Basic Linux Shell Scripting for DevOps Engineers

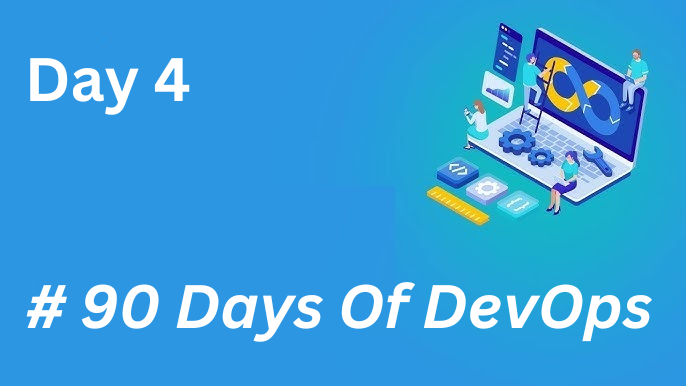
As a DevOps engineer, it's crucial to understand key components of the operating system like the kernel and shell, and how they play a role in shell scripting. These concepts allow you to automate tasks, manage servers, and optimize processes. Let's break down these concepts in an easy-to-understand way with examples, bullet points, and a sprinkle of emojis! 🚀
🧠 What is the Kernel?
The kernel is the "boss" of your computer—it controls everything happening under the hood, ensuring that your programs and hardware work together smoothly. Think of your computer as a big company:
🏢 Kernel as the Manager: It tells the employees (programs) how to do their jobs and ensures each gets enough resources.
⏳ Resource Allocation: It decides how much time each program gets to use the company’s tools (like CPU or memory).
🔄 Efficient Task Switching: It ensures that when one program is done using something, another can take over without crashing the system.
Without the kernel, programs wouldn’t know how to use the computer’s resources effectively, like memory and CPU. It’s like the manager organizing everything behind the scenes!
💬 What is the Shell?
Now that we know the kernel is the "boss," the shell acts as the company's receptionist. It communicates between you (the user) and the operating system (kernel).
💡 Company Example:
Receptionist (Shell): When you enter a company, you don’t go directly to the CEO (kernel). Instead, you first interact with the receptionist (shell). You give instructions (commands), and the receptionist passes them to the appropriate department (OS).
How It Works:
You (the user) ask the shell (receptionist) for something, e.g., "Show me the files in this folder" (
ls
).The shell communicates your request to the OS.
The OS processes the request and sends the result back to the shell.
The shell shows you the result (e.g., list of files).
In short, the shell is a program that allows you to give commands to the operating system, making it easier for you to interact with the computer’s internal functions.
📝 What is Linux Shell Scripting?
Linux Shell Scripting is like giving the computer a set of instructions, written in a file, that it can follow automatically. It’s like an employee following a checklist at a company to complete tasks efficiently.
🔄 Automating Tasks with Shell Scripting:
Imagine a small company where employees have to do repetitive tasks every day, like:
📊 Sending daily reports
🗄️ Backing up important files
🧹 Cleaning up temporary files
Instead of manually doing these tasks every day, a shell script can automate them! Let’s look at a few examples:
💻 Example Tasks for Automation:
Sending Daily Reports:
cat /path/to/report.txt | mail -s "Daily Report" manager@company.com
Backing Up Files:
tar -czf backup_$(date +%F).tar.gz /path/to/documents scp backup_*.tar.gz backup@backup-server:/path/to/backup
Cleaning Up Temporary Files:
rm -rf /path/to/temp/*
🚀 Key Points:
Automation: Just like an employee following a checklist, the computer uses a shell script to perform tasks automatically.
Efficiency: Saves time by automating repetitive tasks.
Simplicity: Scripts are written using simple Linux commands the computer understands.
In real-world DevOps, shell scripting automates routine tasks, making processes more efficient and freeing up engineers for more complex work.
✨ Tasks for DevOps Engineers
1️⃣ What Does Shell Scripting Mean for DevOps?
Shell scripting in DevOps is like giving the computer a list of instructions to automate repetitive tasks. Instead of manually typing commands, we write a script (a small program) to handle the work.
🚀 Why Is It Important in DevOps?
Automate Deployments: Push new code to servers.
Manage Servers: Restart services, clean up logs.
Monitor Systems: Check if a server is running smoothly.
By automating these daily operations, shell scripting saves time and reduces human error.
Example:
Imagine you manage a web app, and every day you need to:
🛠️ Check if the web server is running.
🧹 Clean up old log files.
🗄️ Backup the database.
Instead of doing this manually, you can create a shell script that does all of these tasks automatically!
2️⃣ What is #!/bin/bash
? Can We Write #!/bin/sh
?
The line #!/bin/bash
(or #!/bin/sh
) at the beginning of a script is called a shebang. It tells the operating system which interpreter should execute the script.
⚙️ Explanation:
#!/bin/bash
: This indicates the script should be run using the Bash shell. Bash is a common shell used on Unix-like systems with many features.#!/bin/sh
: This runs the script using the sh shell, which is more minimal and often used for simple scripts.
🛠️ Company Example:
#!/bin/bash
: Use this if your script needs advanced Bash-specific features.#!/bin/sh
: Use this for simpler scripts to ensure compatibility across different systems.
3️⃣ Write a Shell Script that Prints I will complete #90DaysOfDevOps Challenge
.
#!/bin/bash
echo "I will complete #90DaysOfDevOps challenge"
4️⃣ Write a Shell Script That Takes User Input and Prints the Variables.
#!/bin/bash
echo "Enter your name:"
read name
echo "Hello, $name!"
You can also pass input as arguments:
#!/bin/bash
echo "First argument: $1"
echo "Second argument: $2"
5️⃣ Example of an If-Else Statement in Shell Scripting by Comparing Two Numbers.
#!/bin/bash
num1=10
num2=20
if [ $num1 -gt $num2 ]; then
echo "$num1 is greater than $num2"
else
echo "$num1 is less than or equal to $num2"
fi
That’s a wrap! 🎉 With a solid understanding of the kernel, shell, and shell scripting, you’re ready to take on DevOps automation challenges. Start writing scripts to automate tasks, save time, and make your life as a DevOps engineer more efficient!
Subscribe to my newsletter
Read articles from Ramiz Takildar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
