WILT - Garbage Collection in the JVM: A Modern Deep Dive
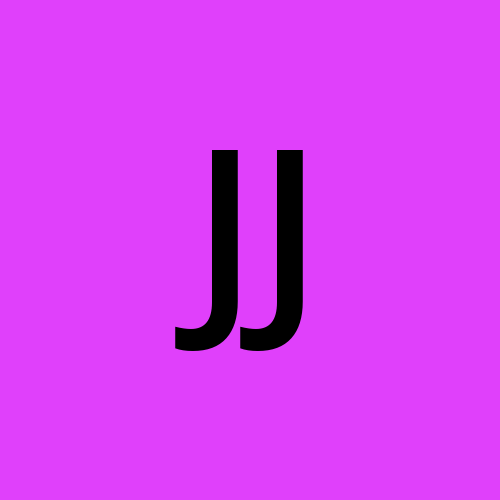
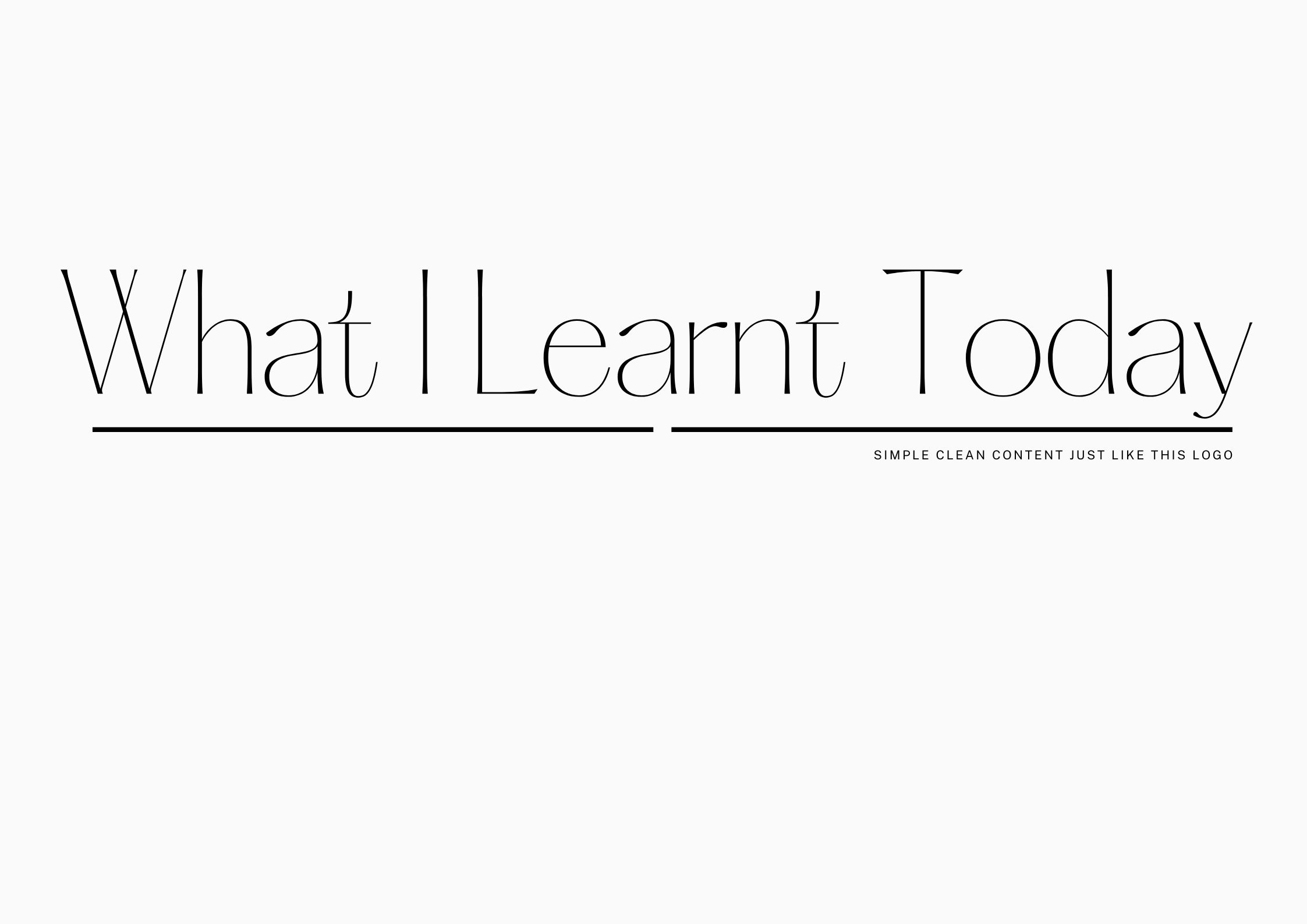
When writing code in high-level languages like Java, one of the biggest advantages we enjoy is automatic memory management. Developers don’t have to worry about manually allocating or freeing memory, thanks to a behind-the-scenes hero called Garbage Collection (GC). This seemingly simple concept has evolved dramatically over the years to keep pace with modern applications, which demand both high performance and efficient memory management.
