How to Send Ether in Solidity: A Guide to transfer, send, and call.

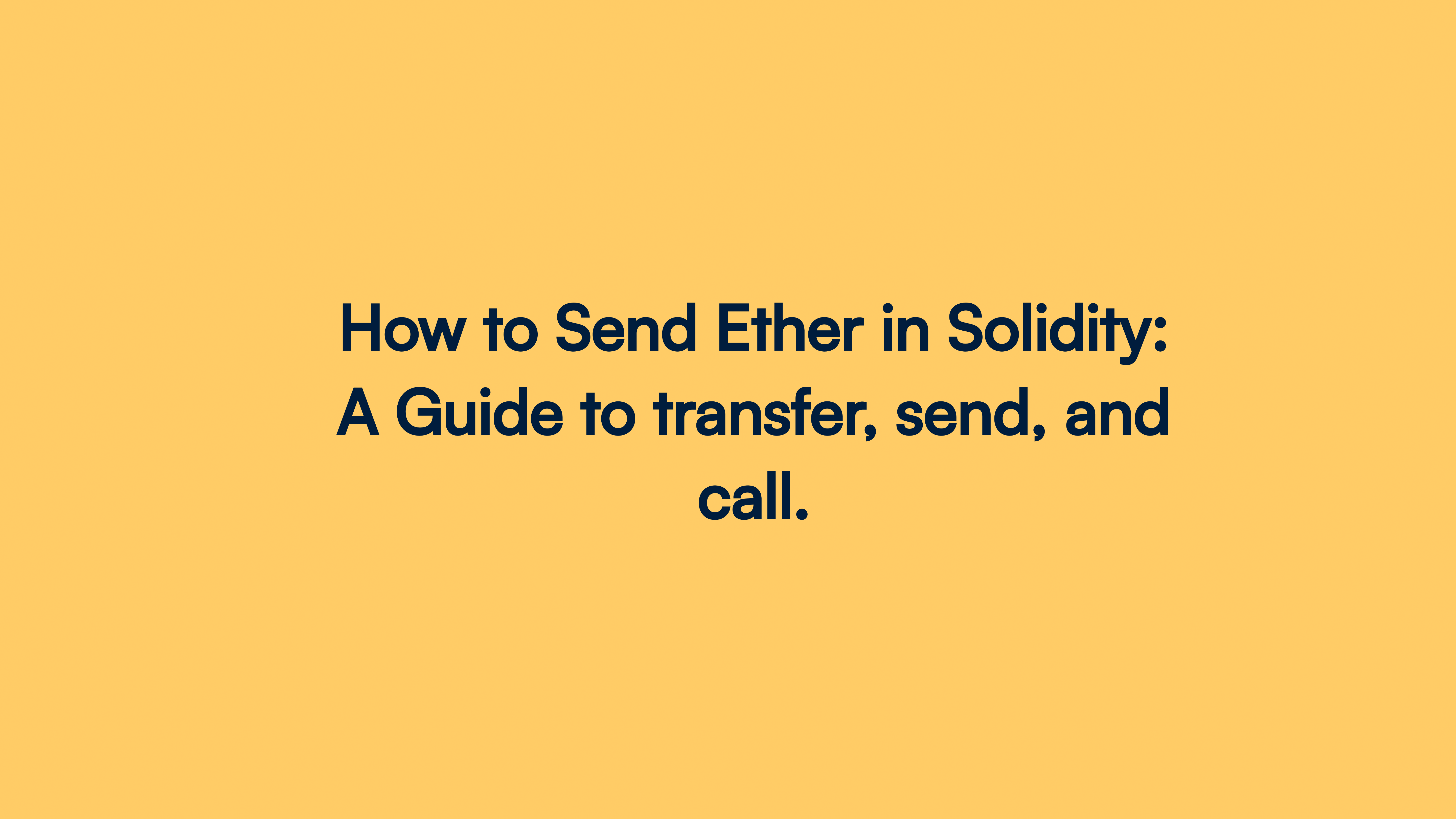
Smart contracts on Ethereum allow for programmatic transfer of ETH between accounts and contracts. Solidity which is the primary programming language for writing smart contracts, provides three ways to send Ether;
Send()
transfer()
call()
Each of these methods has its own characteristics, advantages, and potential risks.
