Understanding the ForEach Loop in SwiftUI

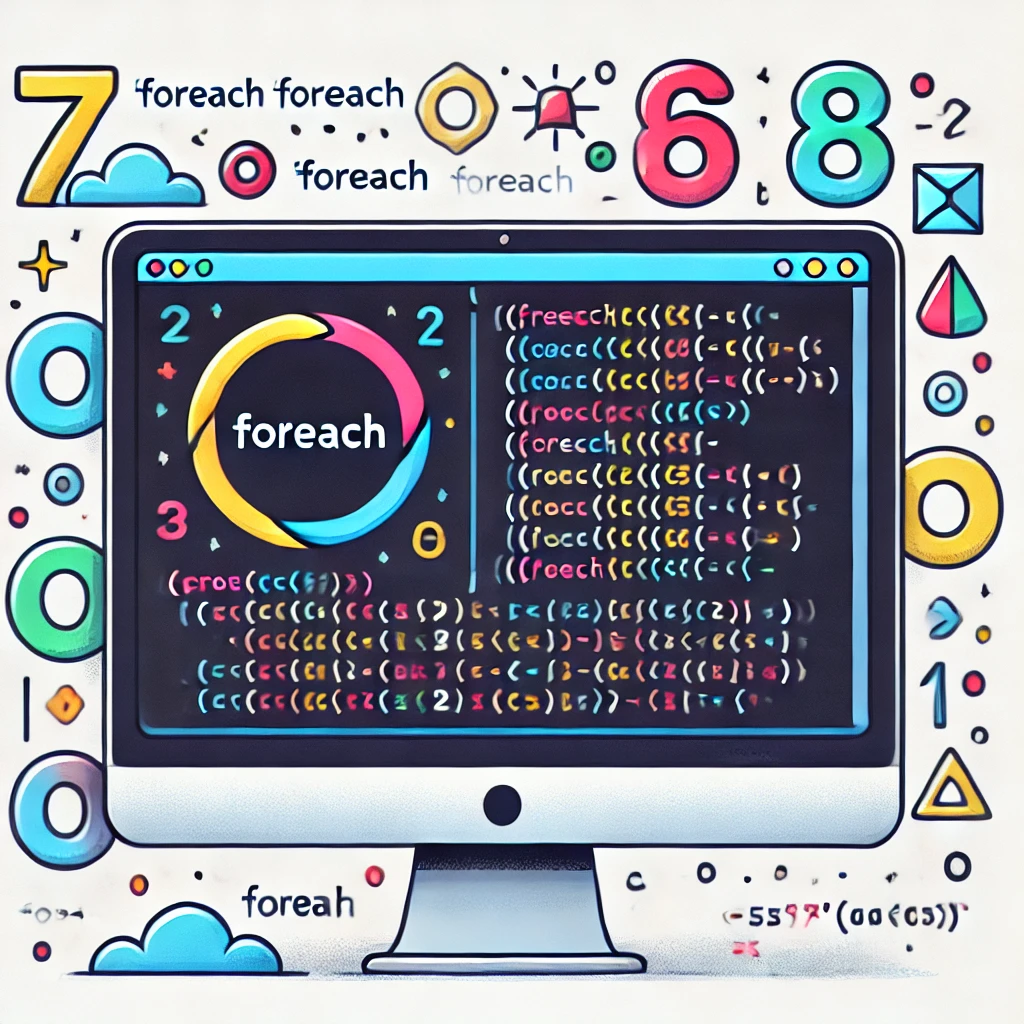
What is forEach
?
forEach
is a special function that belongs to collections like arrays and dictionaries. It helps you loop through each element in a collection and do something with it, such as printing it or performing an action.
Here’s the basic format of forEach
:
A Simple Example:
Let’s say we have a list of numbers, and we want to print them one by one. We can use forEach
to do this easily:
let numbers = [1, 2, 3, 4, 5]
numbers.forEach { number in
print(number)
}
This will print:
1
2
3
4
5
Easy, right? The forEach
loop helps you go through each number in the list and print it.
Difference between for-in
and forEach
:
for-in
allows you to break out of the loop with break
or continue
, while forEach
does not support this.
forEach
uses a closure, which may add slight overhead compared to a for-in
loop, but it's often used for its concise syntax.
Subscribe to my newsletter
Read articles from thevenkat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

thevenkat
thevenkat
I am a Senior iOS Developer, crafting Native iOS applications using Swift. My passion lies in creating innovative solutions that enhance user experience and drive customer satisfaction. I excel in problem-solving, code optimization, and staying updated with the latest trends and technologies in the iOS ecosystem. I thrive in collaborative environments and am always eager to share knowledge and learn from others.