But what exactly is Garbage Collection, and why has it become so sophisticated? What happens under the hood in the JVM when it comes to memory? Let’s dive deep into this fascinating topic, break down the complexity, and explore how the JVM keeps your application running smoothly by collecting and managing memory with precision.
What Is Garbage Collection (GC)?
Garbage Collection is essentially the process of reclaiming memory that is no longer in use by an application. Imagine you are running a program that continuously creates objects — eventually, some objects will no longer be needed. These objects become “garbage” because they are not being referenced anymore. Garbage Collection ensures that such unused objects are cleaned up, freeing memory for new objects.
However, this process is not as simple as it sounds. In a real-world scenario, memory management is much more complicated because modern applications have large heaps, complex object graphs, and high-throughput requirements. That’s where the magic of GC algorithms comes into play.
The JVM Memory Model: Understanding the Heap
Before diving into GC itself, let’s first understand the JVM memory model. The JVM heap, where object allocation happens, is divided into distinct regions:
Young Generation:
This is where all new objects are allocated. The young generation is further split into three areas:
Eden Space: This is the primary area where new objects are created.
Survivor Spaces (S0 and S1): These are two smaller regions where objects are moved (or “promoted”) after surviving a collection in Eden.
Old Generation (Tenured Generation):
- Objects that have lived long enough in the young generation are eventually moved to the old generation. The old generation holds long-lived objects and is typically much larger.
Permanent Generation (Metaspace in Java 8 and later):
- This region stores metadata like class definitions and method information, as opposed to regular objects. With the introduction of Java 8, PermGen was replaced by Metaspace, which dynamically adjusts its size.
GC Phases: How the Garbage Collection Works
The Garbage Collection process works in cycles. Let’s break down the key phases in which a typical GC process operates in the JVM.
1. Mark Phase
This is the first phase of the garbage collection process. The JVM identifies which objects in memory are still “alive” (i.e., referenced by the application). To do this, it starts from a set of root objects, like static variables or thread-local variables, and recursively marks all objects that are reachable from these roots.
The idea is simple: if an object is reachable, it is alive; otherwise, it is garbage.
2. Sweep Phase
Once the mark phase completes, the JVM knows which objects are still being used. The next task is to remove the ones that are not marked. This is where the sweep phase comes in. The GC will simply free up the memory occupied by unreferenced objects.
However, there’s an inherent inefficiency here: over time, this creates fragmented memory, much like defragmenting a hard drive. We’ll address how modern GCs handle this issue shortly.
3. Compacting Phase (Optional)
To prevent fragmentation, some garbage collectors use a compacting phase. In this phase, after sweeping away the garbage, the collector will move the remaining live objects together in contiguous memory blocks. This not only prevents fragmentation but also speeds up object allocation for future requests, as there is a larger contiguous chunk of memory available.
Types of Garbage Collectors: Algorithms of the Trade
Java provides several different GC algorithms, each suited for different use cases. Depending on your application’s needs, the JVM can employ different strategies to achieve optimal performance.