In this article, we’ll explore each one and determine which is considered best practice in modern Ethereum development.
What is Ether (ETH)
Ether (ETH) is the native cryptocurrency of the Ethereum blockchain and it serves as both a digital currency and a means to execute smart contracts.
Ether plays a crucial role in the Ethereum ecosystem it enables transactions, powering decentralized applications (DApps), and compensating miners for validating transactions.
When working with Solidity, developers often need to transfer Ether programmatically between addresses.
Solidity provides three primary methods for sending Ether: transfer(),
send(),
and call().
Choosing the correct method is essential to ensure secure and effective transfers in your smart contracts.
The Three Methods of Sending ETH in Solidity
transfer
Thetransfer()
function is a simple way to send Ether in Solidity. It sends a fixed amount of gas (2300 gas units) to the recipient, which is just enough to allow the receiving contract to execute basic operations like logging an event or updating a variable.// 1. Using transfer function transferEther(address payable _recipient) public payable { _recipient.transfer(msg.value); // Sends 2300 gas, reverts on failure }
When you use
transfer(),
if the Ether transfer fails for any reason, the transaction will automatically revert. This means the funds are returned to the sender and the entire transaction is cancelled. There’s no need to handle errors manually.Use Case:
transfer()
is ideal for simple, safe transfers where you don’t expect any fallback logic in the recipient contract.
send The
send()
function is similar totransfer(),
but it offers more flexibility by allowing manual error handling. Liketransfer(),
send()
also imposes the 2300 gas limit on the recipient, ensuring basic operations are safe.// 2. Using send function sendEther(address payable _recipient) public payable { bool success = _recipient.send(msg.value); // Sends 2300 gas, returns false on failure require(success, "Send failed"); }
However, instead of automatically reverting the transaction when an error occurs,
send()
simply returns a boolean value (true for success, false for failure). This gives the developer more control, as they can write custom logic to handle the case where the Ether transfer fails.Use Case:
- Useful when you expect potential failure and need more control over error handling.
call
`The
call()` function is the most flexible and powerful method for transferring Ether. It allows for sending Ether and making external function calls at the same time, forwarding all available gas to the recipient by default (unless a gas limit is explicitly set).// 3. Using call (recommended) function callEther(address payable _recipient) public payable { (bool success, ) = _recipient.call{value: msg.value}(""); // Forwards all gas, returns success/failure require(success, "Call failed"); }
When you use
call()
, it returns a boolean value (indicating success or failure) and optionally a data payload. It’s most commonly used for sending Ether in complex transactions where interacting with external contracts is necessary, but the flexibility it offers comes with potential risks like a reentrancy attack if proper precautions are not taken.Use Case:
- Preferred for complex interactions where the recipient contract may require more than 2300 gas.
Comparing the three Methods
Gas Limits
transfer()
andsend()
both have a fixed gas limit of 2300 gas, which is enough for simple operations like logging an event.call()
forwards all available gas to the recipient unless a specific gas limit is provided, making it the most flexible.
Error Handling
transfer()
automatically reverts the transaction if it fails, so no manual error handling is required.send()
andcall()
return a boolean indicating success or failure, so the developer needs to handle errors manually using logic likerequire()
.
Security
transfer()
andsend()
are safer for simple transactions because the limited gas prevents reentrancy attacks.call()
is more vulnerable to exploits if not properly secured, as it forwards all gas and can be used in complex interactions.
Conclusion
While all three methods can send Ether, call()
is the recommended approach for modern Ethereum development. Its flexibility, since it is not restricted to 2300 gas, and explicit error handling make it the most robust choice.
However, when using call()
, always remember to:
Check return values.
Guard against re-entrancy attack.
Implement proper security measures.
Subscribe to my newsletter
Read articles from Zainab Wahab directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
