Mastering the Request-Response Cycle in Express.js

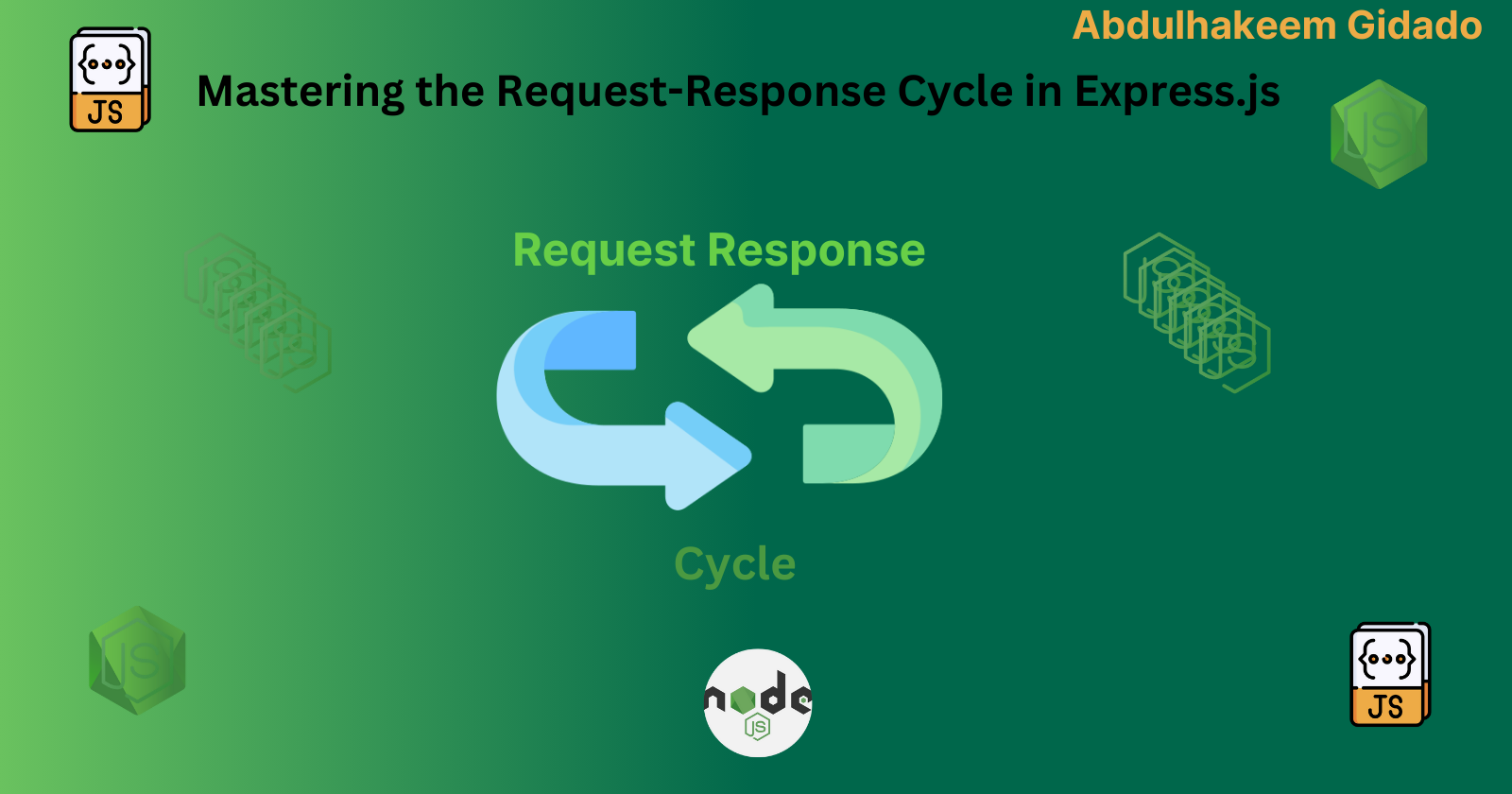
In modern web development, especially in Node.js environments, Express.js stands as one of the most popular frameworks for handling HTTP requests and responses. However, beneath its simplicity lies a sophisticated mechanism that many developers overlook: middleware. Middleware is the unsung hero in the request-response cycle, orchestrating each stage of data flow and logic between client and server.
In this article, we’ll explore the request-response cycle in Express.js, from receiving a request to sending a response, while demystifying the role of middleware and its importance. You’ll discover that understanding middleware is not only key to mastering Express, but it’s also a gateway to efficient and scalable development.
Table of Contents
What Is the Request-Response Cycle?
Middleware: The Backbone of Express
Body Parsing: Middleware That Unpacks the Data
Logging Middleware: Keeping Track of Every Move
Setting Headers: Controlling the Response at Every Stage
Routing: Directing the Traffic
Handling Errors: The Final Middleware
Conclusion: Middleware Is the Magic in Express Development
What Is the Request-Response Cycle?
