Abort It! Streamlining Your Asynchronous JavaScript
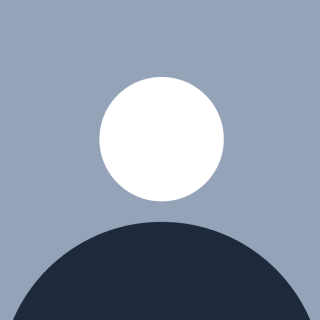
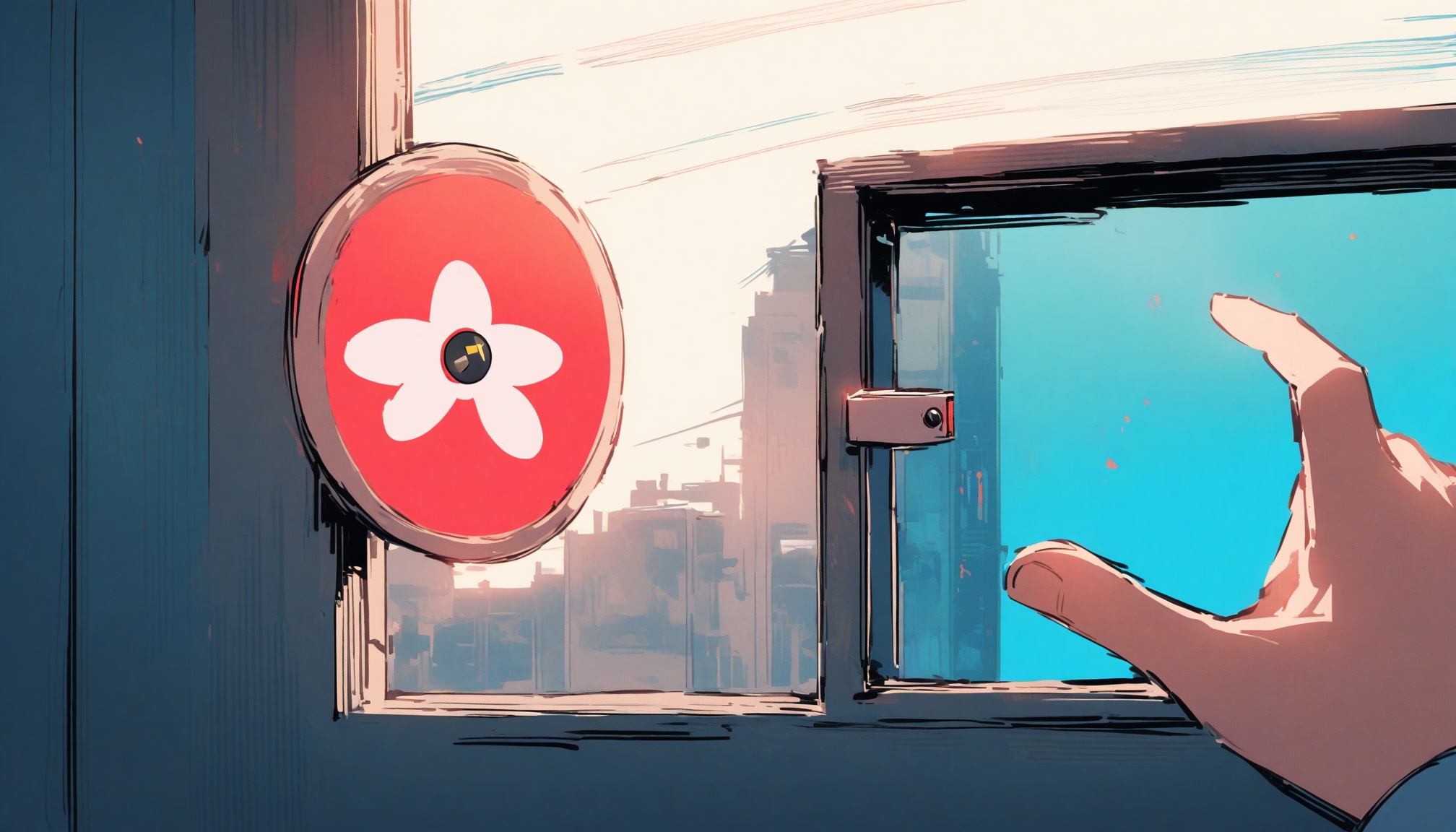
In the JavaScript ecosystem, all network and database calls are inherently asynchronous due to the non-blocking nature of JavaScript's event-driven architecture. Have you ever tried to abort asynchronous tasks? Consider scenarios like when you want to cancel:
A request if it's no longer needed (e.g., a user navigates away from a page): In web applications, users often navigate between different pages or sections. If a user moves away from a page while a network request is still in progress, continuing to process that request becomes unnecessary. Aborting such requests can save bandwidth and reduce server load.
A database query call that is taking too much time: Database queries can sometimes take longer than expected due to various reasons such as server load, complex query operations, or network latency. In situations where a query is taking an excessive amount of time, it might be beneficial to cancel the operation.
The solution to cancel an asynchronous task is AbortController
—a built-in JavaScript class that creates an object used to signal the cancellation of asynchronous operations.
I have added a few examples of how we can use AbortController
to cancel asynchronous tasks.
AbortController with Fetch
To use AbortController
with a fetch call, follow below steps:
Create a new AbortController instance:
const controller = new AbortController();
Extract the signal property from the instance:
const signal = controller.signal;
Pass the signal property to the fetch call:
fetch('your-api-endpoint', { signal }) .then(response => { // handle response }) .catch(error => { if (error.name === 'AbortError') { console.log('Fetch aborted'); } else { // handle other errors } });
Call the abort method from the AbortController instance:
controller.abort();
This setup allows you to cancel the fetch request by calling controller.abort()
, which triggers an AbortError
in the fetch promise.
AbortController with custom Promise
To use AbortController
with a custom promise, follow below steps:
Create an AbortController instance:
const controller = new AbortController();
Extract the signal property from the AbortController instance:
const signal = controller.signal;
Create the custom promise and add 'abort’ event listener inside it:
function customPrmoise(signal) { return new Promise((resolve, reject) => { // long executing async task // Listen for the abort signal signal.addEventListener('abort', () => { reject(new Error('Promise aborted')); }); }); }
Call the custom promise with the signal:
customPrmoise(signal) .then((data) => console.log(data)) .catch((error) => console.error(error.message));
Call the abort method from the AbortController instance:
controller.abort();
This setup allows you to abort the custom promise by calling controller.abort()
, which triggers the abort event listener and rejects the promise with an error.
Conclusion
In summary, AbortController
is an essential tool for managing asynchronous operations in JavaScript, allowing developers to efficiently cancel unnecessary or prolonged tasks, thereby improving application responsiveness and user experience.
For more information refer:
Subscribe to my newsletter
Read articles from Jayaram directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Jayaram
Jayaram
A self taught developer who loves to discuss about tech over coffee breaks