How to Generate a Browser Fingerprint in Angular
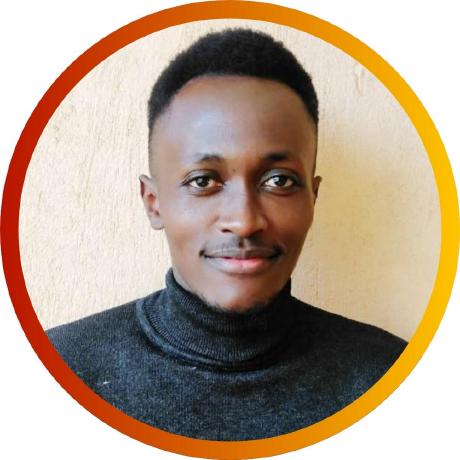
Table of contents
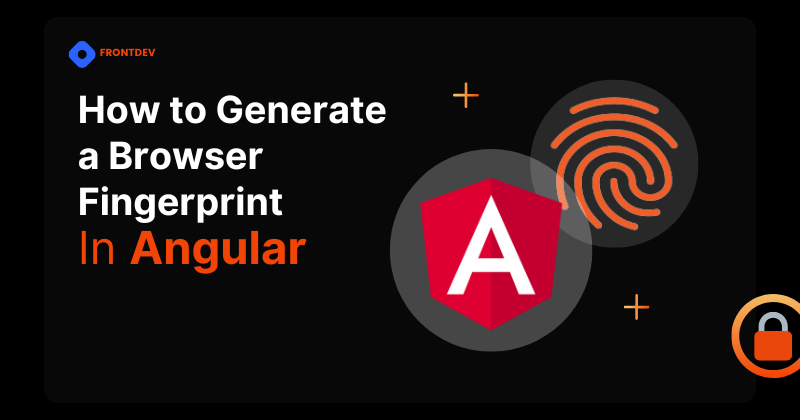
In an era where digital identity and security are paramount, many applications still rely on outdated and easily circumvented user authentication and tracking methods. This technical gap exposes both users and providers to several security vulnerabilities. Enter browser fingerprinting technique designed to improve security protocols without compromising privacy. It leverages unique combinations of browser and device characteristics, offering a better alternative to traditional tracking mechanisms.
But why browser fingerprinting?
Websites can use fingerprints as an additional layer of security, making it harder for fraudsters to impersonate legitimate users even if they have stolen login credentials.
It offers a solution that respects user privacy while providing the precision required for secure authentication and user tracking.
It addresses the inherent weaknesses of cookies and session-based tracking and adapts to the evolving nature of web usage, where users demand security and convenience without compromise.
In this article, you’ll learn the practical application of integrating Fingerprint's browser fingerprinting with Angular. You’ll walk through setting up a simple Angular app, improve user authentication with browser fingerprinting, and ensure your application's security measures are robust and respectful of user privacy.
Browser Fingerprinting in Angular
Consider you're building an online quiz platform that limits users to one submission per quiz to ensure fairness and accuracy in scoring. A savvy user might attempt to bypass this restriction by registering multiple times with different email addresses.
Angular provides a robust client-side environment that can effectively address this challenge through the integration of browser fingerprinting technology. Unlike server-side languages, which are limited in direct interaction with the client's browser, Angular enables the implementation of Fingerprint's browser fingerprinting directly in the user's browser. This approach improves your application's security and prevents users from making multiple submissions by creating numerous accounts.
In this tutorial, you’ll learn how to incorporate Fingerprint Pro into your Angular application to generate unique browser fingerprints. We'll also compare this method against traditional approaches such as session tracking, HTTP cookies, and IP address tracking.
Prerequisites
Familiarity with Angular, including the ability to work with TypeScript and Angular CLI.
A basic understanding of web security concepts.
Development Environment: Node.js, SQLite, Angular CLI, and
npm
(oryarn
) installed on your system.Access to Fingerprint's SDK requires creating a free account on Fingerprint's website.
Project Setup
To follow along with this part of the tutorial, you can find the complete code tutorial on GitHub.
Please note this tutorial uses Node.js (Express) for the backend, SQLite for the database, and bcrypt for password hashing. You’ll need to have Node.js and SQLite installed in your operating system before you can clone and use this tutorial).
