Declarative UI Power: How SwiftUI, Compose, and Flutter Handle Time-Sensitive Tasks

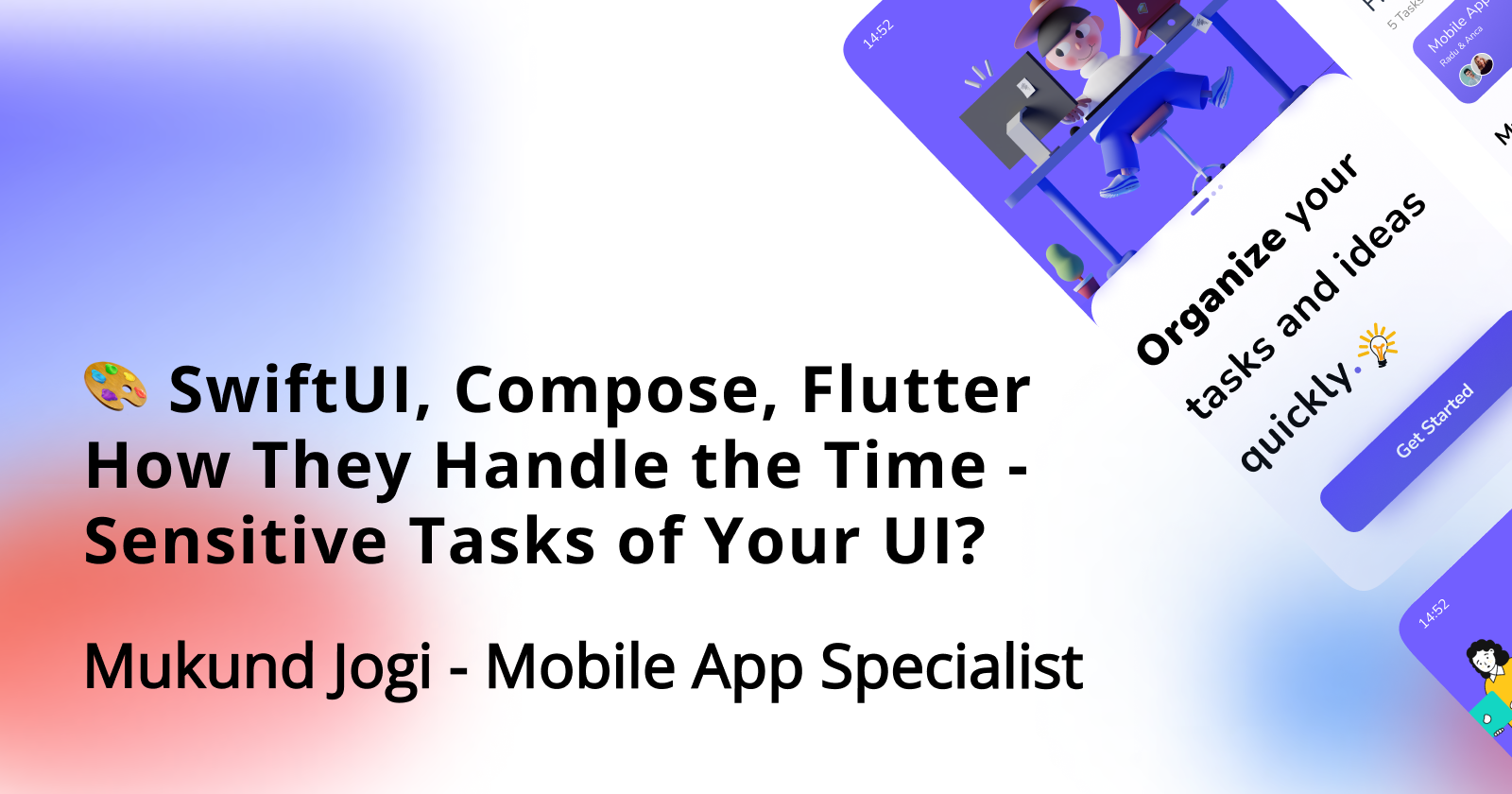
Hey, imagine you're building a house. With a declarative approach, you don't tell the builders to "hammer a nail here, then put a brick there." Instead, you give them a blueprint. "Here's how the house should look," you say. The builders figure out how to get it done.
