Basic Docker Workflow: Build and Run Your First Container

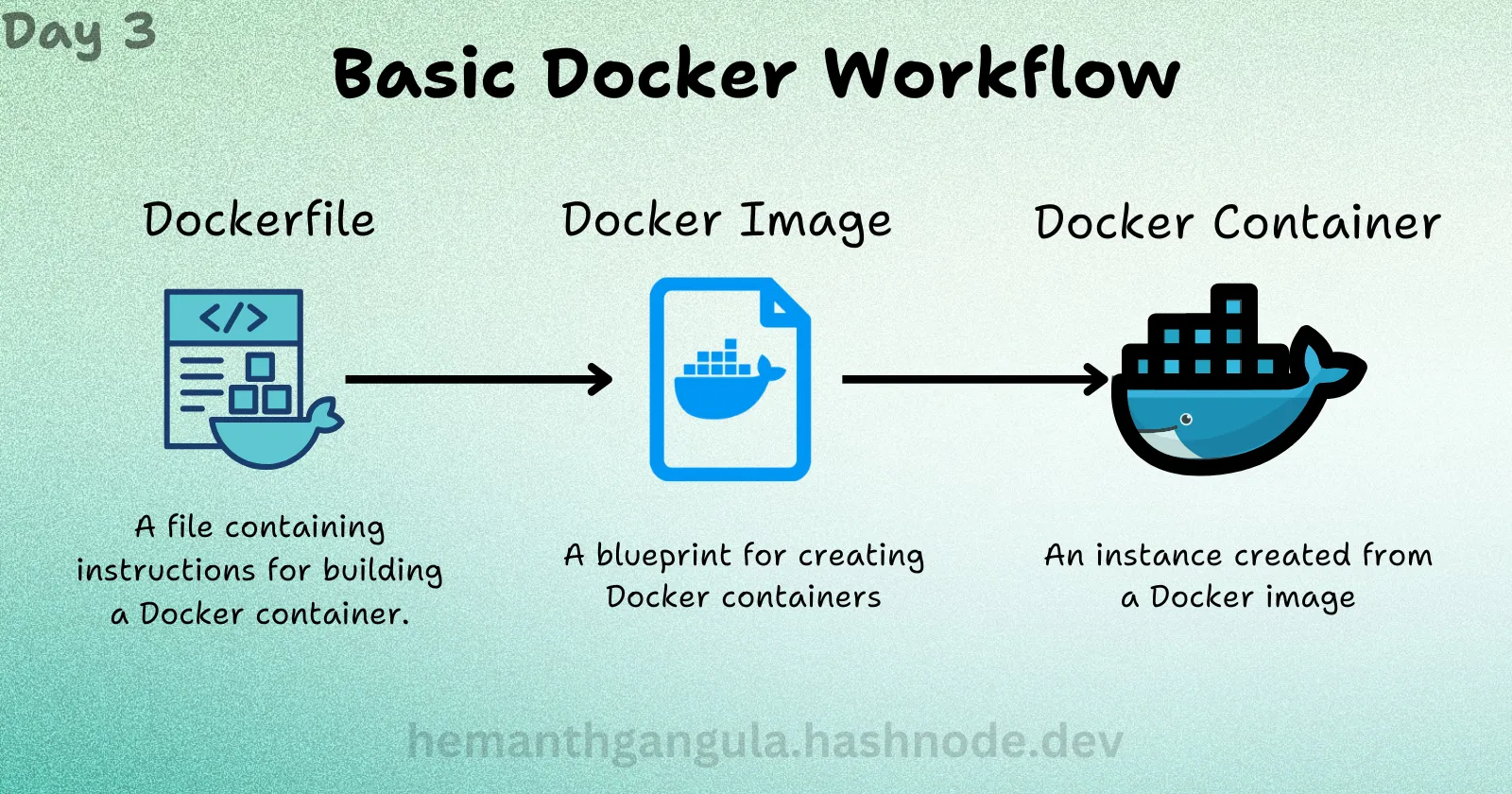
Get ready to explore Docker! In this article, we'll build a simple Docker image and run it from scratch. We'll cover key concepts like Docker files, images, and containers, equipping you with essential commands. Plus, don't miss the hands-on demo to see it all in action!
Welcome to Day 3! In our previous article, we covered the setup of Docker and Docker Compose. If you missed it, make sure to check it out!
To understand Docker's workflow, think of it like this:
Dockerfile → Docker Image → Docker Container
Before we dive into the practical example, let's take a moment to understand the key concepts. Don't rush ahead and risk missing important details. We'll explore Docker concepts thoroughly before jumping into our hands-on demonstration!
Dockerfile:
Think of a Dockerfile as your personal recipe book for creating Docker images. It’s a text document named simply Dockerfile
without any extension—that contains all the instructions needed to assemble an image that packages your application and its dependencies. This is where the magic begins!
Basic structure of a Dockerfile:
FROM: This is your starting point. It specifies the base image you want to use (for example,
FROM ubuntu:latest
).COPY: Here’s where you bring your application files into the image (e.g.,
COPY . /app
).RUN: This command allows you to execute any necessary setup commands while building your image (like installing packages with
RUN npm install
).CMD: Finally, you define what happens when someone runs your container (e.g.,
CMD ["node", "app.js"]
).
