How to Use Grep, Awk, Sed, and Find Commands in Linux
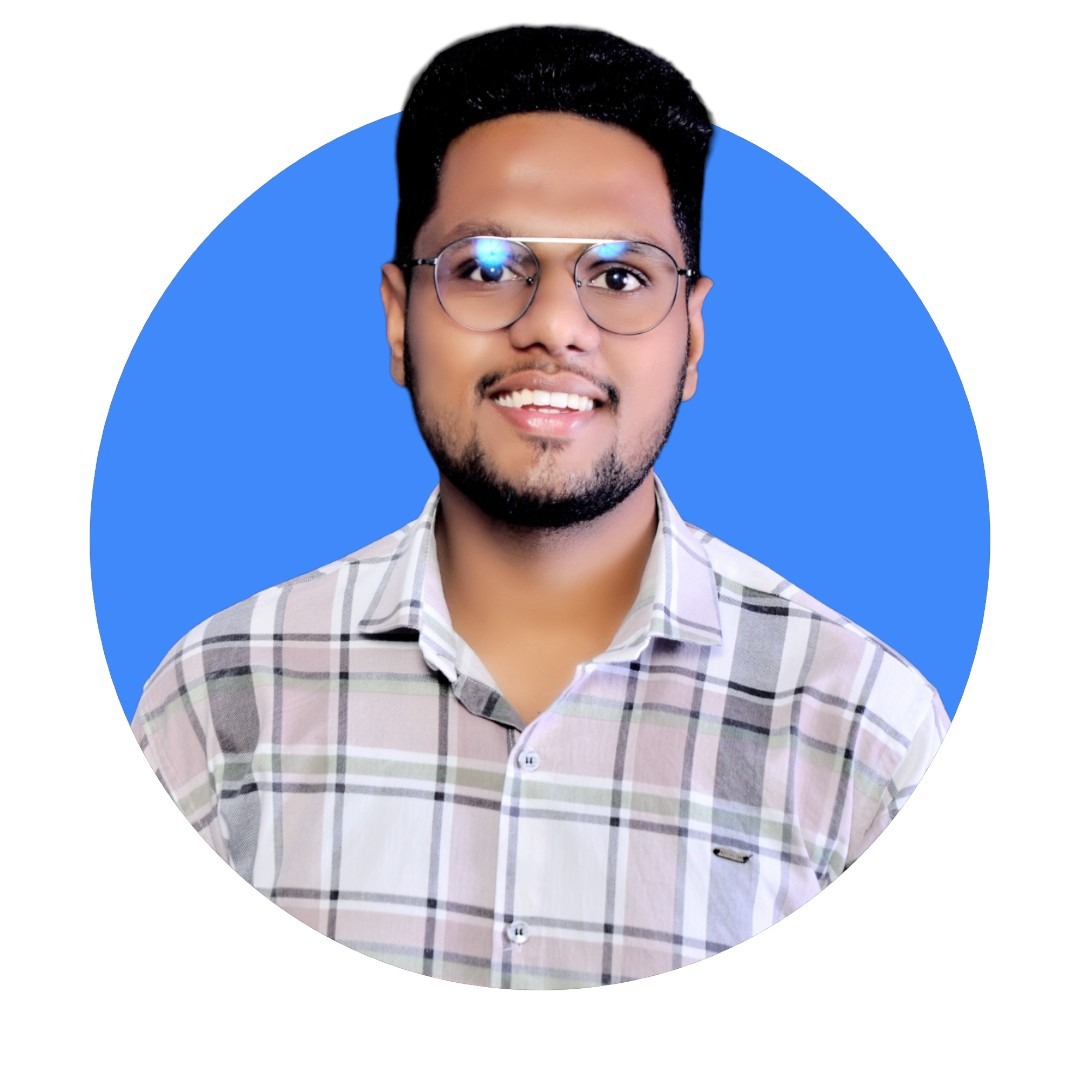
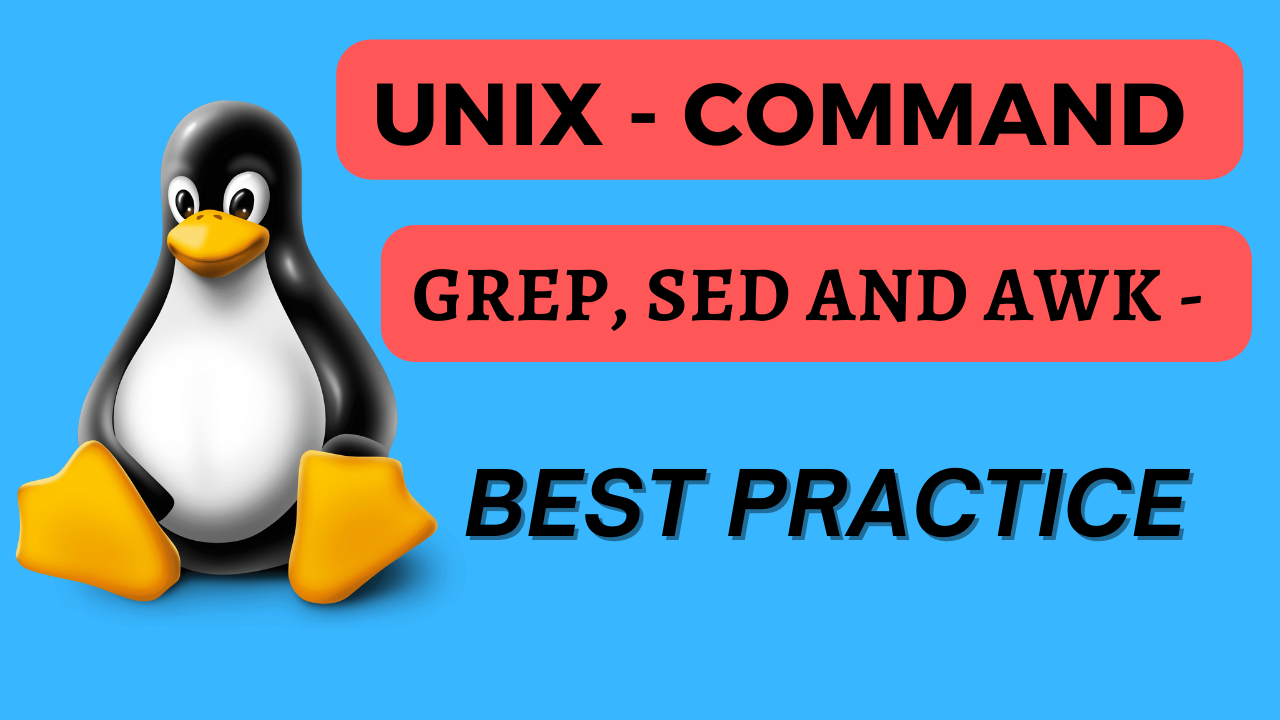
The grep command is one of the most powerful and widely used command-line tools in Unix/Linux for searching text.
It allows you to search for specific patterns within files or input streams, using regular expressions for more complex searches.
The grep command in Unix/Linux is a powerful tool used for searching and manipulating text patterns within files
Basic Syntax
grep [options] 'pattern' [file...]
pattern: The string or regular expression you want to search for.
file: One or more files to search. If no file is specified,grep reads from standard input.
Common Options
Case Sensitivity Options:
-i: Ignores case when matching.
For example, grep -i 'hello' file.txt
Matches "Hello", "HELLO", and "hello".
Inverting Match:
-v: Displays lines that do NOT match the specified pattern.
For example, grep -v 'error' logfile.txt
shows all lines without the word "error".
Line Numbering:
-n: Displays line numbers alongside matching lines.’
For example, grep -n 'pattern' file.txt
outputs matches with their respective line numbers.
Counting Matches:
-c: Counts the number of lines that match the pattern instead of displaying the matching lines.
For example, grep -c 'pattern' file.txt.
Recursive Search:
-r or -R: Searches files in the specified directory and all its subdirectories.
For example, grep -r 'pattern' /path/to/directory
/
.
File Names Only:
-l: Lists the names of files containing the matching lines instead of the lines themselves.
For example, grep -l 'pattern' *.txt.
Displaying Context:
-A N: Shows N lines after a match.
For example, grep -A 2 'pattern' file.txt
displays the matching line and the two lines that follow.
-B N: Shows N lines before a match.
For example, grep -B 2 'pattern' file.txt
displays the matching line and the two lines that precede it.
-C N: Shows N lines before and after a match
For example, grep -C 2 'pattern' file.txt.
