Next.js Server Components and the "use" Hook: Is It Like a Remix Loader?

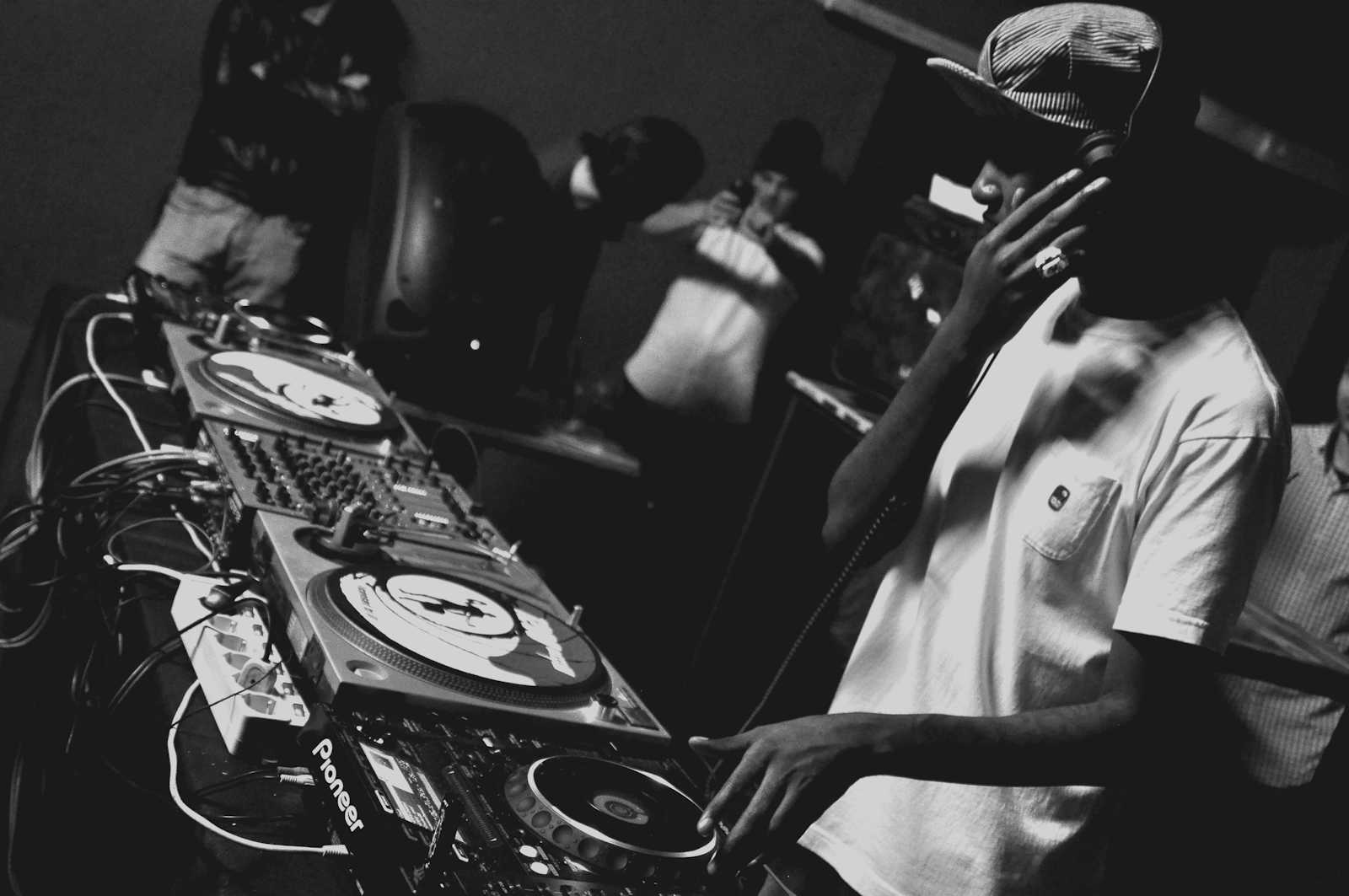
The web development landscape is constantly evolving, and frameworks like Next.js and Remix are leading the charge with new ways to fetch data on the server. Recently, with the introduction of React Server Components (RSC) and the use
hook, Next.js has adopted a new approach to data fetching. If you're familiar with Remix loaders, you might be wondering how these two compare.
