Basics of Python for DevOps Engineers ๐๐

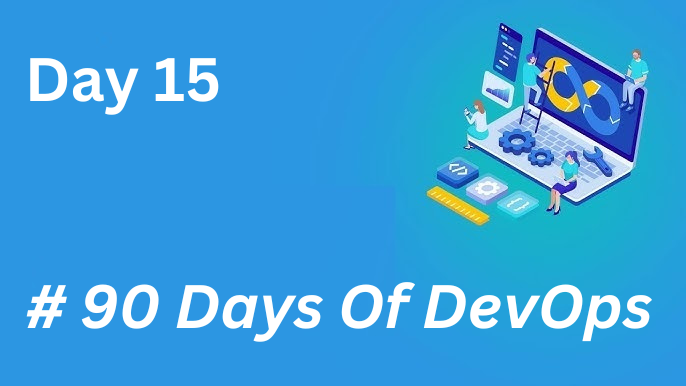
Python has become a vital tool for DevOps engineers, offering simplicity and powerful features for automation, scripting, and more. In this blog, we will cover the initial steps to get you started with Python!
Task 1: Install Python and Check Version ๐ ๏ธ
Installing Python ๐ฅ๏ธ
Windows:
Download the installer from the official Python website.
Run the installer and make sure to check the box that says "Add Python to PATH."
Follow the installation prompts.
macOS:
Open the terminal and use Homebrew:
brew install python
Linux:
Use your package manager. For example, on Ubuntu:
sudo apt update sudo apt install python3
Check Python Version ๐
To verify the installation, open your terminal (or command prompt) and run:
python --version # For Python 2.x
or
python3 --version # For Python 3.x
You should see output like:
Python 3.x.x
Understanding Data Types in Python ๐
Python supports various data types, which are essential for storing and manipulating data. Here are some of the most common ones:
Integers (
int
): Whole numbers, e.g.,5
,-10
,42
Floating-Point Numbers (
float
): Decimal numbers, e.g.,3.14
,-0.001
Strings (
str
): Sequences of characters, e.g.,"Hello, World!"
,'Python is awesome!'
Lists: Ordered, mutable collections of items, e.g.,
[1, 2, 3, 'Python']
Tuples: Ordered, immutable collections of items, e.g.,
(1, 2, 3, 'Python')
Dictionaries (
dict
): Unordered collections of key-value pairs, e.g.,{'name': 'Alice', 'age': 30}
Booleans (
bool
): RepresentsTrue
orFalse
Example of Data Types in Action ๐
Here's a quick example to illustrate these data types:
# Integer
age = 25
# Float
temperature = 36.6
# String
greeting = "Hello, DevOps!"
# List
fruits = ["apple", "banana", "cherry"]
# Tuple
coordinates = (10.0, 20.0)
# Dictionary
person = {
"name": "Alice",
"age": 30
}
# Boolean
is_devops_engineer = True
Conclusion ๐
In this blog, we've covered the basics of installing Python and understanding its fundamental data types. Python is a versatile language that will significantly enhance your DevOps toolkit. Stay tuned for more tips and tricks on leveraging Python in your DevOps journey!
Subscribe to my newsletter
Read articles from Ramiz Takildar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