Regular Expression Support:
-E: Enables extended regular expressions.
For example, grep -E 'pattern1|pattern2' file.txt
searches for either "pattern1" or "pattern2".
-F: Treats the pattern as a fixed string (not a regular expression)
. For example, grep -F 'pattern' file.txt.
Quiet Mode:
-q: Suppresses output; exits with 0 if a match is found, or 1 if not.
Useful in scripts. For example, grep -q 'pattern' file.txt && echo "Found!".
Displaying Only the Matching Part:
-o: Outputs only the matched parts of a line.
For example, grep -o 'pattern' file.txt
shows just the occurrences of the pattern.
Practical Examples
Basic Search:
grep 'hello' file.txt
This searches for the word "hello" in
file.txt
.Output:
Hello World Another line with Hello. Hello again!
Here, the word "hello" is case-sensitive, so it matches "Hello" exactly.
Case-Insensitive Search:
grep -i 'hello' file.txt
Output:
Hello World Another line with Hello. Hello again!
Since
-i
makes the search case-insensitive, it matches all instances of "hello" regardless of case.Search in Multiple Files:
grep 'hello' file1.txt file2.txt
Output:
file1.txt:Hello World file1.txt:Another line with Hello. file1.txt:Hello again!
The output includes filenames where matches are found. Here, the matches are only in
file1.txt
.Count Occurrences:
grep -c 'hello' file.txt
Output:
3
There are 3 lines that contain the word "hello".
Search Recursively:
grep -r 'hello' /path/to/directory/
Output (example):
/path/to/directory/file1.txt:Hello World /path/to/directory/file1.txt:Another line with Hello. /path/to/directory/file1.txt:Hello again!
It will search all files in the directory and its subdirectories for "hello".
Show Line Numbers:
grep -n 'hello' file.txt
Output:
1:Hello World 3:Another line with Hello. 7:Hello again!
The
-n
option shows the line numbers where the pattern "hello" appears.Invert Match:
grep -v 'hello' file.txt
Output:
This is a test file. There is an ERROR here. Some more text to test. This line has a warning.
Show Context Lines:
grep -C 3 'hello' file.txt
This shows 3 lines of context around each match.
Output:
Hello World This is a test file. Another line with Hello. There is an ERROR here. Some more text to test. Hello again!
The
-C 3
option displays 3 lines before and after the match for "hello".Search for Multiple Patterns:
grep -E 'error|warning' file.txt
Output:
There is an ERROR here. This line has a warning.
The
-E
option allows you to use extended regular expressions, in this case searching for either "error" or "warning".Output Only Matching Parts:
grep -o 'hello' file.txt
Output:
hello hello hello
The
-o
option displays only the matched parts of the line, so it will print "hello" for every occurrence in the file.
Summary of Advantages and Disadvantages
Advantages | Disadvantages |
Fast and efficient for searching text patterns | Limited to text searching, not processing |
Supports powerful regular expressions | Complex regular expressions can be hard to use |
Works with pipes, ideal for scripting | No graphical interface, command-line only |
Case-insensitive search with -i option | Case sensitivity by default |
Customizable output (line numbers, filenames, etc.) | Handling of large files can sometimes be slow |
Portable and available on most Unix-based systems | No native support for compressed files |
Recursive search with -r option | Not available natively on Windows |
Simple syntax for basic searches | Lack of built-in text formatting or highlighting |
AWK Command
The awk command is a powerful text-processing tool in Unix/Linux used for pattern scanning, processing, and reporting.
It operates on each line of input, allowing users to search for patterns, extract data, and perform complex operations on text.
It is especially useful when dealing with structured data, such as tables and log files.
Basic Syntax
awk 'pattern {action}' file
pattern
: The condition or pattern to match (optional).action
: The commands to execute when the pattern is matched (optional).file
: The input file to process (optional, can also read from standard input).
If no pattern is provided, awk applies the action to every line. If no action is specified, awk prints every line that matches the pattern.
How awk Works
awk processes each line of input one by one.
Each line is divided into fields (or columns), which are separated by whitespace (or a custom delimiter).
These fields can be accessed using
$1
,$2
,$3
, and so on, where:$1
: Refers to the first field.$2
: Refers to the second field, and so on.$0
: Refers to the entire line.
