Important Annotations in Java Spring Boot

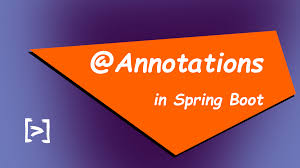
In this blog, we’ll take a look at some of the most important annotations in Spring Boot, explain their use, and show how they make developing applications faster and easier.
1. What Are Annotations in Java Spring Boot?
In Java, annotations are metadata that provide data about the program but do not affect the program’s operation directly. They are often used to give instructions to the compiler, or for runtime processing.
In Spring Boot, annotations play a crucial role by allowing developers to declare what their code should do in a clean, readable way. Instead of writing lengthy XML configurations, annotations allow you to configure your application directly in the code.
2. Core Spring Boot Annotations
@SpringBootApplication
The @SpringBootApplication
annotation is the heart of any Spring Boot application. It combines three annotations:
@Configuration: Marks the class as a source of Spring bean definitions.
@EnableAutoConfiguration: Tells Spring Boot to automatically configure your application based on the dependencies you have added.
@ComponentScan: Scans the package and its sub-packages for Spring components.
@SpringBootApplication public class MySpringBootApp { public static void main(String[] args) { SpringApplication.run(MySpringBootApp.class, args); } }
This annotation saves you from having to manually define configurations or component scanning.
@RestController
@RestController
is a convenient annotation that combines @Controller
and @ResponseBody
. It is used to create RESTful web services in Spring Boot.
@Controller is used to define a controller, while @ResponseBody returns the data directly instead of rendering a view.
@RestController public class HelloController { @GetMapping("/hello") public String sayHello() { return "Hello, World!"; } }
@RequestMapping
The
@RequestMapping
annotation is used to map web requests to specific handler functions. It can be applied to a class or method, allowing you to handle different types of HTTP requests such asGET
,POST
,PUT
, andDELETE
.It has specialized variants like:
@GetMapping("/users") public List<User> getUsers() { return userService.getAllUsers(); }
@Autowired
The
@Autowired
annotation is used for dependency injection. Spring Boot uses this annotation to automatically inject dependencies into your classes. It can be applied to fields, constructors, or setters.@Service public class UserService { @Autowired private UserRepository userRepository; public List<User> getAllUsers() { return userRepository.findAll(); } }
3. Additional Essential Annotations
@Service
The
@Service
annotation marks a class as a service that contains business logic. Spring automatically registers such classes as beans in the Spring context.@Service public class OrderService { public void placeOrder(Order order) { // business logic to place an order } }
@Repository
The
@Repository
annotation is used to indicate that a class is part of the data access layer. It is typically used for database interaction. Spring also translates any persistence-related exceptions into Spring'sDataAccessException
.@Repository public interface UserRepository extends JpaRepository<User, Long> { }
@Entity
@Entity
is used in the context of JPA (Java Persistence API). It marks a class as a JPA entity, meaning it will map to a table in the database.@Entity public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String name; private String email; // Getters and setters }
@Transactional
The
@Transactional
annotation ensures that a series of database operations are treated as a single transaction. If any operation within the transaction fails, all changes are rolled back.@Transactional public void transferMoney(Account from, Account to, double amount) { withdraw(from, amount); deposit(to, amount); }
@Configuration
This annotation indicates that the class contains Spring configuration. It allows you to define beans using the
@Bean
annotation.@Configuration public class AppConfig { @Bean public ModelMapper modelMapper() { return new ModelMapper(); } }
4. Security-Related Annotations
@Secured and @PreAuthorize
These annotations are used to secure methods based on roles or permissions.
@Secured defines roles that are allowed to execute the annotated method.
@PreAuthorize allows more complex expressions for securing methods.
@Secured("ROLE_ADMIN")
public void deleteUser(Long userId) {
// method accessible only to admins
}
5. Real-World Application of Annotations
Imagine you're building a REST API for a bookstore. You can use @RestController
to define your endpoints, @Autowired
to inject services, and @Repository
to handle database operations. @Transactional
can ensure that operations like adding or removing books are safely wrapped in transactions.
Conclusion
Annotations are a powerful feature of Spring Boot that make your code cleaner, more readable, and easier to maintain. By understanding and applying the right annotations, you can simplify the development process and build robust applications efficiently.
Whether you are a beginner or an experienced Java developer, mastering these annotations is essential for creating Spring Boot applications. So go ahead and explore these annotations in your projects!
Subscribe to my newsletter
Read articles from Tanish Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
