Building Resilient Software: A Deep Dive into OOP Principles, SOLID Design, and Beyond
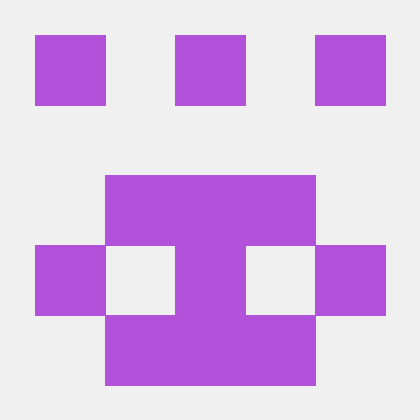
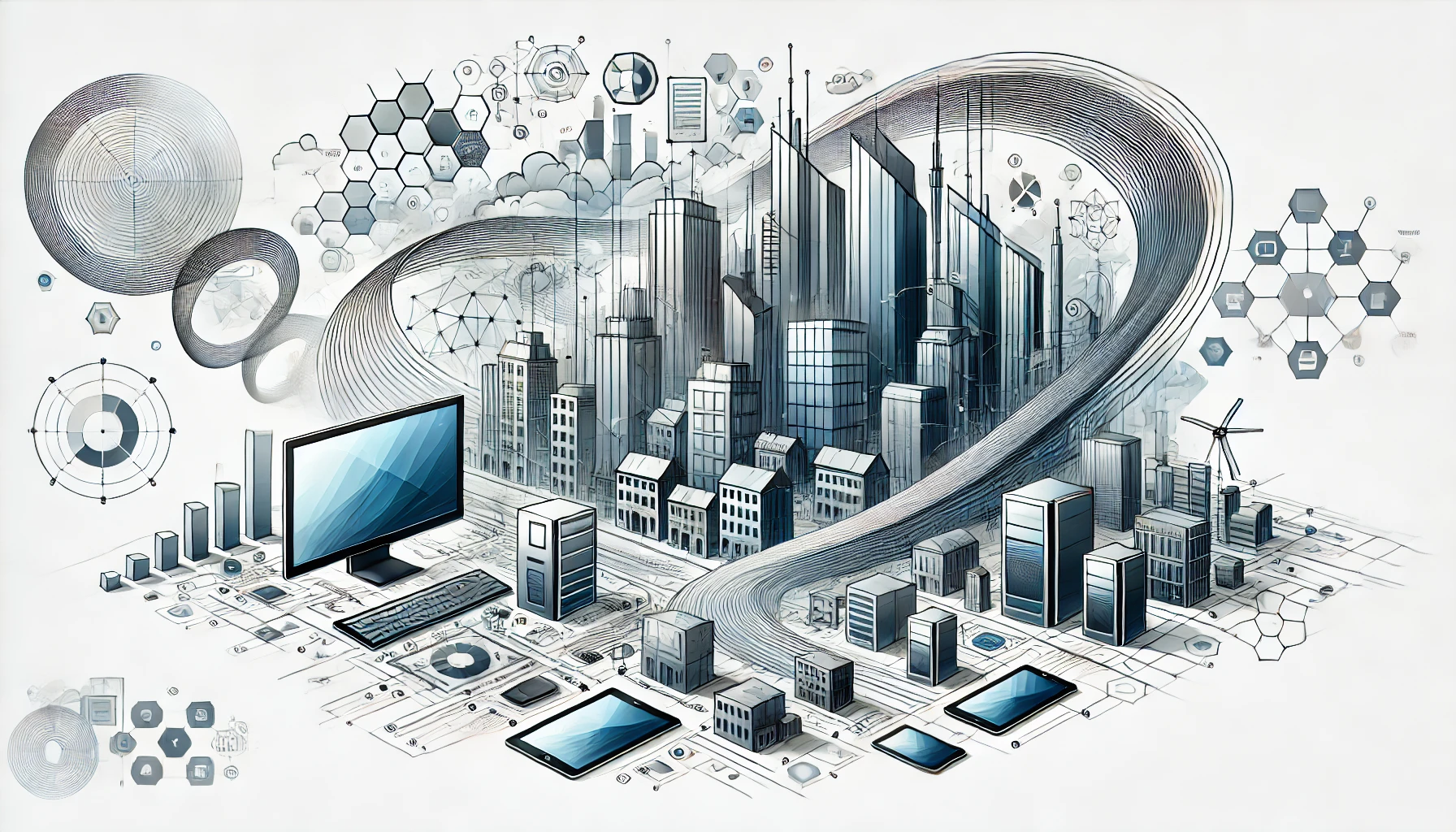
In today's rapidly evolving digital landscape, where applications grow increasingly complex and data-intensive, the importance of solid software architecture principles cannot be overstated. As a software developer who has witnessed the transformation of applications in the internet era, I believe that understanding and implementing Object-Oriented Programming (OOP) principles, SOLID design guidelines, and design patterns is not just good practice – it's essential for creating sustainable, scalable software solutions.
