Essential C# If-Else Questions and Answers for HNDIT Students

Table of contents
- Questions
- Answers
- 1. Check if the number is even or odd:
- 2. Check if a number is positive, negative, or zero:
- 3. Check if the age qualifies as an adult:
- 4. Check if a student has passed or failed:
- 5. Print the greater of two numbers:
- 6. Assign a grade based on the score:
- 7. Print the corresponding day of the week:
- 8. Classify temperature:
- 9. Classify BMI:
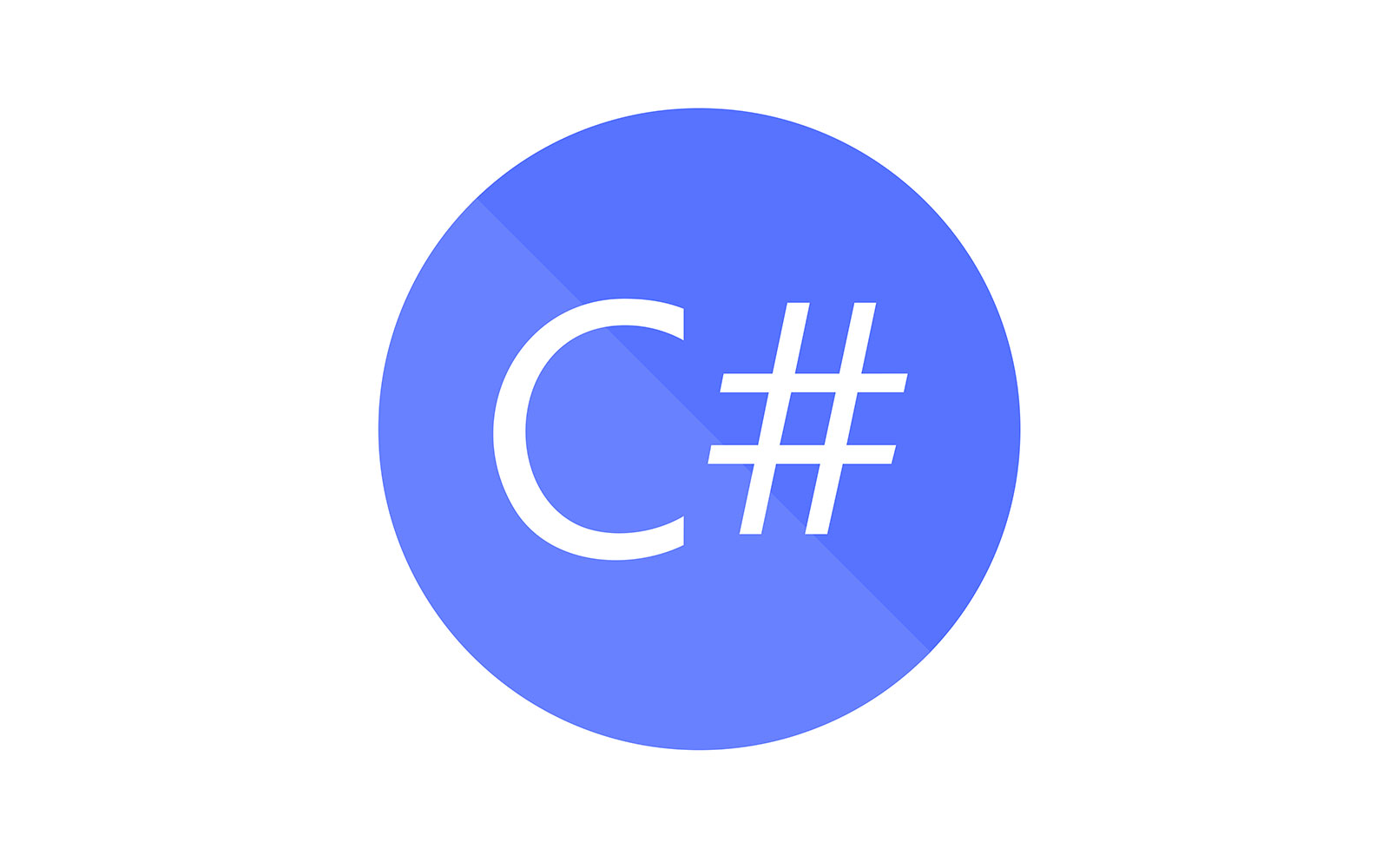
Questions
Write a program that asks the user to enter a number and checks whether the number is even or odd.
Write a program that checks whether a given number is positive, negative, or zero.
Write a program that checks if the entered age qualifies a person as an adult (18 or older).
Write a program that checks if a student has passed or failed based on their score (passing score is 50).
Write a program that takes two numbers as input and prints the greater number.
