"Variables ,Comments and I/O in Pythonđ"

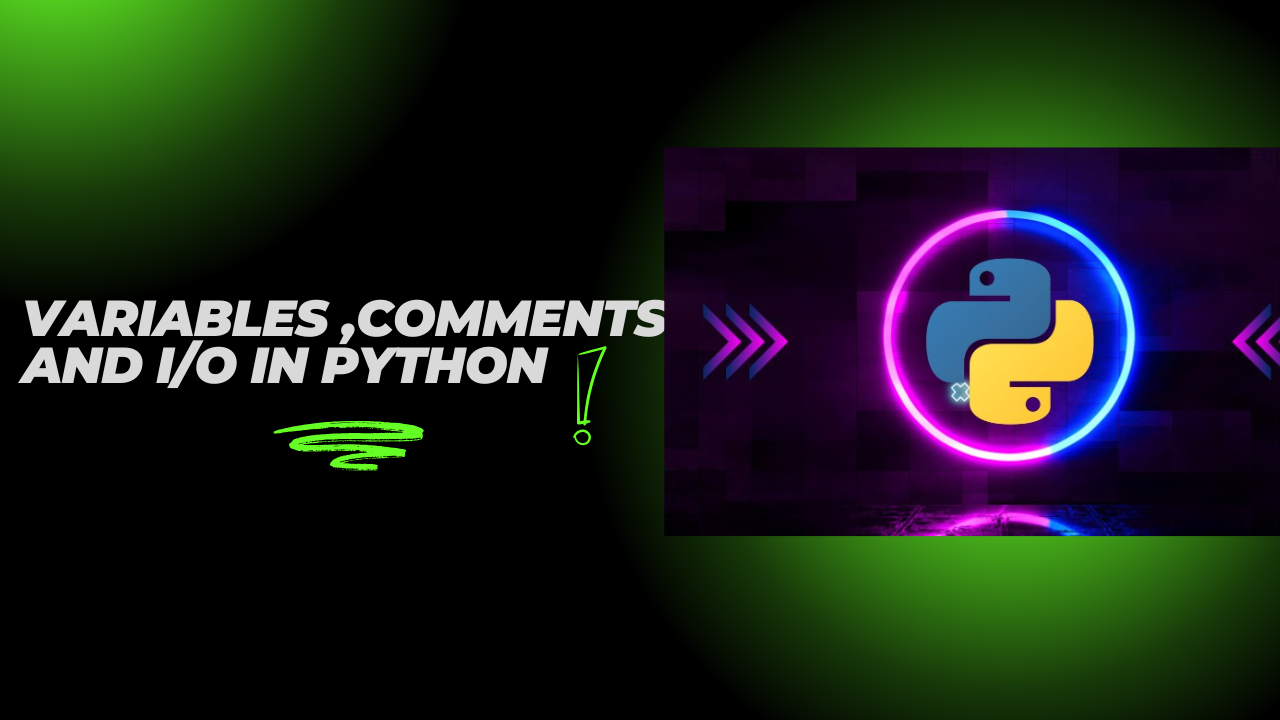
Variables:
A variable is a reference or name that points to an object in memory. Variables themselves donât hold the actual data but they point to objects that holds data. In Python, when you assign a variable, it just holds a reference to an object.
Rules for naming variables:
Allowed Characters: Letters (a-z, A-Z), digits (0-9), and underscores (_).
Variable names canât start with a number.
Case Sensitive: var, Var, and VAR are different variables.
No Reserved Keywords: Python keywords (like for, if, while, etc.) cannot be used as variable names
# Valid variable names
my_name = "unknown"
_age = 25
# Invalid variable names
2name = "rocky" # Starts with a number
for = "hello" # 'for' is a reserved keyword
Variable Scope:
Scope defines where a variable can be accessed in the code
Global Variable:
Defined outside any function and can be accessed anywhere in the program.
Local Variable:
Defined inside a function and only accessible within that function.
# Global variable
x = 100
def example_function():
# Local variable
y = 500
print("Inside function, y:", y) #Inside function, y:500
print("Inside function, x (global):", x) # Can access global x
example_function()
print("Outside function, x:", x) #Outside function, x:100
print(y) # Error! y is not accessible outside the function
Modifying Global Variables:
# Modifying Global Variables:
# To modify a global variable inside a function, you need to use the global keyword.
x = 10 # Global variable
def modify_global():
global x
x = 20 # Modify the global variable
modify_global()
print(x) # Output: 20
Multiple Assignment:
Python allows you to assign multiple variables at once. This can be useful when you need to assign multiple values or swap values between variables.
# Multiple variables assigned at once
a, b, c = 1, 2, 3
print(a, b, c) # Output: 1 2 3
a=b=c=12
print(a, b, c) # Output: 12 12 12
# Swapping variables
x, y = 10, 20
x, y = y, x # Swap x and y
print(x, y) # Output: 20 10
Unpacking Variables:
You can unpack values from sequences (such as lists or tuples) into variables
# Unpacking a list
numbers = [1, 2, 3]
a, b, c = numbers
print(a, b, c) # Output: 1 2 3
# Unpacking a tuple
var = (10, 20)
x, y = var
print(x, y) # Output: 10 20
Comments:
Not executed ,used for understanding or documenting the code
# single line comments
print(3+90) #adding two numbers
'''
multiple line
comments
'''
Input /Output :
input:
input( ) function is used to take i/p from user
output:
print( ) function is used to display output
name = input("enter your name:") #by default input is taken as string
age = int(input("enter age:")) #input data is integer
weight = float(input("weight:")) #input data is decimal values
print(name)
print(type(name)) #string type data
print(age)
print(type(age)) #integer type data
print(weight)
print(type(weight)) #float type data
Subscribe to my newsletter
Read articles from Sandeep directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
