What is a Bundler?

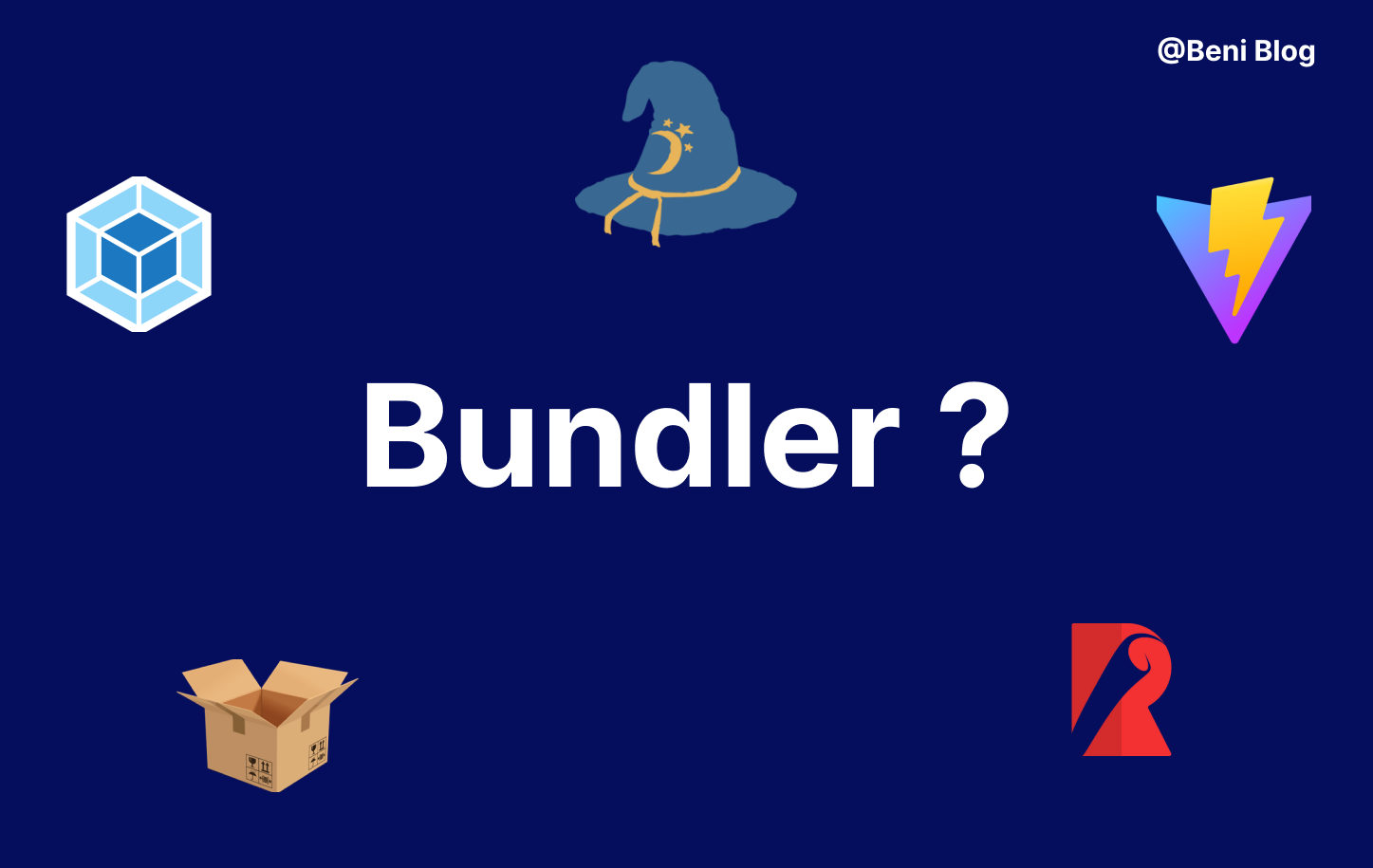
Understanding Bundlers in React :
In simple terms, a bundler is a tool which helps to bundle the entire React project into one single file or few files, which will be then run in the browser. Bundlers are used to improve the application performance, in addition to the reduction of code size, by reducing the number of HTTP requests the browser performs to load content.
