Reading From Solana Network

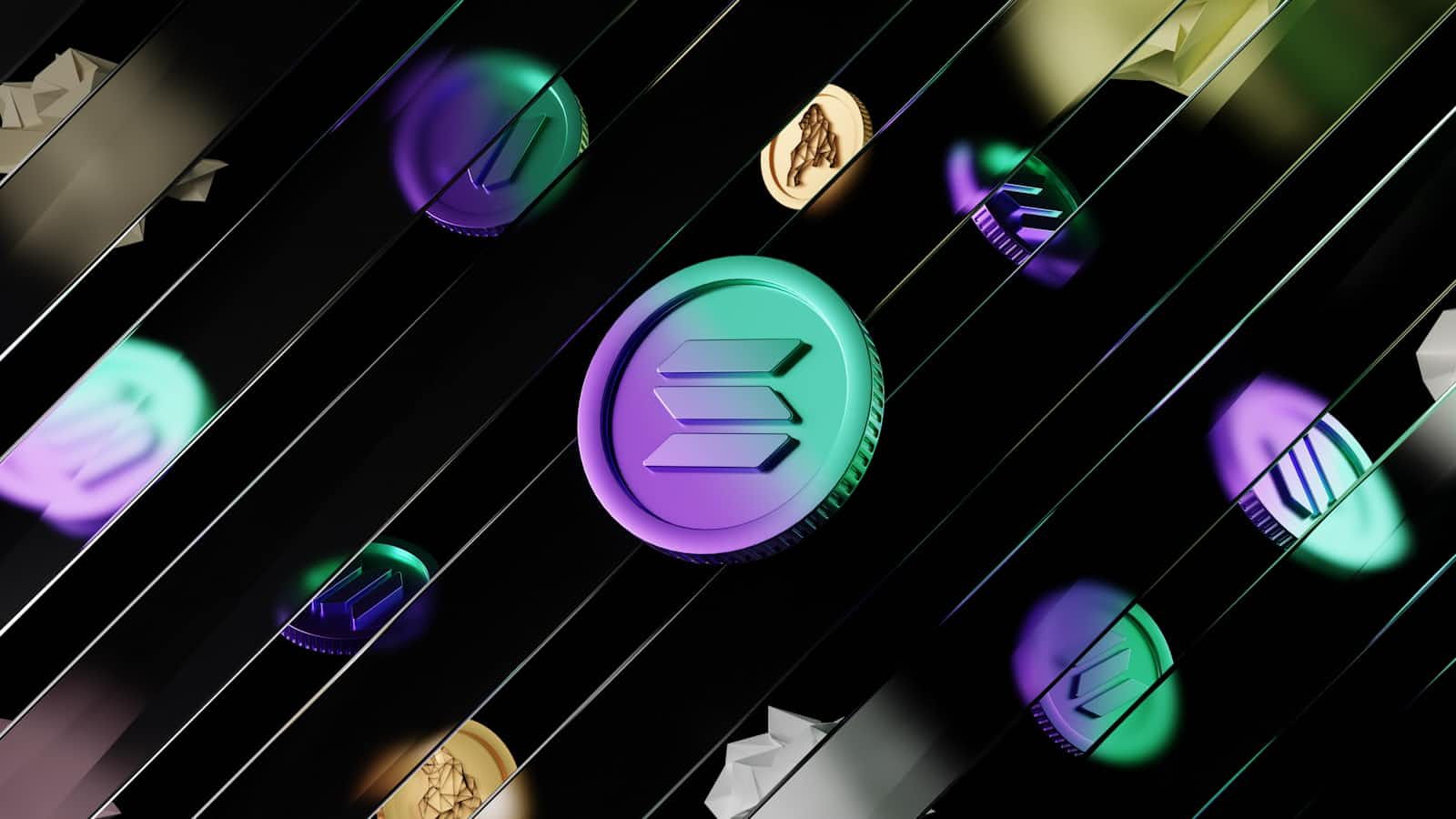
In the neon-lit trenches of the blockchain, where transactions dance on a cryptographic tightrope, lurks a vital skill: Reading the Network. This act, akin to deciphering ancient scrolls in a forgotten language, allows programs to glean valuable insights from the ever-flowing stream of data.
Our intrepid hero, a program written in Rust (a battle-tested language for these digital skirmishes), embarks on a quest to retrieve data from the Solana network.
Equipment Check:
Solana Client: A trusty library, acting as a bridge between our program and the network's intricate protocols. (Think of it as a translator for the blockchain's cryptic tongue.)
Commitment: A unique identifier, akin to a fingerprint, that pinpoints the specific data we seek within the vast network ledger. (Imagine it as a treasure map leading to a specific block of information.)
An Incantation of Code
// Import the Solana client library
use solana_client::rpc_client::RpcClient;
// Connect to a Solana node (think of it as tuning into the network's frequency)
let connection = RpcClient::new("http://localhost:8899"); // Replace with a real node URL
// Define the commitment level (how much confirmation we need for the data)
let commitment = CommitmentConfig::confirmed;
// Specify the commitment we seek (our treasure map)
let blockhash = "deadbeefdeadbeefdeadbeefdeadbeefdeadbeef"; // Replace with a real commitment
// Cast the incantation (send the request to the network)
let result = connection.get_block_with_config(blockhash, commitment).unwrap();
// Decipher the network's response (extract the data)
let data = result.block.unwrap().transactions; // Example: access transaction data
// Rejoice! The data is ours!
println!("Retrieved data from the network: {:?}", data);
Explanation
Import the Solana Client: We enlist the help of the
solana_client
library to interact with the network.Connect to a Node: We establish a connection with a Solana node, a computer that validates transactions and stores the network ledger. (Think of it as a local library branch with access to the blockchain's archives.)
Commitment Level: We define the level of confirmation required for the data. Here,
CommitmentConfig::confirmed
ensures the data has been finalized and won't change.Specify the Commitment: We provide the unique identifier (the treasure map) for the specific data we want to read.
Cast the Incantation: We call the
get_block_with_config
method on the client, sending our request to the network.Decipher the Response: We extract the data from the network's response, typically stored within transactions or blocks.
Victory Lap: We print the retrieved data, a testament to our program's successful foray into the network.
This is just a glimpse into the captivating world of reading from the Solana network. With more advanced techniques, programs can become oracles, prophets of sorts, gleaning information from external sources and feeding it back into the blockchain's intricate ecosystem. The possibilities, like the ever-evolving blockchain itself, are boundless.
Subscribe to my newsletter
Read articles from DAMIAN ROBINSON directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