In this blog post, we'll explore the similarities and differences between the new Next.js pattern with RSC and the Remix loader approach.
Similarities: Next.js RSC and Remix Loaders
Both Next.js RSC and Remix loaders aim to simplify data fetching, making it server-centric for better performance and user experience. Here are the key similarities:
Server-Side Data Fetching
Both frameworks prioritize server-side data fetching. In Remix, this is done through loaders, while in Next.js RSC, data is fetched directly in the server component.
The server-centric approach reduces the JavaScript needed on the client, improving page load times and performance.
Declarative Data Fetching
- Both frameworks make data fetching declarative. You define how to fetch the data, and the framework handles it before rendering the component.
Differences: A Closer Look
While there are many similarities, Next.js RSC and Remix loaders diverge in their implementation and philosophy of how and where data is fetched and used.
1. Location of Data Fetching
Remix Loaders: In Remix, data fetching happens in a separate function called a loader. Loaders are typically defined alongside the route component, and Remix takes care of calling this function before rendering the UI.
Next.js RSC: In Next.js with React Server Components, the data fetching happens inside the server component itself using the
use
hook or simply by awaiting a promise. There is no separate loader concept, making data fetching more inline and integrated within the component logic.
2. The use
Hook vs. Loaders
Remix Loaders: The loader functions in Remix are responsible for fetching all the data needed for a route. You can return multiple pieces of data as an object, making it easy to provide everything needed for that route's UI.
Next.js
use
Hook: With Next.js RSC, theuse
hook allows you to call async functions directly within the server component. This hook helps in suspending the rendering of the component until the data is ready. It enables a more modular approach, allowing you to split data fetching responsibilities across multiple server components instead of having a central loader.
3. Granularity of Data Fetching
Remix Loaders: Loaders are typically used at the route level, providing all the data needed for that specific route.
Next.js RSC: Data fetching with Next.js is more granular, happening at the component level. Each server component can be responsible for its own data, making it possible to divide data-fetching tasks among different components rather than one central loader.
Example Comparison
To illustrate the difference, let's look at an example for both Remix and Next.js RSC.
Remix Loader Example
// Remix loader function
export const loader = async () => {
const data = await fetch('https://api.example.com/data');
return data.json();
};
// Remix component
export default function Component() {
const data = useLoaderData(); // use data from loader
return <div>{JSON.stringify(data)}</div>;
}
In this example, the loader function is defined separately, and it fetches the data for the route. The component then uses the useLoaderData()
hook to access the data.
Next.js RSC Example
// Next.js RSC
async function getData() {
const res = await fetch('https://api.example.com/data');
return res.json();
}
// Server Component
export default async function Page() {
const data = use(getData()); // use hook to fetch data directly
return (
<div>
<h1>Data:</h1>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
}
In this Next.js RSC example, the use
hook is used to fetch the data directly within the component, without relying on a separate function to handle the data fetching.
Summary
Remix loaders offer a route-focused approach, separating the data fetching from the rendering logic. This approach is clean and centralizes the data fetching logic for each route.
Next.js RSC with the
use
hook allows for a more inline and modular approach, where each server component can handle its own data fetching. This integration provides a more streamlined developer experience at the component level.
In practice, both approaches have their benefits and trade-offs. Remix loaders provide a clear separation of concerns, while Next.js RSC with use
gives more flexibility at a component level, making it easier to handle data in smaller, reusable units.
As these frameworks continue to evolve, developers will find that both Remix and Next.js are moving towards reducing client-side overhead, making server-side rendering and server-side data fetching more effective. Whether you prefer the Remix loader pattern or the new Next.js RSC with use
, it ultimately depends on your project needs and the granularity of control you require for data fetching.
Which Approach Should You Use?
If you're building a project that benefits from centralized, route-level data fetching, Remix loaders might be the better choice. If you prefer a component-driven approach with granular data fetching, Next.js with React Server Components is a great option. Either way, both frameworks are powerful tools in the modern web developer's toolkit.
Subscribe to my newsletter
Read articles from Benjamin Temple directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