Basic Examples of awk
Printing All Lines:
awk '{print}' file.txt
This prints all lines from
file.txt
. The{print}
action prints the entire line ($0
).Printing Specific Fields:
awk '{print $1, $3}' file.txt
This prints the first and third fields (columns) from each line of
file.txt
. For example, iffile.txt
has:John Doe 30 Jane Smith 25
Output:
John 30 Jane 25
Printing Line Numbers with Content:
awk '{print NR, $0}' file.txt
NR
is a built-in variable that tracks the line number. This command prints the line number followed by the line content.Using a Pattern to Match Specific Lines:
awk '/error/ {print}' logfile.txt
This searches for lines containing the word "error" in
logfile.txt
and prints them.Performing Mathematical Operations:
awk '{print $1, $2*2}' file.txt
If
file.txt
contains:John 10 Jane 15
Output:
John 20 Jane 30
Field Delimiters: By default,
awk
uses whitespace to separate fields. You can change the delimiter using the-F
option:awk -F ',' '{print $1, $2}' file.csv
If
file.csv
contains:John,Doe,30 Jane,Smith,25
Output:
John Doe Jane Smith
Advantages of awk
Text Processing:
awk
is highly efficient for processing structured text, such as log files, CSV files, and other data formats.Flexible: It can handle both simple and complex text-processing tasks, like searching, replacing, and transforming text.
Patterns and Arithmetic:
awk
can match patterns and perform arithmetic operations on fields, making it a good choice for parsing structured data.Portable: Available on almost all Unix/Linux systems and can be used across different platforms.
Disadvantages of awk
Complex Syntax:
awk
has a steep learning curve for more complex tasks, especially for users unfamiliar with programming or regular expressions.Performance: For extremely large datasets or complex operations,
awk
may not be as fast as more modern tools likePython
orPerl
.Limited Debugging: Debugging
awk
scripts can be difficult because of its compact syntax and lack of native debugging tools.
Features of AWK command
Various features of the Awk command are as follows:
It scans a file line by line.
It splits a file into multiple fields.
It compares the input text or a segment of a text file.
It performs various actions on a file like searching a specified text and more.
It formats the output lines.
It performs arithmetic and string operations.
It applies the conditions and loops on output.
It transforms the files and data on a specified structure.
It produces the format reports.
Find Commands
The
find
command in Linux is used to search for files and directories in a directory hierarchy based on various criteria, such as file name, file type, permissions, modification time, size, etc.It is a powerful utility for locating files and executing commands on them.
Basic Syntax
find [path] [options] [expression]
[path]
: The directory in which to begin the search. If no path is specified,find
starts from the current directory (.
).[options]
: Flags and options that modify the behavior of thefind
command.[expression]
: The criteria or conditions for the search, such as file name, size, or permissions.
Basic Examples of find
Find a File by Name:
find /path/to/directory -name filename
This searches for a file named
filename
in the specified directory. You can use wildcards (*
) to search for partial matches.Example:
find /home/user -name "*.txt"
This finds all
.txt
files in the/home/user
directory.
Find a Directory by Name:
find /path/to/directory -type d -name dirname
The
-type d
option ensures that only directories are returned. Replacedirname
with the name of the directory you want to find.Example:
find /home/user -type d -name "projects"
This finds a directory named
projects
in/home/user
.
Find Files by Type: You can search for files based on their type using the
-type
option:f
: Regular file.d
: Directory.l
: Symbolic link.
Example:
find /path/to/directory -type f
This searches for regular files (-type f
) in the specified directory.
Find Files by Size:
find /path/to/directory -size +10M
This finds files larger than 10 megabytes in the specified directory. You can specify the size in:
c
: Bytes.k
: Kilobytes.M
: Megabytes.G
: Gigabytes.+: Greater than.
-: Less than.
=
: Exactly.
Example:
find /var/log -size -100k
This finds files smaller than 100 kilobytes in the /var/log
directory.
Find Files by Last Modified Time: You can search for files based on when they were last modified using the
-mtime
option.-mtime
allows you to search for files modified a certain number of days ago.+N
: More thanN
days ago.-N
: Less thanN
days ago.N
: ExactlyN
days ago.
Example:
find /path/to/directory -mtime -7
This finds files modified in the last 7 days.