1. Serial Garbage Collector (Single-threaded, stop-the-world)
The Serial GC is one of the simplest garbage collectors. It works in a single thread and performs stop-the-world pauses for both the young and old generations. This means the application is paused while GC occurs. While this sounds harsh, it’s suitable for small applications or low-memory environments where the overhead of managing multi-threaded collections isn’t necessary.
Pros:
Simple and efficient for single-threaded environments
Low memory overhead
Cons:
- Full application pause during garbage collection
2. Parallel Garbage Collector (Throughput-focused)
The Parallel GC is designed to be multi-threaded and works in parallel to take advantage of multiple CPU cores. Its focus is on maximizing throughput by reducing the overall time spent in garbage collection, even if this means slightly longer pause times.
Pros:
High throughput due to parallelism
Suitable for applications where pause times are not critical
Cons:
- Not ideal for latency-sensitive applications
3. CMS (Concurrent Mark-Sweep, Low-latency)
The CMS collector is focused on minimizing pause times and works concurrently with the application. Unlike Serial and Parallel GC, which use stop-the-world pauses, CMS allows most of the garbage collection work (marking and sweeping) to happen concurrently, without pausing the entire application.
Pros:
Low-latency GC, better for real-time applications
Non-blocking most of the time
Cons:
Requires more CPU resources
Does not handle memory fragmentation as effectively, leading to “floating garbage”
4. G1 Garbage Collector (Balanced and Modern)
G1 (Garbage First) is one of the most modern collectors in the JVM and is the default since Java 9. It’s designed to offer a balance between high throughput and low latency. G1 splits the heap into regions and collects garbage from these regions based on a “first” priority — hence the name.
G1 uses a combination of young and old generation collections in a way that reduces both pause times and fragmentation. It also performs compaction as part of its process, so memory stays contiguous over time.
Pros:
Tunable for both low latency and high throughput
Efficient in large heaps with many live objects
Reduces fragmentation automatically
Cons:
- Slightly higher overhead than simpler collectors like Parallel GC
GC Tuning: Tweaking the Performance
Garbage Collection tuning is an essential aspect of optimizing Java applications, especially in production environments. There are several JVM flags you can use to fine-tune the behavior of the garbage collector. Some common parameters include:
-Xms
and-Xmx
: Minimum and maximum heap size, respectively.-XX:+UseG1GC
: Enable the G1 garbage collector.-XX:MaxGCPauseMillis
: Allows you to set a soft goal for maximum GC pause times.
Tuning involves balancing between throughput (how much work the application does) and latency (how quickly the application responds). Reducing the pause times may impact throughput and vice versa.
Conclusion: The Unsung Hero of Java
Garbage Collection is a powerful, dynamic process that keeps Java applications running smoothly without burdening developers with manual memory management. However, it’s not just a set-it-and-forget-it feature. With different collectors available, each optimized for specific workloads, understanding GC helps developers tune their applications for better performance, particularly as they scale.
From simple algorithms like Serial GC to the advanced G1 collector, the JVM’s garbage collection system has evolved to handle modern application demands, ensuring both high throughput and low latency and yeah this is What I Learnt Today
Subscribe to my newsletter
Read articles from Jival Jenson directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
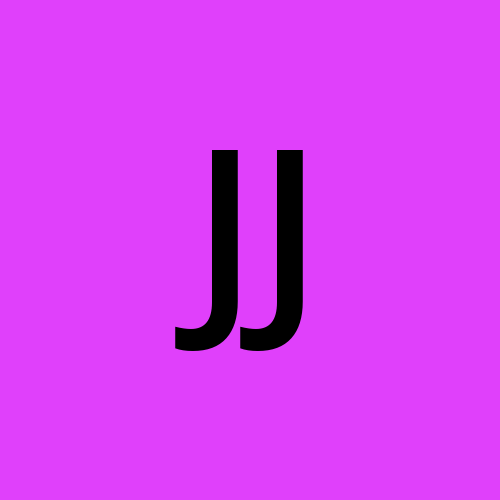