The project structure is divided into two sections:
Backend - Node.js (Express) and SQLite
Frontend - Angular
Here’s what the project looks like:
After cloning the project, proceed to install project dependencies by running the command:
npm install
You can create a new database (SQLite) or use the existing one already in the project (find it in the server.js
file). To create a new database, run the following command in your terminal:
sqlite3 <name>.db
(Replace <name> with your ideal database name)
Next, run the following query to create a table with an ID, username, and password field.
CREATE TABLE <name> *Replace <name> with your ideal name*
id INTEGER PRIMARY KEY AUTOINCREMENT,
username TEXT UNIQUE,
password TEXT
);
You are now ready to test account registration. Since you have a backend and a frontend, you’ll need to run them differently in your IDE (Integrated Development Environment).
For the front-end, you’ll need to run ng serve –open
, which opens your Angular app on port 4200
. Open the browser and navigate to the following link: http://127.0.0.1:4200/signup
.
Your screens should look like this:
Start the Node.js server on port 3000
.
You can create a new account, which will redirect you to the login page. Key in the details and proceed to the welcome screen. Your screen should look like this:
In the next section, you’ll learn how to integrate Fingerprint in an Angular app.
Integrating Fingerprint for Browser Fingerprinting
To use Fingerprint Pro Angular SDK in your Angular application, sign up for a Fingerprint Pro account. You can sign up for a free account, which gives you a 14-day trial. In addition, you need to have the following dependencies installed in your system:
TypeScript >=4.6
Node 16+
Angular 13+
Next, you’ll need to add Fingerprint Pro Angular SDK as a dependency to your project using npm
or yarn
.
npm install @fingerprintjs/fingerprintjs-pro-angular
yarn add @fingerprintjs/fingerprintjs-pro-angular
After dependency installation, head back to your Fingerprint dashboard, navigate to App Settings and copy your API Keys
.
Once you have your API Key, configure the SDK within your Angular application. This typically involves adding it to your app module and configuring it with your Fingerprint Pro API key. In your Angular module app.module.ts
, add FingerprintjsProAngularModule.forRoot()
to the imports sections and initialize it with your API key. Don’t forget to add FingerprintjsProAngularModule
in your imports
section.
With the SDK configured, you can use it within your components to generate and retrieve fingerprints. Inject the Fingerprint Pro service into your components to access its functionality.
Here’s how to implement this in the Angular application in the signup.component.ts
file. Below is how the file looks before injecting a service into the component.
Integrating Fingerprint Pro to capture the user's fingerprint during the signup process involves a few additional steps within the onSubmit method
. You'll want to obtain the fingerprint ID right before or as part of your signup process and then send this ID along with the username and password to your backend for user records. Here's the adjusted onSubmit
method that includes Fingerprint Pro integration:
import { Component, OnInit } from '@angular/core';
import { FormGroup, FormControl, Validators } from '@angular/forms';
import { AuthService } from '../auth.service';
import { Router } from '@angular/router';
import { FingerprintjsProAngularService } from '@fingerprintjs/fingerprintjs-pro-angular';
@Component({
selector: 'app-signup',
templateUrl: './signup.component.html',
styleUrls: ['./signup.component.scss']
})
export class SignupComponent implements OnInit {
signupForm: FormGroup = new FormGroup({});
signupSuccess = false;
constructor(private authService: AuthService, private router: Router, private fingerprintService: FingerprintjsProAngularService) {}
ngOnInit() {
this.signupForm = new FormGroup({
'username': new FormControl(null, Validators.required),
'password': new FormControl(null, [Validators.required, Validators.minLength(6)])
});
}
async onSubmit() {
const username = this.signupForm.value.username;
const password = this.signupForm.value.password;
try {
// Await the promise returned by getVisitorData()
const visitorData = await this.fingerprintService.getVisitorData();
console.log(`Fingerprint ID: ${visitorData.visitorId}`);
this.authService.signup(username, password, visitorData.visitorId).subscribe(
() => {
this.signupSuccess = true;
this.router.navigate(['/login']);
},
error => {
// ... error handling ...
}
);
} catch (error) {
// Handle error in obtaining the fingerprint ID
console.error('Error obtaining fingerprint data', error);
}
}
}
Here’s an explanation of what’s happening in the code above.
Through the
fingerprintService.getVisitorData()
call, we asynchronously obtain the visitor's browser fingerprint data. Usingawait
ensures that the code execution pauses until the fingerprint data is retrieved. ThevisitorData
object includes a visitor, which serves as a unique identifier for the user's browser.With the
username
,password
, andvisitorId
(the browser fingerprint ID), you call theauthService.signup()
method. This method handles the registration logic, which now includes sending the browser fingerprint ID alongside the user credentials.
