Graph Traversal: Breadth-First Search and Depth-First Search | Day #21
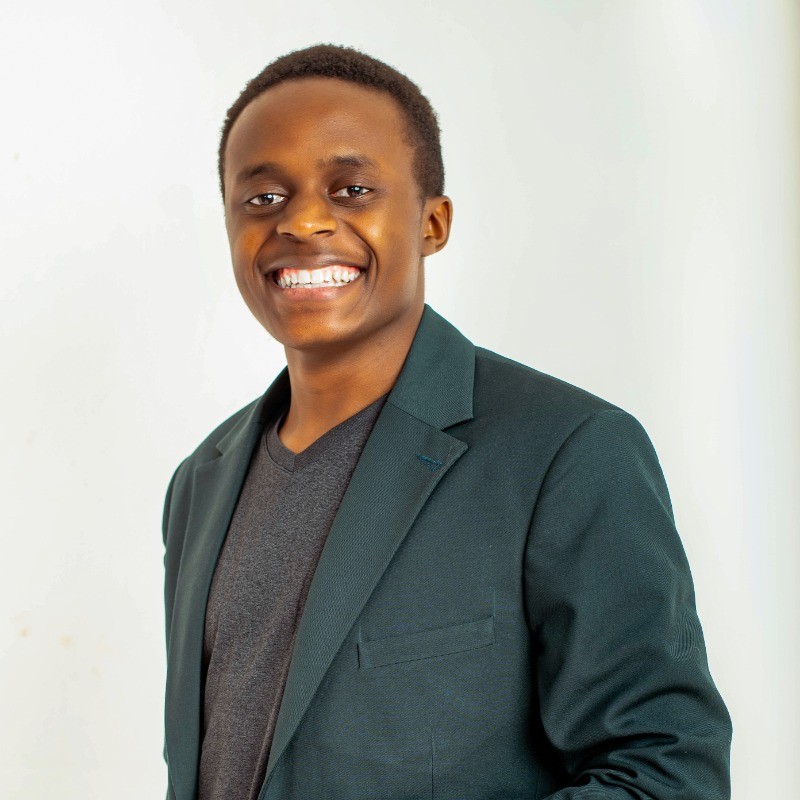
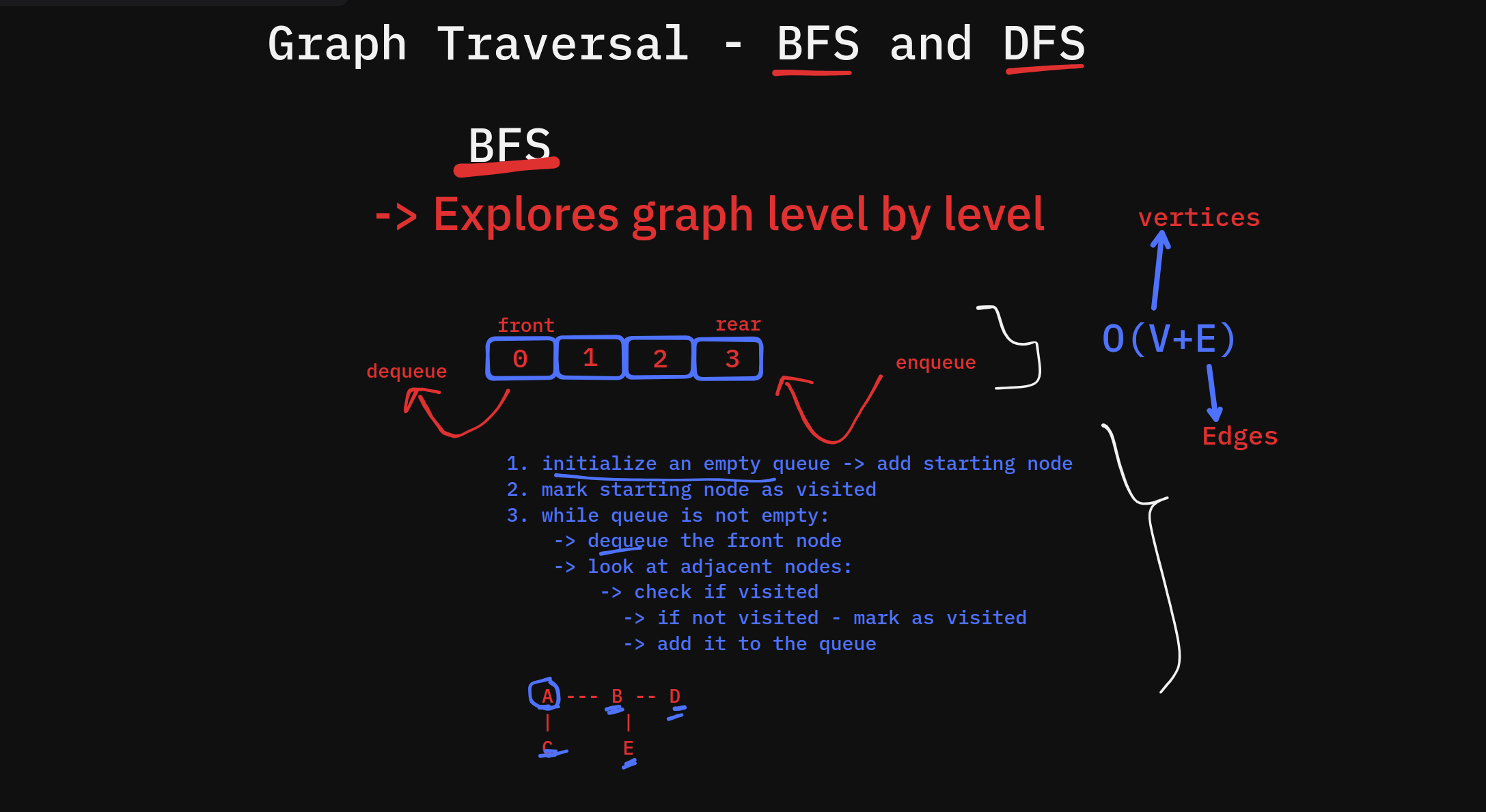
Graph traversal is a fundamental concept in computer science, enabling the exploration of nodes and edges in a graph structure. Graphs are versatile data structures that model various relationships and connections in the real world, making traversal algorithms essential for numerous applications, such as search engines, social networks, and route planning systems. In this article, we'll delve into two primary graph traversal techniques: Breadth-First Search (BFS) and Depth-First Search (DFS). We'll discuss their differences, implementations, time complexities, real-world applications, and when to use each method.
Understanding BFS and DFS
Breadth-First Search (BFS)
BFS is a graph traversal algorithm that explores nodes level by level. It starts from a given node and explores all its neighbors before moving to the next level of nodes. BFS is particularly useful for finding the shortest path in an unweighted graph and is widely employed in network routing and social networking applications.
Depth-First Search (DFS)
In contrast, DFS explores as far as possible along one branch before backtracking. It can be implemented using recursion or a stack. DFS is well-suited for tasks that require exploring all possible paths, such as cycle detection and topological sorting.
Algorithm Walkthrough
Breadth-First Search (BFS)
Initialization: Start with a queue and mark the starting node as visited.
Traversal: While the queue is not empty:
Dequeue a node and process it.
Enqueue all unvisited neighbors, marking them as visited.
Depth-First Search (DFS)
Initialization: Start with a stack (or recursive function) and mark the starting node as visited.