Understanding how to craft a Dockerfile is essential. It ensures that your app behaves consistently, whether it's running on your local machine or a cloud server.
Docker Image:
Now that you have your Dockerfile, it’s time to create a Docker image! This image is like a blueprint of your application, capturing everything it needs to run: the code, runtime, libraries, and dependencies. The best part? Docker images are immutable, meaning they don’t change after they’re built.
How to create an image:
Write your Dockerfile with all the necessary instructions.
Build the image using the command:
docker build -t my-image-name .
This command compiles your Dockerfile into an image, assigning it the name 'my-image-name.' The dot (.) at the end is crucial as it specifies that the Dockerfile is located in the current directory.
You can also pull images from Docker Hub, which is like an app store for Docker images. For instance, if you want to use the latest version of Node.js, just run:
docker pull node:latest
Docker Container:
Once you have your image, it’s time to create a container! A Docker container is a running instance of your image, essentially your application in action. It includes everything needed to run your software in a lightweight, isolated environment.
Basic commands for managing containers:
Run a container: To start a container from your image, you can use:
docker run -d -p 3000:3000 my-image-name
This command runs your container in detached mode (
-d
), mapping port 3000 of the container to port 3000 on your host.List running containers: Want to see what’s active? Use:
docker ps
Stop a container: When you’re done, you can easily stop a running container with:
docker stop [container_id]
Just replace
[container_id]
with the actual ID or name of your container.
Small Demonstration: Dockerizing a Simple Flask App
Now that we’ve covered the Docker basics, let’s put them into action by containerizing a simple Flask application. I’ve made a GitHub repository for this entire series, and today’s files are available in the Day-3 folder.
Start by cloning the repository:
git clone https://github.com/HemanthGangula/docker-simplified-series.git
After cloning, navigate to the Day-3 folder:
cd Day-3
Here, you’ll find today’s demo files. Use ls
or open the folder in your favourite code editor (VS Code, for example) to check the files.
We have a simple Flask application here. Our goal is to containerize this application.
Running the Flask App Manually (Before Containerization)
Before we jump into Docker, let’s run the Flask app manually to understand how it works. Ensure that Python 3 is installed on your system.
First, install the required dependencies using:
pip install -r requirements.txt
Then, run the application:
python3 app.py
The application should now be running on port 5000. Open your browser and visit localhost:5000
to see the app in action.
Great! Now that you know how the app works, let’s move on to containerizing it.
Step 1: Writing the Dockerfile
As you know, the first step in containerizing an app is writing a Dockerfile. Lucky for you, I’ve already written it! Let’s break it down:
FROM python:3.9-slim
WORKDIR /app
COPY . /app
RUN pip install -r requirements.txt
EXPOSE 5000
CMD ["python", "app.py"]
FROM python:3.9-slim: This is our base image, which acts like an operating system inside the container. We’re using a lightweight Python image here, but you could use other base images like Ubuntu, etc.
WORKDIR /app: This creates a working directory named
app
inside the container.COPY . /app: This copies the contents of your current local directory (where your Flask app is) into the
/app
directory inside the container.RUN pip install -r requirements.txt: This installs all the dependencies listed in
requirements.txt
—just like we did manually.EXPOSE 5000: This opens port 5000 in the container, allowing external access (same as when we manually ran the app).
CMD ["python", "app.py"]: This is the command that runs the Flask application inside the container.
Step 2: Building the Docker Image
With our Dockerfile ready, the next step is to build the image. Run the following command:
docker build -t flask-app-demo .
This command compiles your Dockerfile into a Docker image and tags it as flask-app-demo
. Want to check your image? Simply run:
docker images
You’ll see your image listed there!
Running the Docker Container
Now it’s time to run your Flask app inside a Docker container. Use this command:
docker run -d -p 5000:5000 flask-app-demo
-d: Runs the container in detached mode (in the background).
-p 5000:5000: Maps port 5000 on your machine to port 5000 inside the container.
The application should be running now! Open your browser and visit localhost:5000
again, and voila, your app is running inside a Docker container!
Managing Your Container
To check if the container is running, open a terminal and run:
docker ps
You’ll see the container details listed. When you’re done, don’t forget to stop the container:
docker stop <container_name>
That’s it for today’s demo!
Note: If anything feels unclear, don’t worry! I’ll provide an overview of the basic concepts and commands, and we’ll dive deeper into each topic in separate articles. So, be patient and practice.
Feel free to comment down below if you have any questions, and don’t forget to subscribe so you don’t miss the next part of this series. Stay tuned for more Docker goodness!
“Patience is the companion of wisdom.” — St. Augustine
Subscribe to my newsletter
Read articles from Hemanth Gangula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Hemanth Gangula
Hemanth Gangula
🚀 Passionate about cloud and DevOps, I'm a technical writer at Hasnode, dedicated to crafting insightful blogs on cutting-edge topics in cloud computing and DevOps methodologies. Actively seeking opportunities in the DevOps domain, I bring a blend of expertise in AWS, Docker, CI/CD pipelines, and Kubernetes, coupled with a knack for automation and innovation. With a strong foundation in shell scripting and GitHub collaboration, I aspire to contribute effectively to forward-thinking teams, revolutionizing development pipelines with my skills and drive for excellence. #DevOps #AWS #Docker #CI/CD #Kubernetes #CloudComputing #TechnicalWriter