Find Files by Permissions:
find /path/to/directory -perm 755
This finds files with the permission mode
755
(rwxr-xr-x).Find and Delete Files: You can use
find
to locate files and delete them. Be careful with this operation!find /path/to/directory -name "*.tmp" -type f -delete
This finds and deletes all files with the
.tmp
extension.Find Files and Execute a Command: The
-exec
option allows you to execute commands on the files found byfind
.find /path/to/directory -type f -name "*.log" -exec rm {} \;
This finds all
.log
files and deletes them. The{}
is replaced with the current file name, and\;
ends the command.Find Empty Files or Directories: To find empty files or directories, use the
-empty
option.find /path/to/directory -empty
This finds all empty files and directories.
Find Files by User: You can search for files owned by a specific user using the
-user
option.find /path/to/directory -user username
Example:
find /home -user aditya
This finds files owned by the user
aditya
in the/home
directory.
Commonly Used find Options
-name
: Search for files that match the given name. It is case-sensitive.-iname
: Search for files by name, case-insensitive.-type
: Search for files by type (f
for regular file,d
for directory,l
for symbolic link, etc.).-size
: Search for files by size (+N
for larger than,-N
for smaller than,N
for exactly).-mtime
: Search for files based on the modification time.-perm
: Search for files with a specific permission.-user
: Search for files owned by a specific user.-group
: Search for files owned by a specific group.-exec
: Execute a command on the files found.-delete
: Delete files that match the search criteria.-empty
: Find empty files or directories.-mindepth
: Specify the minimum directory depth for the search.-maxdepth
: Specify the maximum directory depth for the search.
Examples of Combining Multiple Conditions
Find Files Matching Multiple Criteria:
find /path/to/directory -type f -name "*.sh" -size +10M
This finds all
.sh
files that are larger than 10 megabytes.Find Files with Multiple Conditions (AND logic):
find /path/to/directory -type f -name "*.log" -mtime -7
This finds
.log
files modified within the last 7 days.Find Files with OR Conditions:
find /path/to/directory \( -name "*.txt" -o -name "*.log" \)
This finds all
.txt
or.log
files. The parentheses\(
and\)
group the conditions, and-o
stands for OR.Find Files Based on Size and Time:
find /path/to/directory -size +50M -mtime -30
This finds files larger than 50 megabytes that were modified in the last 30 days.
Key Points to Remember
{}
in-exec
: Represents the current file name that is passed to the command being executed.Quoting with Wildcards: When using wildcards (
*
,?
), always quote the pattern to prevent the shell from expanding it prematurely.Danger of
-delete
: The-delete
option deletes files immediately without asking for confirmation, so use it with caution.
Advantages of find Command
Flexible: Supports a wide variety of search criteria (by name, size, date, permissions, etc.).
Efficient: Can search deeply through a directory structure and work recursively.
Executable: Allows for actions (like deletion or modification) to be performed on files found.
Disadvantages of find Command
Complexity: Some of its options, especially with
-exec
, can be difficult for new users to understand.Performance: For very large directories,
find
can be slower compared to more modern alternatives likefd
orlocate
Sed Commands in linux
sed (Stream Editor) is a powerful command-line text manipulation tool in Linux.
It is used for parsing and transforming text, performing find and replace operations, inserting, deleting, or modifying lines in a file without opening it in a text editor.
Basic Syntax
sed [options] 'script' [input-file]
script
: Instructions for how to modify the text (e.g., substitution, deletion, etc.).input-file
: The file where changes are applied. If omitted,sed
reads from standard input.
Common sed
Commands
Substitute (
s
): Thes
command is the most used insed
, allowing you to substitute or replace text.sed 's/old_text/new_text/' filename
- Replaces the first occurrence of
old_text
withnew_text
in each line.
- Replaces the first occurrence of
Example:
sed 's/linux/unix/' file.txt
- Replaces the first occurrence of "linux" with "unix" in each line of
file.txt
.
Replace all occurrences: Add the g
flag to replace all occurrences on each line:
sed 's/old_text/new_text/g' filename
Substitute with Case-Insensitive (
i
): Replace text ignoring case sensitivity.sed 's/linux/unix/Ig' file.txt
- Replaces "linux" with "unix" in a case-insensitive manner, replacing all occurrences.