The Foundation: Object-Oriented Programming Principles
Encapsulation: The Art of Information Hiding
Encapsulation is like creating a secure vault for your data. It bundles related data and methods together while restricting access from the outside world. By using private fields and public methods (getters and setters), we maintain data integrity and protect our objects from unauthorized access or modification.
Abstraction: Simplifying Complexity
Think of abstraction as the TV remote control – you press a button to increase volume without needing to understand the complex electronic circuitry behind it. In programming, abstraction allows us to hide complex implementations behind simple interfaces, making our code more manageable and user-friendly.
Inheritance: Building on Existing Foundations
Inheritance enables us to create new classes based on existing ones, promoting code reuse and establishing hierarchical relationships. Like how a car inherits basic vehicle properties but adds its specific features, inheritance allows us to extend and specialize our code efficiently.
Polymorphism: Many Forms, One Interface
Polymorphism provides flexibility by allowing us to treat different objects through a common interface. Whether it's method overriding (runtime polymorphism) or method overloading (compile-time polymorphism), this principle enables more flexible and maintainable code structures.
SOLID: The Blueprint for Maintainable Software
Single Responsibility Principle (SRP)
Each class should have only one reason to change. This principle promotes focused, maintainable code by ensuring that classes handle only one specific functionality.
Open-Closed Principle (OCP)
Software entities should be open for extension but closed for modification. This principle encourages us to design our systems so that new functionality can be added without changing existing code.
Liskov Substitution Principle (LSP)
Objects of a superclass should be replaceable with objects of its subclasses without breaking the application. This ensures that inheritance hierarchies remain robust and intuitive.
Interface Segregation Principle (ISP)
Clients should not be forced to depend on interfaces they don't use. By keeping interfaces focused and specific, we create more flexible and maintainable systems.
Dependency Inversion Principle (DIP)
High-level modules should not depend on low-level modules; both should depend on abstractions. This principle promotes loose coupling and makes our systems more adaptable to change.
The Power of Design Patterns
Design patterns provide proven solutions to common software design problems. They can be categorized into:
Creational Patterns (e.g., Singleton, Factory)
Structural Patterns (e.g., Adapter, Decorator)
Behavioral Patterns (e.g., Observer, Strategy)
These patterns help us create more flexible, reusable, and maintainable code by leveraging best practices developed by experienced developers.
Why These Principles Matter
In my view, these principles are analogous to social laws that govern our society. Just as civilization has advanced through collective adherence to rules and organization, software development thrives when we follow established principles and patterns. Here's why:
Maintainability: Well-organized code following these principles is easier to understand and modify.
Scalability: Applications built on solid principles can grow and adapt to new requirements more efficiently.
Collaboration: When teams follow these principles, they create a common language and understanding that facilitates better collaboration.
Quality: These principles help prevent common pitfalls and promote robust, reliable software solutions.
Conclusion
As our digital world continues to evolve and applications become more complex, following these principles becomes increasingly crucial. They're not just theoretical concepts – they're practical tools that help us build better software. Just as human civilization progressed through organization and adherence to principles, our software ecosystem advances when we embrace and apply these fundamental concepts.
The next time you start a new project or refactor existing code, remember that these principles are your allies in creating maintainable, scalable, and robust applications. In the fast-paced world of software development, they serve as our compass, guiding us toward better architectural decisions and more sustainable solutions.
Subscribe to my newsletter
Read articles from Sri Siddhardha Kurra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
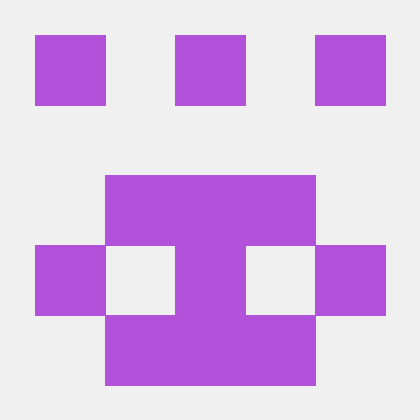