At its core, the request-response cycle is the process by which a client (e.g., a browser or mobile app) sends a request to a server, and the server processes that request and sends back a response. This flow can be visualized as:
- Client Request → 2. Server Processing → 3. Server Response
In Express, middleware plays a central role in server processing, enabling you to manipulate the request before it reaches the final destination (the route handler) and modify the response before sending it back to the client.
Middleware: The Backbone of Express
Middleware functions are at the heart of Express. They are functions that have access to the request object (req
), response object (res
), and the next middleware in the application’s stack. Middleware can perform various tasks such as:
Modifying the request or response objects
Ending the request-response cycle
Passing control to the next middleware function
An Express app can use multiple middleware functions that are executed in sequence, and this sequence forms the middleware stack. Here’s a basic middleware setup:
app.use((req, res, next) =>{
console.log('A new request received at '+ Date.now());
next();
// Pass control to the next middleware
});
This middleware logs the request timestamp and passes control to the next middleware. next()
is the crucial function that developers often misunderstand. It’s used to move to the next piece of middleware or route handler.
Middleware can short-circuit the request-response cycle by either terminating it early (e.g., res.send()
) or diverting it (e.g., through error handling). Mastery of this concept enables more efficient code flow.
Body Parsing: Middleware That Unpacks the Data
When a client sends data (such as form submissions or JSON payloads), Express doesn’t automatically understand that data. Body parsing middleware is used to decode incoming data, so the server can make sense of it.
Example with express.json()
middleware:
const express = require('express');
const app = express();
// Middleware to parse JSON data from the request body
app.use(express.json());
app.post('/data', (req, res) => {
console.log(req.body);
// Now you can access the parsed body data
res.send('Data received');
});
Many developers are unaware of the performance implications of body parsing middleware. Without proper configuration (e.g., setting size limits), large payloads can slow down or crash your application.
// Limit request body to 10KB
app.use(express.json({ limit: '10kb' }));
This improves security and performance, especially for APIs handling large uploads.
Logging Middleware: Keeping Track of Every Move
Logging middleware provides a mechanism to track every request and response, which is critical for monitoring, debugging, and performance analysis.
Example using the popular morgan logging middleware:
const morgan = require('morgan');
// Logs every request in the console
app.use(morgan('dev'));
Morgan logs details like HTTP method, URL, status code, and response time. This information is invaluable for detecting bottlenecks or malicious activity.
Instead of logging every request, advanced logging setups can log requests based on conditions, such as response time thresholds or error status codes.
Setting Headers: Controlling the Response at Every Stage
Headers are metadata sent along with the response to define how the client should interpret the data or control caching behavior. Headers can be set at multiple stages during the request-response cycle.
Example:
javascriptCopy codeapp.use((req, res, next) => {
res.setHeader('X-Powered-By', 'Focus-Express-Server');
next();
});
Headers can include security headers (e.g., to prevent XSS attacks), caching headers, or content type headers.
You can dynamically set headers based on request properties (e.g., user agent, authentication status) or even intercept and modify headers from upstream servers in more complex architectures.
Routing: Directing the Traffic
Once middleware processes the request, it is finally routed to the appropriate handler based on the URL and HTTP method.
app.get('/user/:id', (req, res) => {
res.send(`User ID: ${req.params.id}`);
});
Routers in Express can be modular and layered with middleware to ensure smooth navigation between various routes.
Routers can be mounted to specific paths, which lets you keep your code modular and manageable. You can also apply middleware to specific routers, allowing advanced logic to control which routes are accessible under certain conditions.
const userRouter = express.Router();
userRouter.use((req, res, next) => {
console.log('Middleware for /users routes');
next();
});
app.use('/users', userRouter);
Handling Errors: The Final Middleware
Error handling is another key piece of the request-response cycle. In Express, any error passed to next()
can be caught by an error-handling middleware.
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something went wrong!');
});
By centralizing error handling in middleware, you ensure consistent responses for your users and avoid code duplication.
Many developers forget that asynchronous errors must be passed to next()
, otherwise they won’t trigger the error-handling middleware:
app.get('/', async (req, res, next) => {
try {
const data = await someAsyncOperation();
res.send(data);
} catch (err) {
// Pass error to the centralized error handler
next(err);
}
});
Conclusion: Middleware Is the Magic in Express Development
Express is lightweight by design, and middleware is its core strength. Understanding how middleware manipulates the request-response cycle allows you to:
Secure your application more effectively
Improve performance and resource usage
Create modular and maintainable code
Most developers use middleware without fully realizing its potential. You need to go beyond basic implementations and leverage middleware for advanced data processing, performance optimizations, and security enhancements.
Middleware is more than just code between requests and responses—it's the power behind Express’s flexibility, and mastering it is key to becoming an efficient developer.
Feel free to like, comment and pass it along to your fellow developers! ⚡️
Thanks👋
Subscribe to my newsletter
Read articles from Abdulhakeem Gidado directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
