Understanding TypeError: Using len() with Integers in Python

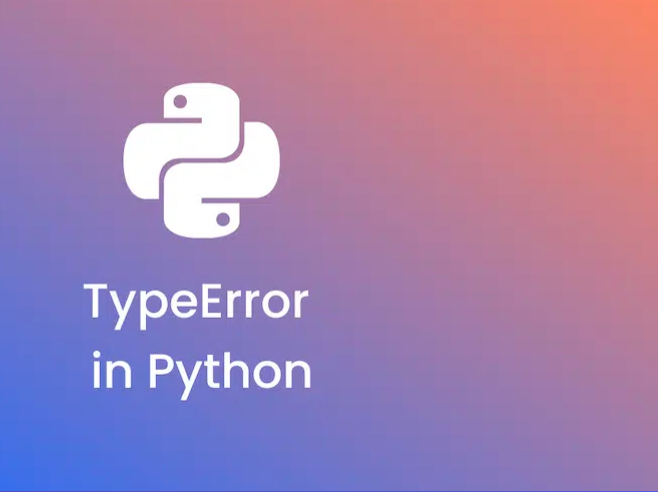
Python is a powerful language, but even seasoned developers can run into errors. One common mistake occurs when trying to use the len()
function on an integer. In this post, we’ll explore why this happens and how to handle it.
The len()
function is used to determine the number of items in a container like a a string, list, dictionary and tuple. However, applying it to an integer raises a TypeError
:
Example 1
n = 1555
print(len(n)) # Raises TypeError: object of type 'int' has no len()
To find the number of digits in an integer, convert it to a string first:
n = 1555
print(len(str(n))) # Output: 4
Example 2:
m = 5667
for i in range(1, len(m)):
print(i)
print(i(m))
The code snippet above would result into two key errors:
The function
len()
cannot be used directly on an integer because integers do not have a length.i(m)
is incorrect becausei
is just a loop variable (an integer) and not a function. TypeError: 'int' object is not callable.The correct way to do it:
m = 5667
for i in range(1, len(str(m)) + 1):
print(i) # Prints numbers from 1 to the length of the digits in m
print(m) # Prints the value of m in each iteration
Output:
1
5667
2
5667
3
5667
4
5667
Understanding data types is crucial in Python programming. Always ensure that the function you’re using is compatible with the data type to avoid errors.
Subscribe to my newsletter
Read articles from Abu Precious O. directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abu Precious O.
Abu Precious O.
Hi, I am Btere! I am a software engineer, and a technical writer in the semiconductor industry. I write articles on software and hardware products, tools use to move innovation forward! Likewise, I love pitching, demos and presentation on different tools like Python, AI, edge AI, Docker, tinyml, software development and deployment. Furthermore, I contribute to projects that add values to life, and get paid doing that!