Delete Lines (
d
): Thed
command deletes specific lines from the input.sed 'Nd' filename
- Deletes the line number
N
. For example, to delete the 3rd line:
- Deletes the line number
sed '3d' filename
Delete lines with a pattern:
sed '/pattern/d' filename
- Deletes all lines matching
pattern
. Example:
sed '/error/d' log.txt
- Deletes all lines containing the word "error".
Print Specific Lines (
p
): Thep
command prints specific lines or ranges of lines.sed -n 'Np' filename
- Prints line number
N
. For example, to print the 2nd line:
- Prints line number
sed -n '2p' file.txt
Print lines matching a pattern:
sed -n '/pattern/p' filename
- Prints only lines that contain
pattern
.
Insert Line Before (
i
): Inserts text before a specific line.sed 'N i\text to insert' filename
- Inserts "text to insert" before line number
N
.
- Inserts "text to insert" before line number
Example:
sed '2i\This is a new line' file.txt
Advantages of sed:
Efficient: It processes input streams line by line, making it fast for large files.
Powerful: Supports complex text manipulation with regular expressions.
Non-interactive: Automates text processing without needing to open files in a text editor.
Scripting: Can be easily used in shell scripts for batch processing.
Disadvantages of sed:
Complex Syntax: Requires understanding of regular expressions and special characters, which may have a learning curve.
Limited Features: While it excels at line-by-line editing, it’s not as feature-rich as other tools like
awk
for more advanced text processing.
Summary of Key sed Commands
Command | Description |
s/old/new/ | Substitutes the first occurrence of old with new . |
s/old/new/g | Replaces all occurrences of old with new . |
Nd | Deletes line number N . |
/pattern/d | Deletes lines matching pattern . |
Np | Prints line number N . |
/pattern/p | Prints lines matching pattern . |
Ni\text | Inserts text before line N . |
Na\text | Appends text after line N . |
Nc\text | Replaces line N with new text. |
/pattern/s/old/new/ | Replaces old with new on lines matching pattern . |
Different Between grep , awk And find Command :
Aspect | grep | awk | find |
Purpose | Searches for specific patterns within files or output. | A powerful text processing tool for pattern scanning and data extraction. | Searches for files and directories based on criteria. |
Search Scope | Searches through the contents of files line by line. | Processes and manipulates text from files or standard input. | Recursively searches for files or directories. |
Use Case | Pattern matching within file content. | Field-based text processing and reporting. | Locating files and directories by name, size, type, etc. |
Syntax | grep [options] pattern [file] | awk 'pattern {action}' [file] | find [path] [options] [expression] |
Pattern Matching | Uses regular expressions for matching patterns. | Uses pattern matching with fields and supports complex expressions. | Matches based on file names, types, permissions, etc. |
File Handling | Works with files by searching inside them. | Processes data in files field by field. | Finds files and directories based on various attributes. |
Field Processing | Cannot process data by fields. | Processes records split into fields (usually space/tab delimited). | Not applicable (focuses on file metadata). |
Common Options | -i (ignore case), -v (invert match), -r (recursive) | -F (field separator), -v (invert match), -f (file for patterns) | -name (name pattern), -type (file type), -exec (execute command) |
Supported Input | Text files and command output. | Text files and standard input. | Directories and file systems. |
Example | grep "error" file.txt | awk '/error/ {print $1}' file.txt | find /home -name "*.txt" |
Best Suited For | Simple text searches and pattern matching. | Complex text processing, extraction, and formatting. | Finding files and directories based on various attributes. |
Key Strengths | Fast and efficient for plain text searches. | Advanced text manipulation with built-in programming features. | Extremely flexible for file system searches. |
Weaknesses | Limited text processing capabilities. | More complex syntax than grep and can be slower on large files. | Slower for simple file searches compared to alternatives like locate . |
Recursion | Yes (with -r or -R option for recursive search). | No recursion for directory trees. | Yes, searches through directories recursively by default. |
Summary:
grep
: Best for simple, fast searches for specific text patterns inside files.awk
: Ideal for field-based text processing, extraction, and formatting.find
: Focuses on searching the file system for files and directories based on various attributes.
Conclusion
In Linux, grep
, awk
, find
, and sed
are essential command-line tools used for text processing and file manipulation. Each command has a specific role and offers unique capabilities, making them indispensable for various tasks.Together, these commands form a powerful suite of tools for handling text and file operations in Linux, enabling users to work efficiently with data, manipulate files, and automate system administration tasks. Each command brings its own strengths to the table, offering versatility and precision for specific tasks, making them indispensable for anyone working on the Linux command line.