Bundlers come with integrated tools that help transpile JSX and modern JS to plain JS which is understandable by the browser this includes syntactical changes for browser compatibility with ES6+ modules. Moreover, it handles style files and transforms them into CSS files which can be then understood by the browser.
How Bundlers Function
To understand how the bundlers work let’s visualize this configuration file for a Webpack bundler :
const path = require('path');
module.exports = {
// Entry point for the bundle (the file that kicks off the app)
entry: './src/index.js',
// Output bundle settings (where the bundled files should go)
output: {
filename: 'bundle.js', // Output filename
path: path.resolve(__dirname, 'dist'), // Directory for bundled output
clean: true, // Clean old files in dist directory before bundling
},
};
The first property is what we will focus on for now. This entry
property tells the bundler where to start the bundling process. which goes as follows:
Step 1: Creating a dependency graph
This part involves going through all the files imported starting from the entry point in a graph-like structure. The bundler starts reading the index.js file, whenever it encounters an import it’s a dependency that should be added to the graph, and then it goes to the dependency in question and adds any dependencies that are linked to this one as well. Here is a simple diagram that illustrates the concept
This diagram represents the resulting dependency graph of an app that has an
index.js
file (the entry point) that calls theApp.js
and that in return call components: Header, Main and Footer which all result in imports, therefore the creation of the structure.Step 2: Module Resolution :
After completing the graph dependency creation, the bundler starts to identify which of the dependencies are third-party libraries coming from the node modules, and which are local files.
Step 3: Transpiling (Not always mandatory)
The process of transpiling happens when we have things that can’t be run directly in the browser like Typescript or JSX in this case, bundlers make use of transpilers like Babel, to get plain javascript understandable and compatible with the browser.
Step 4: Tree Shaking :
This step is where the bundler starts optimizing the output. By removing any unnecessary code such as unused imports.
Note: To make use of the tree-shaking power, it’s essential to work with ES modules in your code, as they have a static structure which makes the imports and exports analyzed and optimized at build time. Also, make sure to avoid side effects in your modules like modifying the DOM, and make sure the package.json file has the property
“sideEffects”
set to false.Step 5: Minification and Compression :
It’s another step to ensure the high quality of resulted code by eliminating any white spaces, and comments as well as compressing files, to make sure the files are sent faster over the network.
Step 6: Code Splitting:
This feature is offered by modern bundlers and it helps you create multiple bundles for each chunk of your application. Which can highly improve load time especially when we are working on big projects. As most react projects are split into routes, a common approach is to bundle each route in its own file and use lazy loading to load only the route that the user navigates to.
// App.js import React, { Suspense, lazy } from 'react'; import { BrowserRouter as Router, Routes, Route, Link } from 'react-router-dom'; // Lazy load components const Home = lazy(() => import('./Home')); const About = lazy(() => import('./About')); const App = () => { return ( <Router> <nav> <Link to="/">Home</Link> <Link to="/about">About</Link> </nav> <Suspense fallback={<div>Please wait loading in progress...</div>}> <Routes> <Route path="/" element={<Home />} /> <Route path="/about" element={<About />} /> </Routes> </Suspense> </Router> ); }; export default App;
Step 7: Output Generation:
After all the processing, transpiling, optimization, and code splitting the bundler generates the final output which goes to the directory specified in the file configuration at the beginning of this section.
Conclusion:
Bundlers play a crucial role in modern web development, particularly in React applications. They streamline the process of preparing code for the browser by bundling multiple files into a single or few files, thereby enhancing performance and reducing load times. By creating a dependency graph, resolving modules, transpiling code, and optimizing through tree shaking, minification, and code splitting, bundlers ensure that the final output is efficient and ready for deployment. Understanding how bundlers function can significantly improve the development workflow and the performance of web applications.
If you found this article helpful, we’d love for you to follow us, like this post, and subscribe to our newsletter for more insightful content. Thank you for your support!
Further Ressources
Subscribe to my newsletter
Read articles from Zainab JINARI directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Zainab JINARI
Zainab JINARI
A full-stack engineer and passionate website designer, experienced in creating projects from scratch. I’m in my 5th year studying Web & Mobile Application Engineering, and I’m also an enthusiast of AI and blockchain technologies. As a full-stack engineering student, I thrive on developing end-to-end solutions, from design to deployment..