Traversal: While there are nodes to explore:
- Pop a node, process it, and push all unvisited neighbors onto the stack (or call the function recursively).
BFS Code Implementation
Python
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
while queue:
node = queue.popleft()
if node not in visited:
print(node, end=" ")
visited.add(node)
queue.extend(graph[node])
# Example graph
graph = {
'A': ['B', 'C'],
'B': ['D'],
'C': ['E'],
'D': [],
'E': []
}
bfs(graph, 'A') # Output: A B C D E
JavaScript
function bfs(graph, start) {
let visited = new Set();
let queue = [start];
while (queue.length > 0) {
let node = queue.shift();
if (!visited.has(node)) {
console.log(node);
visited.add(node);
queue.push(...graph[node]);
}
}
}
// Example graph
const graph = {
'A': ['B', 'C'],
'B': ['D'],
'C': ['E'],
'D': [],
'E': []
};
bfs(graph, 'A'); // Output: A B C D E
DFS Code Implementation
Python
def dfs(graph, node, visited=None):
if visited is None:
visited = set()
if node not in visited:
print(node, end=" ")
visited.add(node)
for neighbor in graph[node]:
dfs(graph, neighbor, visited)
# Example graph
graph = {
'A': ['B', 'C'],
'B': ['D'],
'C': ['E'],
'D': [],
'E': []
}
dfs(graph, 'A') # Output: A B D C E
JavaScript
function dfs(graph, node, visited = new Set()) {
if (!visited.has(node)) {
console.log(node);
visited.add(node);
graph[node].forEach(neighbor => dfs(graph, neighbor, visited));
}
}
// Example graph
const graph = {
'A': ['B', 'C'],
'B': ['D'],
'C': ['E'],
'D': [],
'E': []
};
dfs(graph, 'A'); // Output: A B D C E
Time and Space Complexity
Both BFS and DFS have a time complexity of O(V + E), where V is the number of vertices and E is the number of edges. The space complexity is as follows:
BFS: O(V) for storing the queue and visited nodes.
