Ngrok with FastAPI Made Easy: A Simple 4-Step Tutorial

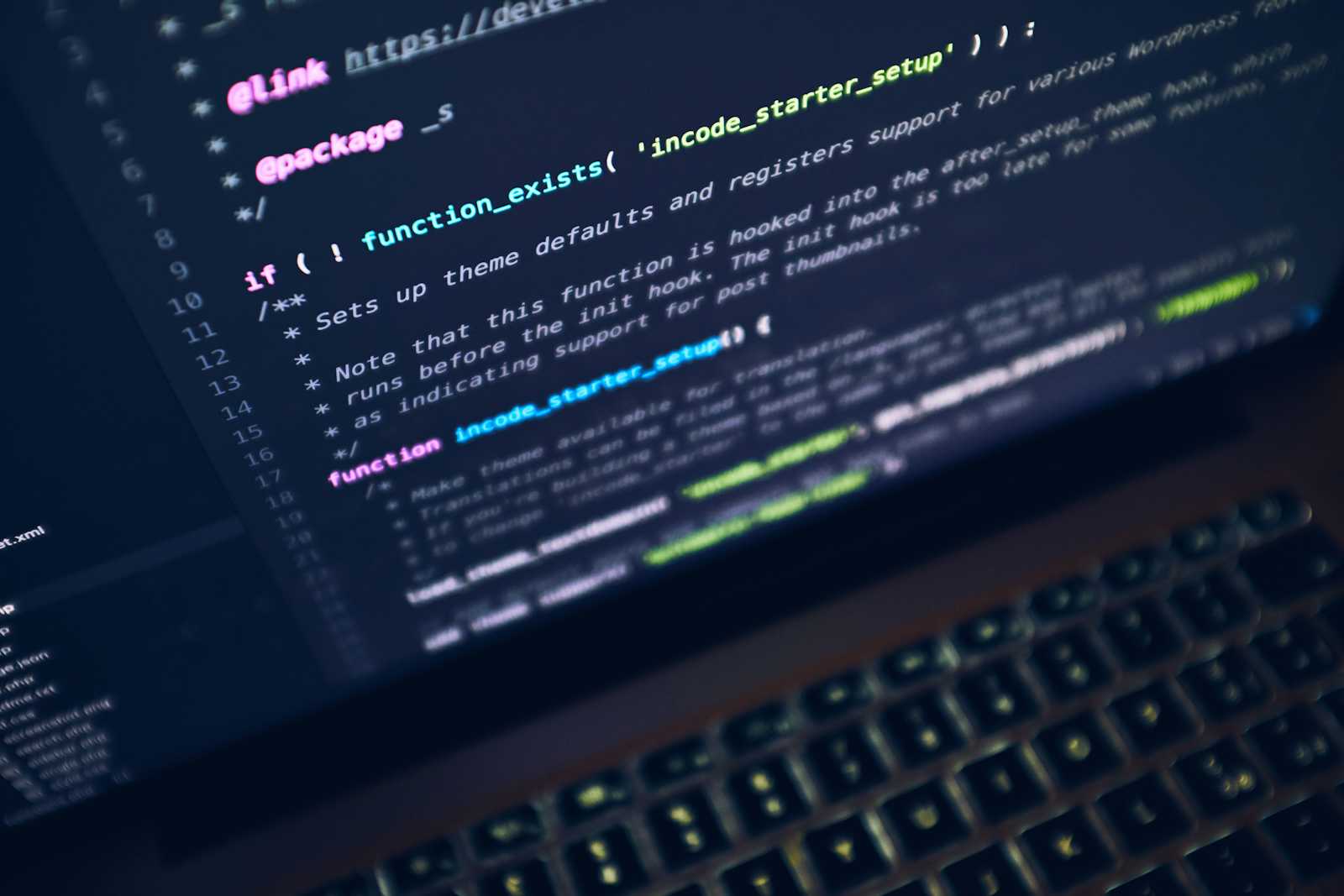
In modern web development, exposing local development servers to the internet for testing and debugging can be challenging. Enter Ngrok, a powerful tool that lets you securely expose a local server to the internet. When combined with FastAPI, a fast and high-performance web framework for Python, it provides developers with an easy way to test APIs in real-world scenarios without requiring full deployment. This guide will walk you through setting up Ngrok with FastAPI..
Prerequisites
Python 3.11+ installed on your machine.
Ngrok account (free or paid, depending on your needs).
Step 1: Installing required packages
Install the packages preferably on a virtualenv which can be setup using the virtualenv
python package.
pip install fastapi[all]
pip install ngrok
Step 2: Setup a simple FastAPI application
Create a simple main.py python application on Fastapi.
from fastapi import FastAPI
app = FastAPI()
@app.get('/greeting')
def get_greeting(name: str):
return {'message': f'Hello, {name}!'}
@app.get('/')
def get_root():
return {'message': 'Go to /docs to view the API documentation.'}
Test the application by running it using the uvicorn ASGI web server which gets installed as part of fastapi[all].
uvicorn main:app --port 8000
# On server startup test the application on http://localhost:8000/docs
# or http:localhost:8000/greeting?name=<your_name>
Step 3: Setup ngrok account and generate authtoken
Login to https://dashboard.ngrok.com/login and signup/login.
Upon login claim your free static domain from the set started screen.
Create a new tunnel auth token and save it from the auth tokens menu (You don’t have to change permissions)
Step 4: Integrate ngrok and fastapi
- Setup the
NGROK_DOMAIN
andNGROK_AUTH_TOKEN
environment variable
- Update code to add the ngrok tunnel
from fastapi import FastAPI
import ngrok
from contextlib import asynccontextmanager
import os
APPLICATION_PORT = 8000 # Specify the port number to be tunneled through ngrok
@asynccontextmanager
async def lifespan(app: FastAPI):
NGROK_DOMAIN = os.getenv('NGROK_DOMAIN')
NGROK_AUTH_TOKEN = os.getenv('NGROK_AUTH_TOKEN')
print(f"Setting up Ngrok Tunnel on {NGROK_DOMAIN}")
ngrok.set_auth_token(NGROK_AUTH_TOKEN)
ngrok.forward(
addr=APPLICATION_PORT,
domain=NGROK_DOMAIN,
)
yield
print("Tearing Down Ngrok Tunnel")
ngrok.disconnect()
app = FastAPI(lifespan=lifespan)
@app.get('/greeting')
def get_greeting(name: str):
return {'message': f'Hello, {name}!'}
@app.get('/')
def get_root():
return {'message': 'Go to /docs to view the API documentation.'}
- Run the application
uvicorn main:app --port 8000
# INFO: Started server process [21872]
# INFO: Waiting for application startup.
# Setting up Ngrok Tunnel on <your_ngrok_domain>
# INFO: Application startup complete.
# INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
🚀 And your application is now live on <your_ngrok_domain>
Conclusion
Using Ngrok with FastAPI allows you to quickly expose your local development server to the internet, making it an invaluable tool for testing, debugging, and demonstrating APIs. Whether you’re building a personal project or a larger application, this combination offers an easy and secure way to share your work with others.
Now that you know how to set it up, go ahead and start building! 💻
Subscribe to my newsletter
Read articles from Ashmin Jayson directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