Subscribe to my newsletter
Read articles from Aditya Gadhave directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
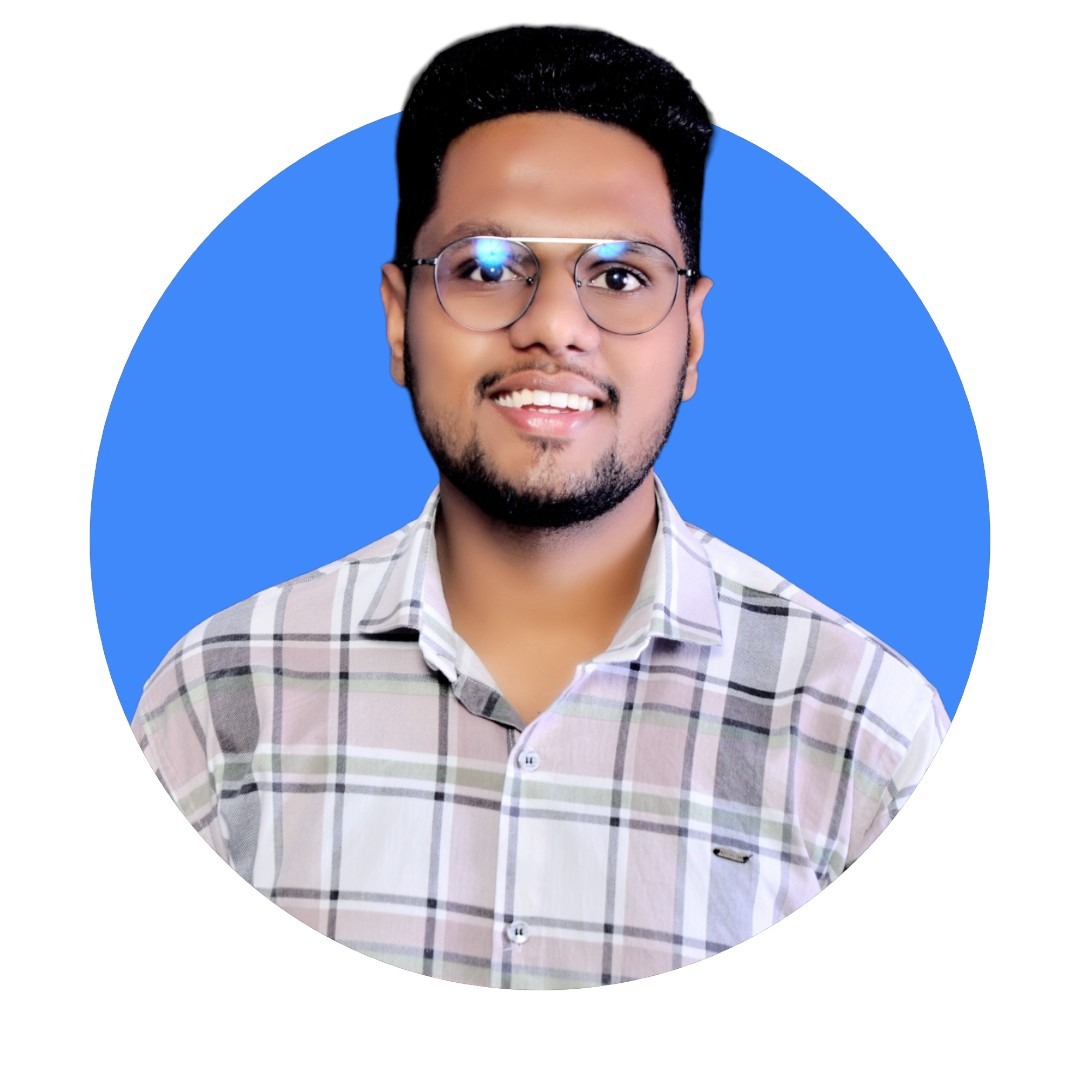
Aditya Gadhave
Aditya Gadhave
👋 Hello! I'm Aditya Gadhave, an enthusiastic Computer Engineering Undergraduate Student. My passion for technology has led me on an exciting journey where I'm honing my skills and making meaningful contributions.