberry", "Grapes");
console.log(fruits); // Outputs: ["Strawberry", "Blueberry", "Grapes", "Mango", "Orange"]
// Removing 2 elements starting at index 2
fruits.splice(2, 2);
console.log(fruits); // Outputs: ["Strawberry", "Blueberry", "Orange"]
6. slice(): Copying Parts of an Array
The slice() method creates a new array by extracting elements from an existing one, without modifying the original one.
let citrus = fruits.slice(1, 3);
console.log(citrus); // Outputs: ["Blueberry", "Orange"]
console.log(fruits); // Original array remains unchanged
7. map(): Transforming Array Elements
The map() method creates a new array by applying a function to each element in the original array. This is incredibly useful for transforming data.
let numbers = [1, 2, 3, 4];
let squared = numbers.map(num => num * num);
console.log(squared); // Outputs: [1, 4, 9, 16]
8. filter(): Filtering Elements Based on Conditions
The filter() method creates a new array with elements that pass a specific test (provided as a function).
let scores = [20, 45, 80, 65, 30];
let passingScores = scores.filter(score => score > 50);
console.log(passingScores); // Outputs: [80, 65]
9. reduce(): Reducing Array to a Single Value
The reduce() method applies a function to accumulate array values into a single result.
// Using reduce() to sum all numbers in the array
let sum = numbers.reduce((acc, curr) => acc + curr, 0);
// The 0 at the end is the initial value for the accumulator
console.log(sum); // Outputs the total sum of the array
10. forEach(): Iterating Over an Array
The forEach() method executes a function for each element in an array.
// Using forEach() to print each fruit in the array
let fruits = ["Apple", "Banana", "Mango"];
fruits.forEach(fruit => console.log(fruit));
// Outputs: Apple, Banana, Mango
Conclusion
Understanding and leveraging JavaScript Arrays and their powerful Array Methods allows you to write efficient, cleaner, and more manageable code. Whether you’re working with large data sets, manipulating content, or creating dynamic applications, arrays are at the heart of it all.
By mastering these array methods, you can take your JavaScript skills to the next level!
What's your favorite array method to use in JavaScript? Let's discuss this in the comments below! 🚀
Thank you for reading! Happy coding!
#JavaScript #Arrays #WebDevelopment #CodingJourney #TechLearning #Day4of30daysJavaScript
Subscribe to my newsletter
Read articles from Stanley Owarieta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
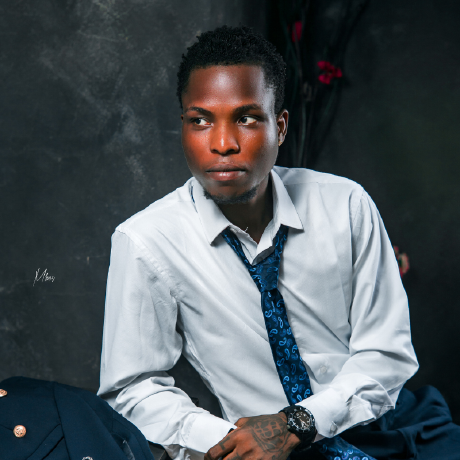
Stanley Owarieta
Stanley Owarieta
I help tech businesses and startups create engaging, SEO-driven content that attracts readers and converts them into customers. From in-depth blog posts to product reviews, I ensure every content is well-researched, easy to understand, and impactful. As a programming learner with HTML, CSS, and JavaScript knowledge, I bring a unique technical perspective to my writing. If you're looking for content that ranks and resonates, let's connect! 📩 Open to collaborations! Message me or email me at freelance@stanleyowarieta.com