That's the gist of declarative UI frameworks like SwiftUI, Compose, and Flutter. You describe what your UI should look like, and the framework handles the heavy lifting of rendering it on the screen.
Now, let's talk about sync and async in this context. Think of it like getting ingredients for your house-building project.
Synchronous:
Imagine going to the store, picking up all the materials at once, and coming back to start building. It's a straight-forward process.
In UI frameworks, synchronous operations happen immediately. If you tell the framework to update a text field, it happens right then and there. No waiting involved.
Asynchronous:
Now imagine you need a special type of brick that needs to be custom-made. You order it, and the builder tells you, "I'll let you know when it's ready." You have to wait for the brick, but you can still start working on other parts of the house while you wait.
This is like asynchronous tasks in UI frameworks. Fetching data from a network, loading images, or even complex calculations โ they take time. So, the framework allows you to keep working on the UI while these tasks happen in the background.
Why is this important?
Imagine you're browsing a shopping app. You tap on a product, and the app needs to fetch details like the product description, price, and reviews. If this happens synchronously, your app freezes while it waits for the data. The user sees a blank screen, which is a terrible experience!
Asynchronous tasks save the day. The app can display a loading indicator, show the basic product information it already has, and fetch the rest of the data in the background. When the data arrives, it seamlessly updates the UI without interrupting the user.
Let's see some examples:
SwiftUI
// Synchronous:
Text("Hello, World!") // This will be rendered immediately
// Asynchronous (using a Task):
Task {
let data = await fetchData() // Fetch data from network
Text("Data: \(data)") // Update the text when data is ready
}
Compose
// Synchronous:
Text("Hello, World!") // Rendered right away
// Asynchronous (using a Coroutine):
LaunchedEffect(key1 = Unit) {
val data = fetchData() // Fetch data from a network
Text("Data: $data") // Update text after fetching data
}
Flutter
// Synchronous:
Text("Hello, World!") // Rendered immediately
// Asynchronous (using async/await):
FutureBuilder<String>(
future: fetchData(),
builder: (context, snapshot) {
if (snapshot.hasData) {
return Text("Data: ${snapshot.data}");
} else if (snapshot.hasError) {
return Text('Error: ${snapshot.error}');
} else {
return CircularProgressIndicator();
}
},
);
Key takeaways:
Declarative UI frameworks let you focus on what your UI should look like, not how to achieve it.
Synchronous tasks happen instantly, while asynchronous tasks can take time.
Managing async tasks in UI frameworks is crucial for smooth and responsive user experiences.
Think of it like building a house. You describe what you want, and the framework figures out the details, synchronously or asynchronously, to deliver a polished and functional home!
Extra Tips:
Avoid blocking the UI thread with long-running operations. Use asynchronous tasks to keep your app responsive.
Think about the user experience. Provide appropriate loading indicators and error messages to keep the user informed while async tasks are running.
Use built-in tools and libraries for managing async tasks. Each framework offers ways to handle asynchronous operations efficiently.
By understanding the dance between synchronous and asynchronous tasks, you can create smooth and user-friendly apps with declarative UI frameworks. Remember, the goal is to deliver a seamless experience, even when dealing with tasks that take time!
๐๐ Let me know if you see any areas where I can make this explanation even better! I'm always up for suggestions and improvements. ๐๐
Clap-clap-clap... clap-clap-clap... Keep clapping until you hit 10! ๐
Want to take your Flutter knowledge to the next level? Check out my recent series on Flutter interview questions for all levels. Get ready to impress in your next interview!
Let's keep sharing, learning, and practicing! ๐ค๐ป
Subscribe to my newsletter
Read articles from Mukund Jogi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mukund Jogi
Mukund Jogi
๐ Iโm from: Ahmedabad, Gujarat, India ๐ Designation: Project Manager (at 7Span) ๐ป Tech Stack: Flutter, Android , iOS ๐ง Let's connect: โฃ LinkedIn: https://linkedin.com/in/mukund-a-jogi/ โฃ Twitter: https://twitter.com/mukundjogi โฃ Medium: https://medium.com/@mukundjogi