Write a program that assigns a grade based on the score a user enters. Use the following conditions: Score >= 90: Grade A Score >= 80: Grade B Score >= 70: Grade C Score >= 60: Grade D Score < 60: Fail
Write a program that takes an integer between 1 and 7 and prints the corresponding day of the week.
Write a program that classifies the temperature into categories: Temperature >= 30: Hot Temperature >= 20: Warm Temperature >= 10: Cool Temperature < 10: Cold
Write a program that takes a BMI value as input and classifies it based on the following: BMI >= 30: Obesity BMI >= 25: Overweight BMI >= 18.5: Normal weight BMI < 18.5: Underweight
Answers
1. Check if the number is even or odd:
using System;
class Program {
static void Main() {
Console.Write("Enter a number: ");
int number = int.Parse(Console.ReadLine());
if (number % 2 == 0) {
Console.WriteLine("The number is even.");
} else {
Console.WriteLine("The number is odd.");
}
}
}
2. Check if a number is positive, negative, or zero:
using System;
class Program {
static void Main() {
Console.Write("Enter a number: ");
int number = int.Parse(Console.ReadLine());
if (number > 0) {
Console.WriteLine("The number is positive.");
} else if (number < 0) {
Console.WriteLine("The number is negative.");
} else {
Console.WriteLine("The number is zero.");
}
}
}
3. Check if the age qualifies as an adult:
using System;
class Program {
static void Main() {
Console.Write("Enter your age: ");
int age = int.Parse(Console.ReadLine());
if (age >= 18) {
Console.WriteLine("You are an adult.");
} else {
Console.WriteLine("You are not an adult.");
}
}
}
4. Check if a student has passed or failed:
using System;
class Program {
static void Main() {
Console.Write("Enter the score: ");
int score = int.Parse(Console.ReadLine());
if (score >= 50) {
Console.WriteLine("You have passed.");
} else {
Console.WriteLine("You have failed.");
}
}
}
5. Print the greater of two numbers:
using System;
class Program {
static void Main() {
Console.Write("Enter the first number: ");
int num1 = int.Parse(Console.ReadLine());
Console.Write("Enter the second number: ");
int num2 = int.Parse(Console.ReadLine());
if (num1 > num2) {
Console.WriteLine("The greater number is: " + num1);
} else {
Console.WriteLine("The greater number is: " + num2);
}
}
}
6. Assign a grade based on the score:
using System;
class Program {
static void Main() {
Console.Write("Enter the score: ");
int score = int.Parse(Console.ReadLine());
if (score >= 90) {
Console.WriteLine("Grade A");
} else if (score >= 80) {
Console.WriteLine("Grade B");
} else if (score >= 70) {
Console.WriteLine("Grade C");
} else if (score >= 60) {
Console.WriteLine("Grade D");
} else {
Console.WriteLine("Fail");
}
}
}
7. Print the corresponding day of the week:
using System;
class Program {
static void Main() {
Console.Write("Enter a number (1-7): ");
int day = int.Parse(Console.ReadLine());
if (day == 1) {
Console.WriteLine("Monday");
} else if (day == 2) {
Console.WriteLine("Tuesday");
} else if (day == 3) {
Console.WriteLine("Wednesday");
} else if (day == 4) {
Console.WriteLine("Thursday");
} else if (day == 5) {
Console.WriteLine("Friday");
} else if (day == 6) {
Console.WriteLine("Saturday");
} else if (day == 7) {
Console.WriteLine("Sunday");
} else {
Console.WriteLine("Invalid number. Please enter a number between 1 and 7.");
}
}
}
8. Classify temperature:
using System;
class Program {
static void Main() {
Console.Write("Enter the temperature: ");
int temp = int.Parse(Console.ReadLine());
if (temp >= 30) {
Console.WriteLine("Hot");
} else if (temp >= 20) {
Console.WriteLine("Warm");
} else if (temp >= 10) {
Console.WriteLine("Cool");
} else {
Console.WriteLine("Cold");
}
}
}
9. Classify BMI:
using System;
class Program {
static void Main() {
Console.Write("Enter the BMI value: ");
double bmi = double.Parse(Console.ReadLine());
if (bmi >= 30) {
Console.WriteLine("Obesity");
} else if (bmi >= 25) {
Console.WriteLine("Overweight");
} else if (bmi >= 18.5) {
Console.WriteLine("Normal weight");
} else {
Console.WriteLine("Underweight");
}
}
}
break;
Subscribe to my newsletter
Read articles from Arzath Areeff directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Arzath Areeff
Arzath Areeff
I co-founded digizen.lk to promote online safety and critical thinking. Currently, I’m developing an AI app to fight misinformation. As Founder and CEO of ideaGeek.net, I help turn startup dreams into reality, and I share tech insights and travel stories on my YouTube channels, TechNomad and Rz Omar.