NestJS Microservices with RabbitMQ & PostgreSQL

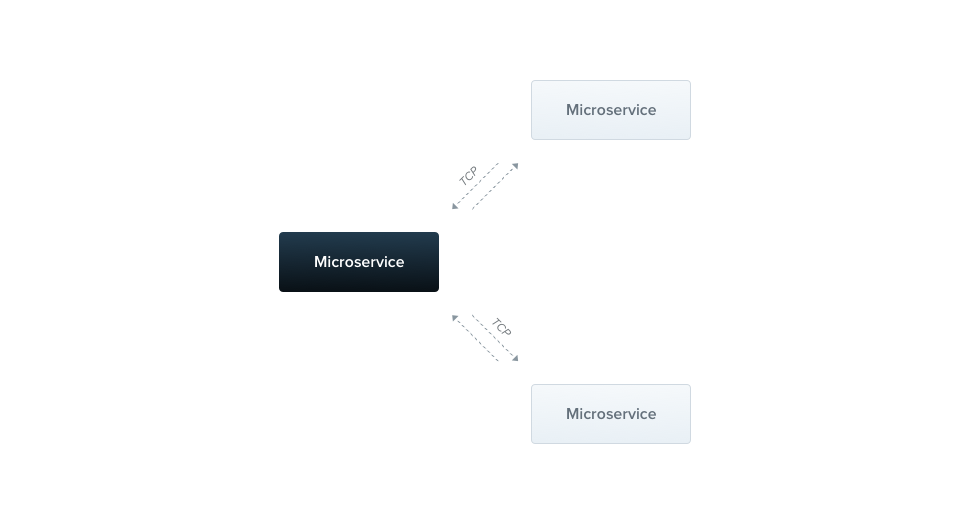
In this blog post, we will walk you through the process of setting up a task manager application using NestJS and RabbitMQ. This application is composed of multiple microservices, each with a specific responsibility. We will cover the installation, configuration, and usage of these microservices.
Microservices Overview
Our task manager application consists of the following microservices โ
Nashville (Backend Facing Frontend) Microservice: Handles incoming requests from the client and forwards them to the Gallatin microservice.
Gallatin (Task Manager) Microservice: Handles task-related operations (CRUD) and emits events to the Ashland microservice.
Ashland (Logger) Microservice: Listens for events from the Gallatin microservice and logs them to the console.
Client Microservice: A simple client that interacts with the Nashville microservice over WebSocket.io.
graph TD;
Client[Client] <--->|HTTP/WebSocket| Frontend[Backend Facing Frontend]
Frontend -->|gRPC| TaskManager[Task Manager]
TaskManager -->|DB Connection| PostgreSQL[PostgreSQL]
TaskManager -->|Message Queue| RabbitMQ[RabbitMQ]
RabbitMQ -->|Logs| Logger[Logger]
How It Works:
Client โ Backend Facing Frontend: Communicates via HTTP/WebSocket.
Backend Facing Frontend โ Task Manager: Uses gRPC for communication.
Task Manager โ PostgreSQL: Stores data.
Task Manager โ RabbitMQ: Sends tasks for processing.
RabbitMQ โ Logger: Logs task information.
Features
The task manager application provides the following features โ
Add new tasks
Delete existing tasks
Event-driven architecture using RabbitMQ
Prerequisites
Before you begin, ensure you have met the following requirements โ
NodeJS (>= 20.16.0)
Yarn (>= 1.22.19)
Docker (for RabbitMQ & PostgreSQL)
Installation
Follow these steps to set up the task manager application โ
- Clone the repository โ
git clone https://github.com/faizahmedfarooqui/nestjs-rabbitmq-task-manager.git
cd nestjs-rabbitmq-task-manager
- Install dependencies โ
yarn install
- Setup RabbitMQ โ
docker run -d --name rabbitmq -p 5672:5672 -p 15672:15672 rabbitmq:3-management
# Access the RabbitMQ management console at http://localhost:15672
# Username: guest
# Password: guest
- Setup PostgreSQL โ
docker run -d --name postgres -e POSTGRES_PASSWORD=yourpassword -p 5432:5432 postgres
# Access the PostgreSQL database at localhost:5432
# Username: postgres
# Password: yourpassword
- Configure Variables โ
Update the RabbitMQ and PostgreSQL configurations in
services/gallatin-task-manager/src/app.module.ts
Update the RabbitMQ configurations in
services/ashland-logger/src/main.ts
- Run the Nashville (Backend Facing Frontend) Microservice โ
yarn start:nashville
- Run the Gallatin (Task Manager) Microservice โ
yarn start:gallatin
- Run the Ashland (Logger) Microservice โ
yarn start:ashland
- Run the Client Microservice โ
yarn start:client
Running Tests
To ensure everything is working correctly, you can tests for each microservice โ
- Run all tests of Gallatin Task Manager Microservice โ
yarn test:gallatin
- Run all tests for Nashville BFF Microservice โ
yarn test:nashville
- Run all tests for Ashland Logger Microservice โ
yarn test:ashland
Conclusion
In this blog post, we have covered the setup and configuration of a task manager application using NestJS, RabbitMQ and PostgreSQL.
By following the steps outlined above, you should be able to get the application up and running, and understand the role of each microservice in the system.
You can find the codebase here โ
Happy coding!
About Me ๐จโ๐ป
I'm Faiz A. Farooqui. Software Engineer from Bengaluru, India.
Find out more about me @ faizahmed.in
Subscribe to my newsletter
Read articles from Faiz Ahmed Farooqui directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Faiz Ahmed Farooqui
Faiz Ahmed Farooqui
Principal Technical Consultant at GeekyAnts. Bootstrapping our own Data Centre services. I lead the development and management of innovative software products and frameworks at GeekyAnts, leveraging a wide range of technologies including OpenStack, Postgres, MySQL, GraphQL, Docker, Redis, API Gateway, Dapr, NodeJS, NextJS, and Laravel (PHP). With over 9 years of hands-on experience, I specialize in agile software development, CI/CD implementation, security, scaling, design, architecture, and cloud infrastructure. My expertise extends to Metal as a Service (MaaS), Unattended OS Installation, OpenStack Cloud, Data Centre Automation & Management, and proficiency in utilizing tools like OpenNebula, Firecracker, FirecrackerContainerD, Qemu, and OpenVSwitch. I guide and mentor a team of engineers, ensuring we meet our goals while fostering strong relationships with internal and external stakeholders. I contribute to various open-source projects on GitHub and share industry and technology insights on my blog at blog.faizahmed.in. I hold an Engineer's Degree in Computer Science and Engineering from Raj Kumar Goel Engineering College and have multiple relevant certifications showcased on my LinkedIn skill badges.