DFS: O(V) for storing the visited nodes, with additional space for the call stack (recursion) or explicit stack (iterative).
Real-World Applications
BFS:
Finding the shortest path in unweighted graphs (e.g., Google Maps).
Web crawling, where each page links to other pages.
DFS:
Cycle detection in graphs.
Solving mazes and puzzles.
Topological sorting in scheduling tasks.
When to Use BFS vs. DFS
BFS: Best used when the shortest path is needed, or when exploring nodes closest to the source first is beneficial.
DFS: Ideal for scenarios requiring exploration of all possible paths, such as pathfinding in complex scenarios or checking for graph connectivity.
Conclusion
BFS and DFS are fundamental algorithms in graph theory, each with unique strengths and applications. Understanding these traversal methods is crucial for solving a wide range of problems in computer science and software development. Practice implementing both algorithms with various datasets to solidify your understanding.
Call to Action
Now that you have a solid foundation in graph traversal algorithms, try implementing both BFS and DFS on your own. Explore more advanced topics like Dijkstra’s Algorithm or A Search* to deepen your knowledge of graph algorithms. If you have any questions or feedback, feel free to leave them in the comments!
Subscribe to my newsletter
Read articles from Bonaventure Ogeto directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
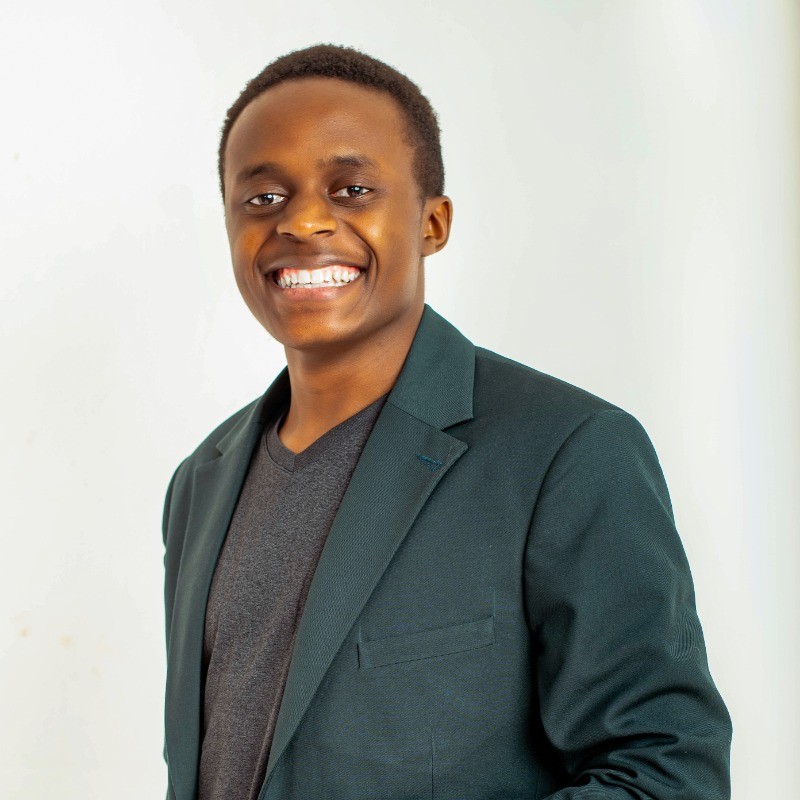
Bonaventure Ogeto
Bonaventure Ogeto
Software Engineer & Technical Writer