You can learn more about using Fingerprint Pro Angular SDK in the SDK's GitHub repository or read our documentation.
Server-side Validations and Security Considerations
Proper server-side validations and security considerations are important to leverage Fingerprint's Pro benefits. The goal here is to ensure that the data collected is used effectively and handled in a manner that respects user privacy and complies with global data protection regulations.
Upon receiving the browser fingerprint ID and the user's credentials at the backend, validate each registration to ensure its integrity and uniqueness. This involves:
Check that the fingerprint ID received is valid and not previously associated with another user account. This prevents duplicate accounts and fraudulent sign-ups.
Implementing rate limiting to detect and mitigate potential abuse, such as repeated sign-up attempts. Analyzing behavior patterns in conjunction with fingerprint IDs can also highlight suspicious activities.
Advantages of using Fingerprint
Fingerprint is a platform that specializes in advanced device fingerprinting with a 99.5% accuracy. This involves collecting and analyzing a unique set of data points about a user's device (browser, operating system, screen size, fonts, plugins, etc.) to create an identifying “fingerprint.”
Fingerprint Pro poses several distinct advantages over traditional user tracking methods. It’s easy to use and implement, regularly updated, and complies with modern fingerprinting best practices.
Fingerprinting vs. Session Tracking
Session tracking maintains a server-side record of each user's interactions with a web application during a single session. This often involves assigning a unique session ID to each user when they first access the site, which is stored on the client side using cookies. This session ID persists throughout their interaction with the site.
The main limitation of session tracking is its reliance on the user's session remaining active and the cookie's persistence. Users clearing their cookies or using incognito mode can disrupt session continuity. Browser fingerprinting prevails by offering a method of user identification that does not rely on session continuity or cookies, ensuring users can be identified across sessions and even if they take measures to clear their browsing data.
Fingerprinting vs. HTTP Cookies
Cookies store data on a user’s device, and websites use them to track user sessions, preferences, and personalized experiences. But there’s a limitation with HTTP Cookies. Generally, cookies are session-specific and can be easily deleted or blocked by users. Privacy regulations like GDPR and CCPA have imposed strict rules on their use.
Browser fingerprinting prevails over this limitation by:
Persistence: Fingerprinting remains effective beyond sessions, even if cookies are deleted. Cross-Browser: Fingerprinting works across browsers, whereas cookies are tied to a specific browser.
Fingerprinting vs. IP Tracking
IP tracking identifies and tracks users based on their Internet Protocol (IP) address. It's used for geolocation, security, and personalizing user experiences based on location.
The primary limitation of IP tracking is its lack of granularity and the fact that IP addresses can change (through VPN use, dynamic IP allocation, etc.) or be shared among multiple users. Browser fingerprinting provides a more precise method of identification, as it combines multiple attributes to create a unique identifier for each user unaffected by changes in IP address or network conditions.
Conclusion
You’ve learnt how to integrate Fingerprint's browser fingerprinting technology in an Angular application. Such an integration improves the security of your application by providing a unique and persistent identifier for each user. Additionally, it offers a seamless user experience that is unaffected by the traditional limitations of cookies and session management.
The advantages of using Fingerprint over conventional tracking methods in your Angular application have been highlighted, demonstrating the reliability of Fingerprint in curbing fraudulent activities.
Subscribe to my newsletter
Read articles from Kelvin Mburu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
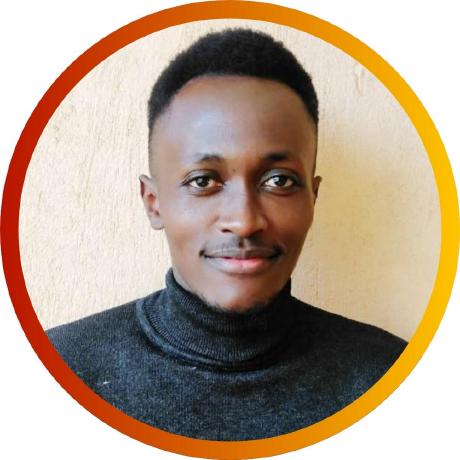
Kelvin Mburu
Kelvin Mburu
Lifelong Learner | Front-End Engineer | Code x Coffee