Real-Time Communication in Web Applications Using Socket.io
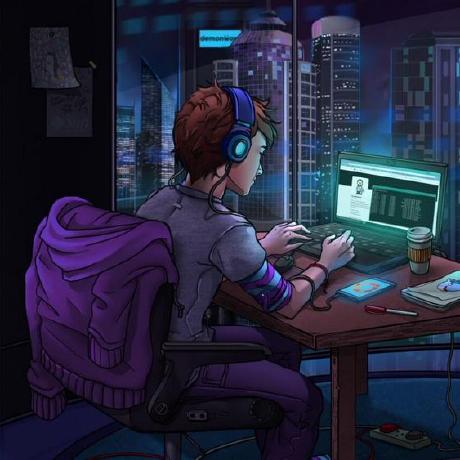
In today's fast-paced digital environment, real-time communication is a cornerstone of many modern web applications. Whether it’s for live updates in social media, collaboration tools, or network monitoring, the need for instantaneous data transfer has become increasingly important. In this post, I’ll walk through how I implemented real-time communication in my cybersecurity monitoring tool using Socket.io, and why this technology plays a critical role in my project.
The Importance of Real-Time Communication in Cybersecurity Monitoring
In the world of cybersecurity, time is of the essence. The faster you can detect anomalies or potential threats in network traffic, the quicker you can respond to mitigate risks. This is where real-time communication becomes crucial. For example, if my monitoring tool detects a Distributed Denial of Service (DDoS) attack, or suspicious network behavior, immediate alerts are sent to administrators for timely action.
In traditional web applications, data is often fetched using HTTP requests. However, for applications like network monitoring, constant polling of the server introduces delays and is resource-intensive. Instead, WebSockets, a communication protocol that allows persistent connections between the client and server, offers a more efficient solution. With Socket.io, built on top of WebSockets, I was able to implement real-time updates seamlessly.
How I Implemented Socket.io in My Flask and React Setup
Backend: Flask Integration
For the backend of my cybersecurity tool, I used Flask, a lightweight and flexible Python framework. To implement Socket.io, I installed the flask-socketio
extension, which provides WebSocket support for Flask applications.
Here’s a quick overview of how I set it up:
Install the necessary dependencies:
bashCopy codepip install flask-socketio pip install eventlet # Optional, for async support
Set up Socket.io on the Flask server:
pythonCopy codefrom flask import Flask, render_template from flask_socketio import SocketIO, emit app = Flask(__name__) socketio = SocketIO(app) # Endpoint to monitor traffic @socketio.on('connect') def handle_connect(): emit('message', {'data': 'Connected to server'}) @socketio.on('network_data') def handle_network_data(data): # Process network monitoring data here emit('alert', {'data': 'Anomaly detected!'}, broadcast=True) if __name__ == '__main__': socketio.run(app)
This code snippet shows how a client connects to the server, and how network monitoring data is received and processed. If an anomaly is detected, an alert is emitted back to all connected clients.
Frontend: React and Socket.io Client
On the frontend, I used React to create a dynamic and interactive user interface. To integrate Socket.io, I installed the socket.io
-client
package, which allows React to listen for real-time updates from the Flask backend.
Install the Socket.io client:
bashCopy codenpm install socket.io-client
Connect React to the Socket.io server:
javascriptCopy codeimport React, { useEffect, useState } from 'react'; import io from 'socket.io-client'; const socket = io('http://localhost:5000'); const NetworkMonitor = () => { const [message, setMessage] = useState(''); const [alerts, setAlerts] = useState([]); useEffect(() => { // Listen for a connection message socket.on('message', (data) => { setMessage(data.data); }); // Listen for anomaly alerts socket.on('alert', (data) => { setAlerts((prevAlerts) => [...prevAlerts, data.data]); }); return () => { socket.disconnect(); }; }, []); return ( <div> <h2>Network Monitor</h2> <p>Status: {message}</p> <div> <h3>Alerts</h3> <ul> {alerts.map((alert, index) => ( <li key={index}>{alert}</li> ))} </ul> </div> </div> ); }; export default NetworkMonitor;
In this component, I connect the frontend to the Flask Socket.io server. When the client receives an anomaly alert from the server, it dynamically updates the alert list in real-time, giving users live feedback on network security events.
Performance Considerations: Handling Multiple Connections
One of the challenges of building real-time applications is handling multiple simultaneous connections efficiently. As more users or devices connect to the application, the server needs to manage a high number of WebSocket connections without performance degradation.
Here are some of the key performance considerations I took into account:
Efficient Resource Management:
Eventlet: I chose to use Eventlet as the asynchronous networking library for Flask, allowing the server to handle thousands of simultaneous clients without blocking.
Broadcasting: In scenarios where multiple clients need to receive the same data (e.g., network anomaly alerts), Socket.io’s broadcast feature was highly efficient. It allows me to emit data to all connected clients in one action, reducing overhead.
Error Handling and Timeouts:
I implemented error handling for cases where the WebSocket connection might drop or encounter errors. Reconnecting the client automatically when the connection is lost is crucial for ensuring consistent monitoring.
Timeouts were set up to avoid hanging connections, ensuring that idle clients do not waste server resources.
Scaling with Multiple Servers:
- To scale the real-time application for larger environments, I considered deploying the Flask server using Redis as the message broker. This allows multiple Socket.io servers to work together and share the load of handling WebSocket connections.
Conclusion
Implementing real-time communication in a web application using Socket.io is both challenging and rewarding. In my cybersecurity monitoring tool, it plays a crucial role in providing instantaneous feedback on network activity, allowing for rapid response to potential threats. From Flask to React, the integration of Socket.io enables smooth, real-time data transfer between the backend and frontend, enhancing the responsiveness and scalability of the application.
By carefully managing performance and ensuring robust error handling, I was able to create a system capable of handling multiple simultaneous connections without sacrificing speed or reliability. Real-time applications like this are not only in demand but also crucial for modern, high-performance web tools.
Subscribe to my newsletter
Read articles from Amon Kiprotich directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
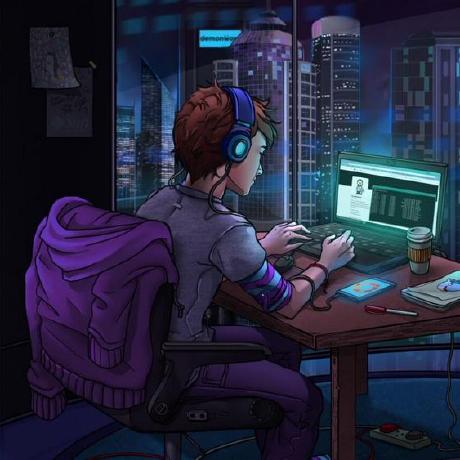